Clean Code - The Practical Guide
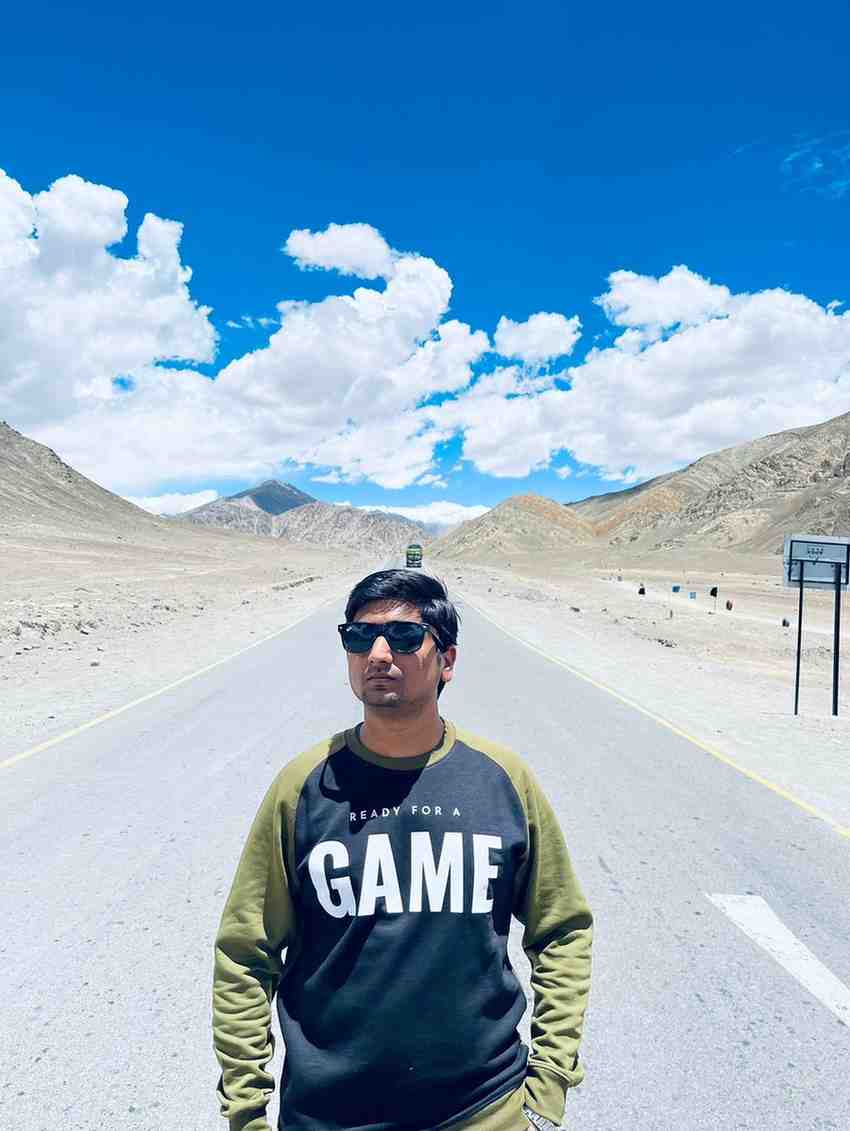

Introduction
In the vast landscape of software development, writing code is not just about instructing a computer to perform a task; it's about crafting a language that communicates with fellow developers, both present and future. Clean code is the art of writing code that is not only functional but also elegant, efficient, and understandable to others.
Imagine a coach flipping through a playbook where each play is neatly arranged, labeled, and easily accessible. This organized playbook is not just a collection of random strategies but a carefully curated guide to success on the field. With every play clearly defined and structured, the team can execute with precision, knowing exactly what to do in any situation.
In the realm of software development, clean code serves as the playbook for developers. Each line of code is akin to a play in the playbook—purposeful, structured, and easily understandable. When the code base is clean and well-organized, developers can navigate through it seamlessly, making changes and enhancements with confidence.
In this blog, we embark on a journey into the realm of clean code, exploring its principles, practices, and significance in modern software development. Whether you're a seasoned developer looking to refine your craft or a newcomer eager to learn the ropes, join us as we delve into the art of writing code that not only works but also stands the test of time. Let's elevate our programming practices and strive for cleaner, more elegant solutions that not only solve problems but also inspire and empower others.
What is clean code?
Clean code refers to source code that is well-organized, easy to read, and easy to understand. It emphasizes clarity and simplicity, making it easier for developers to maintain, modify, and debug. Clean code is not just about functionality; it's about writing code in a way that minimizes complexity and maximizes readability.
Clean code is not just about whether code works; it's primarily about whether it's easy to read and understand. As developers, we spend a significant amount of time reading and understanding code, whether it's our own past code or code written by colleagues. Clean code prioritizes readability and comprehension to enhance productivity and prevent loss of time. It's crucial for maintaining code bases over time and ensuring their longevity. There's no one-size-fits-all solution for writing clean code, but it's essential for effective software development.
