System Design Concepts for Full-Stack Java Developers

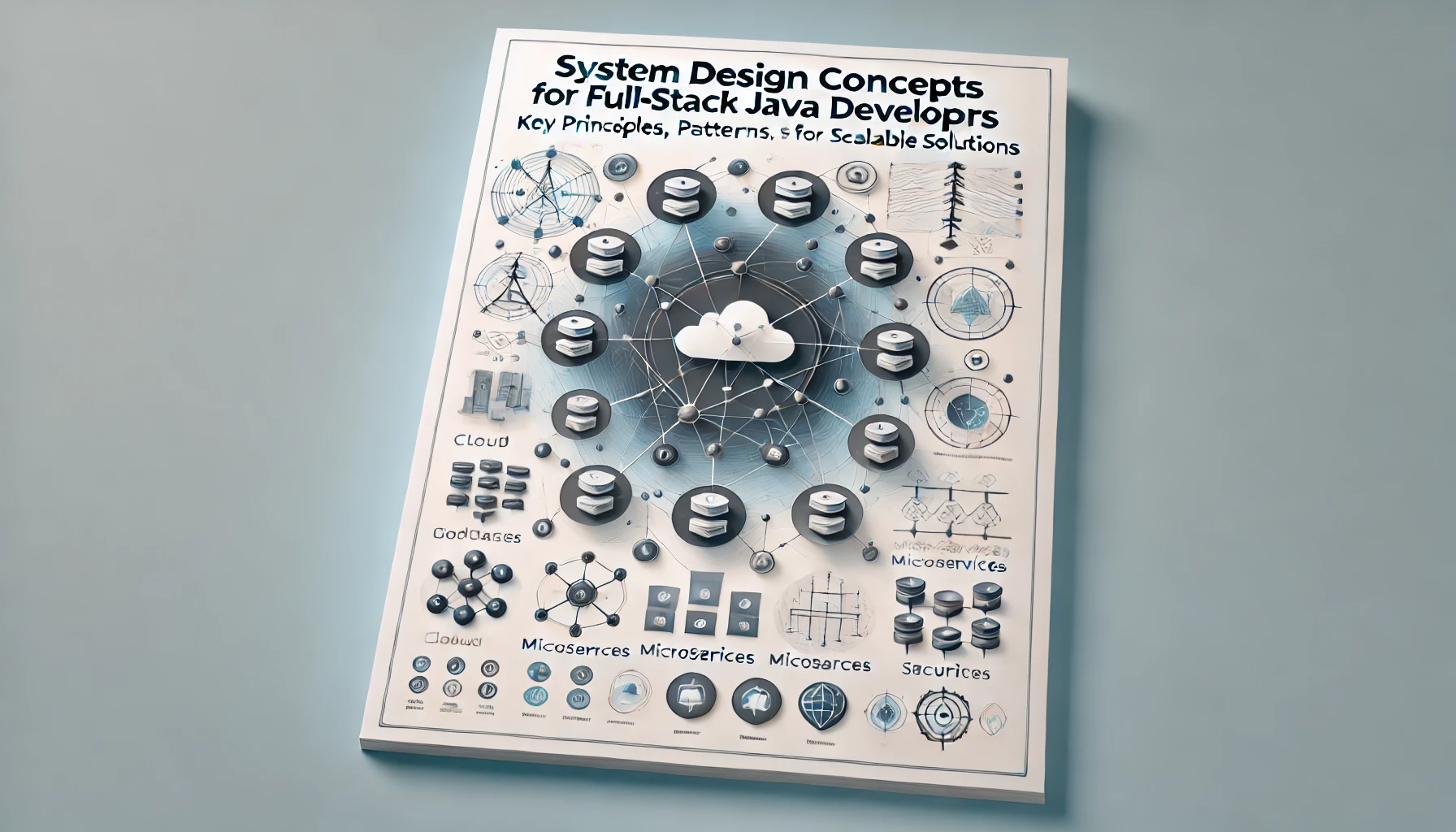
1. Key Goals of System Design
Scalability: The system's ability to handle increased load by adding resources. Includes vertical scaling (more power to a single node) and horizontal scaling (adding more nodes).
