Javascript for 3d animations with Three.js
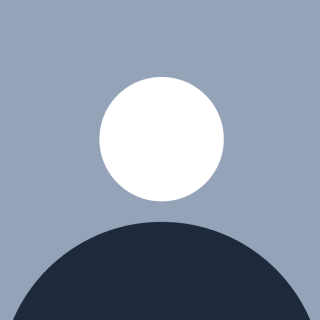
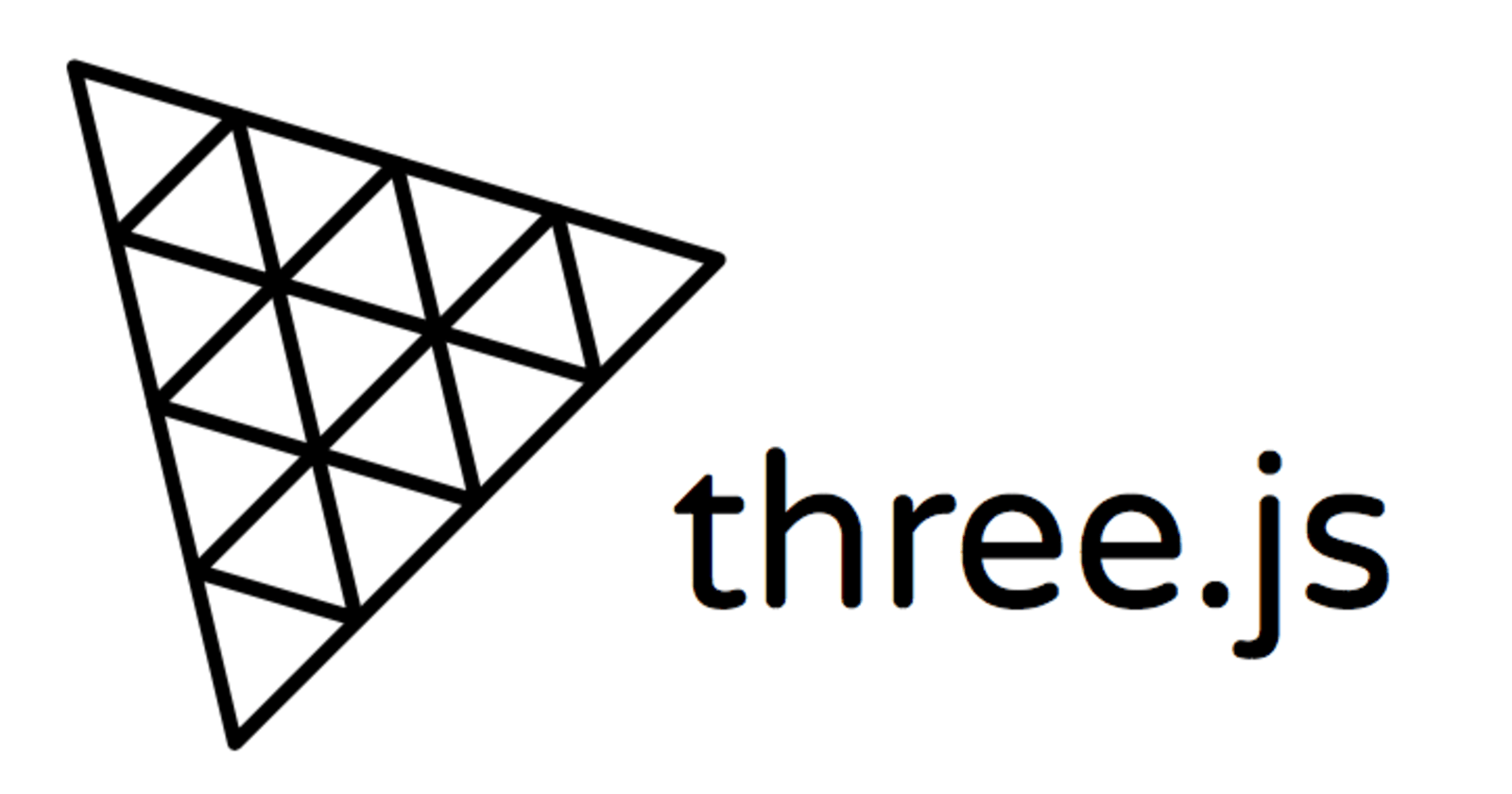
Introduction
Introduction
Overview of 3D animations in web development
3D animations have become an integral part of modern web development, enhancing user engagement and providing immersive experiences. They are widely used in various applications, from interactive websites to complex simulations.
