Genuary2025_ScrollMaps
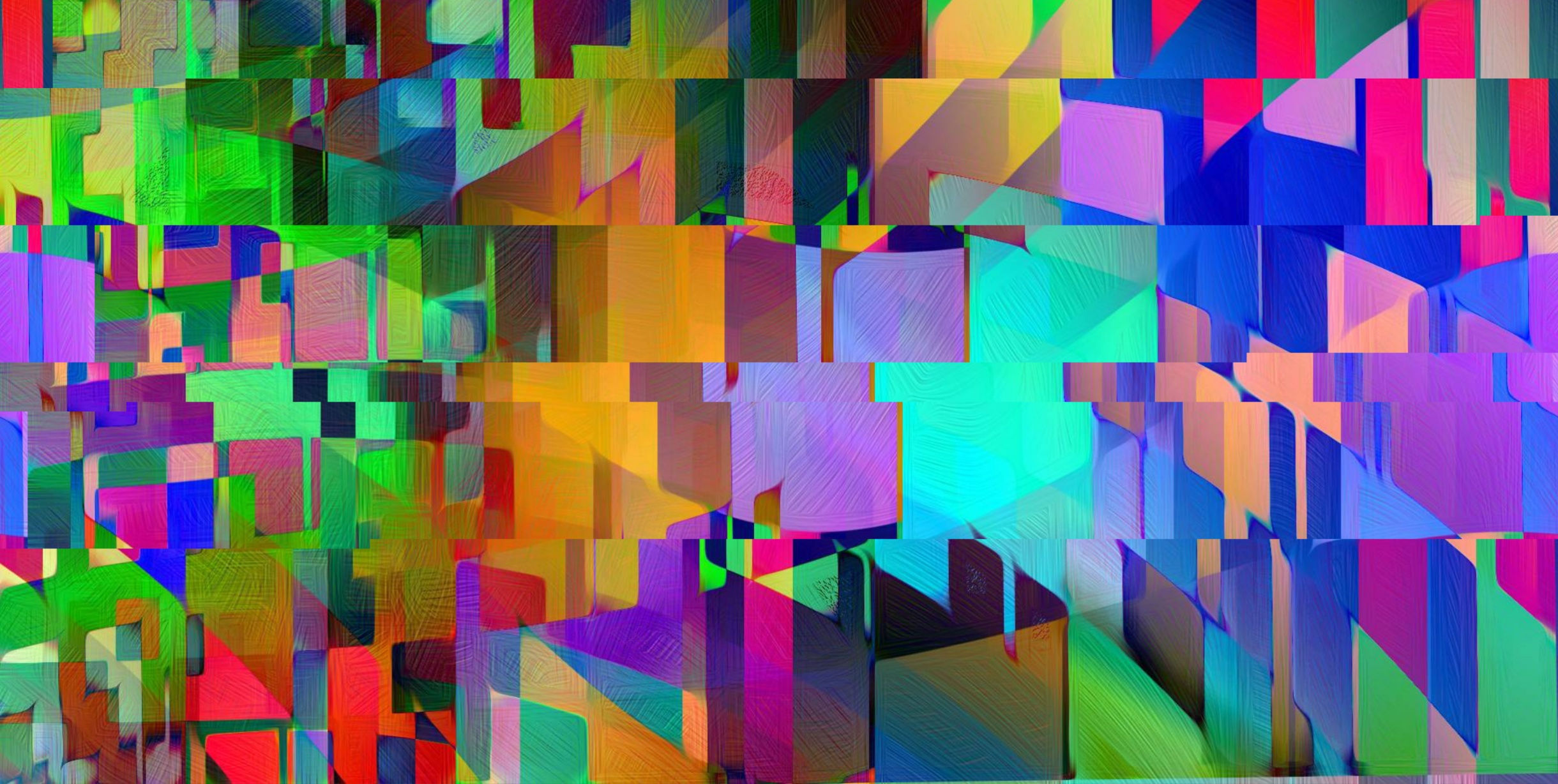
3 min read
Table of contents

MappingBeyondHeat
The prompt for Genuary2025 Day 21 is “CollisionDetection“, Day 28 is “ InfiniteScroll “ & Day 30 is “ AbstractMap ”
ScrollMaps uses Python, Hydra & SonicPi to bring the three prompts together: Heat Maps & Radial Maps in Python, Abstraction & scrolling with Hydra and Collision Detection used for the SonicPi composition.
