Mastering JavaScript Promises: A Comprehensive Guide with Real-World Examples
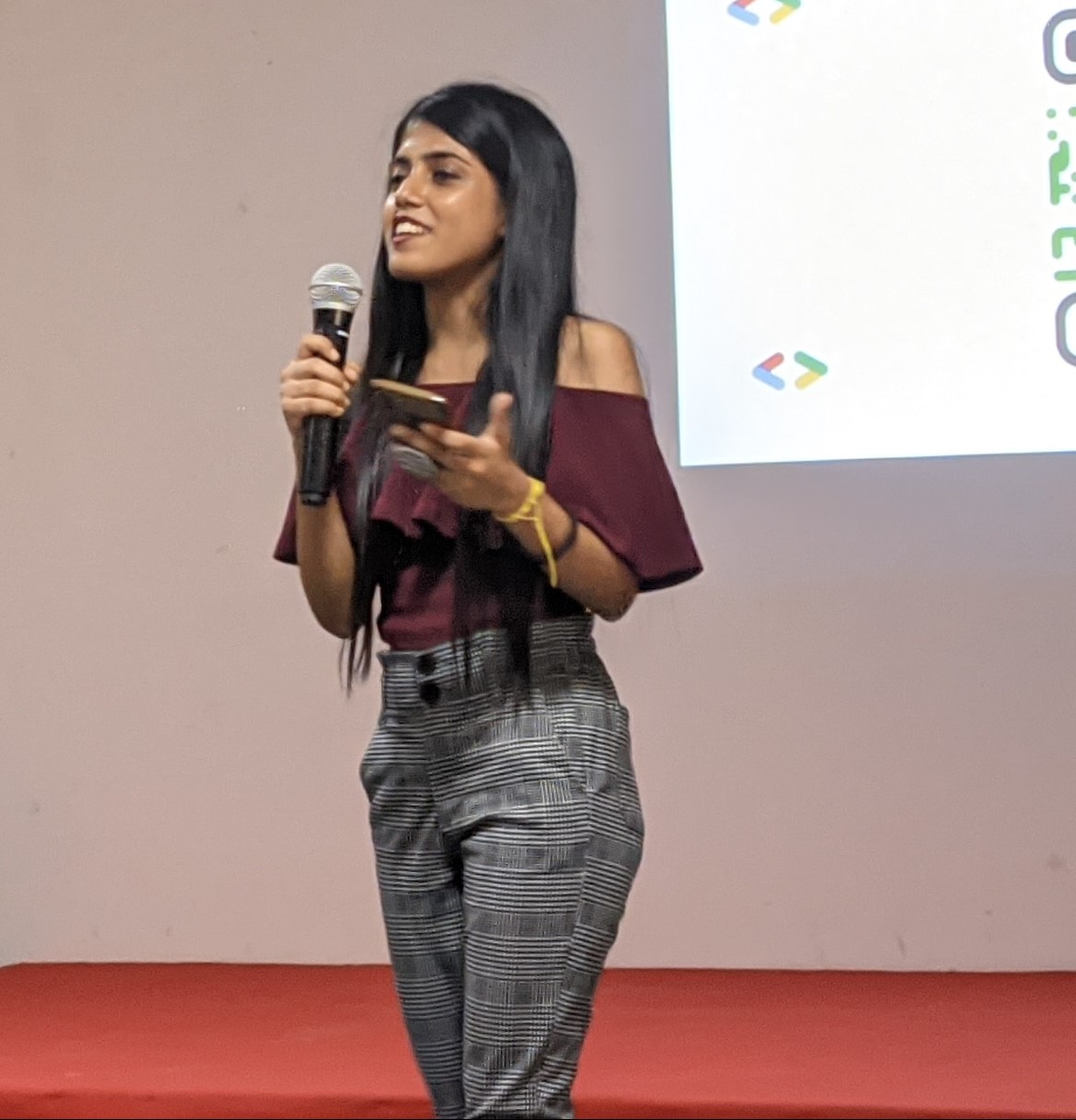

JavaScript Promises are a powerful tool for handling asynchronous operations. They act as a container for a future value, representing the eventual completion (or failure) of an async task. Whether you're fetching data from an API, processing payments, or updating a user's wallet, Promises make your code cleaner and more manageable.
