Exploring Web Workers in JavaScript

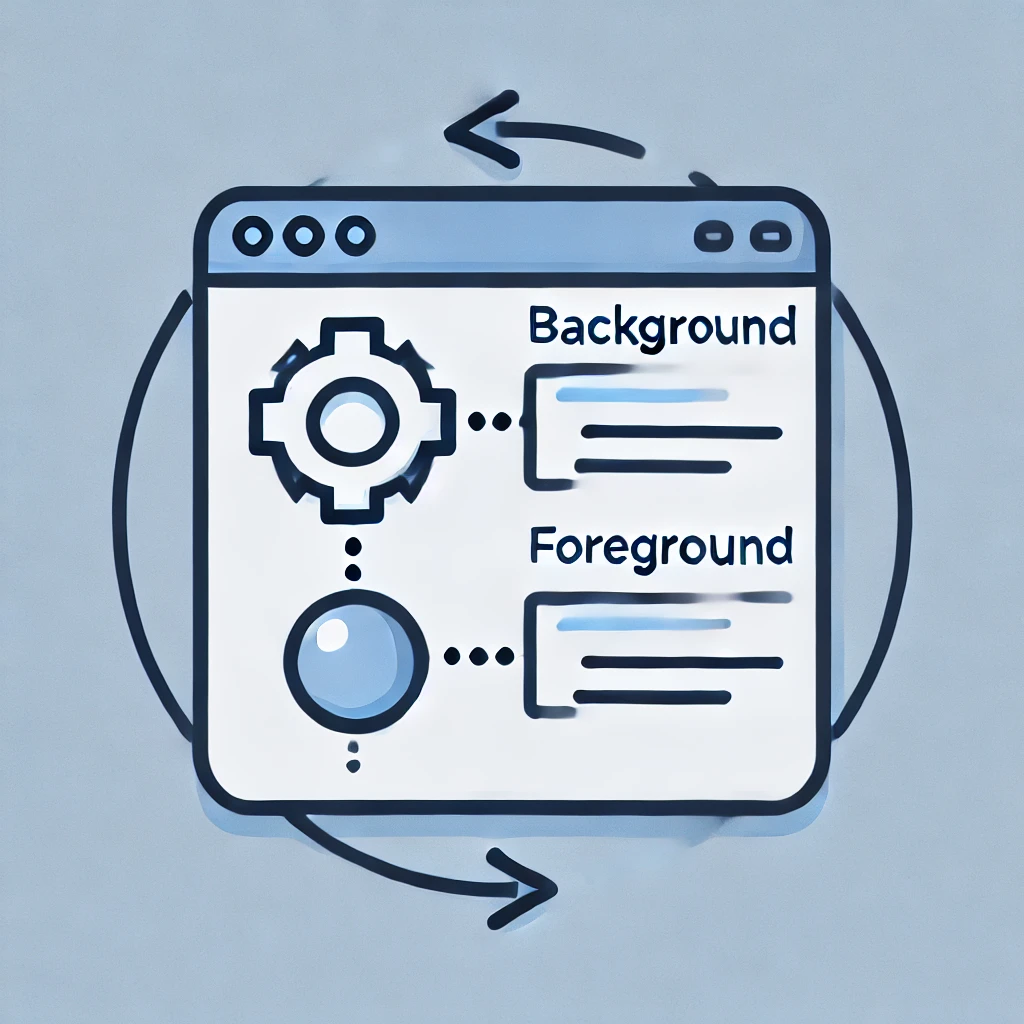
Modern web applications often require handling complex tasks without compromising the user experience. This is where the Web Worker API comes into play. Web Workers allow developers to run scripts in a background thread, ensuring the main thread remains responsive.
What Are Web Workers?
Web Workers are a JavaScript feature designed to execute code in a separate thread, independent of the main execution thread. By leveraging Web Workers, you can offload heavy computations or long-running tasks, avoiding UI freezes.
Core Features:
- Parallel Execution: Web Workers enable concurrent processing by utilizing separate threads.
- Thread Isolation: They do not have direct access to the DOM, minimizing the risk of UI disruptions.
- Message-Based Communication: The main thread and workers exchange data using the
postMessage
method and message events.
Ideal Use Cases for Web Workers
Consider using Web Workers for:
- Data-Intensive Computations: Performing CPU-heavy tasks like scientific calculations.
- Large-Scale Data Parsing: Handling JSON or XML data.
- Real-Time Processing: Working with streaming data, like audio or video.
- Background Operations: Running encryption, image processing, or file compression tasks.
Implementing a Web Worker
Simple Example
To use a Web Worker, follow these steps:
Create a Worker Script:
// myWorker.js self.onmessage = function(event) { const input = event.data; const squared = input * input; // Example: square the input self.postMessage(squared); };
Initialize and Communicate with the Worker:
// main.js
const worker = new Worker('myWorker.js');
worker.onmessage = function(event) {
console.log('Result from worker:', event.data);
};
worker.postMessage(5); // Send data to the worker
How It Works
- The
Worker
constructor loads the worker script. - The
onmessage
handler listens for messages from the worker. - The
postMessage
method sends data to the worker.
Constraints of Web Workers
While Web Workers are a valuable asset, they have some limitations:
- No DOM Access: Workers cannot directly interact with the DOM. Use messages to relay results to the main thread.
- Same-Origin Policy: Workers can only load scripts from the same domain unless CORS is enabled.
- Resource Overhead: Creating workers and passing messages can introduce a small performance cost.
- Browser Support: Although widely supported, always verify compatibility for your users' browsers.
Advanced Techniques
Using Web Workers with Modules
You can specify ES module scripts when creating a worker:
const worker = new Worker('myWorker.js', { type: 'module' });
Shared Workers
Shared Workers allow multiple scripts to communicate with the same worker instance:
const sharedWorker = new SharedWorker('sharedWorker.js');
sharedWorker.port.onmessage = function(event) {
console.log('Message from shared worker:', event.data);
};
sharedWorker.port.postMessage('Hello from the main script');
Termination
When a worker is no longer needed, terminate it to conserve resources:
worker.terminate();
Summary
Web Workers are an essential feature for improving the performance and responsiveness of modern web applications. By delegating heavy tasks to background threads, they help maintain a seamless user experience. Although they have certain constraints, their ability to handle intensive tasks without blocking the UI makes them a powerful tool for developers.
Explore the possibilities of Web Workers to unlock higher performance in your web projects!
Subscribe to my newsletter
Read articles from The Syntax Node directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

The Syntax Node
The Syntax Node
I am a JavaScript Full Stack Developer with expertise in front-end frameworks like React and Angular, and back-end technologies such as Node.js and Express.