Unlocking Java's Strategy Pattern: Streamline with Lambda Expressions

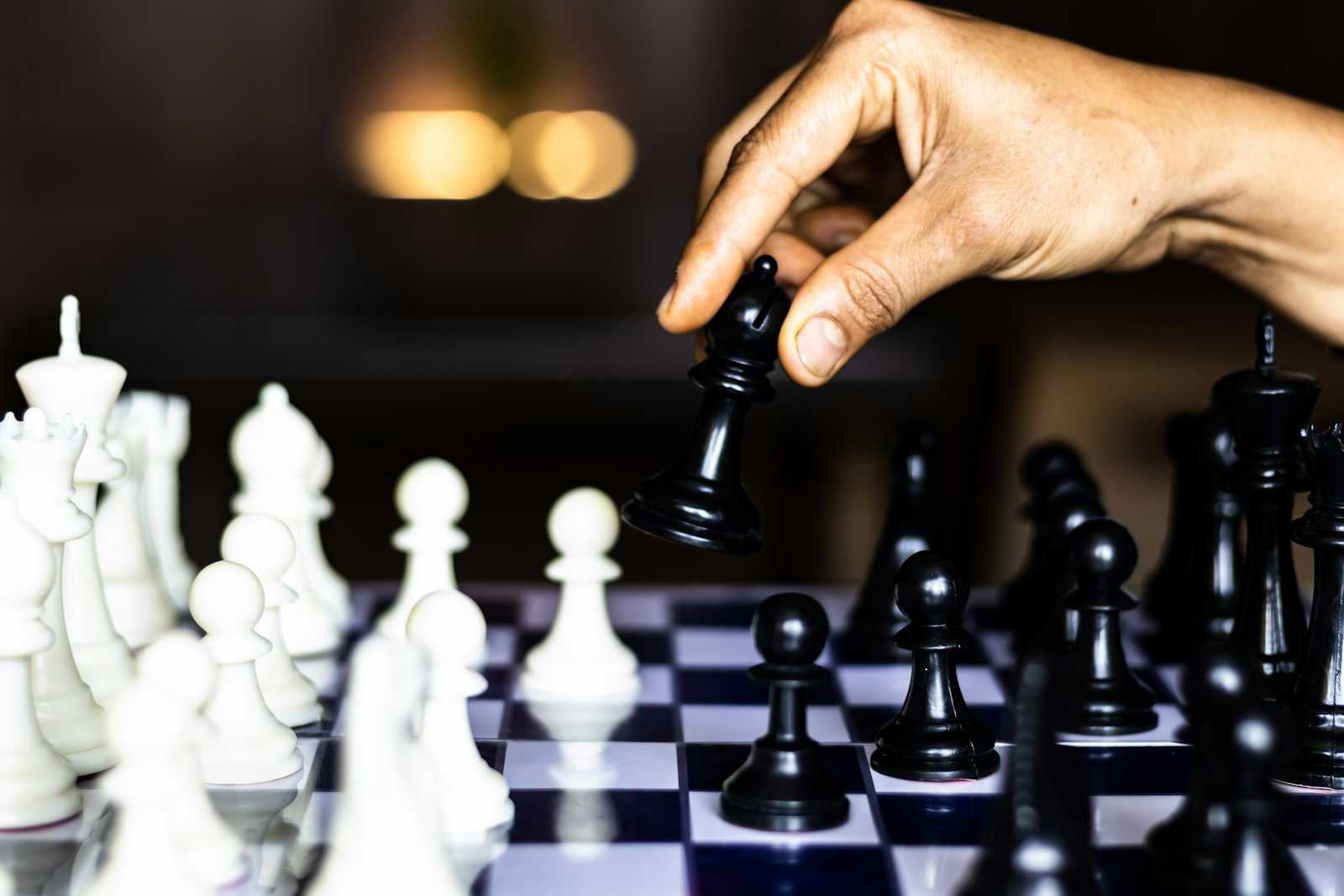
Introduction
Hey there! Let's dive into design patterns—specifically, the Strategy Pattern. Once you get the hang of it, you'll start seeing it everywhere. The Strategy Pattern is all about defining a group of algorithms, wrapping each one up, and making them easy to switch around. Sounds fancy, right? But honestly, traditional implementations can feel a bit... cumbersome. There's a lot of boilerplate code and extra classes just to change a behavior.
