How to Build Your Own Free Chatbot (Max IA)

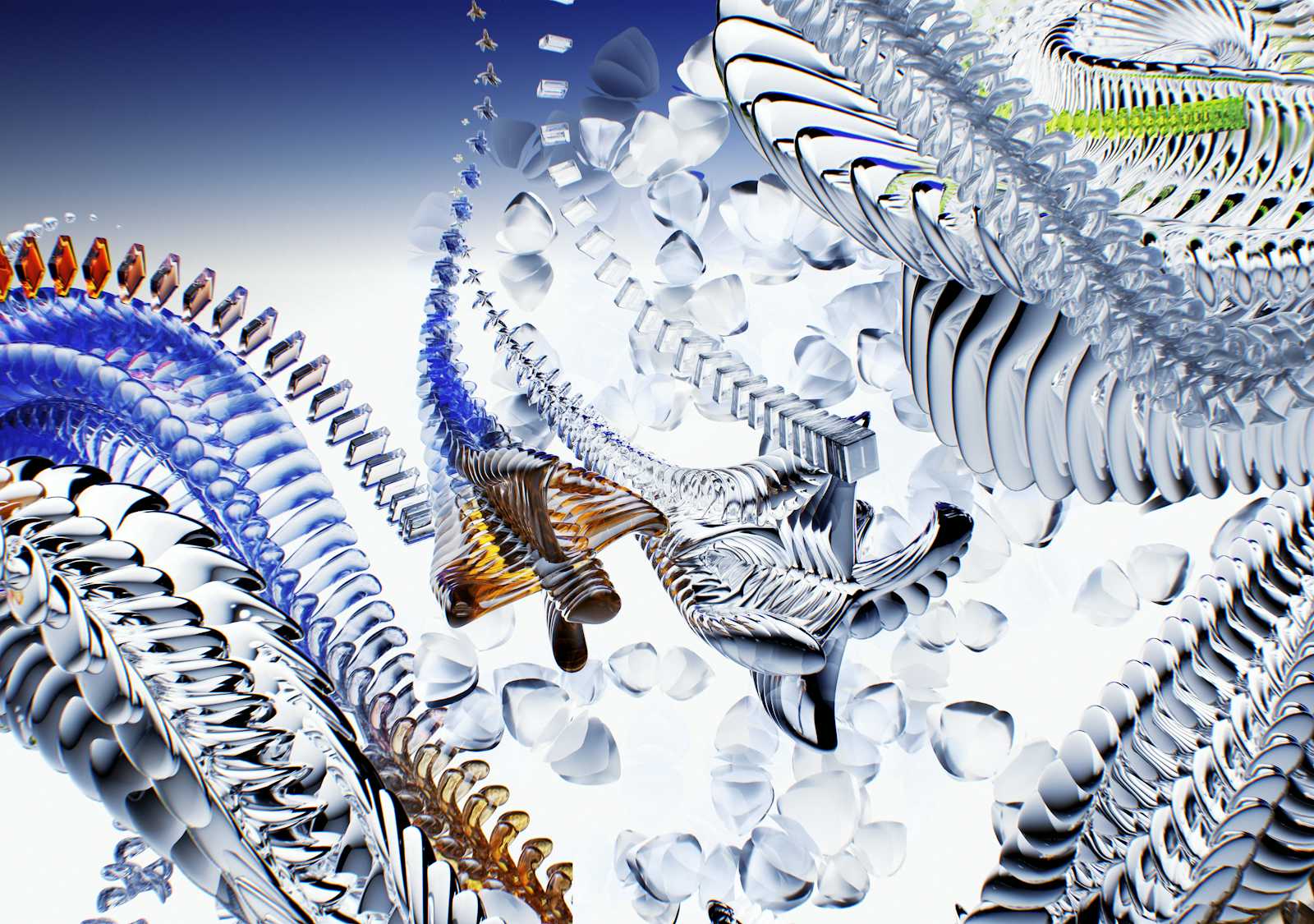
Let’s talk chatbots—specifically, how to build one that feels less like a rigid Q&A machine and more like a dynamic, multi-modal assistant. I want to share the blueprint I used for Max IA, focusing on free or low-cost tools, lightweight architecture, and subtle technical optimizations that add polish without overcomplicating things.
Core Architecture: Balancing Power and Simplicity
Every chatbot starts with its language model (LM), the engine driving its responses. For cost-effective scalability, I recommend starting with Google’s Gemini API via their AI Studio SDK. It’s RESTful, supports streaming for real-time interactions, and handles context windows up to 1 million tokens in the latest iteration. However, if you’re aiming for enterprise-grade throughput (or simply enjoy tinkering with open-source models), Groq’s LPU inference engine is a real game-changer. I deployed their Llama-3-70b variant, which processes over 300 tokens per second—ideal for applications where latency is critical.
Pro tip: If you plan to self-host smaller LMs later, utilize model quantization techniques (like GGUF or AWQ). This effectively reduces memory overhead without significantly sacrificing output quality.
