Embed Yotpo Loyalty Widgets in Headless Stores

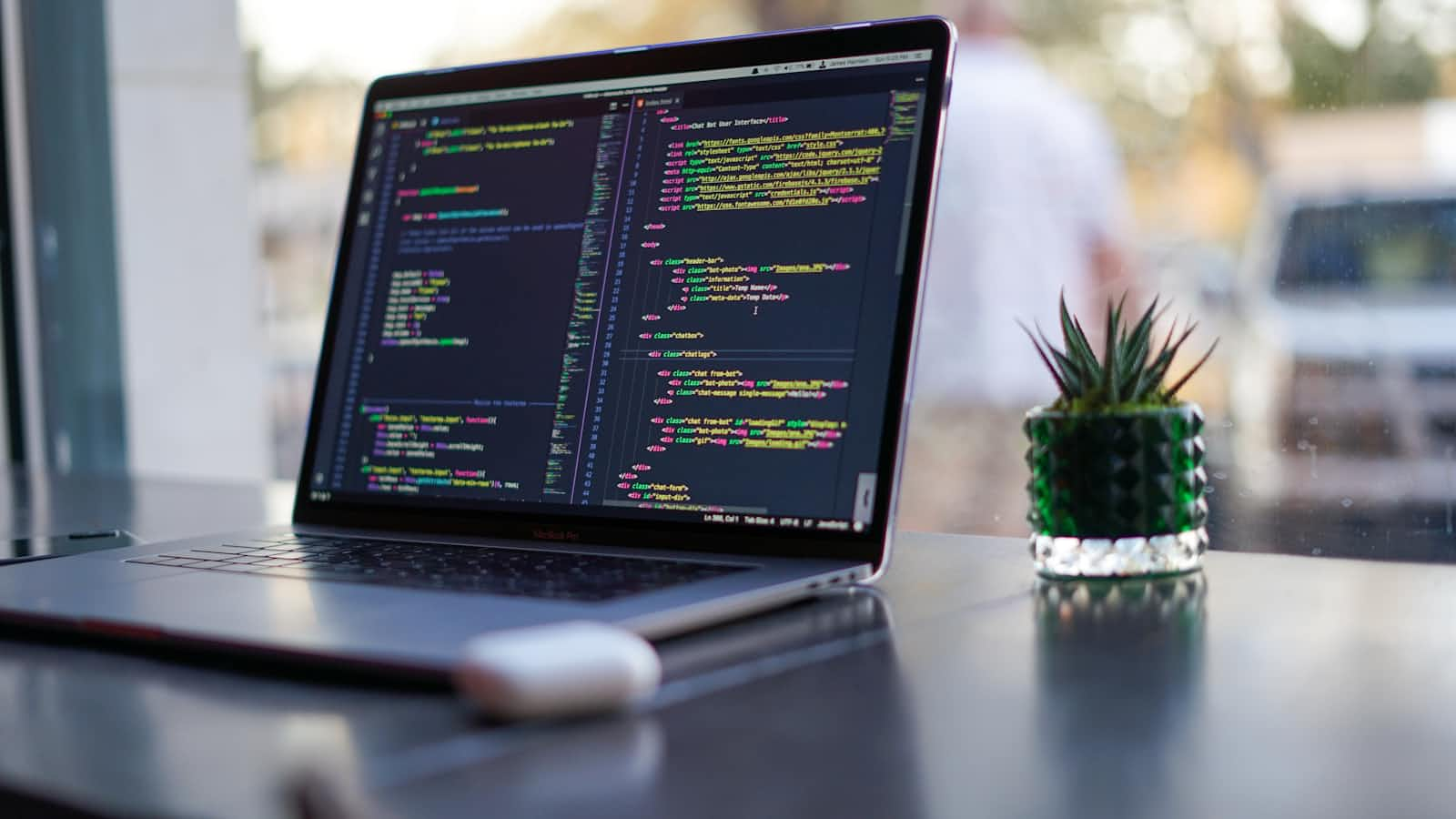
Currently, I am working on turning a Liquid-based Shopify store into a headless Hydrogen-based store. Part of this was transferring Yotpo embedded forms to the new headless site. As for the loyalty and referrals portion of Yotpo, you can embed these forms directly into your site without the need of countless API requests and manual styling.
Here are some official Yotpo documents on similar topics. I used these to figure this out myself with some extra digging and tinkering. However, I feel as if these are leaving out key details and don’t directly address many of the headless platforms.
Now, Let’s get on with the tutorial
Enable yotpo in CSP
CSP stands for content security policy. This allows you to import scripts and media from URLs from outside of your site. If you are using Hydrogen, your CSP is located in entry.server.jsx
. If you are using another React application, you will likely not have a CSP unless you have configured one manually. You can go to the next step if so.
