Day 10 of C:Basic to Advanced : Strings

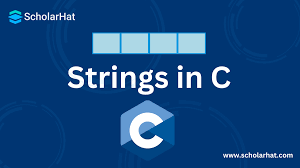
INTRODUCTION
String is basically a sequence of characters or symbols between a pair of double quotes “__” in which the characters of different data types can also be stored in a string.
Strings basically stores every character/symbol of the given string in a specific block which thereby carries out a specific size for that block like for int=4 bytes ,etc.
String can also be accessed in single quotes ‘__’ to assigning a character array.
For performing various string operations we shall use the header file “string.h“
There is no special type of variables for strings, Strings are stored in the form of arrays.
Steps of Declaring an String
Initializing a String
Assigning a value
Displaying a string
INITIALIZING A STRING
The first step is to declare an array(string)
You can also initialize the size of the string if you need.
If you are assigning the string characters separately it is better to assigning the size of string. It is also good if you are asking for input from the user to enter the input string into the program so that the user can know what is his last limit.
It is too ok to give that task to the compiler to calculate the size of the string.
ASSIGNING A VALUE
- You can perform an assignment operation , and you can assign one array of characters to another array of characters like other interpreter languages like Python using strcpy().
DISPLAYING A STRING
You can display a string by calling it out or using printf().
Also we can display a string like performing various operations on the string.
strcpy(): It is used to copy a string value and assigned it to the other string.
strcat(): It is used to concatenate(add) two or more strings together.
sizeof() or strlen(): It is used to calculate how much is the length of the given string.
strcmp(): It is used to compare which string is larger by comparing both.
#include<stdio.h>
#include<string.h>
int main()
{
char car1[]="bmwM3GTR";
char car2[]="ShelbyMustang350";
char nfs;
printf("Best Racing Car:%s\n",car1); //Accessing String
printf("%d\n",sizeof(car2));
printf("Rivals:%s",strcat(car1,car2));
strcpy(car1,nfs);
printf("Gaming Car is:%s\n",nfs);
printf("Best is:%s\n",strcmp(car1,car2));
return 0;
)
//Reversing a String
#include<stdio.h>
#include<string.h>
int main()
{
char prog[10]="hello"
int i,j;
j=sizeof(prog[10]);
printf("Size of String is:%d\n",j);
reverse();
return 0;
}
int reverse(int i,int j)
{
for(i=j;i>=0;i--)
{
printf(i);
}
}
PREPROCESSORS
The Preprocessor lets you define constants and is defined with library
#define name=value (Synatax)
#define PIE=3.1457..
We can use wherever we want as it accessible even before assigning the value.
CONSTANTS
keyword: const
constants are the fixed value used throughout the program
Synatax: const name=value;
We will learn about further operations on Strings with pointers as we progress through the upcoming topics. ⏭️
Connect me on
Subscribe to my newsletter
Read articles from Soham Sandbhor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
