Methods for Implementing Feature Flag Management in Your Spring Boot Application
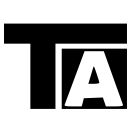
4 min read
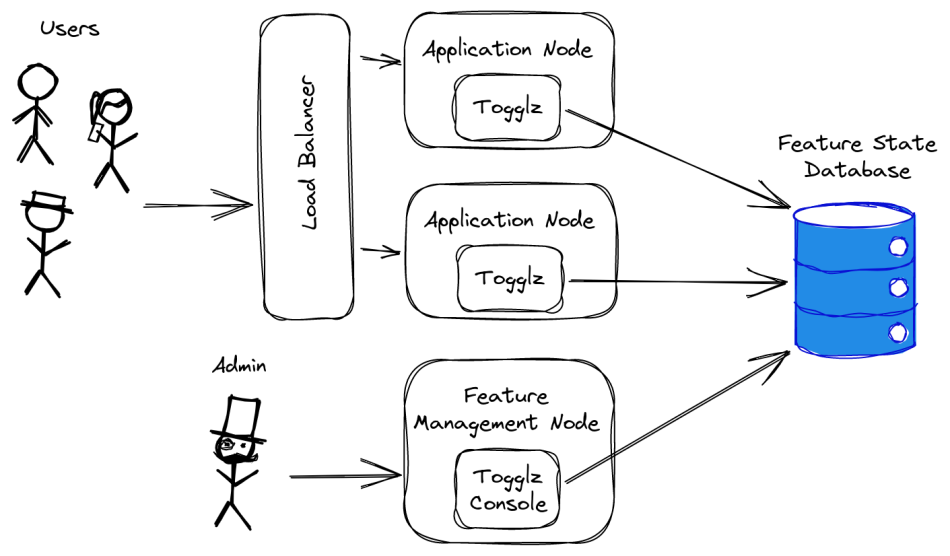
1. What is Togglz, and Why Use It for Feature Flag Management?
Feature flags help developers and product managers control the exposure of new features to users. Togglz is an open-source Java library that simplifies feature flagging by providing an easy-to-use API and UI for managing features. It integrates seamlessly with Spring Boot, making it a perfect choice for Java developers.
1.1 Benefits of Using Feature Flags
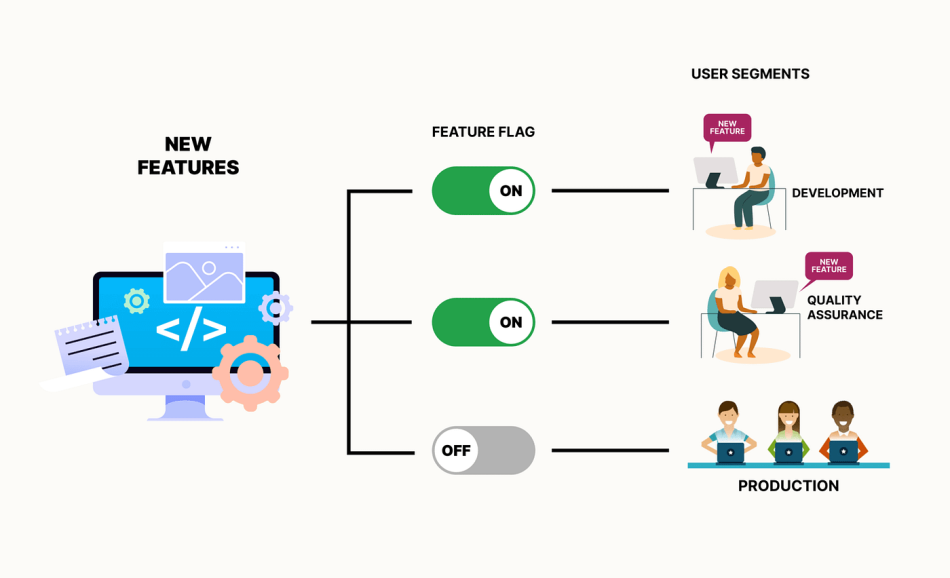
Feature flags offer several benefits in software development, such as:
- Continuous Deployment: Deploy code changes without releasing them to users.