Why is clean code important?
Readability and Maintainability:-
Clean code is easier to read and understand, reducing the time and effort required to maintain and modify it. This leads to faster bug fixes, easier feature additions, and smoother code reviews.
Reduced Technical Debt:-
Technical debt accumulates when shortcuts are taken or code quality is compromised in favor of quick fixes or tight deadlines. Clean code reduces technical debt by prioritizing long-term maintainability and reducing the cost of future changes.
Enhanced Collaboration:-
Clean code improves collaboration among developers by providing a common understanding of how the code works and where to find specific functionality. It also reduces the risk of miscommunication and misunderstandings between team members.
Improved Productivity:-
Developers spend a significant portion of their time reading and understanding code. Clean code reduces cognitive overhead and makes developers more productive by allowing them to focus on solving problems rather than deciphering complex code.
Better Quality Software:-
Clean code results in better quality software that is more reliable, easier to test, and less prone to bugs and errors. This leads to higher customer satisfaction and a better overall user experience.
In summary, clean code is essential for building maintainable, scalable, and high-quality software applications. It improves readability, reduces technical debt, enhances collaboration, boosts productivity, and ultimately leads to better software outcomes for both developers and end-users.
Properties of clean code
Readable and Meaningful:-
Clean code should be easy to understand for other developers who might work on it in the future. This means using descriptive names for variables, functions, and classes, as well as writing clear and concise comments where necessary.
Reduces Cognitive Load:-
Clean code minimizes the mental effort required to understand it. This involves breaking down complex tasks into smaller, more manageable components and organizing the code in a logical manner.
Concise and "To the Point":-
Clean code gets straight to the point without unnecessary complexity or verbosity. It eliminates redundant or superfluous code, keeping only what is essential for the functionality.
Avoids Unintuitive Names, Complex Nesting, and Big Code Blocks:-
Clean code uses meaningful and intuitive names for variables, functions, and classes, making it easier to understand their purpose and usage. It also avoids deep nesting of control structures and large code blocks, which can make code harder to follow and maintain.
Follows Common Best Practices and Patterns:-
Clean code adheres to established best practices and design patterns in software development. This includes principles like DRY (Don't Repeat Yourself), SOLID, and others that promote modular, reusable, and maintainable code.
Well-Tested:-
Clean code is thoroughly tested to ensure its correctness and robustness. This involves writing unit tests, integration tests, and other forms of automated testing to validate the code's behavior under various conditions.
Documented When Necessary:-
Clean code includes appropriate documentation to explain its purpose, usage, and any important considerations for developers working with it. This documentation should be clear, concise, and kept up-to-date as the code evolves.
Consistent Formatting and Style:-
Clean code follows a consistent formatting and coding style throughout the code-base. This includes aspects such as indentation, spacing, naming conventions, and so on, which help improve readability and maintainability.
Performance Considerations:-
While clean code prioritizes readability and maintainability, it also takes performance into account where necessary. This involves optimizing critical sections of code, avoiding unnecessary overhead, and considering factors like memory usage and algorithmic efficiency.
Fun to Write and Maintain:-
Clean code is enjoyable to work with for developers. It's satisfying to write code that is elegant, efficient, and easy to understand, and it's less stressful to maintain code that is well-organized and documented.
By adhering to these principles, developers can produce code that is not only functional but also easy to understand, modify, and extend over time.
Things to be considered
Rules and concepts
DRY (Don't Repeat Yourself):-
This principle emphasizes the avoidance of duplicating code. Duplicated code increases the risk of inconsistencies and makes maintenance more difficult. Instead, reusable code should be encapsulated into functions, classes, or modules.
KISS (Keep It Simple, Stupid):-
This principle advocates for simplicity in design and implementation. Complex solutions can lead to confusion and bugs, while simpler solutions are easier to understand, debug, and maintain.
YAGNI (You Ain't Gonna Need It):-
This principle discourages adding functionality that is not currently needed. Speculative features can bloat the codebase, increase complexity, and introduce unnecessary dependencies. Only implement features that are required by the current requirements.
Patterns & Principles
Design Patterns:-
Design patterns are reusable solutions to common problems in software design. Examples include the Singleton pattern, Factory pattern, Observer pattern, etc. These patterns provide proven solutions to recurring design challenges and promote clean, maintainable code.
SOLID Principles:-
S - Single Responsibility Principle
O - Open/Closed Principle
L - Liskov Substitution Principle
I - Interface Segregation Principle
D - Dependency Inversion Principle
These principles form the foundation of object-oriented design and promote modular, flexible, and maintainable code.