In this blog, we’ll dive deep into JavaScript Promises, explore their use cases, and provide practical examples to help you master them.
What is a Promise?
A Promise is an object that represents the eventual completion (or failure) of an asynchronous operation. It has three states:
Pending: The initial state, neither fulfilled nor rejected.
Fulfilled: The operation completed successfully.
Rejected: The operation failed.
Here’s a simple example:
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Operation successful!");
}, 2000);
});
promise.then((result) => {
console.log(result); // Output: "Operation successful!"
}).catch((error) => {
console.log(error);
});
Promise Chaining
One of the most powerful features of Promises is chaining. This allows you to execute a sequence of asynchronous operations in a clean and readable way.
Example: E-commerce Checkout Flow
Imagine you’re building an e-commerce platform. The checkout process involves:
Creating an order.
Proceeding to payment.
Showing the order summary.
Updating the user’s wallet.
Here’s how you can handle this using Promise chaining:
const cart = ["shoes", "pants"];
createOrder(cart)
.then((orderId) => {
console.log("Order created:", orderId);
return proceedToPayment(orderId);
})
.then((paymentInfo) => {
console.log("Payment successful:", paymentInfo);
return showOrderSummary(paymentInfo);
})
.then((balance) => {
console.log("Order summary shown. Updating wallet...");
return updateWallet(balance);
})
.then((resBalance) => {
console.log("Final wallet balance:", resBalance.balance);
})
.catch((err) => {
console.log("Error:", err.message);
});
Error Handling in Promises
Errors in Promises are handled using the .catch()
method. If any Promise in the chain is rejected, the control jumps directly to the nearest .catch()
block.
Example:
createOrder([]) // Empty cart will trigger an error
.then((orderId) => {
console.log("Order created:", orderId);
return proceedToPayment(orderId);
})
.catch((err) => {
console.log("Error:", err.message); // Output: "Cart is empty!"
});
Advanced Promise Methods
JavaScript provides several utility methods to handle multiple Promises efficiently:
1. Promise.all
Waits for all Promises to resolve. If any Promise is rejected, it immediately throws an error.
Promise.all([createOrder(cart), proceedToPayment("order123")])
.then((results) => {
console.log("All operations completed:", results);
})
.catch((err) => {
console.log("Error:", err.message);
});
2. Promise.allSettled
Waits for all Promises to settle (either resolve or reject), regardless of errors.
Promise.allSettled([createOrder(cart), proceedToPayment("order123")])
.then((results) => {
console.log("All operations settled:", results);
});
3. Promise.race
Returns the result of the fastest Promise (either resolved or rejected).
Promise.race([createOrder(cart), proceedToPayment("order123")])
.then((result) => {
console.log("Fastest operation completed:", result);
});
4. Promise.any
Returns the result of the first resolved Promise. If all Promises are rejected, it throws an aggregated error.
Promise.any([createOrder(cart), proceedToPayment("order123")])
.then((result) => {
console.log("First successful operation:", result);
})
.catch((err) => {
console.log("All operations failed:", err.errors);
});
Real-World Use Cases
API Calls: Fetching data from a server.
File Operations: Reading or writing files asynchronously.
Database Queries: Executing queries and handling results.
Payment Processing: Handling payment gateways and updating user wallets.
Best Practices
Always handle errors using
.catch()
.Use
Promise.all
for parallel execution of independent tasks.Avoid nesting Promises; use chaining instead.
Use
async/await
for cleaner syntax (a topic for another blog!).
Conclusion
JavaScript Promises are essential for managing asynchronous operations effectively. By understanding their states, chaining, and advanced methods, you can write cleaner, more efficient code. Whether you're building an e-commerce platform or handling chat API calls, Promises will make your life easier.
Start implementing Promises in your projects today, and watch your code become more readable and maintainable!
By following this guide, you’ll not only master Promises but also write code that’s efficient and easy to debug. Happy coding!
