How to Setup Google Recaptcha V3 - A complete tutorial
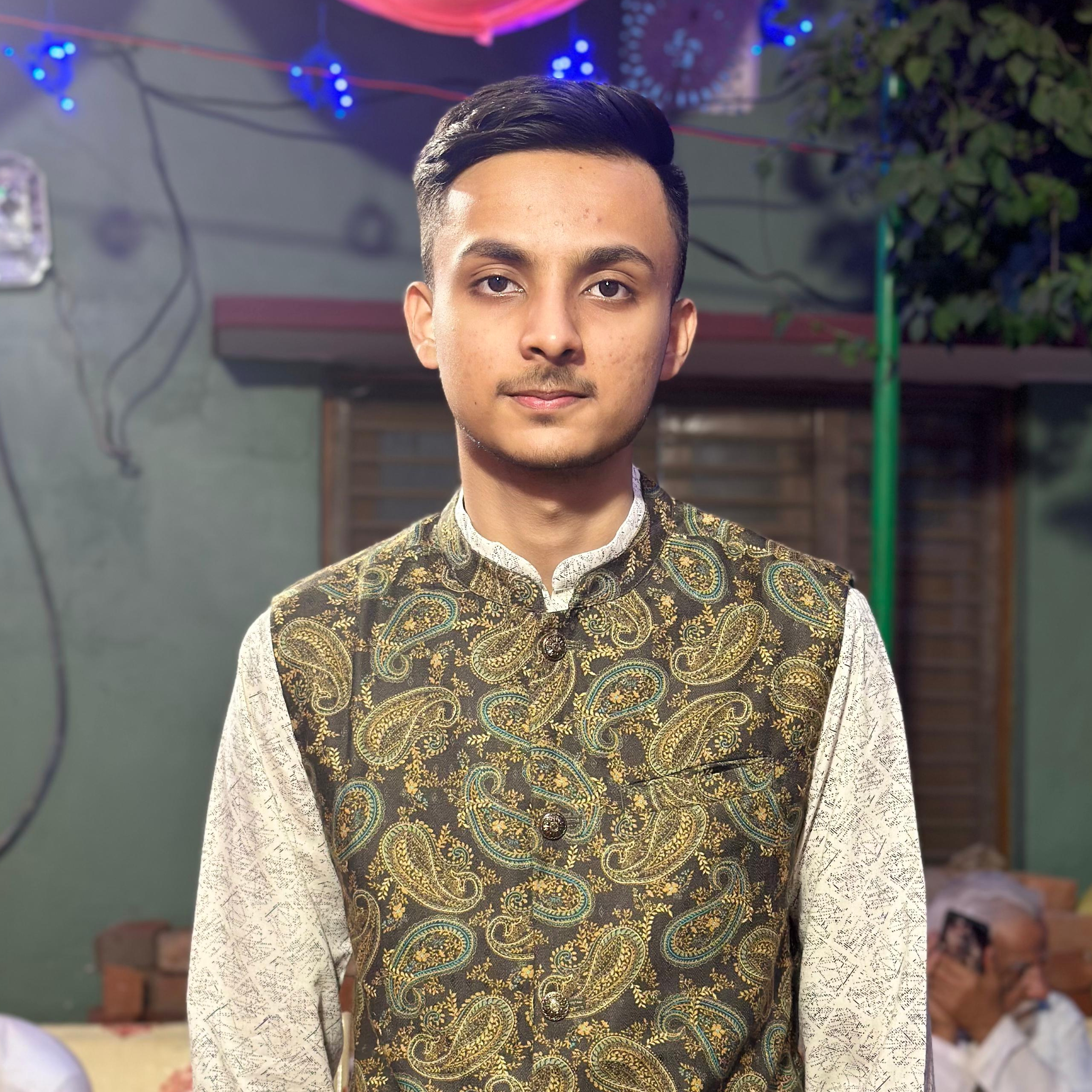

Let’s face it: the internet isn’t always a friendly place. For every helpful person, there’s a swarm of pesky bots trying to spam forms, scrape data, or even hack into systems. That’s where Google Recaptcha comes in - a silent guardian, a watchful protector. (Yes, I just went full Dark Knight on you.)