Test-Driven Development (TDD)
Test-Driven Development is a development approach where tests are written before the code implementation. This follows a cycle of:
Write a Test: First, write a test that specifies a desired behavior or feature.
Write the Code: Implement the code necessary to make the test pass.
Run the Tests: Execute all tests to ensure the new code did not break existing functionality.
Refactor: Once the tests pass, refactor the code to improve its structure, readability, and performance while keeping the tests passing.
TDD promotes clean code by ensuring that code is thoroughly tested and that new features are added incrementally while maintaining existing functionality.
Paradigm-specific Considerations
Functional Programming:-
Emphasizes immutability, pure functions, and higher-order functions. This paradigm encourages a declarative style of programming, which can lead to cleaner, more concise code with fewer side effects.
Object-Oriented Programming:-
Focuses on encapsulating behavior and state within objects. Principles like encapsulation, inheritance, and polymorphism help promote modular design and code reuse.
Procedural Programming:-
Prioritizes procedures or functions that perform operations on data. While not as modular as OOP or as declarative as FP, procedural code can still be kept clean by adhering to principles like DRY and KISS, and by organizing code into cohesive functions or modules.
Continuous improvement and refactoring are indispensable practices in our journey towards maintaining a robust and sustainable codebase. While we strive to craft exemplary code from the outset, the iterative nature of software development means that opportunities for enhancement will inevitably arise as our projects evolve. By embracing the ethos that "Refactoring today is the work we save tomorrow," we acknowledge the value of investing time and effort into improving code quality upfront, leading to long-term benefits such as reduced technical debt, increased agility, and enhanced developer productivity.
As a team, we recognize that integrating refactoring into our development workflow is essential for addressing shortcomings and inefficiencies as they emerge, rather than allowing them to accumulate and impede progress. This proactive approach fosters a culture of craftsmanship and quality within our team, promoting collaboration, innovation, and a shared commitment to excellence in software engineering practices.
By continuously refining and optimizing our code, we can mitigate the accumulation of complexity, eliminate redundancy, and adapt more effectively to changing requirements and technological advancements. Through this iterative process of improvement, we not only ensure the resilience and longevity of our software projects but also position ourselves for sustained innovation, competitive advantage, and customer satisfaction in an ever-evolving technological landscape.
Naming
Click here
Comments
Click here
Code Formatting
Click here
Functions/Methods
Click here
Control Structure & Errors
Click here
Classes, Objects and Data Containers
Click here
Key Takeaways
1. Naming:
Descriptive names: Use nouns for variables and properties, and verbs for methods.
Specificity: Be as specific as possible without being redundant.
Consistency: Avoid slang, unknown abbreviations, and maintain consistency in naming conventions.
Clarity: Make names easy to read and understand by humans.
2. Comments and Formatting:
Minimal comments: Most comments are discouraged; only include necessary comments like legal information, warnings, or explanations.
Vertical formatting: Keep related concepts together and separate unrelated ones using blank lines and line breaks.
Horizontal formatting: Keep lines short, use indentation, and split code across multiple lines for readability.
IDE assistance: Utilize auto-formatting tools and IDE features to aid in writing clean code.
3. Functions:
Parameters: Limit the number of parameters and consider grouping them into dictionaries or objects for clarity.
Function body: Keep functions small and focused on a single task to maintain clarity and avoid repetition.
Abstraction levels: Ensure consistency between the level of abstraction implied by the function name and its implementation.
DRY principle: Avoid repeating code and strive for reusability.
Side effects: Minimize unexpected side effects to enhance code readability.
4. Control Structures:
Positive wording: Use positive language in control structures for clarity.
Avoid deep nesting: Utilize guard clauses and extract logic into separate functions to prevent deep nesting.
Polymorphism and factory functions: Reduce code duplication by employing polymorphism and factory functions.
Error handling: Use real errors instead of synthetic errors to improve code clarity.
5. Objects and Classes:
Differentiation: Distinguish between real objects with methods and data structures that only hold data.
Class size: Keep classes small by focusing on a single responsibility.
Law of Demeter: Avoid diving too deeply into object hierarchies to maintain code clarity.
SOLID principles: Follow principles like SRP and OCP to enhance maintainability and improve code readability.
Clean code prioritizes human readability and understanding over mere functionality. By following guidelines for naming, comments, formatting, functions, control structures, and objects/classes, developers can ensure their code is clear, concise, and maintainable. Consistency, clarity, and simplicity are key principles that should guide the writing and organization of code, ultimately leading to better collaboration, easier debugging, and enhanced software quality.
Conclusion
In conclusion, the pursuit of clean code transcends the mere execution of programming tasks; it is an art form, a philosophy, and a commitment to excellence in software development. Throughout this discussion, we have delved into the intricacies of various aspects that constitute clean code, ranging from naming conventions to the principles of object-oriented programming. At its core, clean code is about prioritizing the human experience of reading and understanding code over mere functionality.
