New core 'Types' added

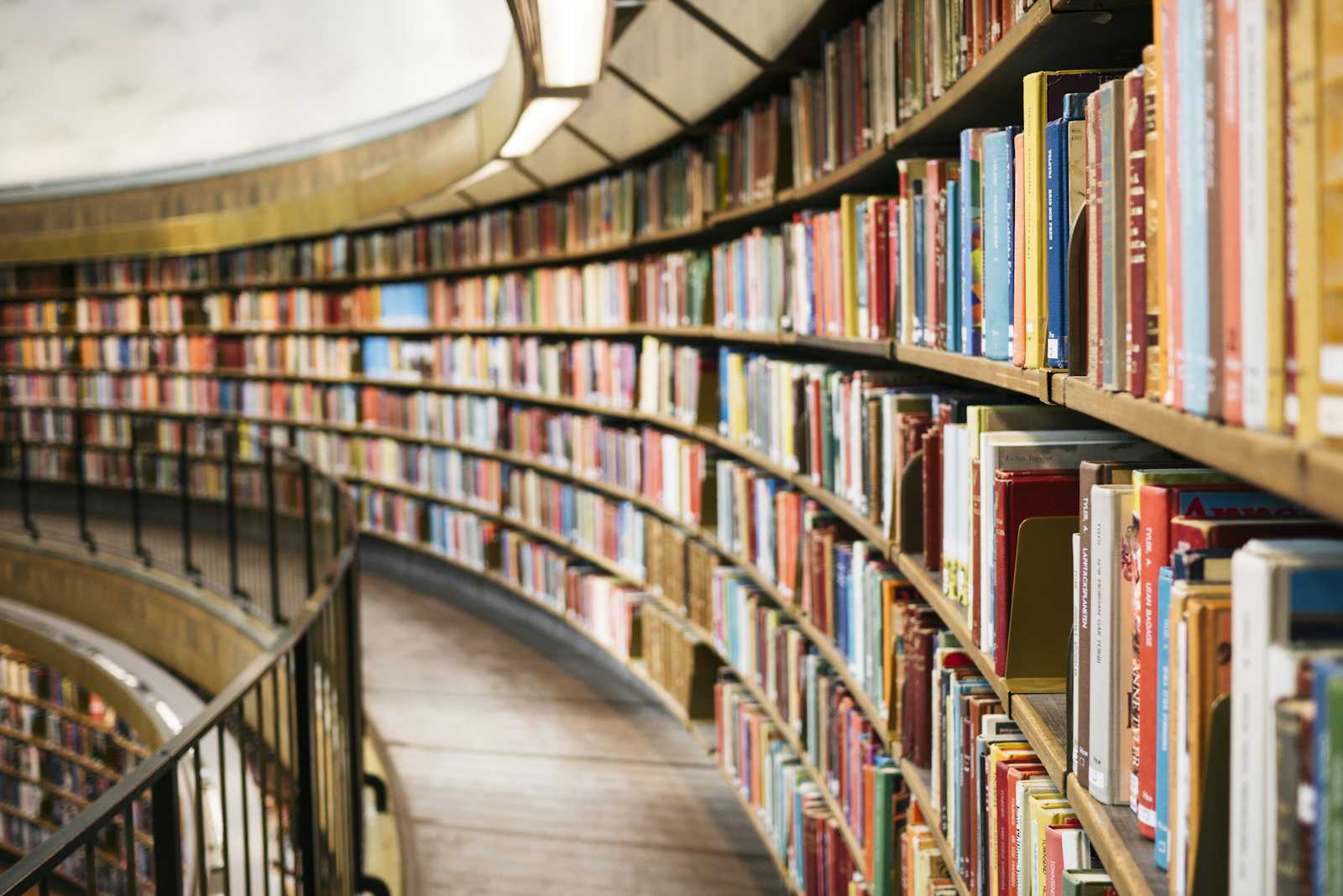
Well it’s been ages since I last wrote any blog articles, but I’ve been pretty busy on EK9 development and it has moved on in leaps and bounds.
So here’s a quick update on what’s been added and why.
I have also adjusted the type inference mechanism to be able to deal with simple expressions for the types on function returns, method returns and properties. This can now deal with simple Constructors and enables a sightly simpler syntax. See the ‘SteveSupplier’ and ‘StephenSupplier’ functions for examples of this.
Any Type
At first when I designed EK9 I made the point that variables should be strongly typed.
But, I also highlighted how important ‘polymorphism’ was. While I was creating some tests in the EK9 language I found that it would be useful to mix a range of instances of typed values in ‘Lists’ and then via the use of a ‘dispatcher’ process each of those values (using their correct type).
However, with the current design of the language this was not possible, so I added the ‘Any’ type. Obviously I then had to make every construct type ‘extend’ the ‘Any’ type (this includes functions).
