Understanding RAM, SSD, and HDD: Latency, Read/Write Speeds, and More
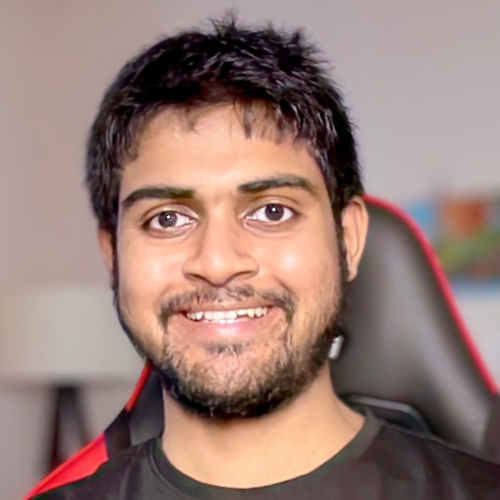
Table of contents
- Understanding RAM, SSD, and HDD: Latency, Read/Write Speeds, and More
- RAM, SSD, and HDD: Latency and Read/Write Speeds
- Understanding RAM: A Developer's Guide
- In JavaScript, variables are stored in memory (RAM), and the JavaScript engine manages memory allocation and deallocation automatically.
- Example: How Variables Store Data in RAM
- How it Works in RAM:
- How Objects Work (Reference in RAM)
- How it Works in RAM:
- Key Takeaways
- How Many Array Elements Can Fit in 1 KB RAM? ๐งฎ
- 1. char (Character) in JS
- 2. int (Integer) in JS
- 3. float (Floating-Point Number) in JS
- 4. double (Double Precision Floating-Point) in JS
- 5. pointer (Reference in JS)
- Key Takeaways
- What is Bandwidth in RAM?
- What is MT/s (MegaTransfers per Second)?
- RAM Paging and Virtual Memory
- What Does a CPU Do?
- How a CPU Works (Simplified Steps)
- Key CPU Components
- What is GHz (Gigahertz) in a CPU?
- How It Works
- Example:
- Does Higher GHz Always Mean Faster?
- What Are CPU Cores and How Do They Work?
- How Do CPU Cores Work?
- Single-Core vs Multi-Core CPUs
- Hyper-Threading (Intel) & Simultaneous Multi-Threading (AMD)
- Why Are More Cores Useful?
- Cache Memory: Faster than RAM
- What is NVMe in SSD?
- What is SATA in HDD/SSD?
- Understanding Storage Units (Comparing to 1 KB)
- Understanding Time Units (Comparing to 1 Second)
- Final Thoughts

Understanding RAM, SSD, and HDD: Latency, Read/Write Speeds, and More
RAM, SSD, and HDD: Latency and Read/Write Speeds
When choosing storage and memory for your system, understanding latency and read/write speeds is crucial. Here's how RAM, SSD, and HDD compare:
Latency Comparison (Lower is Better)
RAM: ~10 ns (nanoseconds) โก (Fastest)
NVMe SSD: ~0.02 ms (microseconds)
SATA SSD: ~0.1 ms
HDD: ~5 ms ๐ (Slowest)
Read/Write Speed Comparison (Higher is Better)
RAM (DDR4/DDR5): 25,000 - 50,000 MB/s ๐
NVMe SSD (PCIe 4.0/5.0): 3,000 - 14,000 MB/s โก
SATA SSD: 500 - 600 MB/s โก
HDD (7200 RPM): 80 - 160 MB/s ๐ข
Understanding RAM: A Developer's Guide
RAM (Random Access Memory) is a high-speed, volatile memory used by the CPU to temporarily store data needed for immediate execution. Unlike SSDs or HDDs, RAM allows fast read and write operations but loses data when power is turned off.
RAM Addressing and Storage
Each byte in RAM has a unique memory address that allows the CPU to locate and retrieve data efficiently. The memory is structured as a large array, where each word (usually 4 or 8 bytes) is assigned an address in hexadecimal format (e.g., 0x00000000
).
In memory, the variable (word
) points to the first character ('H'
), and each character points to the next one stored in the next memory block.
This happens because strings are stored as contiguous blocks in memory, and each character is placed at the next sequential memory address.
How JavaScript Handles This Internally
In low-level languages like C, this is explicitly managed with pointers.
In JavaScript, the engine manages memory references automatically.
When you do:
let word = "hello";
word
stores a reference to the first character ('h'
).The JavaScript engine tracks the entire string and retrieves the full value when needed.