Reliability: Ensures the system continues functioning even in the presence of failures using redundancy and replication.
Maintainability: Designing systems that are easy to update, debug, and extend with minimal disruption.
Performance: Achieving low latency (response time) and high throughput (amount of work done per unit of time).
2. Architectural Patterns
Layered Architecture: Organizes the application into layers (presentation, business logic, data access) to separate concerns.
Microservices: A collection of small, independent services communicating over a network. Each service focuses on a single business capability.
Event-Driven Architecture: Uses events to trigger and communicate between services. Common in systems requiring real-time updates.
MVC/MVVM: Patterns for separating concerns in UI-driven applications. MVC splits into Model, View, Controller, while MVVM adds ViewModel for binding.
3. Database Design
SQL vs NoSQL: SQL databases are relational and structured, while NoSQL databases are designed for scalability and unstructured data (e.g., MongoDB, Cassandra).
Database Normalization and Denormalization: Normalization reduces redundancy, while denormalization improves read performance at the cost of storage.
Indexes and Query Optimization: Indexes speed up data retrieval, but excessive indexing can slow down writes.
Caching: Temporary storage (e.g., Redis) for quick access to frequently used data, reducing database load.
4. Scalability and Load Balancing
Load Balancers: Distribute incoming traffic across servers (e.g., HAProxy, AWS ELB) to prevent overloading a single server.
Horizontal Scaling: Adding more instances to handle increased load.
CDNs: Serve static resources (images, CSS, JS) from edge servers closer to the user, reducing latency.
5. System Communication
APIs: REST uses stateless HTTP methods, while GraphQL provides flexible querying with a single endpoint.
Inter-Service Communication: Synchronous methods like REST/gRPC and asynchronous methods like Kafka or RabbitMQ for decoupled systems.
Rate Limiting and Circuit Breakers: Control traffic to avoid overwhelming services and gracefully degrade when dependent services fail.
6. Security Considerations
Authentication: Verifying user identity using methods like OAuth 2.0 or JSON Web Tokens (JWT).
Secure Data: Encrypting data in transit (SSL/TLS) and at rest (database encryption).
OWASP Best Practices: Avoiding vulnerabilities like SQL injection, XSS, and CSRF through secure coding practices.
7. High-Level Design Workflow
Requirements Gathering: Identifying what the system must do (functional) and how it must perform (non-functional).
System Components: Breaking the system into manageable parts like front-end, back-end, and database.
Database Schema Design: Creating a structure to store and relate data (e.g., ER diagrams).
Data Flow: Visualizing how data moves through the system using sequence or data flow diagrams.
Performance Strategies: Techniques like caching, load balancing, and database sharding to handle scaling.
8. Common Interview Systems
URL Shortener: Maps long URLs to short keys using a hash function or a key-value store.
E-Commerce Platform: Requires user management, product catalogs, and order processing with payment integration.
Social Media Feed: Handles personalized newsfeeds, requiring real-time updates and ranking algorithms.
Messaging System: Delivers real-time communication using WebSockets or message brokers like RabbitMQ.
9. Tools and Techniques
UML Diagrams: Represent system components, processes, and relationships visually for better understanding.
Tools: Draw.io, Lucidchart for creating system design diagrams.
Cloud Platforms: Familiarity with AWS (e.g., EC2, S3), Azure, or GCP services for hosting scalable applications.
10. Java-Specific Considerations
Frameworks: Spring Boot for building robust back-end applications and Hibernate for ORM.
Threads and Concurrency: Managing threads efficiently using executors or modern thread pools.
Logging and Monitoring: Tools like SLF4J and Prometheus for system observability and debugging.
Subscribe to my newsletter
Read articles from Mihai Popescu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