Poetry
Maps Scrolling
Paths Unrolling
Abstraction Evading
Infinite Scrolls Shifting
Lifting
To the next point where the shade is in
Video
Code
Python
HeatMaps
#battleship is a 10 x 10 board game, for the purpose of this, we will be arranging various new classes of ships
import numpy as np
import seaborn as sns
import matplotlib.pylab as plt
def battle():
#using the 0 & 1 to represent a ship versus the ocean and having a weighted value of 0.8 for ocean, 0.18 for boats and 0.02 for mines
boats = [0,1,2,3,4,5,6,7,8,9,10,11]
prob = [0.025,0.025,0.05,0.05,0.05,0.05,0.1,0.1,0.1,0.1,0.2,0.15]
#by adding the (10,10) instead of 5, able to make an array with the weighted values
shipyard2 = np.random.choice(boats, (16,16), p=prob)
return shipyard2
def plotting(x):
letters = ['A','B','C','D','E','F','G','H','I','J','K','L','M','N','O','P'] #set for the columns
uniform_data = x
ax = sns.heatmap(uniform_data, linewidth=0.5,cmap="Reds") #changing it to blue for ocean representation
ax.set_xticklabels(letters) #setting the ticks of the x axis to letters
plt.show()
return x
#plotting(battle())
Plot
import numpy as np
import seaborn as sns
import matplotlib.pyplot as plt
def plotting(num,var_mean1,var_mean2,rate,styling):
sns.set_theme(style="dark")
# Simulate data from a bivariate Gaussian
n = num
mean = [0, var_mean2]
cov = [(2, var_mean1), (var_mean1, .2)]
rng = np.random.RandomState(rate)
x, y = rng.multivariate_normal(mean, cov, n).T
# Draw a combo histogram and scatterplot with density contours
f, ax = plt.subplots(figsize=(6, 6))
sns.scatterplot(x=x, y=y, s=5, color=".15")
sns.histplot(x=x, y=y, bins=50, pthresh=.1, cmap= styling)
sns.kdeplot(x=x, y=y, levels=5, color="w", linewidths=1)
plotting(5000,0.4,1,5,'Set3')
plotting(20000,0.8,0,10,'mako')
plotting(250,1.2,0.5,3,'cool')
Hydra
Abstacting the Map
s1.initScreen()
