Day 7 Programming (Error-Handling try-catch). (Threads) (Multithreads).
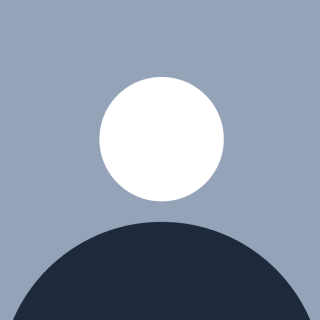

Error Handling in Java:
Error handling in Java helps you manage problems that can occur while your program runs (e.g., file not found, invalid input, divide by zero). Java uses exceptions to represent and handle these errors.
What are Exceptions?
An exception is an event that occurs during the execution of a program, disrupting its normal flow.
When an exception occurs, Java creates an exception object that contains information about the error and its location.
Types of Exceptions:
Checked Exceptions
Exceptions that must be handled at compile time.
Example:
IOException
,SQLException
.Handled using try-catch blocks or throws keyword.
Unchecked Exceptions (Runtime Exceptions)
Exceptions that occur at runtime and do not need to be declared or handled explicitly.
Example:
NullPointerException
,ArithmeticException
.
Errors
Serious problems that are generally beyond the control of the program.
Example:
OutOfMemoryError
,StackOverflowError
.
How to Handle Exceptions?
Java provides a structured way to handle exceptions using the try-catch-finally mechanism.
1. Try-Catch Block
Try Block: Code that might throw an exception is written here.
Catch Block: Code to handle the exception is written here.
2. Finally Block (Optional)
Code inside the
finally
block always runs, regardless of whether an exception occurred or not.Often used to release resources like closing files or databases.
public class Main {
public static void main(String[] args) {
try {
int result = 10 / 0;
} catch (ArithmeticException e) {
System.out.println("Error: Division by zero.");
} finally {
System.out.println("Execution complete.");
}
}
}
Best Practices for Error Handling:
Use Specific Exceptions:
Catch specific exceptions instead of using a generalException
type In the above use we used ArithmeticException eAvoid Overusing Exceptions:
Only use exceptions for exceptional cases, not for normal program logic.Always Clean Up Resources:
Usefinally
to close files, database connections, etc.
Threads and Multithreading in Java
In Java, multithreading allows a program to run multiple tasks concurrently, improving efficiency and responsiveness. A thread is the smallest unit of a program that can execute independently.
What is a Thread?
A thread is like a separate path of execution within a program.
By default, every Java program runs in a single thread called the main thread.
Multithreading enables a program to perform multiple operations at the same time (e.g., playing music while downloading a file).
Why Use? Threads
Creating Threads in Java
There are two main ways to create a thread in Java:
Extending the
Thread
classImplementing the
Runnable
interface
1. Extending the Thread
Class
When you extend the class, you override its method to define the code that the thread will execute.Threadrun()
class MyThread extends Thread {
@Override
public void run() {
// Code that runs in the thread
for (int i = 1; i <= 5; i++) {
System.out.println("Thread running: " + i);
}
}
}
public class Main {
public static void main(String[] args) {
MyThread thread = new MyThread(); // Create a thread
thread.start(); // Start the thread
System.out.println("Main thread is running...");
}
}
2. Implementing the Runnable
Interface
The interface is preferred when the class needs to extend another class (since Java supports single inheritance).Runnable
class MyRunnable implements Runnable {
@Override
public void run() {
for (int i = 1; i <= 5; i++) {
System.out.println("Runnable thread: " + i);
}
}
}
public class Main {
public static void main(String[] args) {
MyRunnable task = new MyRunnable(); // Create a task
Thread thread = new Thread(task); // Create a thread and pass the task
thread.start(); // Start the thread
System.out.println("Main thread is running...");
}
}
Key Methods in Thread Class
start()
: Starts the thread and calls therun()
method.run()
: Contains the code that executes in the thread.sleep(milliseconds)
: Pauses the thread for a specified time.join()
: Makes the current thread wait for another thread to complete.isAlive()
: Checks if a thread is still running.
Multithreading in Action
Java allows running multiple threads run independently of each other. If an exception occurs in one thread, it does not affect the execution of other threads.
class MyTask extends Thread {
private String name;
public MyTask(String name) {
this.name = name;
}
@Override
public void run() {
for (int i = 1; i <= 3; i++) {
System.out.println(name + " running: " + i);
try {
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Main {
public static void main(String[] args) {
MyTask thread1 = new MyTask("Thread-1");
MyTask thread2 = new MyTask("Thread-2");
thread1.start();
thread2.start();
}
}
Thread-1 running: 1
Thread-2 running: 1
Thread-3 running: 1
Thread-1 running: 2
Thread-2 encountered an exception: Error in Thread-2
Thread-3 running: 2
Thread-1 running: 3
Thread-3 running: 3
Thread States
A thread in Java can be in one of these states:
New: Created but not started (
Thread t = new Thread()
).Runnable: Ready to run but waiting for the CPU.
Running: Currently executing.
Blocked/Waiting: Waiting for another thread to finish or a resource to become available.
Terminated: Completed execution.
With this, I conclude my Java learning series. Next, I plan to explore Java Input/Output streams and file handling, which go beyond the fundamentals of the language. I will revisit and revise the concepts shared so far, focusing on writing Java programs to solidify my understanding. Following that, I aim to build a Java application that replicates aspects of my DevOps and cloud environment, helping me apply these concepts in a practical context.
Thank you and Happy Learning :)
Subscribe to my newsletter
Read articles from Sumeet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Sumeet
Sumeet
Skilled in managing carrier-grade ISP infrastructure, enterprise environments, and server operations. Enthusiastic about optimizing high-performance networks and exploring emerging technologies. Committed to continuous learning and driven to leverage cloud solutions and automation tools to enhance innovation and efficiency.