- A/B Testing: Run experiments to test different variations of a feature.
- Gradual Rollout: Introduce new features to a small group of users and gradually increase the audience.
- Quick Rollback: Instantly disable a feature if a problem arises, without requiring a new deployment.
1.2 Why Choose Togglz?
Togglz offers a robust solution for feature flag management with the following advantages:
- Integration with Spring Boot: Togglz provides Spring Boot starters that simplify the setup process.
- Flexible Storage Options: It supports various storage options for feature states, including in-memory, file-based, and database-backed implementations.
- Built-in Admin Console: A web-based admin console for managing feature flags at runtime.
- API Support: Provides APIs to manage feature flags programmatically.
2. Setting Up Togglz in a Spring Boot Application
To implement feature flag management in your Spring Boot application using Togglz, you need to integrate it into your project, configure the necessary settings, and create feature toggles.
2.1 Adding Togglz Dependency
First, add the Togglz Spring Boot starter dependency to your pom.xml if you're using Maven:
<dependency>
<groupId>org.togglz</groupId>
<artifactId>togglz-spring-boot-starter</artifactId>
<version>2.9.8.Final</version>
</dependency>
For Gradle, add the following dependency:
implementation 'org.togglz:togglz-spring-boot-starter:2.9.8.Final'
2.2 Defining Features
Create an enum to define the features that you want to manage using Togglz:
package com.example.featureflags;
import org.togglz.core.Feature;
import org.togglz.core.annotation.EnabledByDefault;
import org.togglz.core.annotation.Label;
public enum MyFeatures implements Feature {
@Label("New User Dashboard")
@EnabledByDefault
NEW_USER_DASHBOARD,
@Label("Beta Feature")
BETA_FEATURE;
}
2.3 Configuring Togglz
You need to create a configuration class to set up Togglz with your feature enum:
package com.example.featureflags;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.togglz.core.spi.FeatureProvider;
import org.togglz.core.util.NamedFeature;
import org.togglz.spring.boot.actuate.autoconfigure.TogglzAutoConfiguration;
@Configuration
public class TogglzConfig {
@Bean
public FeatureProvider featureProvider() {
return () -> MyFeatures.class;
}
}
2.4 Enabling the Togglz Console
To use the Togglz console for managing feature flags, add the following property to your application.properties or application.yml:
togglz.console.enabled=true
togglz.console.path=/togglz-console
You can access the Togglz console at http://localhost:8080/togglz-console to manage your feature flags through a UI.
3. Managing Features Programmatically with API Calls
Besides using the UI, you may want to manage feature flags programmatically through API calls. This section shows how to build REST endpoints for this purpose.
3.1 Creating a Feature Toggle Controller
You can create a Spring MVC controller to expose endpoints that interact with Togglz:
package com.example.featureflags;
import org.springframework.web.bind.annotation.*;
import org.togglz.core.manager.FeatureManager;
@RestController
@RequestMapping("/api/features")
public class FeatureToggleController {
private final FeatureManager featureManager;
public FeatureToggleController(FeatureManager featureManager) {
this.featureManager = featureManager;
}
@GetMapping("/{feature}")
public boolean isFeatureActive(@PathVariable String feature) {
return featureManager.getFeatureState(() -> feature).isEnabled();
}
@PostMapping("/{feature}/{state}")
public void updateFeatureState(@PathVariable String feature, @PathVariable boolean state) {
featureManager.setFeatureState(() -> feature, state);
}
}
3.2 Testing the API Endpoints
Use Postman or any other API client to test the endpoints. You can check the status of a feature flag or update it via API calls:
- Get Feature Status: GET /api/features/NEW_USER_DASHBOARD
- Update Feature Status: POST /api/features/NEW_USER_DASHBOARD/true
4. Conclusion
Implementing feature flag management in your Spring Boot application using Togglz offers great flexibility and control over feature rollouts. By integrating with both API calls and a UI, you provide developers and product managers multiple avenues to manage feature toggles effectively. If you have any questions or need further clarification, feel free to comment below.
Read more at : Methods for Implementing Feature Flag Management in Your Spring Boot Application
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
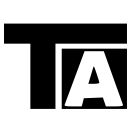
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.