Python: How to Make and Use Single Line For-Loops
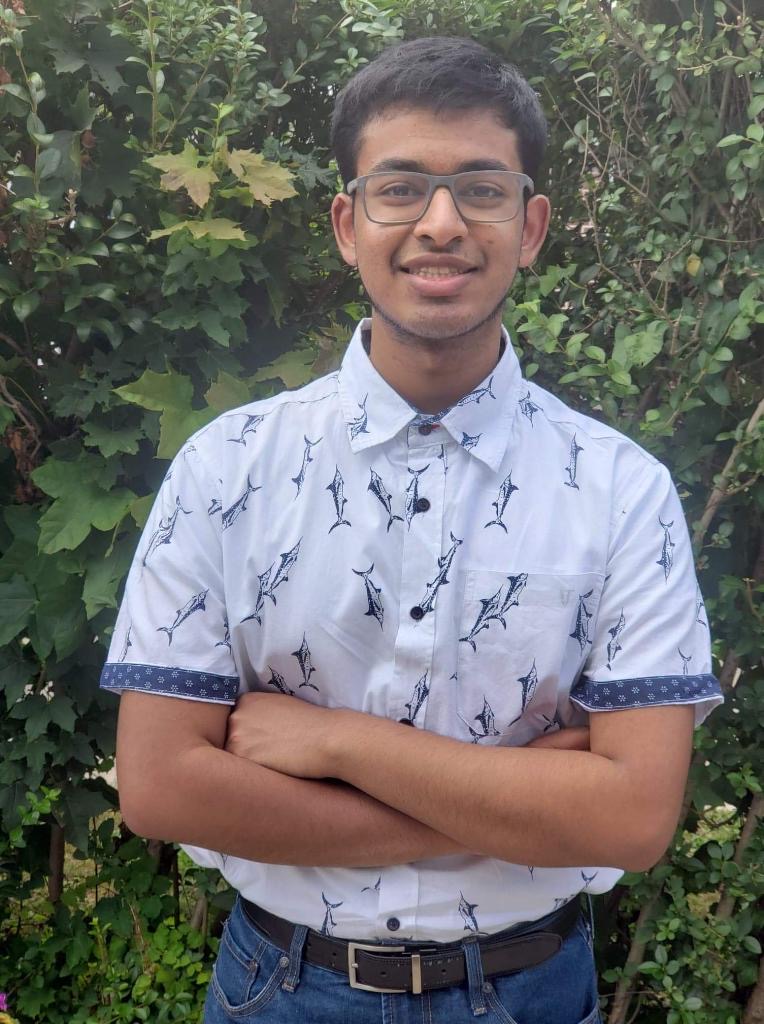
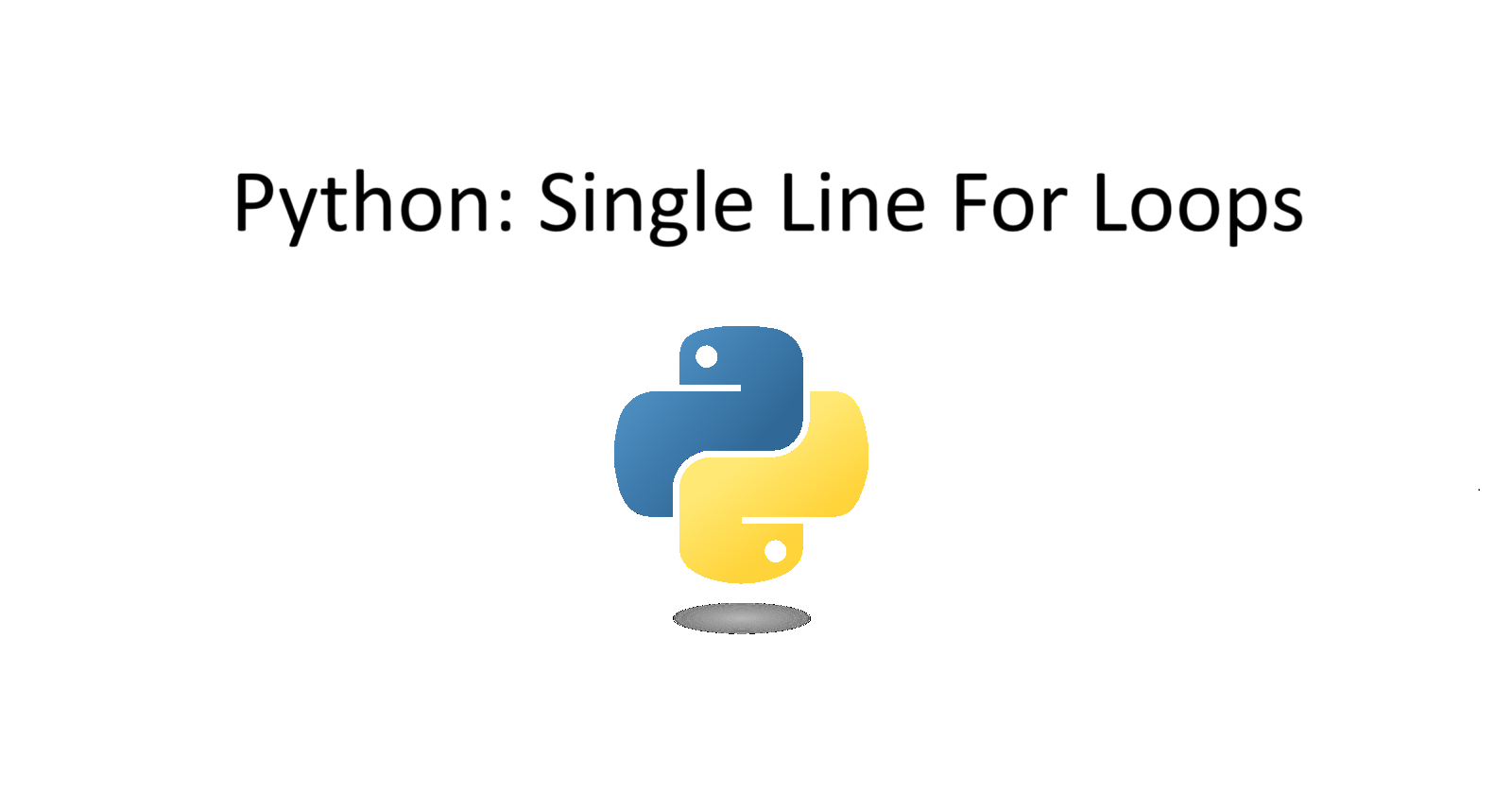
Overview
This article will explain how to create a for loop in one line of code and why and when using this is preferred than the standard for loop syntax.