Here are the CSP links you need to allow to use Yotpo in your Hydrogen app.
const {nonce, header, NonceProvider} = createContentSecurityPolicy({
connectSrc: [
'https://cdn-widgetsrepository.yotpo.com',
'https://loyalty.yotpo.com',
],
scriptSrc: [
'https://cdn-widgetsrepository.yotpo.com', // Loader script
'https://loyalty.yotpo.com', // Potential dynamic script loading
],
styleSrc: [
'https://cdn-widgetsrepository.yotpo.com',
'https://loyalty.yotpo.com',
],
imgSrc: [
'https://cdn-widgetsrepository.yotpo.com', // Yotpo-hosted images
'https://loyalty.yotpo.com',
],
mediaSrc: [
'https://cdn-widgetsrepository.yotpo.com',
],
defaultSrc: [
'https://cdn-widgetsrepository.yotpo.com',
'https://loyalty.yotpo.com',
],
});
This could be overkill haha, but I added it in most sources just in case. You likely will only need it in default and script sources.
Copy script tag to root.jsx
Copy this script tag into your root component. For Hydrogen, your root component is in the file root.jsx
.
<script src="https://cdn-widgetsrepository.yotpo.com/v1/loader/<YOUR_YOTPO_GUID>" async></script>
Copy client script to client component
Go into your Yotpo Loyalty & Referrals dashboard. Select Display On-Site. Find the widget you want to embed, and click the 3 dots. Then select Embed Code to show the embed code to display your client side widget.
Paste this client div into your desired client component.
<div className="yotpo-widget-instance" data-yotpo-instance-id="<ISTANCE_ID>"></div>
Note: This will not work yet, since we still have to authenticate the client side. This is covered in the next 2 steps.
Pull yotpo customer info via server-side
In a server side component, pull the current customer’s Yotpo information from the Yotpo API. I do this by email, since autheticated customers will have their emails stored in the session object.
See the Yotpo Loyalty API documentation here: https://loyaltyapi.yotpo.com/reference/reference-getting-started
const fetchData = async (url, headers) => {
try {
const response = await fetch(url, { method: 'GET', headers: new Headers(headers) });
if (!response.ok) throw new Error('Network response was not ok');
return await response.json();
} catch (error) {
console.error('Error fetching data:', error);
return null;
}
};
const customerEmail = '<customer_email_here>';
const apiKey = context.env.YOTPO_API_KEY;
const guid = context.env.YOTPO_GUID;
const customerDetails = await fetchData(
`https://loyalty.yotpo.com/api/v2/customers?customer_email=${customerEmail}&with_history=true`,
{
'x-guid': guid,
'x-api-key': apiKey,
}
);
Create Customer Signature
For authentication over a headless source, Yotpo requires a customer signature. This signature is hex representation of a sha256 hashed concatenation of the customer email and Yotpo API Key:
<customer_email><apiKey>
Make sure you implement this in your server side logic, to make sure that your customer information and API keys are not exposed anywhere to the end user. You can (and must for the next step) pass this signature safely to the client.
If you are using Hydrogen, you can make your Yotpo API calls and customer signature in your loader function. Then pull these values client side as needed.
function generateYotpoSignature(customerEmail, apiKey) {
const payload = `${customerEmail}${apiKey}`;
const hash = crypto.createHash('sha256');
hash.update(payload);
return hash.digest('hex');
}
const signature = generateYotpoSignature(
customerDetails.email,
apiKey
);
Yotpo Client Authentication div
This div utilizes the signature that is created above, and connects the correct customer to the widget.
Say you are implementing a custom rewards page. If your customer is authenticated on your store, they will also be authenticated on this widget. So they will see their amount of points, ability to redeem them, etc.
Make sure this is included on the client side of any component that uses an embedded yotpo widget.
<div
id="swell-customer-identification"
data-authenticated="true"
data-email={customerDetails.email}
data-id={customerDetails.third_party_id}
data-token={signature}
style={{ display: "none" }}
></div>
Initialize Yotpo Widgets in browser console
For some reason, this did not work for me until I added this step. My yotpo widgets would not show until I ran yotpoWidgetsContainer.initWidgets();
after the DOM was fully loaded in the console. I used a useEffect hook to call this method once the DOM was loaded.
Put this hook in the same client side component as you put the client widget div.
useEffect(() => {
const initializeWidgets = () => {
if (
window.yotpoWidgetsContainer &&
typeof window.yotpoWidgetsContainer.initWidgets === "function"
) {
yotpoWidgetsContainer.initWidgets();
console.log("Yotpo widgets initialized!");
}
};
if (document.readyState === "complete") initializeWidgets()
else {
const handleLoad = () => initializeWidgets();
window.addEventListener("load", handleLoad);
return () => window.removeEventListener("load", handleLoad);
}
}, []);
Conclusion
This should be it! If not, carefully go back and look over all of your code and read the text I have included. If you are still having issues, you can reach out to me or view the Yotpo documentation. Yotpo also has a very good support chat, but keep in mind that some of the Yotpo support team is not aware of issues surrounding headless stores and integration.
Subscribe to my newsletter
Read articles from David Williford directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

David Williford
David Williford
I am a developer from North Carolina. I have always been fascinated by the internet, and scince high school I have been trying to understand the magic behind it all. ECU Computer Science Graduate