Understanding and Resolving the "ECONNREFUSED ::1" Error: A Beginner's Guide to IPv4, IPv6, and Port Binding

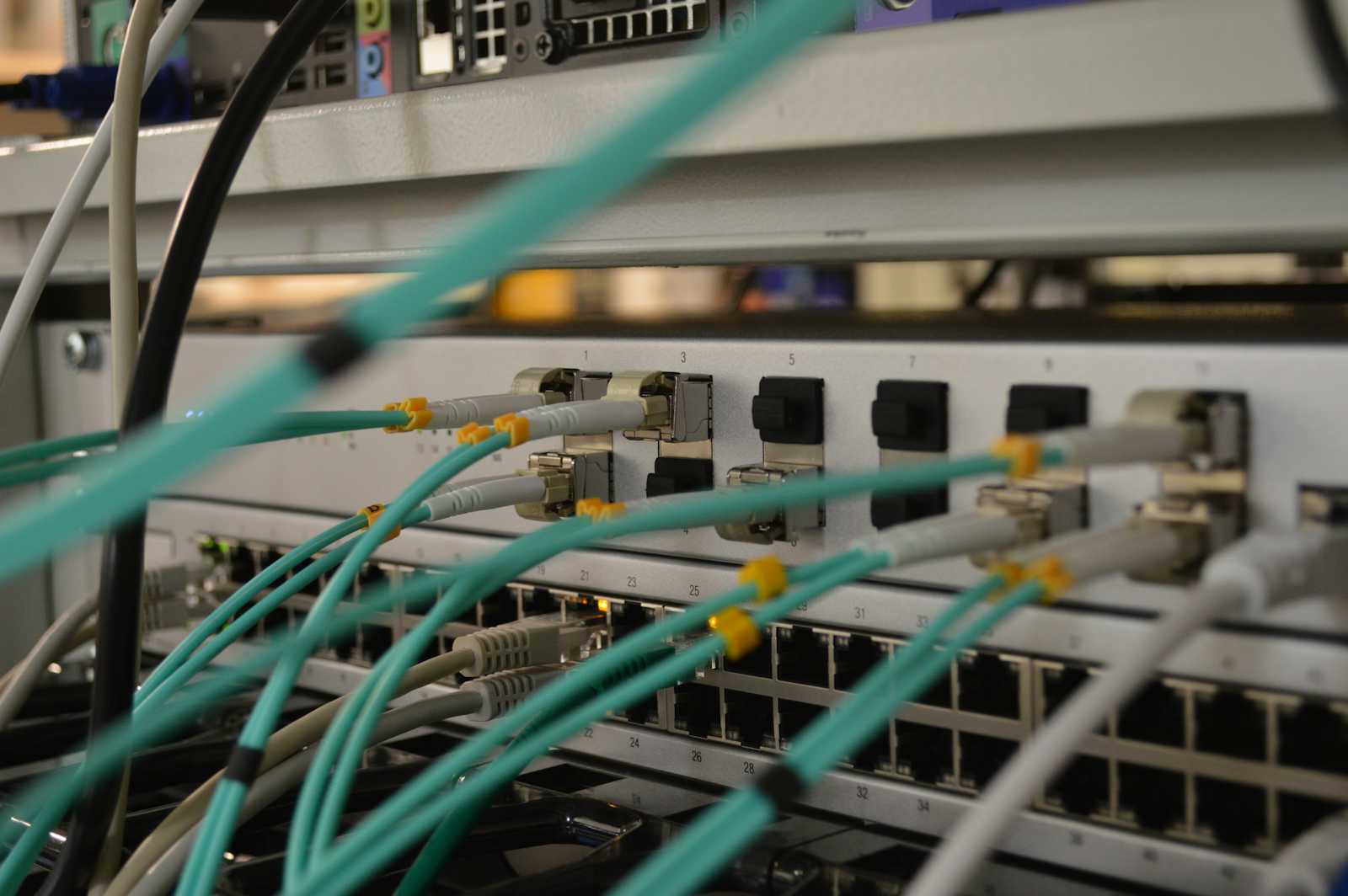
If you're a beginner working with local servers and APIs, you might have encountered the frustrating ECONNREFUSED ::1
error. This error often occurs when your application tries to connect to a server but fails because of a mismatch between IPv4 and IPv6 configurations. In this blog, we'll break down the problem, explain the concepts of IPv4, IPv6, and port binding, and provide step-by-step solutions to resolve the issue.