Key Takeaways
โ
Each character in memory points to the next one.
โ
The variable stores a reference to the first character.
โ
JavaScript handles memory automatically, so you donโt need to manage pointers manually.
Why is Understanding RAM Important for Developers?
For developers, understanding RAM is crucial for optimizing performance in programming, especially in:
Memory Management โ Efficient allocation and deallocation prevent memory leaks.
Multithreading & Concurrency โ Proper memory usage improves parallel processing.
Data Caching โ Faster access to frequently used data improves performance.
Low-Level Optimization โ Helps in embedded systems, gaming, and high-performance computing.
Types of RAM
SRAM (Static RAM) โ Faster, used for CPU caches.
DRAM (Dynamic RAM) โ Slower but cost-effective, used in system memory.
DDR (Double Data Rate RAM) โ The most common type, evolving through DDR1 to DDR5.
RAM Type | Year | Speed (MT/s) | Bandwidth (GB/s) |
DDR3 | 2007 | 800 - 2133 | 6.4 - 17.0 GB/s |
DDR4 | 2014 | 1600 - 3200 | 12.8 - 25.6 GB/s |
DDR5 | 2021 | 3200 - 8400 | 25.6 - 67.2 GB/s |
In JavaScript, variables are stored in memory (RAM), and the JavaScript engine manages memory allocation and deallocation automatically.
Example: How Variables Store Data in RAM
let x = 10;
let y = x;
y = 20;
console.log(x); // 10
console.log(y); // 20
How it Works in RAM:
let x = 10;
โ A memory block is assigned in RAM forx
, and10
is stored in that block.let y = x;
โ A new memory block is created fory
, and the value ofx
(10
) is copied.y = 20;
โ The value iny's
memory block is updated to20
, butx
remains10
.
Variable | Memory Address | Stored Value |
x | 0x1000 | 10 |
y | 0x1004 | 20 |
Since numbers (primitives) are stored by value, modifying y
does not affect x
.
How Objects Work (Reference in RAM)
Unlike primitive values, objects are stored in RAM by reference, meaning variables store a memory address pointing to the object, not the actual data.
Example: Objects in RAM
let obj1 = { value: 10 };
let obj2 = obj1; // obj2 gets a reference to the same object in RAM
obj2.value = 20; // Modifies the same memory block
console.log(obj1.value); // 20
console.log(obj2.value); // 20
How it Works in RAM:
obj1
is created and stored in heap memory withvalue: 10
.obj2 = obj1;
does not create a new object, instead,obj2
gets a reference toobj1
's memory.Changing
obj2.value
modifies the same memory block.
Variable | Memory Address | Stored Value |
obj1 | 0x2000 | { value: 20 } |
obj2 | 0x2000 | (same object reference) |
Thus, obj1
and obj2
point to the same object in RAM.
Key Takeaways
Primitive types (numbers, strings, booleans) store values directly in memory.
Objects and arrays store a reference (address) in memory, and modifying one reference affects all others.
Understanding how variables reference memory helps in avoiding unintended side effects, especially in large applications.
How Many Array Elements Can Fit in 1 KB RAM? ๐งฎ
To determine how many elements we can store in 1 KB (1024 Bytes) of RAM, we need to consider the data type size of the elements.
Data Type | Size per Element | Max Elements in 1 KB (1024 Bytes) |
Char (char ) | 1 Byte | 1024 elements |
Integer (int ) | 4 Bytes | 256 elements |
Float (float ) | 4 Bytes | 256 elements |
Double (double ) | 8 Bytes | 128 elements |
Pointer (void* ) | 8 Bytes (64-bit systems) | 128 elements |
Thus, the number of elements depends on the data type you're using.
In JavaScript, data types are a bit different from low-level languages like C, as JavaScript is dynamically typed. However, here's how you can relate char, int, float, double, and pointers in JavaScript.
1. char
(Character) in JS
JavaScript does not have a separate
char
type like C.A character is just a string of length 1.
Example:
let charExample = 'A'; // A character in JS is just a string with one letter
console.log(typeof charExample); // Output: 'string'
2. int
(Integer) in JS
JavaScript does not have a specific integer type like C (
int
).Instead, all numbers (integers or floats) are of type
number
.
Example:
let intExample = 42; // Integer value
console.log(typeof intExample); // Output: 'number'
3. float
(Floating-Point Number) in JS
JavaScript stores all numbers as floating-point (64-bit IEEE 754).
Thereโs no distinction between
int
andfloat
.