In this blog, we’re exploring the world of Recaptcha V3 - what it is, how it works, and how you can set it up to protect your site like a pro. Ready? Let’s go! 🚀
What is Recaptcha V3?
Google Recaptcha is a tool that helps websites determine if the user is a real person. Its main goal is to stop bots without bothering genuine users. Unlike older captchas that made you squint at distorted letters or click on numerous traffic lights, Recaptcha V3 operates quietly in the background. No clicking, no puzzles, no hassle. Just smooth, bot-free browsing.
Difference between Recaptcha V2 and V3
Features | Recaptcha V2 | Recaptcha V3 |
User Interaction | Requires user interaction, like checking a box ("I'm not a robot") or solving image puzzles. | Operates silently in the background with no user interaction required. |
Verification Method | Binary result: confirms whether the user is a human or a bot. | Provides a score (0.0 to 1.0) indicating the likelihood of a user being human. |
Impact on UX | Can disrupt the user experience with visible challenges. | Enhances user experience by running invisibly. |
Implementation | Adds visible elements like the checkbox or CAPTCHA widget to the webpage. | Works via an invisible script that analyzes user behavior. |
Use Cases | Ideal for simple forms or when active user verification is acceptable. | Best suited for complex applications requiring nuanced bot detection. |
Setting Up Google Recaptcha V3
To get started with Recaptcha V3, you first need to create a new project and get the keys needed for integration. Here’s how you can do it:
Create a New Recaptcha Project
To create a new Recaptcha project, simply open this link and register a new site.
Be sure to select Score based (v3) as the Recaptcha type, since we are using V3 in this tutorial.
Add your domain so Recaptcha can work on your site. If you want it to run in a development environment, you also need to add
localhost
as a domain, without any ports.
Copy the Site Key and Secret Key
Once you fill out the form and submit it, you will receive two keys. Copy and save both of them, as we will need them later.
This was all for the non coding setup, now let’s jump on the code.
Installing and Configuring Recaptcha in your project
Step 1: Install the necessary packages
For this tutorial, we are using a great library called React Google Recaptcha V3. It will help us integrate Google Recaptcha V3 into your app.
npm i react-google-recaptcha-v3
Step 2: Paste Recaptcha keys in .env file
Once you’ve copied the Site Key and Secret Key from the Recaptcha dashboard, the next step is to securely store them. The best practice is to use environment variables to keep your keys safe and separate from your codebase.
Open the
.env.local
file in your Next.js project. If it doesn’t exist, create one in the root directory.Add the following lines, replacing the placeholders with your actual keys:
NEXT_PUBLIC_GOOGLE_RECAPTCHA_SITE_KEY=your_site_key_here RECAPTCHA_SECRET_KEY=your_secret_key_here
The
NEXT_PUBLIC_
prefix ensures the Site Key is accessible on the client side.The Secret Key, on the other hand, is used only on the server and should never be exposed to the client.
Save the file and restart your development server to ensure the new environment variables are loaded.
By storing the keys in the .env.local
file, you protect sensitive data and make it easy to switch between environments (e.g., development, staging, production).
Step 3: Add Recaptcha Script to the Head
To use Google Recaptcha V3, you need to include its script on your website. In a Next.js project, the best way to add this script globally is by including it in the _document.tsx
file.
Open the
_document.tsx
file in thepages
directory of your Next.js project. If the file doesn’t exist, create it.Add the following code inside the
<Head>
tag:import { Html, Head, Main, NextScript } from 'next/document'; export default function Document() { return ( <Html lang="en"> <Head> {/* Add the Recaptcha script */} <script src={`https://www.google.com/recaptcha/api.js?render=${process.env.NEXT_PUBLIC_GOOGLE_RECAPTCHA_SITE_KEY}`} async defer ></script> </Head> <body> <Main /> <NextScript /> </body> </Html> ); }
- The
async
anddefer
attributes ensure that the script doesn’t block page rendering.
- The
Save the file, and now the Recaptcha script will be automatically included on every page of your application.
