Webflow export to TinaCMS integration

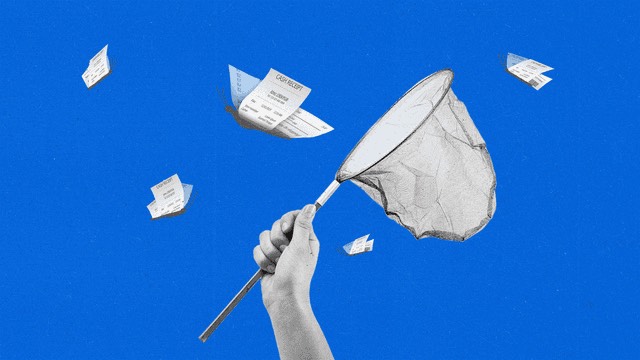
Below is a step-by-step guide that walks you through:
Exporting a Webflow project into a static template.
Preparing the exported files for TinaCMS usage.
Using an AI coding tool (like ChatGPT, GitHub Copilot, etc.) to assist in writing or modifying code where needed.
We’ll assume you already have a Webflow project that you can export. We’ll also assume some general familiarity with Git, Node.js, and a code editor.
1. Export Your Webflow Site
Open Your Project
Log into your Webflow dashboard.
Select the project you want to export.
Export the Code
Click on the “Project settings” or “Export code” button (depending on your Webflow plan and editor view).
Download the exported ZIP file. You will end up with a folder containing
index.html
(and possibly more pages), a CSS folder, a JS folder, and an images/assets folder.
Unzip the Export
Unzip the file.
Inside, you’ll see typical static assets:
/css style.css ... /js script.js ... /images (or /assets) logo.png ... index.html pages (if multiple pages exist)
At this point, you effectively have a static website version of your Webflow project.
2. Set Up a Local Development Environment for TinaCMS
TinaCMS can work with a variety of frameworks (Next.js, Gatsby, or even standalone static setups). The easiest approach is to integrate TinaCMS within a Next.js or Gatsby project, because TinaCMS frequently relies on a React environment.
Option A: Next.js + TinaCMS
Tip: If you prefer to keep the site fully static, you can still use Tina in “Cloud” mode or as a “Headless CMS” for static sites, but that requires more advanced configuration. For a straightforward approach, consider a Next.js setup so you can take advantage of dynamic editing routes and local content.
Initialize a new Next.js project
npx create-next-app my-tina-site cd my-tina-site
Install TinaCMS
npm install tinacms react-tinacms-editor --save
Copy Webflow Export into Your Project
You can place your static exports from Webflow into the Next.js
/public
folder or convert each HTML page into a Next.js page.For example, if you have
index.html
, you could rename it (or adapt it) intopages/index.js
in Next.js, copying over the<head>
,<body>
, and relevant markup.
Adjust paths
Ensure that references to CSS, JS, and images are updated. If you are moving your assets to the
/public
folder, you might do something like:<!-- Example updated link in Next.js page --> <link rel="stylesheet" href="/css/style.css" /> <script src="/js/script.js" defer></script> <img src="/images/logo.png" alt="Logo" />
If you have multiple pages, each page can be turned into its own
.js
file in thepages
directory, or you can do a simpler approach and keep them as static HTML in thepublic
folder.
Add TinaCMS configuration
Create a new
tina/config.ts
file for your Tina config (or.js
, but.ts
is recommended):import { defineConfig } from 'tinacms'; export default defineConfig({ clientId: "<Your TINA Client ID>", // from app.tina.io if you use Tina Cloud branch: "main", // or your Git branch token: "<Your TINA read/write token>", // for authenticated access to your repo build: { outputFolder: "admin", publicFolder: "public", }, media: { tina: { publicFolder: "public", mediaRoot: "images", }, }, schema: { collections: [ { name: "pages", label: "Pages", path: "content/pages", fields: [ { type: "string", name: "title", label: "Title", }, { type: "rich-text", name: "body", label: "Body", }, ], }, ], }, });
Generate Tina
You can generate the TinaCMS admin interface by running:
npx tina-cloud@latest build
This will produce a
/admin
folder (or whatever you set inbuild.outputFolder
in the config).
Enable Tina in Your Next.js Pages
In the
_app.js
file (or_app.tsx
if TypeScript):import '../styles/globals.css' import { TinaEditProvider } from 'tinacms/dist/edit-state' function MyApp({ Component, pageProps }) { return ( <TinaEditProvider editMode={<Component {...pageProps} />}> <Component {...pageProps} /> </TinaEditProvider> ) } export default MyApp
This wraps your app with the Tina edit context. When you navigate to
<your-site>/admin
, you’ll enter edit mode for Tina.
Option B: Standalone Static & TinaCMS (Advanced / Headless)
If you simply want to keep your site static without Next.js or Gatsby, you can still integrate Tina via its Cloud or self-hosted approach, but it involves extra steps. You’d generate your site locally, commit changes to a Git repo, then push them to your hosting. Tina can be configured to run as a “headless” CMS in which content is read from (and written to) your Git repository. This approach is a bit more advanced, so many people use Next.js or Gatsby to simplify it.