Example:
let floatExample = 3.14; // Decimal number
console.log(typeof floatExample); // Output: 'number'
4. double
(Double Precision Floating-Point) in JS
In JavaScript, all numbers (including decimals) use double-precision floating-point (
64-bit
).No need to specify
double
explicitly, as JS does it automatically.
Example:
let doubleExample = 99.9999999999; // JS stores all numbers in double precision
console.log(typeof doubleExample); // Output: 'number'
5. pointer
(Reference in JS)
JavaScript does not have pointers like C/C++.
However, objects, arrays, and functions are stored by reference, which is similar to pointers.
Example:
let obj1 = { value: 10 };
let obj2 = obj1; // obj2 now references the same memory location as obj1
obj2.value = 20; // Changing obj2 affects obj1 as well
console.log(obj1.value); // Output: 20
console.log(obj2.value); // Output: 20
Here, obj2
does not copy obj1
, it references the same memory address, like a pointer in C.
Key Takeaways
Type | JavaScript Equivalent | Notes |
char | 'A' (String) | JavaScript does not have a separate char type. |
int | 42 (Number) | JS does not distinguish between int and float. |
float | 3.14 (Number) | Stored as a floating-point number. |
double | 99.99 (Number) | All numbers in JS use 64-bit double precision. |
pointer | Object reference | Objects and arrays in JS behave like pointers. |
Since JavaScript is dynamically typed, all numbers (int
, float
, double
) are of type number
, and objects behave like pointers.
Would you like a deeper explanation of memory references and garbage collection in JavaScript? ๐
What is Bandwidth in RAM?
Bandwidth measures how much data can be transferred per second between RAM and the CPU.
Formula: Bandwidth=Speed (MT/s)รBus Width (Bytes)ร2\text{Bandwidth} = \text{Speed (MT/s)} \times \text{Bus Width (Bytes)} \times 2
Example for DDR4-3200: 3200ร8ร2=51.2 GB/s3200 \times 8 \times 2 = 51.2 \text{ GB/s}
What is MT/s (MegaTransfers per Second)?
MT/s represents how many million data transfers happen per second in RAM. Since DDR RAM transfers twice per cycle, MT/s is twice the clock speed (MHz).
RAM Type | Clock Speed (MHz) | Effective Speed (MT/s) |
DDR3-1600 | 800 MHz | 1600 MT/s |
DDR4-3200 | 1600 MHz | 3200 MT/s |
DDR5-6400 | 3200 MHz | 6400 MT/s |
RAM Paging and Virtual Memory
When RAM runs out of space, the OS uses virtual memory (stored on the disk) as a temporary extension. This process, called paging, slows performance since SSDs and HDDs are much slower than RAM.
What Does a CPU Do?
The CPU (Central Processing Unit) is the brain of the computer. It executes instructions, performs calculations, and manages data flow between components.
How a CPU Works (Simplified Steps)
Fetch โ Retrieves an instruction from RAM.
Decode โ Interprets the instruction.
Execute โ Performs the operation (math, logic, moving data).
Store โ Saves the result in memory or registers.
This cycle repeats billions of times per second (measured in GHz).
Key CPU Components
Component | Function |
ALU (Arithmetic Logic Unit) | Performs calculations & logic operations. |
CU (Control Unit) | Directs how instructions are processed. |
Registers | Small, fast memory inside CPU for quick data access. |
Cache Memory | Stores frequently used data to speed up tasks. |
Cores | Independent processing units inside the CPU (dual-core, quad-core, etc.). |
What is GHz (Gigahertz) in a CPU?
GHz (Gigahertz) measures the clock speed of a CPU, indicating how many cycles it can execute per second.
1 GHz = 1 billion cycles per second (1,000,000,000 Hz).
How It Works
A CPU cycle is a single operation (fetch, decode, execute, store).
Higher GHz means the CPU can process more instructions per second.
Example:
2.5 GHz CPU โ 2.5 billion cycles per second
4.0 GHz CPU โ 4.0 billion cycles per second (faster)
Does Higher GHz Always Mean Faster?
โ Not necessarily! Performance also depends on:
Number of cores (More cores = better multitasking).
CPU architecture (Efficiency, power consumption).
Cache size & memory speed.
What Are CPU Cores and How Do They Work?
A CPU core is an independent processing unit inside the CPU that executes tasks.
Modern processors have multiple cores, allowing them to handle multiple tasks simultaneously (multitasking).
How Do CPU Cores Work?