What is the "ECONNREFUSED ::1" Error?
The ECONNREFUSED ::1
error means that your application tried to connect to the IPv6 loopback address (::1
) but was refused. This typically happens when:
Your server is only listening on the IPv4 loopback address (
127.0.0.1
).Your application resolves
localhost
to the IPv6 address (::1
) instead of the IPv4 address (127.0.0.1
).
This mismatch prevents your application from connecting to the server, resulting in the error.
Understanding IPv4, IPv6, and Port Binding
1. What is IPv4?
IPv4 (Internet Protocol version 4) is the fourth version of the Internet Protocol.
It uses a 32-bit address format, e.g.,
127.0.0.1
.The loopback address for IPv4 is
127.0.0.1
, which refers to the local machine.
2. What is IPv6?
IPv6 (Internet Protocol version 6) is the sixth version of the Internet Protocol.
It uses a 128-bit address format, e.g.,
::1
.The loopback address for IPv6 is
::1
, which also refers to the local machine.
3. What is Port Binding?
- A server listens for incoming connections on a specific IP address and port.
For example, a server might bind to 127.0.0.1:11434
, meaning it only accepts connections from the IPv4 loopback address on port 11434
.
Why Does the Error Occur?
The error occurs because:
Your server is bound to
127.0.0.1
(IPv4).Your application resolves
localhost
to::1
(IPv6).
The server refuses the connection because it isn't listening on ::1
.
How to Diagnose and Resolve the Issue
Step 1: Check if the Server is Running
Use the netstat
command to check if the server is running and listening on the correct address and port.
On Linux/macOS:
bash
Copy
netstat -an | grep 11434
On Windows:
bash
Copy
netstat -an | findstr 11434
Expected Output:
Copy
TCP 127.0.0.1:11434 0.0.0.0:0 LISTENING
If the server is listening on 127.0.0.1:11434
, it means it's bound to the IPv4 loopback address.
Step 2: Test the Server with curl
Use curl
to test the server's accessibility with both localhost
and 127.0.0.1
.
Test with localhost
:
bash
Copy
curl http://localhost:11434/api/generate
Test with 127.0.0.1
:
bash
Copy
curl http://127.0.0.1:11434/api/generate
If the first command fails but the second one works, it confirms that localhost
is resolving to ::1
(IPv6) instead of 127.0.0.1
(IPv4).
Step 3: Update Your Application
To fix the issue, update your application to explicitly use the IPv4 address (127.0.0.1
) instead of localhost
.
Example in JavaScript (Node.js):
javascript
Copy
const axios = require('axios');
async function main() {
const url = 'http://127.0.0.1:11434/api/generate'; // Use IPv4 address
const payload = {
model: 'llama2-uncensored',
prompt: 'What is water made of?',
stream: false // Use a boolean, not a string
};
try {
const response = await axios.post(url, payload, {
headers: {
'Content-Type': 'application/json'
}
});
console.log('Response from API:', response.data);
} catch (error) {
console.error('Error making request:', error.message);
console.error('Error details:', error.response?.data || error.stack);
}
}
main();
Conclusion
The ECONNREFUSED ::1
error is a common issue caused by a mismatch between IPv4 and IPv6 configurations. By understanding the difference between IPv4 and IPv6, checking server binding, and explicitly using 127.0.0.1
in your application, you can easily resolve this issue. Additionally, tools like netstat
and curl
are invaluable for diagnosing and troubleshooting such problems.
By following the steps outlined in this blog, you'll be well-equipped to handle similar issues in the future. Happy coding! ๐
Subscribe to my newsletter
Read articles from rohith reddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
