Docker Volumes: With Real-Time Examples

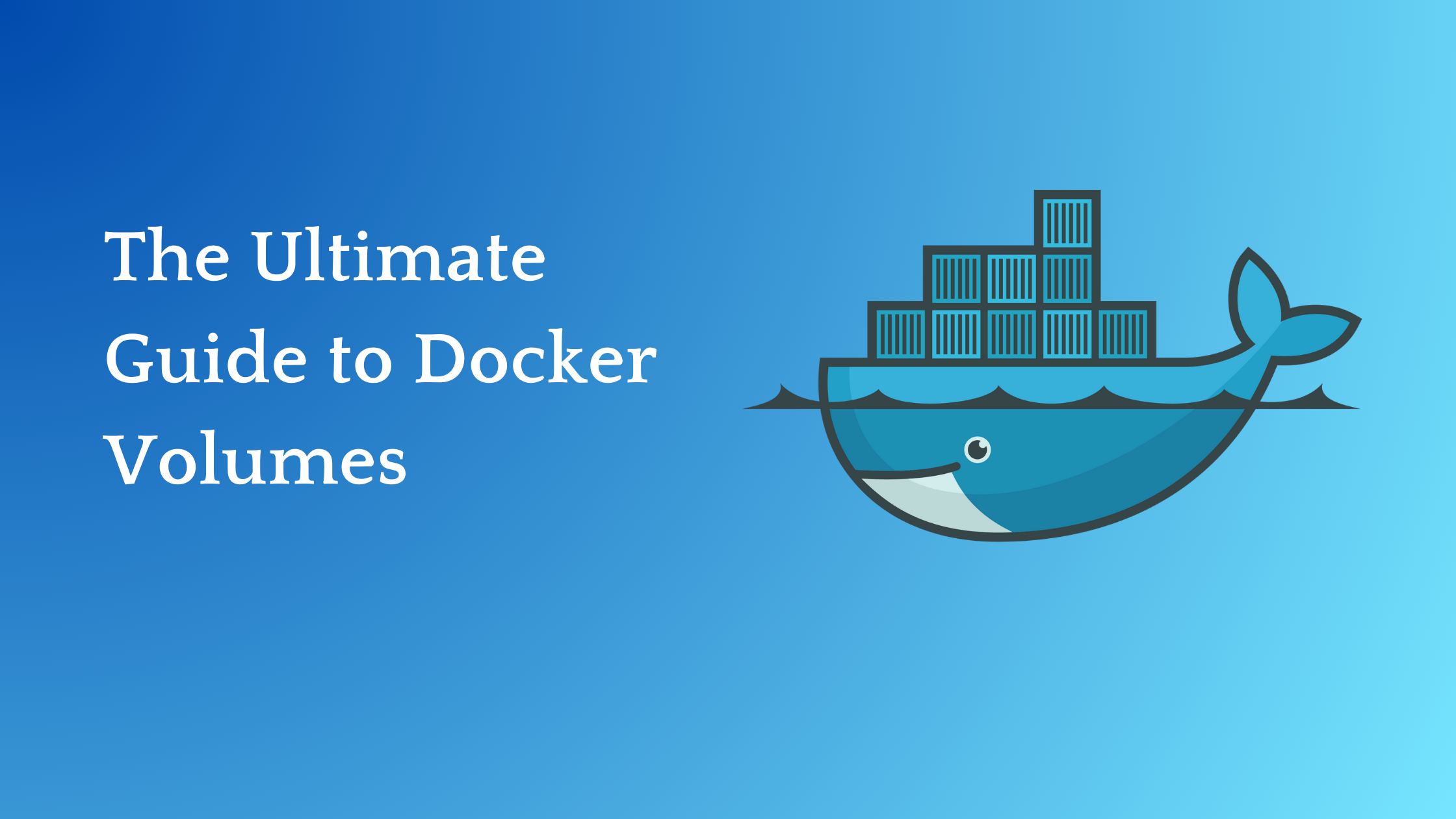
While working with docker, I observed that these are the 4 pillars for docker [Images, Containers, Volumes and Networks], In this blog we are going to discuss about Docker volumes. Docker volumes are the preferred way to persist data generated by and used by Docker containers. In this blog, we'll break down the anatomy of Docker volumes and demonstrate their use with practical, real-world examples.
What Are Docker Volumes?
Docker Volume is a mechanism that helps us to store data outside the container. They prevent data loss when your container stops. You can easily connect the volume to a new container, and your data from the previous one will carry over to the new container.
When a container writes data, it typically writes to its writable layer. However, this data is temporary and will be lost when the container is stopped or removed. To avoid this, Docker allows you to mount a volume to a specific directory in the container's file system. If any data we write inside the container, it automatically stores the data on volume. So the data will present even the container is deleted.
To better understand this, think of containers as whiteboards used during meetings. When the meeting ends (the container stops), the whiteboard is wiped clean (data is lost). A Docker volume is like a notebook where all important notes (data) are stored securely, ready to be referenced even after the meeting.
Production Issues:
In production, we usually maintain multiple containers for a single service, its hard to maintain the configurations upto date for all containers. Docker volume helps us to share the data between multiple containers. If we update the data on one container, it will replicate to all the containers.
Common commands in volumes:
To create a volume:
docker volume create volume-name
To see the list of volumes:
docker volume ls
To see the full details about volume:
docker volume inspect volume-name
To delete a volume:
docker volume rm volume-name
NOTE: We can’t remove the volumes which are attached to containers. It should be free from container.
