Design Pattern in React -Children Props

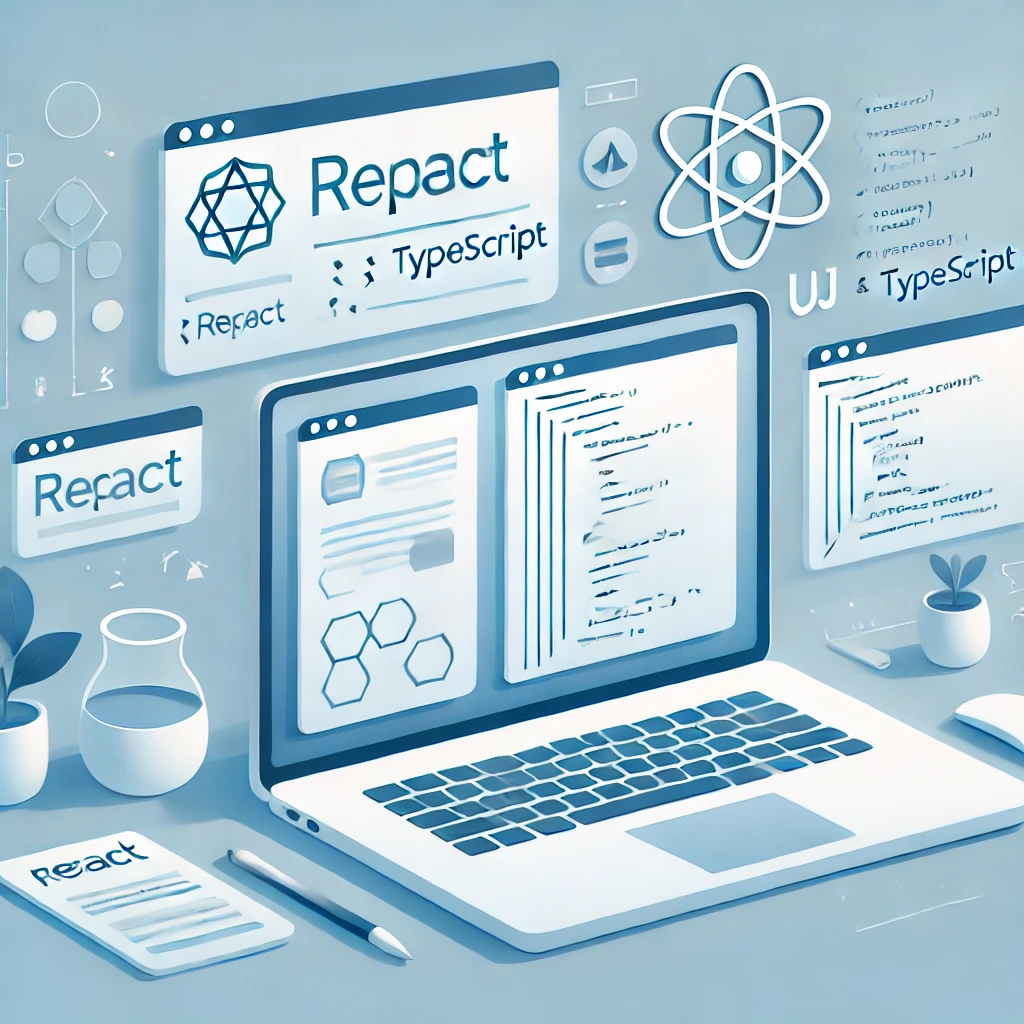
React’s flexible architecture provides numerous patterns for organizing components and passing data between them.
One of the most versatile and powerful patterns is the Children Design Pattern, where components receive children elements via the children
prop.
In this article, we will explore the children
Design Pattern, its use cases, benefits, and implementation in real-world scenarios.
Understanding the children
Prop
The children
prop is a special prop automatically passed to every React component. It represents the content nested between the opening and closing tags of a component. This allows developers to compose components dynamically by embedding other elements within them.
Example:
const Wrapper = ({ children }) => {
return <div className="wrapper">{children}</div>;
};
const App = () => {
return (
<Wrapper>
<h1>Heading</h1>
<p>This is a paragraph.</p>
</Wrapper>
);
};
export default App;
In this example, the Wrapper
component renders whatever elements are passed as its children.
Advantages of the Children Design Pattern
Reusability: Components can be designed to work with arbitrary children, making them highly reusable.
Flexibility: Developers can pass any valid React elements as children, enabling dynamic and flexible component composition.
Separation of Concerns: The parent component can focus on layout and structure, while the child components handle content or behavior.
Customizability: Components can accept a wide range of children, from simple text to complex JSX structures.
Common Use Cases
1. Layout Components
Layout components often use the children
prop to render dynamic content while maintaining a consistent structure or style.
Example:
const Card = ({ children }) => {
return <div className="card">{children}</div>;
};
const App = () => {
return (
<Card>
<h2>Title</h2>
<p>Card content</p>
</Card>
);
};
export default App;
2. Higher-Order Wrapper Components
Wrapper components like modals or tooltips often rely on the children
prop to embed custom content within their predefined structure.
Example:
const Modal = ({ children, onClose }) => {
return (
<div className="modal">
<div className="modal-content">
{children}
<button onClick={onClose}>Close</button>
</div>
</div>
);
};
const App = () => {
return (
<Modal onClose={() => console.log('Modal closed!')}>
<h1>Modal Header</h1>
<p>This is modal content.</p>
</Modal>
);
};
export default App;
3. Customizable Components with Render Props
The Children Design Pattern can work in conjunction with render props to provide greater control over how child components are rendered.
Example:
const ListItems = ({ items, children }) => {
return (
<ul>
{items.map((item, index) => (
<li key={index}>{children(item)}</li>
))}
</ul>
);
};
const App = () => {
const tasks = [
{ id: 1, name: 'Task 1', completed: true },
{ id: 2, name: 'Task 2', completed: false },
{ id: 3, name: 'Task 3', completed: true },
];
return (
<ListItems items={tasks}>
{(task) => (
<span style={{ textDecoration: task.completed ? 'line-through' : 'none' }}>
{task.name}
</span>
)}
</ListItems>
);
};
export default App;
4. Conditional Rendering of Children
Components can conditionally render their children based on specific conditions or props.
Example:
const ConditionalWrapper = ({ condition, children }) => {
return condition ? <div className="highlight">{children}</div> : children;
};
const App = () => {
return (
<ConditionalWrapper condition>
<p>This content is conditionally wrapped.</p>
</ConditionalWrapper>
);
};
export default App;
5. Cloning and Manipulating Children
React provides the React.Children
API and React.cloneElement
method to manipulate children or inject additional props dynamically.
Example:
const ListItems = ({ children }) => {
return (
<ul>
{React.Children.map(children, (child) =>
React.cloneElement(child, { className: 'enhanced-list-items-class' })
)}
</ul>
);
};
const App = () => {
return (
<ListItems>
<li>List Item 1</li>
<li>List Item 2</li>
<li>List Item 3</li>
</ListItems>
);
};
export default App;
Conclusion
The Children Design Pattern is a cornerstone of React development, enabling developers to create modular, flexible, and reusable components. Happy Learning!!! :)
Subscribe to my newsletter
Read articles from The Syntax Node directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

The Syntax Node
The Syntax Node
I am a JavaScript Full Stack Developer with expertise in front-end frameworks like React and Angular, and back-end technologies such as Node.js and Express.