Subscribe to my newsletter
Read articles from Sayantani Deb directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
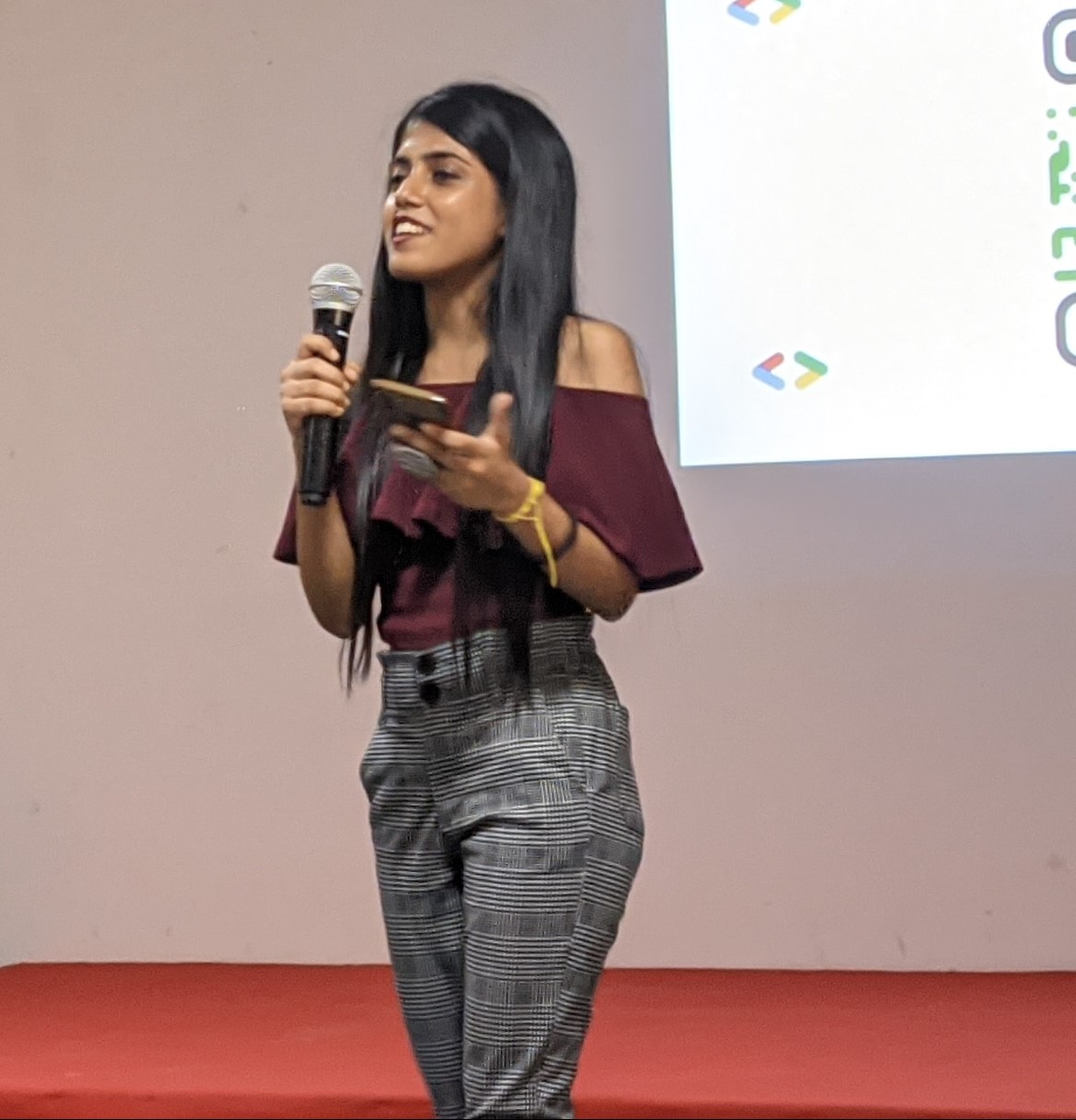
Sayantani Deb
Sayantani Deb
A Javascript Porgrammer who loves to build cool projects in the weekends!