Each core follows the Fetch-Decode-Execute Cycle to process instructions:
Fetch โ Retrieves an instruction from memory (RAM).
Decode โ Converts the instruction into a format the CPU understands.
Execute โ Performs the instruction (calculations, data movement, logic).
Store โ Saves the result in registers or RAM.
This process happens billions of times per second (measured in GHz).
Single-Core vs Multi-Core CPUs
Feature | Single-Core CPU | Multi-Core CPU |
Processing Power | Handles one task at a time | Handles multiple tasks at once |
Performance | Slower for multitasking | Faster for multitasking |
Heat & Power Usage | Uses less power but bottlenecks on heavy tasks | More efficient for modern software |
Example:
A Dual-Core CPU can process two tasks at the same time.
A Quad-Core CPU can process four tasks simultaneously.
An Octa-Core CPU can handle eight threads at once.
Hyper-Threading (Intel) & Simultaneous Multi-Threading (AMD)
Threads are virtual cores created by splitting a physical core into two logical units.
A Quad-Core CPU with Hyper-Threading acts like 8 logical cores, improving efficiency.
Benefit: Boosts performance in multi-threaded applications like gaming, video editing, and AI.
Why Are More Cores Useful?
โ
Multitasking โ Running multiple apps smoothly.
โ
Faster Processing โ Complex tasks (video editing, programming, AI, etc.).
โ
Gaming & Graphics โ Modern games use multiple threads for physics, AI, and rendering.
Cache Memory: Faster than RAM
CPUs have built-in L1, L2, and L3 cache to store frequently used instructions. These are even faster than RAM but have much smaller capacities (few MBs).
What is NVMe in SSD?
NVMe (Non-Volatile Memory Express) is a protocol for SSDs that connects via PCIe, offering low latency and high-speed data transfers compared to SATA SSDs. NVMe SSDs can reach speeds of 7,000+ MB/s, whereas SATA SSDs max out around 600 MB/s.
What is SATA in HDD/SSD?
SATA (Serial ATA) is a storage interface used in HDDs and SSDs. While still widely used, SATA is much slower than NVMe, with HDDs reaching around 160 MB/s and SATA SSDs around 600 MB/s.
Understanding Storage Units (Comparing to 1 KB)
Unit | Value | Comparison to 1 KB |
Bit (b) | Smallest unit | 1/8 of a Byte |
Byte (B) | 8 bits | 1 Byte = 8 bits |
Kilobyte (KB) | 1024 Bytes | 1 KB = 1024 Bytes |
Megabyte (MB) | 1024 KB | 1 MB = 1,024 KB |
Gigabyte (GB) | 1024 MB | 1 GB = 1,024 MB |
Terabyte (TB) | 1024 GB | 1 TB = 1,024 GB |
Understanding Time Units (Comparing to 1 Second)
Unit | Value | Comparison to 1 Second |
Millisecond (ms) | 1/1,000 sec | 1000 ms = 1 sec |
Microsecond (ยตs) | 1/1,000,000 sec | 1,000,000 ยตs = 1 sec |
Nanosecond (ns) | 1/1,000,000,000 sec | 1,000,000,000 ns = 1 sec |
Picosecond (ps) | 1/1,000,000,000,000 sec | 1,000,000,000,000 ps = 1 sec |
Final Thoughts
For developers, understanding RAM helps optimize software performance. Knowing how memory is allocated, accessed, and managed is key to writing efficient code. When optimizing applications, consider:
Using the right data structures to minimize memory usage.
Optimizing cache utilization for high-speed processing.
Avoiding excessive paging to prevent slowdowns.
With this knowledge, developers can design faster, more efficient applications! ๐
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
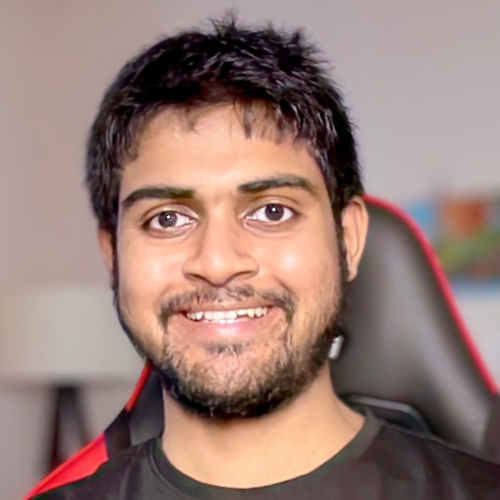
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.