Step 4: Wrap Login Component with Google Recaptcha Provider
To use Recaptcha V3 smoothly on your login page, you need to wrap it with GoogleRecaptchaProvider
like this. You will need to pass the Recaptcha Site key to the provider for it to work.
import { GoogleReCaptchaProvider } from 'react-google-recaptcha-v3';
const Login = () => {
const handleLogin = () => {
// Your login logic
};
return (
<form>
<h1>Login</h1>
<input type="text" />
<button onClick={handleLogin}>Login</button>
</form>
);
};
const LoginPage = () => {
return (
<GoogleReCaptchaProvider reCaptchaKey={process.env.NEXT_PUBLIC_GOOGLE_RECAPTCHA_SITE_KEY}>
<Login />
</GoogleReCaptchaProvider>
);
};
export default LoginPage;
Step 5: Create an API route for Token Validation
- Inside the
pages/api
directory, create a new file calledvalidate-recaptcha.ts
and add the following code.
import type { NextApiRequest, NextApiResponse } from 'next';
const handler = async (req: NextApiRequest, res: NextApiResponse) => {
if (req.method !== 'POST') {
return res.status(405).json({ message: 'Method Not Allowed' });
}
const { token } = req.body;
if (!token) {
return res.status(400).json({ message: 'Token is required' });
}
try {
const secretKey = process.env.RECAPTCHA_SECRET_KEY!;
const response = await fetch(`https://www.google.com/recaptcha/api/siteverify`, {
method: 'POST',
headers: { 'Content-Type': 'application/x-www-form-urlencoded' },
body: new URLSearchParams({
secret: secretKey,
response: token,
}).toString(),
});
const data = await response.json();
const threshold = 0.5;
if (data.success && data.score >= threshold) {
return res.status(200).json({ score: data.score, success: true });
} else if (data.success && data.score < threshold) {
return res
.status(403)
.json({ message: 'Score below threshold. Possible bot detected.', success: false });
} else {
return res.status(400).json({ message: 'Token validation failed', success: false });
}
} catch (error) {
console.error(error);
return res.status(500).json({ message: 'Internal Server Error' });
}
};
export default handler;
What This Route Does:
Accepts a POST request with the
token
sent from the client.Sends the
token
and your Recaptcha Secret Key to Google’s API for validation.Returns a response with the user’s score and success status.
Step 6: Integrate the API Route on the Client
Now that you’ve created the API route for validating the Recaptcha token, it's time to use it on the client side. This step involves generating the Recaptcha token on the client and sending it to your API for validation. Here's how you can do it:
import { useGoogleReCaptcha } from 'google-recaptcha-v3';
import { useState } from 'react';
const LoginPage = () => {
const { executeRecaptcha } = useGoogleReCaptcha();
const [loading, setLoading] = useState(false);
const [error, setError] = useState('');
const handleLogin = async () => {
if (!executeRecaptcha) {
setError('Recaptcha not initialized');
return;
}
setLoading(true);
setError('');
try {
// Generate the Recaptcha token
const token = await executeRecaptcha('login');
// Send the token to the API for validation
const response = await fetch('/api/validate-recaptcha', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ token }),
});
const result = await response.json();
if (result.success && result.score >= 0.5) {
console.log('Recaptcha validation successful!');
// Proceed with login logic (e.g., authenticate the user)
} else {
setError(result.message);
}
} catch (err) {
console.error('Error validating Recaptcha:', err);
setError('Something went wrong. Please try again.');
} finally {
setLoading(false);
}
};
return (
<div>
<h1>Login</h1>
<button onClick={handleLogin} disabled={loading}>
{loading ? 'Validating...' : 'Login'}
</button>
{error && <p style={{ color: 'red' }}>{error}</p>}
</div>
);
};
export default LoginPage;
Let's break down what's happening in the above code:
First, we are using the
useGoogleReCaptcha
hook to get theexecuteRecaptcha
function.Next, we are checking if
executeRecaptcha
is initialized when the login button is clicked. If it's not, we display an error.After that, we are calling
executeRecaptcha
to generate a token, passing a unique action name like'login'
.Then, we send the generated token to our API for validation.
If the validation is successful and the score is above a set threshold (e.g., 0.5), we proceed with the login logic.
If the validation fails, we show the error message returned by the backend.
During this process, we handle loading and error states to provide feedback to the user.
Finally, we update the UI to show a loading message and display any errors that occurs.
