What is the best way to covert Webflow project to react ui components

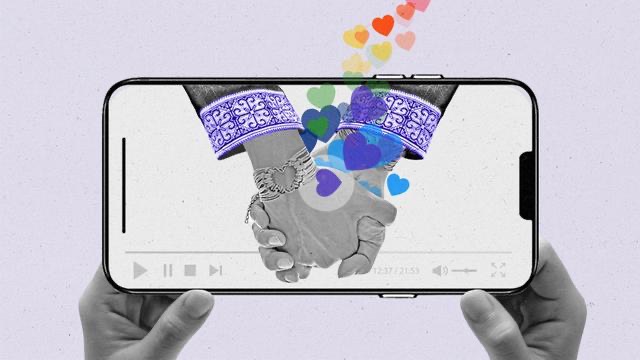
There are several established methods to convert Webflow projects into React components, each with different approaches and tooling options:
Official Solution: DevLink
DevLink is Webflow's official tool that allows you to build components in Webflow and use them directly in React projects, ensuring pixel-perfect design conversion.
Third-Party Tools
Webflow Componentizer A npm package that converts Webflow sites into React components by scanning HTML for data attributes. Installation is done via:
npm install webflow-componentizer --save-dev
This tool works by identifying components through data attributes and generating basic React components that you can customize.
Appfairy CLI A command-line tool that can convert entire Webflow projects to React with a simple workflow:
npm install appfairy -g
The process involves:
Creating a
.appfairy
directoryExporting Webflow project into this directory
Running the
appfairy
command to generate React components.
Manual Conversion Process
Step 1: Export and Prepare
Design your website in Webflow
Export the Webflow code as HTML, CSS, and JavaScript files.
Step 2: Component Creation
Convert the exported HTML into JSX using online converters
Create React components from the converted code
Maintain the styling by importing the Webflow-generated CSS.
Best Practices
Keep static components in Webflow for style updates
Use component-based architecture for better maintainability
Ensure proper integration of Webflow's responsive design principles.
The choice of method depends on your specific needs:
For simple sites: Manual conversion might be sufficient
For complex projects: Use tools like DevLink or Appfairy
For component-specific needs: Consider Webflow Componentizer.
What are the common pitfalls when integrating React components into Webflow projects
Several significant challenges arise when integrating React components into Webflow projects:
JavaScript Conflicts
JavaScript library conflicts occur between Webflow's native libraries and React's ecosystem, particularly affecting animations, interactions, and core features.
Animation Issues
Webflow animations often fail to work properly in React implementations because:
Animations may freeze at the first keyframe
React's rendering cycle can interfere with Webflow's animation timing
The data-wf-page and data-wf-site attributes must be properly configured.
Styling Inconsistencies
CSS Conflicts
Webflow's built-in CSS can clash with React component styles
Maintaining responsive design consistency becomes challenging when mixing both platforms.
Integration Limitations
Code Synchronization
Once React code is modified locally, it cannot be synchronized back with Webflow
Changes to the code break consistency with the online Webflow version
Refactoring becomes problematic if future Webflow updates are needed.
Performance Concerns
Multiple React instances can lead to increased bundle sizes
Fetching and injecting rendered JS code into divs can cause performance issues
State management becomes complex when dealing with multiple React components.
Development Workflow Issues
Setting up the development environment requires additional configuration
Local style modifications become cumbersome
Managing multiple codebases increases maintenance overhead.
Communication Barriers
Data exchange between React components and the Webflow platform requires careful handling
Component props and runtime properties need precise configuration
Version compatibility must be maintained between both platforms.
The best approach to mitigate these issues is to:
Carefully plan component architecture before integration
Use tools like DevLink for official support
Implement proper version control strategies
Consider micro-frontend architecture for complex implementations.
How can I resolve conflicts between Webflow's JavaScript libraries and React components
Several strategies can help resolve JavaScript library conflicts between Webflow and React components:
Runtime Initialization
To properly initialize Webflow's JavaScript after React renders:
useEffect(() => {
window.Webflow && window.Webflow.destroy();
window.Webflow && window.Webflow.ready();
window.Webflow && window.Webflow.require('ix2').init();
document.dispatchEvent(new Event('readystatechange'));
})
HTML Configuration
Essential Attributes Add required Webflow data attributes to your HTML tag:
<html lang="en" data-wf-page="YOUR_PAGE_ID" data-wf-site="YOUR_SITE_ID">
Component Architecture
Isolation Strategy
Create separate containers for Webflow and React components
Avoid direct DOM manipulation in React components where Webflow interactions exist
Use React's component lifecycle methods to manage Webflow initialization
State Management
Keep Webflow's state management separate from React's
Use React's state management for dynamic content
Allow Webflow to handle animations and interactions independently
Performance Optimization
Script Loading
Load Webflow's JavaScript libraries before React components
Avoid duplicate jQuery inclusions
Use module imports instead of global scripts to prevent variable conflicts
Animation Handling
Initialize Webflow animations after React component mounting
Ensure animation triggers are properly set up in both environments
Use React's useLayoutEffect for critical DOM measurements before animations
These solutions help maintain the functionality of both platforms while preventing conflicts in DOM manipulation and state management.
Learn more
Subscribe to my newsletter
Read articles from Erik Chen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