3. Use an AI Coding Tool to Assist
To use an AI coding tool (e.g., ChatGPT, GitHub Copilot, or similar) effectively, you can break down your tasks into “prompts” or “requests” for the AI. Here’s how:
Set Up Your Prompt
Provide the AI tool with context and the goal. For example:
“I have a Webflow-exported website with index.html, style.css, script.js, and images. I am creating a Next.js project to integrate TinaCMS. Please help me convert the static HTML and references into a Next.js-compatible page, maintaining the layout from the original HTML.”
Copy-Paste or Reference Your Code
Paste your HTML code into the AI’s text box (if it’s not too large) or reference which code the AI should modify.
If using GitHub Copilot, comment your code to instruct it:
// Copilot, convert the below Webflow layout into a Next.js page
Iterate and Refine
The AI might give you a rough conversion. Test it in your local environment.
If an error arises, copy the error message and the relevant code, then feed it back to the AI with a prompt like:
“I got this error when trying to load the page: [paste error]. Please help me troubleshoot.”
Ask for TinaCMS Integration Snippets
You can also ask the AI for example code on how to insert Tina fields into a Next.js page. For example:
“Show me how to add a TinaCMS schema for a homepage that includes a hero section, a heading, and a paragraph.”
Generate Additional Config
If you need advanced TinaCMS config (like multiple collections for blog posts, or specifying media uploads), instruct the AI:
“Add an additional collection for ‘blog’ with fields for title, date, and body. Make sure it’s integrated in my existing
tina/config.ts
.”
By prompting your AI tool step by step, you’ll streamline the process of converting your Webflow site into a React-based project (Next.js or other frameworks) and hooking it up to TinaCMS.
4. Testing & Deployment
Local Testing
Run your Next.js project locally:
npm run dev
Navigate to
http://localhost:3000
.Your site should be displayed with the design you brought in from Webflow.
TinaCMS Admin
Go to
http://localhost:3000/admin
.You should see the TinaCMS editor interface (if configured properly).
Any changes you make via Tina should update your local files or your Git-based content, depending on your configuration.
Production Deployment
Common Pitfalls
Path Issues
Make sure relative paths to images and CSS/JS are correct in your React/Next.js or static environment.
When placing files in
/public
, references become/filename.ext
.
Overwriting Markup
- Converting a large HTML file to a React file can be messy. Consider using partial components if the layout is complex.
TinaCMS Schema
- If your site has many pages or different content types, you’ll need a well-structured Tina schema. Start simple, then extend.
Git / Tina Collaboration
- If using Tina’s Git-based approach, ensure you have appropriate repo permissions. For Tina Cloud, ensure the tokens and client IDs are correct.
Putting It All Together
Export from Webflow – You now have static HTML, CSS, JS.
Set up Next.js / TinaCMS – For an easier dynamic experience.
Move or adapt your Webflow files to Next.js pages (or keep them in
/public
).Configure Tina with a
tina/config.ts
that points to your content collections.Use an AI coding tool to help refactor code and create Tina schemas. Provide the AI with clear prompts and relevant code segments.
Test locally – Access the admin interface at
/admin
.Deploy – Use your preferred hosting.
With these steps, you can retain your Webflow design while adding the power of TinaCMS for content editing. The AI coding tool is especially helpful for quickly converting large HTML segments to React, generating example TinaCMS config, and troubleshooting errors without extensive manual rewriting.
Example Prompt for the AI Tool
Here is an example prompt you could give to ChatGPT or a similar tool once you have your Next.js project up and running but need help integrating your exported Webflow code:
Prompt
I have a Next.js project that I created usingnpx create-next-app
. I have a Webflow export with the files:index.html
,/css/style.css
, and/js/script.js
. Please help me:
Convert
index.html
into apages/index.js
file for Next.js.Update references to styles and scripts so they work in Next.js by placing them in
/public/css
and/public/js
.Show me how to wrap the page with a TinaCMS EditProvider so that I can eventually edit text on the home page.
The AI tool should provide you with code or instructions that you can copy-paste (or adapt) to your local files. You can keep prompting it for adjustments if something doesn’t match your exact structure or needs.
Conclusion
Exporting a Webflow project as a static site is straightforward—just grab the exported HTML, CSS, JS, and images. Integrating with TinaCMS typically involves using a React-based framework like Next.js (or Gatsby). The AI coding tool can help you automate the conversion of large HTML files to React components, generate Tina schemas, and troubleshoot errors.
By following the steps above and iteratively refining your setup using the AI’s assistance, you can end up with a nicely edited (in real-time) static site that preserves your Webflow design while giving you or your editors a friendly, inline editing experience with TinaCMS. Good luck with your project!
Subscribe to my newsletter
Read articles from Erik Chen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
