C++ Event System

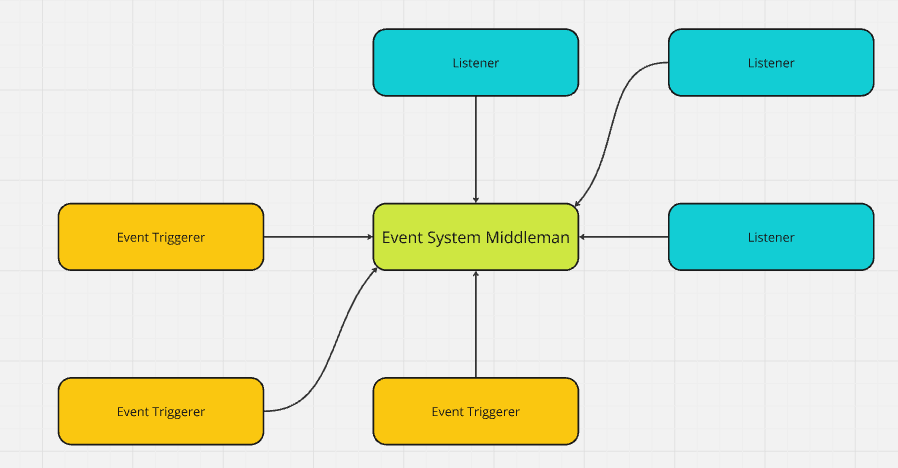
This article is a breakdown of how I have implemented an event system within my custom C++ game engine. This system is inspired by the one described in ‘Games Programming Patterns’, but with some key differences - one of these being that it is not based around inheritance.
High-level Overview
The aim for this system is for anywhere in the code base to be able to send a global message (event) to anywhere else in the engine, without the two places having to know about each other. To achieve this the system uses a static middle-man class which both sides of the communication know about. This way it makes a flow like this:
Which, as a result, if a single listener or event trigger is removed from the system then nothing will break, and no dependencies will be lost.
System Flow
The premise behind the system is that there are ‘events‘, which have callbacks associated with them, and can be triggered from anywhere. The diagram below shows a basic example of the flow:
The event in question here is ‘OnScreenSizeChanged‘. It has one callback associated with it, which is a function called ‘WindowSizeChanged‘, and is from the ‘Window‘ class.
So, how this flow starts is that whenever the screen is resized, then the code that detects this fires the event of ‘OnScreenSizeChanged‘. What this does is run through the list of callbacks associated and calls them one by one. Presumably resulting in the ‘WindowSizeChanged()‘ function handling the resizing of the screen. Then the code returns back to wherever triggered the event.
Key Drawback
How this looks in code is like this:
// ------------- In initial game setup ------------- //
CREATE_EVENT_NAME_INSTANCE("OnScreenSizeChanged");
// ------------- In window class setup ------------- //
REGISTER_EVENT_CALLBACK("OnScreenSizeChanged", Window::WindowSizeChanged);
// ------------- In function which detects if the screen size has changed ------------- //
RAISE_EVENT("OnScreenSizeChanged");
Nice and simple, right!
In-Practice
In reality there is more going on than shown above. Not from an end user perspective, but from a behind the scenes view.
Firstly, the functions called in the previous snippet are actually macros. These macros are just wrappers around calls into the static middleman class, making the whole flow nicer and more logical to use. Here are the macros in order:
#define CREATE_EVENT_NAME_INSTANCE(EventFunctionName)\
{\
Engine::EventSystem::EventHandler::RegisterEventName(EventFunctionName); \
}
#define REGISTER_EVENT_CALLBACK(EventFunctionNameHashed, functionToBeCalled)\
{\
std::function<void()> lambdaForCalling = [this](){functionToBeCalled(); }; \
Engine::EventSystem::EventHandler::AddCallbackToEvent(EventFunctionNameHashed, lambdaForCalling, (void*)this);\
}
#define RAISE_EVENT(EventFunctionName)\
{\
Engine::EventSystem::EventHandler::RaiseEvent(EventFunctionName);\
}
Still fairly simple so far. However, key eyed readers will have noticed that the macros do not take in a string, they want to be passed a hashed version of the name. This is to speed up the process overall, as comparing strings is very slow, and comparing integers is much much faster. So, the reality of the macro usage looks more like this, than the one shown before:
// ------------- Static hashing of event name done on startup ------------- //
// In reality there is a large file storing all of these called: 'HashedEventNames.h'
static const unsigned int kOnScreenSizeChanged = StringHash::Hash("OnScreenSizeChanged");
// ------------- In initial game setup ------------- //
CREATE_EVENT_NAME_INSTANCE(kOnScreenSizeChanged);
// ------------- In window class setup ------------- //
REGISTER_EVENT_CALLBACK(kOnScreenSizeChanged, Window::WindowSizeChanged);
// ------------- In function which detects if the screen size has changed ------------- //
RAISE_EVENT(kOnScreenSizeChanged);
StringHash::Hash is a function that I have defined. It doesn’t overly matter what hash function you use so long as it gives different results for each unique input it receives.