But here’s the good news: with modern Java and its sweet lambda expressions, we can make the Strategy Pattern lightweight, elegant, and, dare I say, fun to use.
Ready to see how it works? Let’s dive in!
The Problem
Alright, so picture this: you've got a service that calculates discounts. Sounds simple, right? But here's the catch—there are different types of discounts, and each one has its own rules. For instance:
Seasonal Discounts: Limited-time offers based on seasons or holidays.
Long-Term Client Discounts: Special rewards for loyal, long-term clients.
Staff Discounts: Perks for your awesome team members.
Student Discounts: Discounts for the studious crowd because education deserves a break.
And here's where things get tricky: each discount has its own dedicated method (see code). Now you're staring at four methods, each doing its own thing. Before you know it, you've got a service that's bloated with logic and harder to maintain than you'd like.
import java.util.List;
import java.util.stream.Collectors;
public class DiscountService {
public List<Item> applySeasonalDiscount(List<Item> items) {
return items.stream()
.filter(Item::isDiscountable)
.map(item -> {
double discountPercentage = 0.1;
discountPercentage += item.getPrice() > 500 ? 0.05 : 0.0;
double discountedPrice = item.getPrice() * (1 - discountPercentage);
return item.withPrice(discountedPrice);
})
.collect(Collectors.toList());
}
// Method to calculate discount for long-term clients
public List<Item> applyLongTermClientDiscount(List<Item> items) {
return items.stream()
.filter(Item::isDiscountable)
.map(item -> {
double discountPercentage = 0.15;
discountPercentage += item.getPrice() > 1000 ? 0.10 : 0.0;
double discountedPrice = item.getPrice() * (1 - discountPercentage);
return item.withPrice(discountedPrice);
})
.collect(Collectors.toList());
}
public List<Item> applyStaffDiscount(List<Item> items) {
return items.stream()
.filter(Item::isDiscountable)
.map(item -> {
double discountPercentage = 0.20;
double discountedPrice = item.getPrice() * (1 - discountPercentage);
return item.withPrice(discountedPrice);
})
.collect(Collectors.toList());
}
public List<Item> applyStudentDiscount(List<Item> items) {
return items.stream()
.filter(Item::isDiscountable)
.map(item -> {
double discountPercentage = 0.15;
discountPercentage += item.getPrice() < 200 ? 0.05 : 0.0;
double discountedPrice = item.getPrice() * (1 - discountPercentage);
return item.withPrice(discountedPrice);
})
.collect(Collectors.toList());
}
}
Want to add a new type of discount? Get ready to add yet another method and possibly risk messing with existing ones. Not exactly the cleanest, most flexible approach, huh?
Strategy design pattern
Using the Strategy Pattern is a fantastic way to improve the DiscountService
class. It makes the code more flexible and organized. Each discount type can have its own strategy, which keeps the code tidy, easier to maintain, and simple to expand.
Here's how the code might look:
Let's start by defining the strategy interface:
public interface DiscountStrategy {
double calculateDiscountedPrice(Item item);
}
An example of the strategy implementation :
public class SeasonalDiscountStrategy implements DiscountStrategy {
private final double DISCOUNT_PERCENTAGE = 0.1;
private final double EXPENSIVE_ITEM_DISCOUNT_PERCENTAGE = 0.15;
private final double EXPENSIVE_ITEM_THRESHOLD = 500.0;
@Override
public double calculateDiscountedPrice(Item item) {
double discountPercentage = item.getPrice() > EXPENSIVE_ITEM_THRESHOLD ?