Through meticulous naming, minimal yet purposeful comments, and thoughtful formatting, developers create code that is not only functional but also comprehensible to others. By adhering to principles such as modular function design, clear control structures, and object-oriented best practices, clean code fosters readability, simplicity, and maintainability.
In essence, clean code is not merely a set of rules or guidelines; it is a mindset—a commitment to craftsmanship and excellence in software development. As we continue to evolve in our understanding and practice of clean code, let us remain steadfast in our dedication to writing code that not only speaks to machines but also resonates with our fellow humans. For in the end, clean code is not just about computers; it's about people.
Subscribe to my newsletter
Read articles from Saurabh Mahajan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
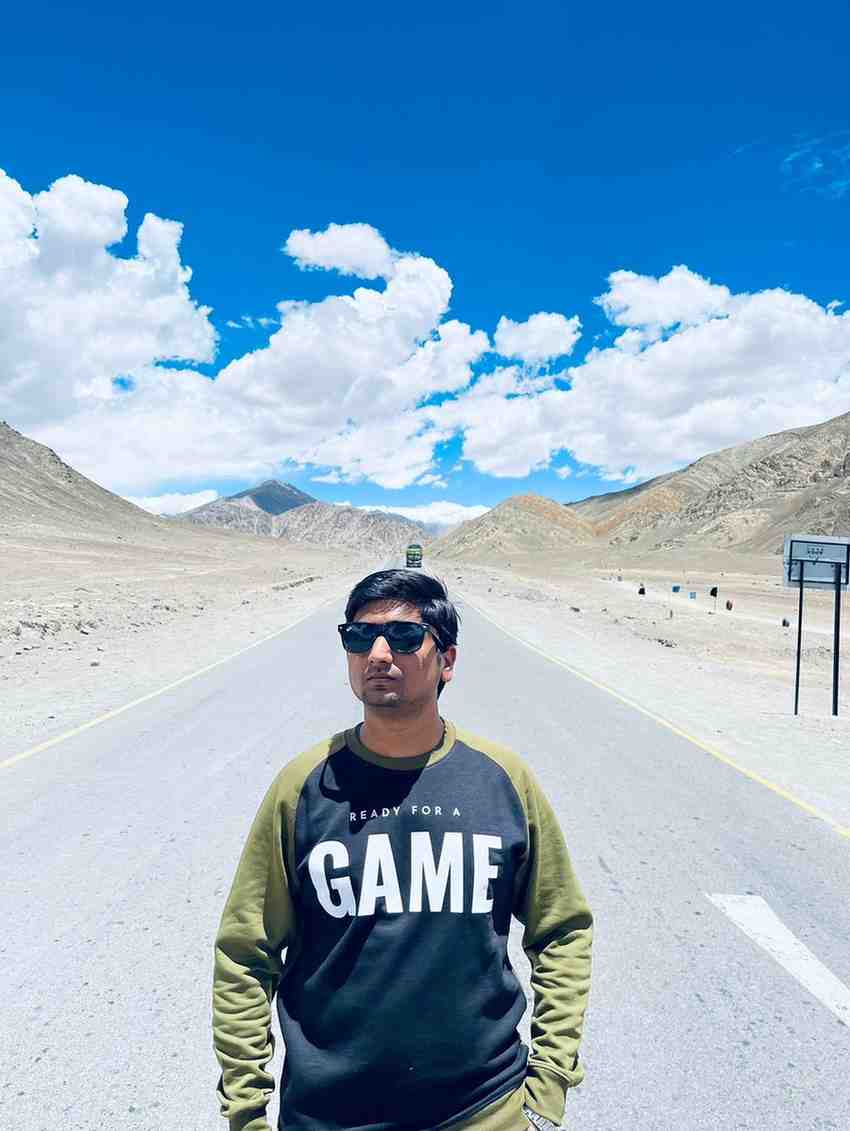
Saurabh Mahajan
Saurabh Mahajan
Greetings! 🚀 I'm a dynamic Fullstack Software Engineer, driving advancements in technology to empower organizations globally. Proficient in a diverse tech stack, I contribute to the architecture and development of high-performance applications. Beyond coding, I thrive on creating empowering and collaborative environments, championing technological innovation, and fostering connections to push the boundaries of excellence. As a Gamer🎮, and a Footballer⚽️, I bring passion to both my professional and personal pursuits. A Wanderlust🛣️ enthusiast and Orophile⛰️, I thrive on exploring diverse landscapes. A Melomane🎵 and avid TV😍 enthusiast, I find joy in the finer aspects of life. Also, a proud enthusiast of Tea☕️ who appreciates bike rides, beaches, and mountains alike. Always eager to connect with like-minded professionals and individuals who share a zest for technology, innovation, and life's adventures. Let's push boundaries together! 🌐