Standard For Loop Syntax
A for loop is written with at least two lines:
for i in range(0, 3):
print(i)
How to create a One Line For Loop
Instead of writing “print(i)” on the next line, it can be written after the colin or “:“
for i in range(0, 3): print(i)
Using a Single Line For Loop to Print Lists
A single line for loop can also print out a statement or variable that contains a casted list statement. Usually, the for loop sentence is written first and then on the next line, the statement to print out a certain result is written. In a one line for loop statement, the variable is written first and then the for loop statement.
''' Below shows the variable numbers_in_a_list is
assigned to data that is casted within a list '''
numbers_in_a_list = [number for number in range(1, 10)]
print(numbers_in_a_list)
Using Single Line for Loops for Lambda Functions
In Python, lambda functions are written in one line to first declare variables and then a statement that uses the declared variables to perform an operation. Single line for loops are often used with lambda functions.
How to make a Lambda Function
Below shows how to make a lambda function. The word “lambda” has to be written first and then declare as much variables as needed. After declaring the variables, a colin or “:” is written and a statement to perform an operation that uses the declared variables.
addition = lambda var1, var2 : var1 + var2
print(addition(2, 3))
# Output: 5
How to use a Single Line for Loops with Lambda Functions
Single line for loops is often recommended to be used with Lambda functions where the statement is formatted in a list:
subtraction = lambda x, y : [x-y for i in range(0,4)]
print(subtraction(2,5))
# Output: [-3, -3, -3, -3]
The usual way of writing a one line for loop will not work with a lambda function because the colin or “:” would be repeated twice and then Python has trouble reading the syntax.
Subscribe to my newsletter
Read articles from Andrew Dass directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
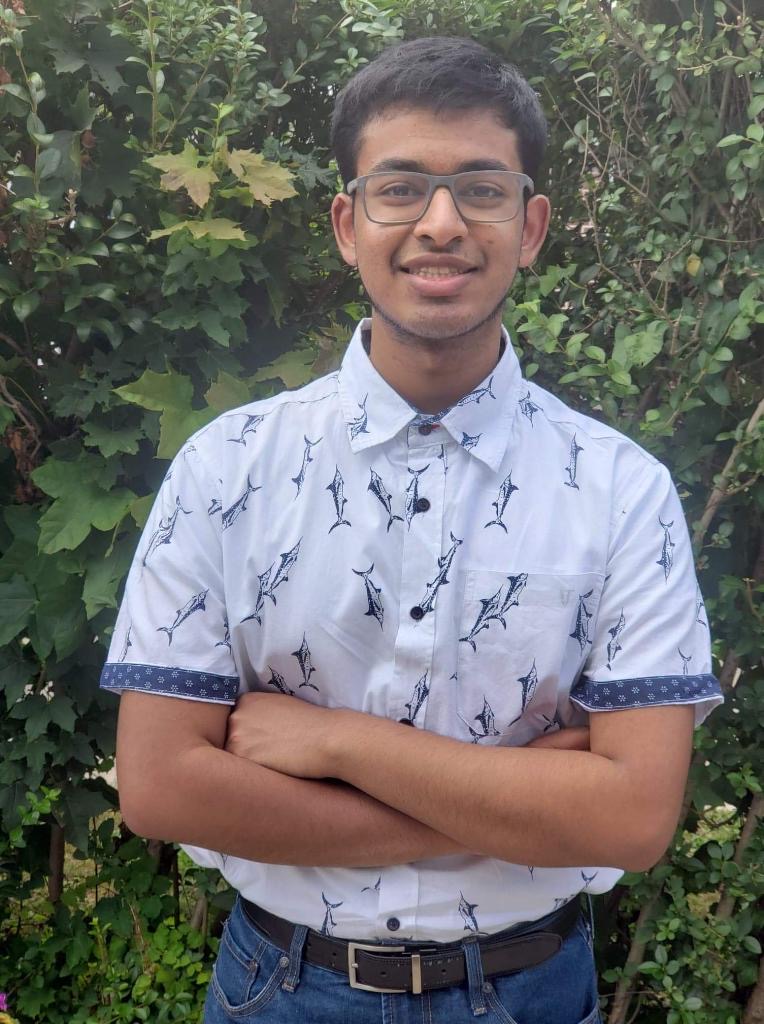
Andrew Dass
Andrew Dass
On my free time, I like to learn more about hardware, software and different technologies. I publish articles about my recent learnings or the projects I have done.