Simplifying React Functional Components with TypeScript

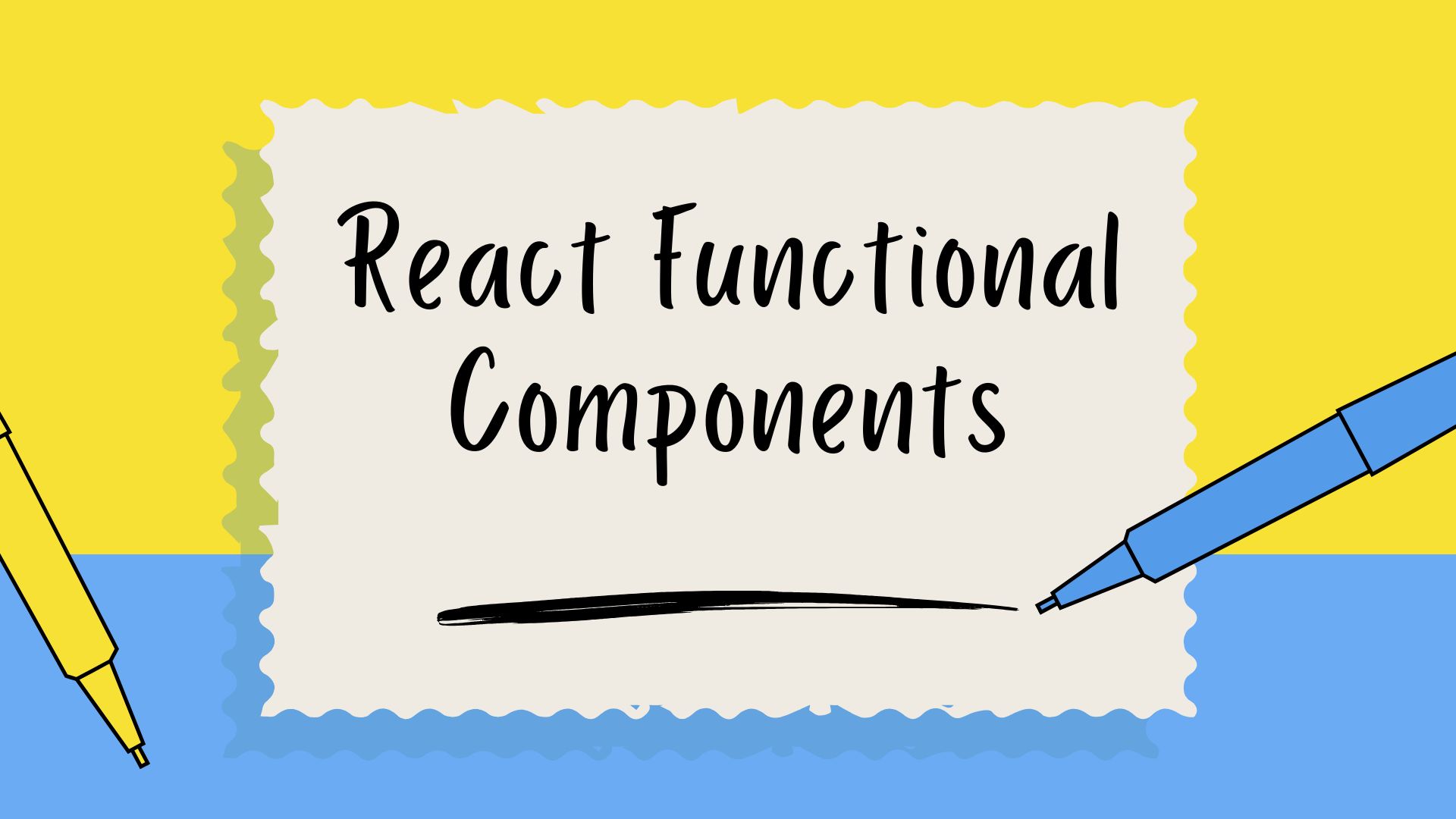
React and TypeScript together provide a robust solution for creating maintainable, type-safe front-end applications. This guide will demonstrate how to create a React functional component using TypeScript, offering a clear example, detailed explanation, and practical tips to enhance your development experience.
Why Combine React with TypeScript?