src(s1).scale(2).repeat(1,2).modulatePixelate(src(s1),0.31).modulateScrollX(src(s1)).modulateScrollY(src(s1).repeat(3,3).colorama()).out()
Quad Code
s1.initScreen()
s2.initScreen()
s3.initScreen()
s0.initScreen()
src(s1).modulateRotate(s1).out(o0)
src(s2).modulatePixelate(s2).out(o2)
src(s3).ModulateScrollX(s3).out(o1)
src(s0).out(o3)
render()
SonicPi - Detection
def Chosen(x,timer)
#takes the arrays and uses a random seed to reshuffle the order and returns back
use_random_seed Time.now.to_i / timer
y = x.shuffle.shuffle
y = y * 3
z = y.shuffle
return z
end
def Collisions(a, b)
#checks to see which numbers are the same position between the two arrays
#treats this as the collision detection
c = []
count = 0
#this will do a counter to see which numbers are in the same position
#and for those that are in the same position it will return those that "collide"
while count < a.length - 1
if a[count] == b[count]
c.append(a[count])
end
count = count + 1
end
puts c
return c
end
#detection comes with the chipbass sound
live_loop :detection2 do
use_random_seed Time.now.to_i / 3
#with this loop counter this would play the next tick, in every collision iteration
x = Collisions(Chosen([30,4,5,12,6,19,7,8,9,12,17,25],7),Chosen([30,4,5,12,6,19,7,8,9,12,17,25],9))
with_fx [:flanger,:ixi_techno].choose do
use_synth [:chipbass,:piano,:prophet ].choose
play x.tick * 5
end
sleep [0.25,0.5,0.75,1].choose
end
live_loop :detection1 do
use_random_seed Time.now.to_i / 3
#with this loop counter this would play the next tick, in every collision iteration
x = Collisions(Chosen([30,4,5,12,6,19,7,8,9,12,17,25],3),Chosen([30,4,5,12,6,19,7,8,9,12,17,25],2))
with_fx [:flanger,:ixi_techno].choose do
use_synth [:chipbass,:piano,:prophet ].choose
play x.tick
end
sleep [0.25,0.5,0.75,1].choose
end
0
Subscribe to my newsletter
Read articles from Kofi / Illestpreacha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
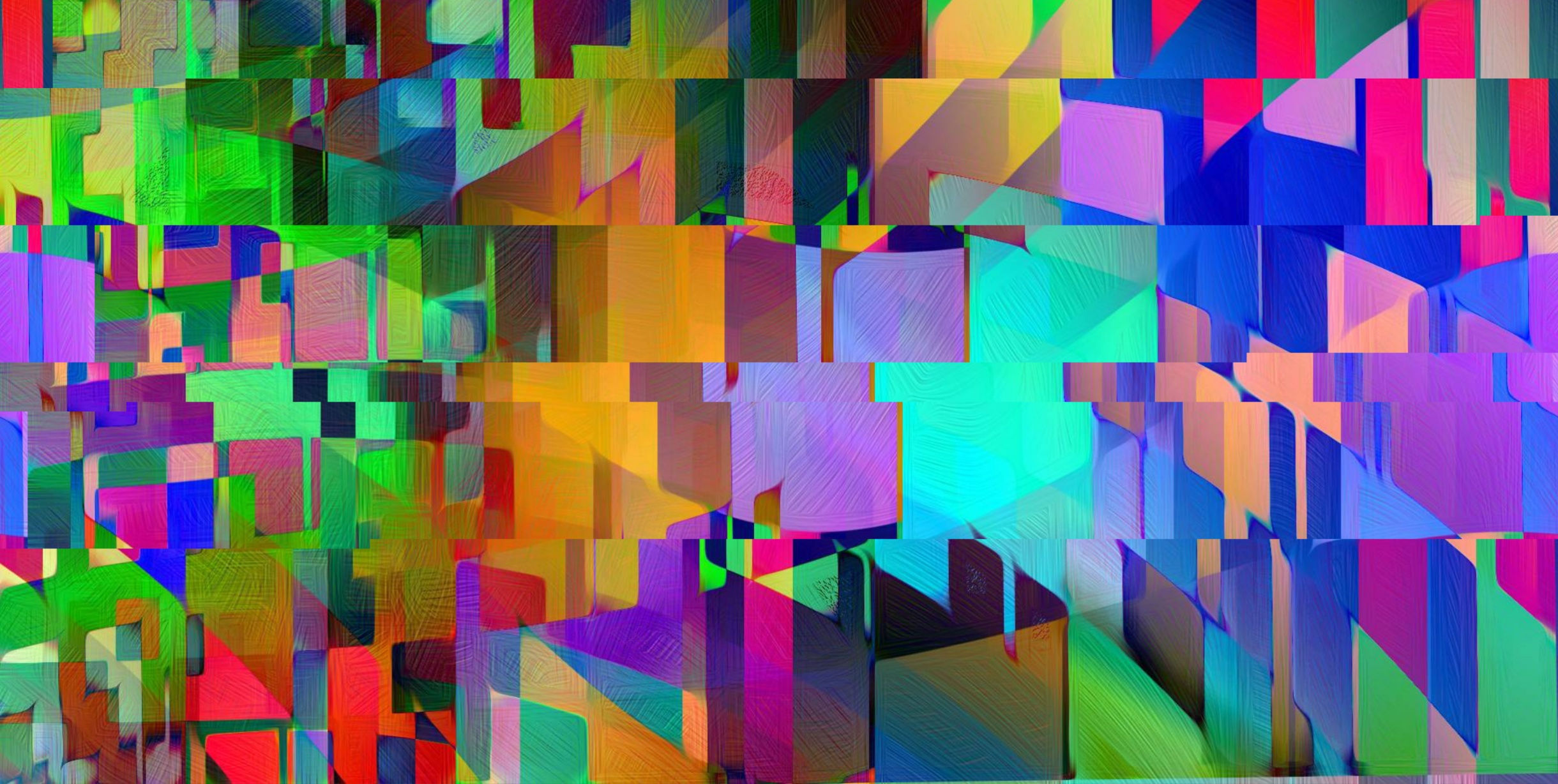