The Vital Backend
But how does the function actually get called when the event is fired? This is the important question here. First of all, there are actually three different types of callbacks that can be associated. Ones which take no data as input, ones which pass a string through, and a final version which takes in arbitrary data (void*). The reason for this is that some events need more context than simply receiving an event name. For example, if two colliders collide, it may be beneficial to pass through data about the colliders into where is going to be handling the event.
With this in-mind, this is what the back-end looks like:
typedef std::unordered_map<unsigned int, std::vector<std::pair<std::function<void()>, void*>>> EventMapType; // No data is passed in
typedef std::unordered_map<unsigned int, std::vector<std::pair<std::function<void(std::string)>, void*>>> EventMapType_Data_String; // String data is passed in
typedef std::unordered_map<unsigned int, std::vector<std::pair<std::function<void(void*)>, void*>>> EventMapType_Data; // Arbitrary data is passed in (void*)
static EventMapType_Data_String mEventsRegistered_data_string;
static EventMapType_Data mEventsRegistered_data;
static EventMapType mEventsRegistered;
Nice and complex :D
To break down this data type:
// Map of hashed event name, to list of functions with their calling object
std::unordered_map<unsigned int, std::vector<std::pair<std::function<void()>, void*>>>
It shows that for each hashed event name there is a list of associated function callbacks. The object associated with them is there so that the callbacks can be un-registered at a later data:
#define REMOVE_EVENT_CALLBACK_REGISTERED(EventFunctionNameHashed)\
{\
Engine::EventSystem::EventHandler::RemoveCallbackRegistered(EventFunctionNameHashed, (void*)this);\
}
REMOVE_EVENT_CALLBACK_REGISTERED(kOnScreenSizeChanged);
Whenever an event is raised, all callbacks associated with it are called. The only downside to doing it this way is that the function being called into is being run on the thread of the place that triggered the event, which is not necessarily the thread which setup the class which is being called into. Normally this is fine, but it is something to keep in mind while writing callbacks.
Setting Up Events From File
After using the system for a while it started getting very tedious to have to manually add the line for registering a new event every time a new one was needed, and forgetting to do so became annoying. So, to streamline this flow I added automated loading of event names from file:
{
"Events": [
"OnGamePaused",
"OnGameUnPaused",
"OnCreditsOpened",
"OnCreditsClosed",
"OnPlayerDeath",
"OnPlayerRespawn",
"OnPlayerLevelUp",
"OnCutsceneSkip",
"OnSplashScreenEnded",
"OnProjectCubeLogoFinish",
"OnMainMenuLoaded",
"OnNewColliderLoaded",
"OnScreenSizeChanged",
"OnAchievementCompleted",
"OnEnemySpawnTrigger",
"OnAudioOverlayToggled",
"OnEventSystemOverlayToggled",
"OnPlayModeToggle",
"OnSceneAdded",
"OnSceneRemoved"
]
}
So instead of manually registering new event names, all I need to add the name to a file and it will be pulled through automatically. The one drawback of this is that the hashed versions of the event names still need to be manually added, but that is not much of an issue as it is really quick to do. And it is required to be done for you to actually be able to trigger the event, so you cannot forget to do it and still use the flow.
Conclusion
To conclude, this article has covered how I have implemented a low dependency C++ event system into my custom game engine. And it has covered the back-end principles that power its functionality.
For next week’s article I am going to be covering frustum culling.
Thanks for reading :D
Subscribe to my newsletter
Read articles from Platwo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Platwo
Platwo
Professional games programmer from England, who has a first class degree, and has worked in the industry at both AAA and smaller indie levels. And who's next endeavour is to make my own custom game engine and game, which my blogs will be covering in detail.