Introduction to Three.js as a tool for creating 3D animations
Three.js is a powerful JavaScript library that simplifies the creation of 3D graphics in the browser. It provides a wide range of features and tools that make it accessible for both beginners and experienced developers to create stunning 3D animations.
Purpose of the article: to guide beginners on getting started with Three.js
This article aims to provide a comprehensive guide for beginners to get started with Three.js, covering everything from setting up the environment to creating simple 3D objects and animations.
Setting Up the Environment
Preparing the Development Environment
To begin creating 3D animations with Three.js, it's essential to set up a suitable development environment. This involves ensuring you have the necessary software and tools, as well as a basic understanding of JavaScript and web development concepts.
Required Software and Tools
You'll need a code editor, such as Visual Studio Code or Sublime Text, and a modern web browser like Chrome or Firefox. Additionally, Node.js and npm (Node Package Manager) are required for managing packages and dependencies.
Basic Knowledge Prerequisites
Familiarity with HTML, CSS, and JavaScript is crucial. Understanding the Document Object Model (DOM) and basic programming concepts will also be beneficial.
Installing Three.js
Three.js can be installed in two primary ways: using npm or incorporating it via a Content Delivery Network (CDN).
Using npm
To install Three.js using npm, run the following command in your terminal:
npm install three
This will add Three.js to your project's dependencies, allowing you to import and use it in your JavaScript files.
Incorporating via a CDN
Alternatively, you can include Three.js in your project by adding a script tag in your HTML file that points to a CDN:
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
Setting Up a Project Structure
Organize your project by creating a directory structure that separates your HTML, CSS, and JavaScript files. This will help maintain a clean and manageable codebase as your project grows.
Understanding the Basics of Three.js
Core Components of Three.js
Three.js is built around several core components that work together to create 3D graphics.
Scene
The scene is the container that holds all the objects, lights, and cameras in your 3D world. It acts as the stage where all the action takes place.
Camera
The camera determines what part of the scene is visible to the viewer. It defines the perspective from which the scene is rendered.
Renderer
The renderer is responsible for drawing the scene from the camera's perspective onto the screen. It translates the 3D scene into a 2D image.
Creating a Basic Scene
To create a basic scene in Three.js, you need to set up the scene, add a camera, initialize the renderer, and render the scene.
Setting Up a Scene
First, create a new scene:
const scene = new THREE.Scene();
Adding a Camera
Next, add a camera to the scene. A common choice is the PerspectiveCamera, which mimics the way the human eye sees:
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
camera.position.z = 5;
Initializing the Renderer
Initialize the renderer and set its size to match the window dimensions:
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
Rendering the Scene
Finally, render the scene using the renderer:
function animate() {
requestAnimationFrame(animate);
renderer.render(scene, camera);
}
animate();
This setup will create a basic 3D scene that you can expand by adding objects, lights, and more complex interactions.
Creating a Simple 3D Object
Introduction to Geometries and Materials
In Three.js, creating 3D objects involves defining geometries and materials. Geometries determine the shape of the object, while materials define its appearance, such as color and texture.
Common Types of Geometries
Three.js provides several built-in geometries, including BoxGeometry, SphereGeometry, and PlaneGeometry. These geometries serve as the building blocks for creating various 3D shapes.
Basic Materials and Their Properties
Materials in Three.js, such as MeshBasicMaterial and MeshStandardMaterial, control how objects interact with light and how they appear on the screen. Properties like color, opacity, and texture can be adjusted to achieve the desired look.
Adding a Cube to the Scene
To add a cube to your scene, you'll need to define its geometry and material, combine them into a mesh, and then add the mesh to the scene.
Defining Geometry and Material
First, create a BoxGeometry for the cube and a MeshBasicMaterial to define its appearance:
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
Combining to Create a Mesh
Next, combine the geometry and material to create a mesh:
const cube = new THREE.Mesh(geometry, material);
Adding the Mesh to the Scene
Finally, add the cube mesh to your Three.js scene:
scene.add(cube);
This process will render a simple green cube in your 3D scene, which you can further manipulate and animate.
Lighting and Shadows
Importance of Lighting in 3D Scenes
Lighting is crucial in 3D scenes as it enhances realism, depth, and mood. Proper lighting can dramatically affect how objects appear, highlighting textures and creating shadows that add to the scene's overall visual appeal.