Voice Synthesis: Moving Beyond Robotic TTS
Text-to-speech (TTS) is often a stumbling block for chatbots, making them sound unnatural. Azure’s Neural Voice API provides a solution with prosody controls and SSML markup. This allows you to inject nuances like pauses, emphasis, and even laughter into the chatbot's responses. Their prebuilt voices, such as “en-US-JennyNeural,” leverage deep convolutional networks to achieve a startlingly natural cadence. For a truly personal touch, their Custom Voice portal even lets you clone a voice with just 30 minutes of training data.
const ssml = `<speak version="1.0"
xmlns="http://www.w3.org/2001/10/synthesis"
xmlns:mstts="http://www.w3.org/2001/mstts"
xml:lang="en-US">
<voice name="en-US-AndrewMultilingualNeural">
<mstts:express-as style="Empathetic">
${text}
</mstts:express-as>
</voice>
</speak>`;
Under the hood with Max IA, the chatbot’s text output is piped into Azure’s Speech SDK using Python’s asynchronous client. For efficiency, I recommend a bonus tip: cache frequently used phrases locally to minimize API calls and improve responsiveness.
Vision Integration: Understanding Images Contextually
To enable image handling, I integrated Azure’s Computer Vision API into Max IA’s workflow. When a user uploads a photo, the backend sends a multipart/form-data POST request to Azure’s OCR and describe-image endpoints. The real magic happens in the post-processing stage. I employ regular expressions (regex) to extract key entities (like dates, URLs, and brands) and then feed these back into the LM as contextual metadata. This allows the chatbot to provide intelligent responses like, “This receipt shows a $42.50 charge at Starbucks on June 15th,” instead of just giving generic image descriptions.
const analyzeImageWithAzure = async (file: File) => {
try {
const imageData = await file.arrayBuffer();
// Send raw bytes to the Azure Vision endpoint
const response = await fetch(visionEndpoint, {
method: "POST",
headers: {
"Content-Type": "application/octet-stream",
"Ocp-Apim-Subscription-Key": visionKey,
},
body: imageData,
});
if (!response.ok) {
throw new Error("Azure Computer Vision request failed");
}
const result = await response.json();
// =====================
// Gather multiple insights
// =====================
// Description
const caption = result?.description?.captions?.[0]?.text || "No caption found";
const captionConfidence =
result?.description?.captions?.[0]?.confidence || 0;
// Limit to maximum 6 tags
const tagNames = result?.tags?.map((tag: any) => tag.name) || [];
const tags = tagNames.slice(0, 5).join(", ") || "No tags";
// Categories
const categories =
result?.categories
?.map(
(cat: any) => `${cat.name} (score: ${(cat.score * 100).toFixed(1)}%)`
)
.join(", ") || "None";
Fine-Tuning: Low-Effort, High-Impact Customization
Pre-trained language models are incredibly versatile, but injecting specific domain knowledge is essential for a truly helpful chatbot. To keep customization efficient, I opted for a method focused on context augmentation rather than extensive model retraining. Here’s how it works:
Knowledge Embeddings: I processed relevant documents (PDFs, notes, articles, etc.) by converting them into numerical representations using embedding models like text-embedding-3-small (OpenAI) or BERT-base.
Knowledge Storage: These embeddings are then stored in a system designed for efficient similarity search, either locally or in the cloud.
Contextual Prompting: For each user query, the system retrieves the most relevant document snippets based on the query’s similarity to the stored knowledge embeddings. These snippets are then added to the prompt given to the language model, providing crucial context.
For Max IA, I divided my blog posts and GitHub repositories into 512-token segments and created embeddings. Now, when users ask about my projects, the chatbot can intelligently reference specific code snippets or relevant articles from my content by leveraging this contextual information.
Deployment: Gluing Everything Together
The backend is structured as a server application with key endpoints: /chat (for handling language model queries), /speak (for TTS synthesis), and /vision (for image processing). To efficiently manage concurrent API requests—crucial when processing images and generating voice simultaneously—I used asynchronous programming to maintain responsiveness.
For hosting, Vercel’s Serverless Functions handle approximately 90% of Max IA’s traffic completely within their free tier. When dealing with heavier loads, I utilize a Hugging Face Space with a Dockerized version of the application for scalable and reliable performance.
Why This Stack Works (and Potential Tweaks)
Cost-Effectiveness: The free tiers of Gemini and Azure services are surprisingly generous, comfortably covering the needs of around 5,000 monthly users. Beyond that, Groq’s pay-as-you-go pricing offers linear scalability.
Low Latency: Employing asynchronous workflows keeps response times consistently under 2 seconds, even when incorporating vision and voice processing into the interaction.
Simplified Maintenance: The stateless architecture minimizes maintenance complexity. Session cookies are used for short-term memory, further simplifying operations.
If I were to revisit and improve one aspect, it would be implementing WebSockets for bidirectional streaming. This enhancement would enable real-time interaction, allowing users to interrupt responses or adjust the chatbot’s tone mid-conversation for a more dynamic experience.
Final Thoughts
Building a compelling chatbot isn't necessarily about chasing the absolute state-of-the-art models or over-engineering complex pipelines. It’s fundamentally about skillfully stitching together readily available APIs into a cohesive flow that feels genuinely intelligent and helpful to the user. The best approach is often iterative: start with a foundational single endpoint, like text-only chat, and then incrementally layer in more advanced features like voice, vision, and memory. Crucially, test with real users early and often. Nothing reveals brittle edge cases and unexpected user behaviors quite like real-world interactions, whether it’s a friend playfully uploading memes or asking about a very specific niche hobby.
Ultimately, the code itself is often not the most challenging aspect. The real art lies in the subtle details: a carefully considered 200ms delay here, a well-placed emoji there, or meticulously tweaking the temperature parameter to strike the right balance between creative output and coherent responses. With the powerful tools available today, you’re not just coding a bot—you’re thoughtfully designing a personality and an experience.
Subscribe to my newsletter
Read articles from Max Comperatore directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