Wrapping Up
And like this we have successfully integrated Google Recaptcha V3 with our Next.js project.
Please like and share the article if you found it helpful. Do also follow me on X.
Thanks for reading. Follow me for more articles!
Subscribe to my newsletter
Read articles from Ammar Mirza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
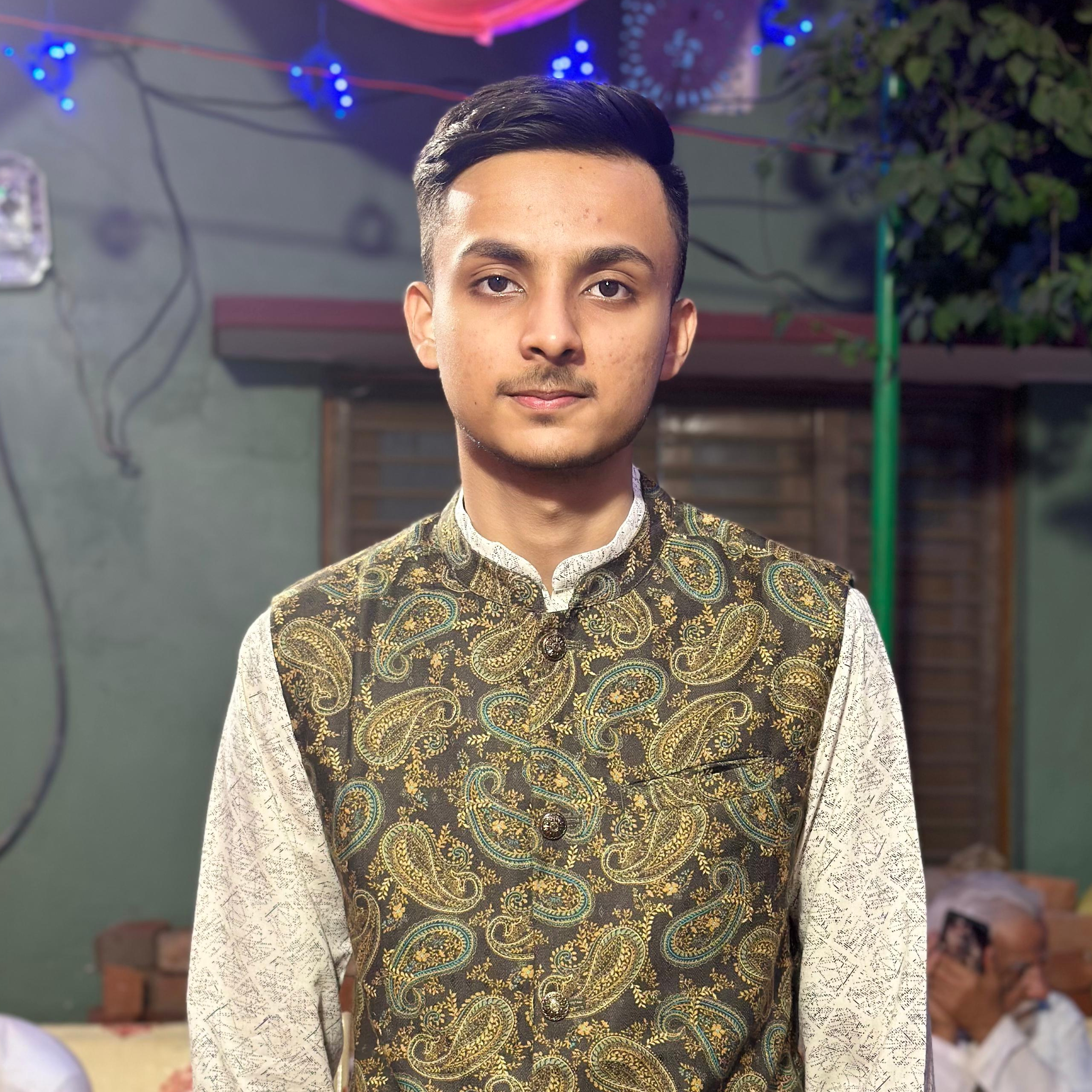
Ammar Mirza
Ammar Mirza
Just a normal guy who loves tech, food, coffee and video-games.