It took a reasonable amount of effort, but now it is possible to do this:
#!ek9
defines module example.dispatch.any
defines text for "en"
Text
welcome()
"Welcome"
defines type
CardSuit
Hearts
Diamonds
Clubs
Spades
EmailAddress as String constrain as
matches /^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$/
defines record
R1
property <- "Steve"
default operator ?
defines function
SteveSupplier()
<- rtn <- "Steve"
StephenSupplier()
<- rtn <- "Stephen"
defines class
DispatcherExample
process() as dispatcher
-> value as Any
<- rtn as String: "Unknown type"
private process()
-> value as Integer
<- rtn as String: `Integer value of ${value}`
private process()
-> value as Float
<- rtn as String: `Floating point value of ${value}`
private process()
-> value as Text
<- rtn as String: `Text value of ${value.welcome()}`
private process()
-> value as CardSuit
<- rtn as String: `CardSuit value of ${value}`
private process()
-> value as EmailAddress
<- rtn as String: `EmailAddress value of ${value}`
private process()
-> value as R1
<- rtn as String: `R1 property value of ${value.property}`
private process()
-> value as Supplier of String
<- rtn as String: `Function value of ${value()}`
defines function
showExampleOfAnyAndDispatcher()
//The compiler works out the 'Any' is the best 'type'
list <- [
1,
3.142,
Text("en"),
CardSuit.Hearts,
EmailAddress("stephenjohnlimb@gmail.com"),
R1(),
SteveSupplier,
StephenSupplier
]
//Now use a dispatcher.
processor <- DispatcherExample()
for listItem in list
value <- processor.process(listItem)
assert value?
//EOF
This use of ‘Any’ in conjunction with the ‘dispatcher’ approach removes the need for any type casting, type checking or assumptions. Clearly if you were just dealing with ‘classes’ or ‘traits’ then a common method name/signature across all the varied types might remove the need for the dispatcher.
Also, note that functions that have specific ‘signatures’ are automatically given the appropriate ‘super function’. Here you can see that function ‘SteveSupplier’ and ‘StephenSupplier’ have been given a ‘super function’ of ‘Supplier of String’ (that has a ‘super function’ of ‘Any’).
Optional Type
While the Optional type was already defined in EK9, I decided to take a more ‘RUST’ approach to the access of the value (optionally held). So, I added some compiler checks specifically for the EK9 Optional type. The short example below highlights this:
#!ek9
defines module error.on.optional.access
defines function
testSimpleValidIfCheck1()
o <- Optional("Steve")
if o?
value <- o.get()
assert value?
testUsingTernary() as pure
o <- Optional("Steve")
value <- o? <- o.get() else String()
assert value?
<?-
Demonstrates how direct access to 'get()' will be detected
if a call to 'o?' is not made first.
-?>
testInvalidGetAccess1()
o <- Optional("Steve")
//You will get a compiler error here, because no check has been done
value <- o.get()
assert value?
//Safer access without the need for o? call first.
assuredValue <- o.getOrDefault("Stephen")
assert assuredValue?
//EOF
The function ‘testInvalidGetAccess1’ highlights that the compiler will emit an error if no check has been made before getting the optional value held. The safer way is to use one of the many other methods on the Optional type (including ‘getOrDefault’).
Result Type
While I was reworking the ‘Optional’ type I decided to introduce the ‘Result’ type (as I could see that when used in RUST it had some merit). However, to be honest I did not like the way RUST employed the ‘Result’ type. So I’ve had a go at trying to make something slightly easier to use (well easier for me anyway).
The ‘Result’ type is a generic class much like ‘Optional’ but it has two type parameters; the ‘Ok’ type and the ‘Error’ type. These two types cannot be the same, they must be different (in EK9).
The key methods on the ‘Result’ are ‘?’ (is-set), ‘isOk()’, ‘isError()’ then ‘ok()’ and ‘error()’ to access the appropriate values.
Just like ‘Optional’ there are checks built into the compiler for ‘Result’ that check access is safe as shown below.
#!ek9
defines module result.access
defines function
testEmptyResult()
r <- Result() of (String, Integer)
assert not r.isOk() and not r.isError()
testOkOnlyResult()
r <- (Result() of (String, Integer)).asOk("Steve")
assert r.isOk() and not r.isError()
testErrorOnlyResult()
r <- (Result() of (String, Integer)).asError(-1)
assert not r.isOk() and r.isError()
testSimpleResultUse()
r <- Result("Steve", Integer())
assert r.isOk() and r.isError()
okValue <- r.isOk() <- r.ok() else String()
assert okValue?
//You can also do this
errorValue <- r.isError() <- r.error() else Integer()
assert not errorValue?
assuredValue1 <- r.okOrDefault("Stephen")
assert assuredValue1?
assuredValue2 <- r.getOrDefault("Stephen")
assert assuredValue2?
testCompilerErrorWhenAccessBeforeCheckingOk()
r <- Result(String(), Integer())
//Compiler will emit error here, because no check has been made
okValue <- r.ok()
assert okValue?
//Compiler will emit error here, because no check has been made
asLower <- r.ok().lowerCase()
assert asLower?
//Compiler will emit error here, because no check has been made
errorValue <- r.error()
assert errorValue?
//EOF
The short example above shows some of the more simple methods on the ‘Result’ type, there are lots of other methods available for a more ‘functional’ approach; such as ‘whenOk()’ and ‘whenError()’.
Summary
All in all, I’m quite happy with the range of built-in types defined in EK9 now. In the next post I’ll cover each of the phases of the EK9 compiler and how far I’ve come and how much further there is to go (a long way!).
Subscribe to my newsletter
Read articles from Steve directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