TypeScript extends JavaScript with static typing, which brings several advantages to React development:
Early Error Detection: TypeScript detects errors at compile time, allowing developers to address issues early and reducing the likelihood of bugs in production.
Enhanced Tooling: Gain access to better autocomplete, refactoring, and inline documentation in your code editor.
Improved Clarity: Type annotations serve as documentation, making your code more readable and maintainable.
Step-by-Step: Creating a Functional Component
Let’s build a React functional component with TypeScript, step by step.
Step 1: Define the Props Type
Start by defining the structure of the props your component will accept. When defining the type, it's a good practice to keep optional parameters at the end. This ensures clarity and avoids confusion when passing props.
// Define the type for the component props
type UserCardProps = {
name: string; // The user's name (required)
onMessageSend: (message: string) => void; // Callback function when a message is sent
age?: number; // The user's age (optional)
};
Step 2: Create the Functional Component
Leverage the props type to define the functional component and ensure the return type is JSX.Element
.
import React from "react";
// Functional component that displays user information and provides a message button
const UserCard: React.FC<UserCardProps> = ({ name, age, onMessageSend }): JSX.Element => {
return (
<div>
{/* Display the user's name */}
<h2>{name}</h2>
{/* Conditionally render the user's age if provided */}
{age && <p>Age: {age}</p>}
{/* Button to send a message using the onMessageSend callback */}
<button onClick={() => onMessageSend(`Hello, ${name}!`)}>Send Message</button>
</div>
);
};
export default UserCard;
Step 3: Use the Component in a Parent Component
Here’s an example of how the UserCard
component can be used in a parent component:
import React from "react";
import UserCard from "./UserCard";
// Parent component that uses the UserCard component
const App: React.FC = (): JSX.Element => {
// Function to handle sending a message
const handleSendMessage = (message: string): void => {
alert(message); // Display the message in an alert box
};
return (
<div>
{/* Render the UserCard component with required props */}
<UserCard name="Alice" age={30} onMessageSend={handleSendMessage} />
</div>
);
};
export default App;
Tips for Writing Type-Safe React Components
Use Explicit Prop Types: Clearly define the structure of props with
type
orinterface
and mark optional properties with?
.Example:
// Define the props for a Profile component type ProfileProps = { username: string; // Required username property bio?: string; // Optional bio property };
Avoid Overuse of React.FC: Although
React.FC
can be convenient, many developers opt to explicitly define thechildren
prop to maintain greater precision and control.Example:
// Define props with explicit children typing type ContainerProps = { children: React.ReactNode; // Children can be any valid React node }; // Functional component with explicit children prop const Container: React.FC<ContainerProps> = ({ children }) => ( <div className="container">{children}</div> );
Set Defaults for Optional Props: Simplify your components by providing default values for optional props in the function signature.
Example:
// Functional component with default value for the user prop const WelcomeMessage: React.FC<{ user?: string }> = ({ user = "Guest" }) => ( <p>Welcome, {user}!</p> );
Leverage TypeScript Utility Types: Types like
Partial
,Pick
, andOmit
simplify prop transformations.Example:
// Full profile type with all properties type FullProfile = { id: string; name: string; email: string; }; // Basic profile type excluding the email property type BasicProfile = Omit<FullProfile, "email">;
Use Enums for Fixed Sets of Values: Enums ensure type safety for props with a predefined set of values.
Example:
// Enum for button types enum ButtonType { PRIMARY = "primary", SECONDARY = "secondary", } // Props for a Button component type ButtonProps = { type: ButtonType; // Type of the button, constrained to the enum values onClick: () => void; // Click event handler }; // Functional component rendering a button const Button: React.FC<ButtonProps> = ({ type, onClick }) => ( <button className={type} onClick={onClick}>Click Me</button> );
Conclusion
Integrating TypeScript with React functional components improves type safety and makes your codebase more robust. By clearly defining props, using default values, and leveraging utility types, you can write maintainable and error-resistant components. Happy learning! :)
Subscribe to my newsletter
Read articles from The Syntax Node directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

The Syntax Node
The Syntax Node
I am a JavaScript Full Stack Developer with expertise in front-end frameworks like React and Angular, and back-end technologies such as Node.js and Express.