EXPENSIVE_ITEM_DISCOUNT_PERCENTAGE
: DISCOUNT_PERCENTAGE;
return item.getPrice() * (1 - discountPercentage);
}
}
The new DiscountService
:
import java.util.List;
import java.util.stream.Collectors;
public class DiscountService {
private final DiscountStrategy discountStrategy;
public DiscountService(DiscountStrategy discountStrategy) {
this.discountStrategy = discountStrategy;
}
public List<Item> applyDiscount(List<Item> items) {
return items.stream()
.filter(Item::isDiscountable)
.map(item -> item.withPrice(discountStrategy.calculateDiscountedPrice(item)))
.collect(Collectors.toList());
}
}
So, here's the scoop—every time you want to add a new "strategy," you need to create a brand-new class. That means setting up a class, implementing the interface, and writing what might be just a few lines of code. If you have four types of discounts, congrats—you've just made four separate classes. And don't forget: each class needs its own file, which can clutter up your project.
But wait, there's more. Need to tweak something? You'll be hopping between multiple files just to find the logic. Even if the logic is small, the amount of boilerplate—like method headers, constructors, and imports—makes it feel like you're doing way more work than necessary. Sure, the Strategy Pattern is awesome for complex, reusable behavior, but when your strategies are simple and focused, all that extra structure can feel like carrying a backpack full of bricks to a picnic. It's sturdy but unnecessarily heavy for the task.
The lightweight approach
Instead of setting up a regular interface, let's go with a functional one. A functional interface is just an interface with a single abstract method.
@FunctionalInterface
public interface DiscountStrategy {
double calculateDiscountedPrice(double price);
}
In the new DiscountService
we pass the DiscountStrategy as a prameter, aka our lambda function.
import java.util.List;
import java.util.stream.Collectors;
public class DiscountService {
public List<Item> applyDiscount(List<Item> items, DiscountStrategy discountStrategy) {
return items.stream()
.filter(Item::isDiscountable)
.map(item -> item.withPrice(discountStrategy.calculateDiscountedPrice(item.getPrice())))
.collect(Collectors.toList());
}
}
Example of usage :
public class Main {
public static void main(String[] args) {
List<Item> items = List.of(
new Item("Laptop", 1200, true),
new Item("Book", 150, true),
new Item("TV", 600, false)
);
DiscountService discountService = new DiscountService();
List<Item> seasonalDiscountedItems = discountService.applyDiscount(items,
price -> price * (1 - price > 500 ? 0.15 : 0.1));
System.out.println("Seasonal Discounts: " + seasonalDiscountedItems);
}
}
As you can see, this approach is much more straightforward. The code clearly shows what it's doing, and adding a new discount strategy is easy. You don't need to create a new class; you can simply pass a lambda function with the logic, and the discountService will handle the calculation for you.
Which Approach to Choose?
Use the Traditional Strategy Pattern:
Go for this if you expect to have complex or reusable discount logic.
It's a good fit if each strategy needs extra behavior beyond just calculating discounts.
Use Functional Interfaces and Lambdas:
Choose this if the strategies are simple and don't need to be reused or have extra behavior.
It's great for concise, inline implementations in small-to-medium-sized projects.
Both approaches work well, and your choice should depend on how complex your project is and what it needs.
Conclusion
In conclusion, the Strategy Pattern is a fantastic tool in software design. It gives you flexibility and makes your code easier to maintain by letting you swap out different algorithms. The traditional way of doing this can be a bit clunky with lots of extra code. But, by using Java's lambda expressions and functional interfaces, you can simplify the Strategy Pattern, making it more efficient and easier to handle. This modern method not only cuts down on code complexity but also boosts readability and adaptability, especially for projects with straightforward strategies. Whether you go with the traditional method or the lambda-based approach depends on your project's complexity and needs, but both offer great ways to handle dynamic behavior in your applications.
Subscribe to my newsletter
Read articles from MAADA Loukmane directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

MAADA Loukmane
MAADA Loukmane
Moroccan software developer, Java/Spring. Love to learn, eager to write.