To delete multiple volumes:
docker volume rm volume-name volume-name volume-name
To delete unused volumes:
docker volume prune
Practical implementation of volumes:
Lets create a volume and mount it to container:
docker volume create swiggy
Now i have created a swiggy volume
To inspect the volume:
[root@ip-172-31-13-80 ~] docker volume inspect swiggy
[
{
"CreatedAt": "2025-01-25T08:17:45Z",
"Driver": "local",
"Labels": null,
"Mountpoint": "/var/lib/docker/volumes/swiggy/_data",
"Name": "swiggy",
"Options": null,
"Scope": "local"
}
]
Now we can see the mountpoint: /var/lib/docker/volumes/swiggy/_data
Lets mount it to container:
docker run -itd --name cont1 --mount src=swiggy,destination=/myapp ubuntu
Now the container will be created, inside the container /myapp folder will be there. Lets go to container check it.
Go to container:
docker exec -it cont1 bash
Now we will enter into the container, we can see myapp folder is there.
Lets add some files and folders inside the myapp folder
cd myapp
touch file{1..4}
mkdir devops{1..3}
ls
Now exit from the container and check the swiggy volume, the data is present on volume.
Go to volume path
cd /var/lib/docker/volumes/swiggy/_data/
now check the files:
Even if we add some files here, then the data will replicate inside the container.
Now lets go to container1 and check the files present in myapp folder.
NOTE: So we can clearly observe that the data is replicated between container to host. we can mount our volumes to multiple containers to replicate the same process.
Host to Container:
Bind mounts have been part of Docker since the beginning. They help us to link a file or folder from our Docker host to the container’s file system. If the file or folder doesn’t already exist in the container, Docker will create it automatically when we start the container. Any changes you make to that file or folder on our Docker host will show up in the container, and any changes made inside the container will show up on our Docker host.
However, this can create security risks. For example, if something inside the container changes a file, that change will also affect the file on our Host. So, you need to be careful when using bind mounts.
Bind mounts are especially useful during development. You can link your code folder to the container, so when you update your code, the changes will appear in the container immediately. This saves time since you don’t have to rebuild the image every time you make a change.
Practical implementation of Bind mounts:
lets create a folder in Dockerhost
mkdir zomato && cd zomato
now create some files
touch index.html app.py style.css requirements.txt
Now lets create the container inside the same directory:
docker run -itd --name cont2 -v "$(pwd)":/appcode ubuntu
Now lets get into the container and check the same files
docker exec -it cont2 bash
cd appcode
Now lets create some files inside the container, that will replicate in Dockerhost on the same zomato folder
now lets exit from container and check in docker host
So here also the data is replicated on between host to container. We can create multiple containers from same path to replicate the data to containers.
In the above 2 models, we observed that data replication is like a 2 way [host to container, container to host]. This can create security risks. For example, if something inside the container changes a file, that change will also affect the file on our Host. So, we need to restrict by preventing the changes on container we need to create readonly volumes.
Read Only Volumes
We can make a volume read-only so that the container can read from that volume and can not write. It can be used if you have any security concerns depending upon the requirement of the application. These volumes will create while creating the container only
command to create a container with read only volume:
docker run -itd --name mustafa -v uber:/app:ro ubuntu
In the above command, uber is the volume that will created on host, and /app is the path where we mounted inside the container. ro represents the readonly volume.
Now lets enter into the container and add some data on /app folder, we will get permission denied error.
docker exec -it mustafa bash
cd app
see, we cant write the data from container, but if we write the data on uber volume in docker host, it will replicate here.
Sharing the volumes from container-container
we can share the volume from one container to another container while creating.
docker run -itd --name devops --privileged=true --volumes-from=mustafa ubuntu
In the above command, we can see the volumes from mustafa container is going to share with devops container.
Creating a volume using Dockerfile
Not only with the commands, we can also create the docker volumes with Dockerfile
FROM ubuntu
VOLUME [/mycode]
If we build the above Dockerfile and run the image, automatically a container and a random volume will be created. Now the volume will present inside the volume, when ever we run the image, automatically this volume will creates.
Examples of docker volumes
Example-1:
create a volume
docker volume create paytm
go to that volume path
/var/lib/docker/volumes/paytm/_data/
add some code in that volume
Now lets create a container by mounting the volume
docker run -itd --name freelancer -p 1234:80 --mount src=paytm,destination=/usr/local/apache2/htdocs/ httpd
Now lets access the application using 1234 port
We can share this volume to any container.
Example-2:
Create a folder on Docker host
mkdir testapp && cd testapp
Keep the code inside the folder
Now create a container from the same folder
docker run -itd --name cont99 -p 1122:80 -v "$(pwd)":/usr/share/nginx/html/ nginx
Now lets access the application using 1122 port
Example-3:
We can directly create a container and volume first
docker run -itd --name app-container -p 2233:80 -v mydata:/usr/share/nginx/html nginx
If we access the app using 2233 port, we will get only nginx page
Lets add the data on mydata volume
cd /var/lib/docker/volumes/mydata/_data/
make some modifications on index.html file and refresh the browser
Conclusion
Hope you found this blog helpful. I have tried to cover all the basics about volume or mount you need to know as beginners. There are many more things about this topic that I will cover in future blogs.
Give me your heart 💖
If you found this blog helpful for your interviews or in learning Docker troubleshooting, please hit a heart for 10 times and drop a comment! Your support motivates me to create more content on DevOps and related topics. ❤️
If you'd like to connect or discuss more on this topic, feel free to reach out on LinkedIn.
Linkedin: linkedin.com/in/musta-shaik
Subscribe to my newsletter
Read articles from Shaik Mustafa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