Types of Lights in Three.js
Three.js offers various types of lights to simulate different lighting conditions and effects.
AmbientLight
AmbientLight provides a uniform light that affects all objects in the scene equally, without casting shadows. It's useful for ensuring that no part of the scene is completely dark.
DirectionalLight
DirectionalLight simulates sunlight, where light rays are parallel and come from a specific direction. It can cast shadows, making it ideal for outdoor scenes.
PointLight
PointLight emits light in all directions from a single point, similar to a light bulb. It can cast shadows and is suitable for simulating localized light sources.
Adding Lights to the Scene
To illuminate your scene, you need to create light sources and add them to the scene.
Creating a Light Source
Here's how to create and add different types of lights:
// Ambient Light
const ambientLight = new THREE.AmbientLight(0x404040); // Soft white light
scene.add(ambientLight);
// Directional Light
const directionalLight = new THREE.DirectionalLight(0xffffff, 1);
directionalLight.position.set(1, 1, 1).normalize();
scene.add(directionalLight);
// Point Light
const pointLight = new THREE.PointLight(0xffffff, 1, 100);
pointLight.position.set(50, 50, 50);
scene.add(pointLight);
Adjusting Light Properties
You can adjust properties like color, intensity, and position to achieve the desired lighting effect. For example, changing the intensity of a light can make it brighter or dimmer.
Enabling Shadows
To enable shadows, you need to configure both the renderer and the lights:
// Enable shadow map in the renderer
renderer.shadowMap.enabled = true;
// Enable shadows for the directional light
directionalLight.castShadow = true;
// Configure shadow properties
directionalLight.shadow.mapSize.width = 512; // Default
directionalLight.shadow.mapSize.height = 512; // Default
directionalLight.shadow.camera.near = 0.5; // Default
directionalLight.shadow.camera.far = 500; // Default
By setting up lighting and shadows, you can create more dynamic and visually appealing 3D scenes.
Animation Basics
Introduction to Animation Loop in Three.js
An animation loop in Three.js is essential for creating dynamic and interactive 3D scenes. It continuously updates the scene, allowing for smooth animations and real-time interactions.
Simple Animation Techniques
Three.js provides various techniques to animate objects, such as changing their position, rotation, or scale over time. These animations can be achieved by updating object properties within the animation loop.
Rotating Objects
To rotate an object, you can increment its rotation properties within the animation loop:
function animate() {
requestAnimationFrame(animate);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
Moving the Camera
Moving the camera can create the effect of navigating through the scene. You can adjust the camera's position or lookAt properties within the animation loop:
function animate() {
requestAnimationFrame(animate);
camera.position.x += 0.01;
camera.lookAt(scene.position);
renderer.render(scene, camera);
}
animate();
Using the requestAnimationFrame for Smooth Animations
The requestAnimationFrame
function is used to create smooth animations by synchronizing the frame rate with the display's refresh rate. It ensures that animations run efficiently and smoothly across different devices:
function animate() {
requestAnimationFrame(animate);
// Update object properties here
renderer.render(scene, camera);
}
animate();
By utilizing these techniques, you can create engaging and fluid animations in your Three.js projects.
Resources for Further Learning
Official Three.js documentation
API Documentation: Comprehensive reference for all classes, methods, and properties in Three.js.
Manual: Guides and explanations on various topics to assist both beginners and advanced users.
Examples: A collection of practical examples demonstrating different features and capabilities of Three.js.
Conclusion
Recap of Key Concepts and Steps for Getting Started
In this article, we've explored the foundational aspects of using Three.js for creating 3D animations. We began by setting up the development environment, including the necessary software and tools. We then delved into the core components of Three.js, such as the scene, camera, and renderer, and demonstrated how to create a basic 3D scene. Additionally, we covered the creation of simple 3D objects, the importance of lighting and shadows, and the basics of animation loops to bring your scenes to life.
Encouragement to Experiment and Explore Advanced Topics
As you become more comfortable with Three.js, I encourage you to experiment with different geometries, materials, and lighting setups to enhance your 3D scenes. Don't hesitate to explore advanced topics such as textures, shaders, and integrating Three.js with other libraries and frameworks. The possibilities are vast, and with practice, you'll be able to create increasingly complex and visually stunning animations. Keep experimenting and pushing the boundaries of your creativity!
Subscribe to my newsletter
Read articles from MUHAMMAD MUBIN FARID directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by