How to Copy AWS SSM Parameters: CLI Commands & Python Scripts Explained
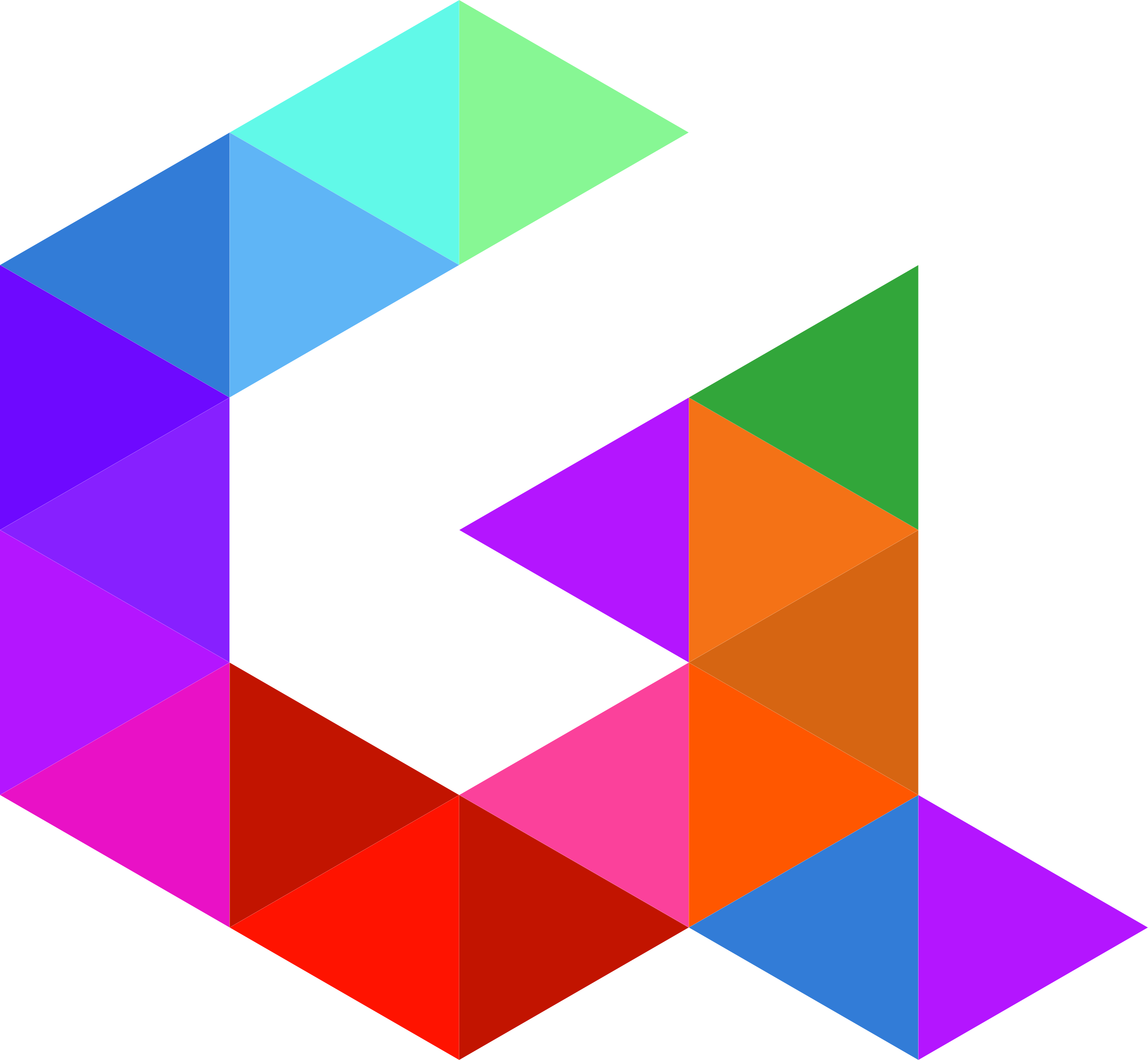
Introduction
AWS Systems Manager Parameter Store is a powerful service that allows you to securely store and manage configuration data and secrets. However, there are times when you need to duplicate or rename an existing parameter, whether for environment migrations, backups, or automation workflows.
In this guide, weβll explore how to copy AWS SSM parameters using both AWS CLI and Python scripts (Boto3). By the end of this tutorial, you'll be able to duplicate parameters with ease while ensuring security and efficiency.
Why Copy AWS SSM Parameters?
Before diving into the technical steps, let's explore common use cases for copying SSM parameters:
β
Environment Migration β Moving parameters from a development to a staging or production environment.
β
Parameter Renaming β Reorganizing parameters for better structure.
β
Backup & Disaster Recovery β Keeping a backup of important parameters.
β
Cross-Account Sharing β Duplicating parameters across AWS accounts.
Method 1: Copying SSM Parameters Using AWS CLI
AWS CLI provides a straightforward way to fetch and create parameters. Below is a step-by-step guide.
Step 1: Get the Parameter Value
First, retrieve the existing parameter value using:
aws ssm get-parameter --name "/old/parameter/name" --query "Parameter.Value" --output text
If it's a SecureString, include --with-decryption
:
aws ssm get-parameter --name "/old/parameter/name" --with-decryption --query "Parameter.Value" --output text
Step 2: Create the New Parameter
Now, take the retrieved value and store it under a new name:
aws ssm put-parameter --name "/new/parameter/name" --value "your-value-here" --type "String" --overwrite
For a SecureString parameter:
aws ssm put-parameter --name "/new/parameter/name" --value "your-value-here" --type "SecureString" --overwrite
Step 3: Automate the Process
To automate, use this one-liner command to copy an existing parameter to a new one:
aws ssm get-parameter --name "/old/parameter/name" --query "Parameter.Value" --output text | \
xargs -I {} aws ssm put-parameter --name "/new/parameter/name" --value "{}" --type "String" --overwrite
For SecureString:
aws ssm get-parameter --name "/old/parameter/name" --with-decryption --query "Parameter.Value" --output text | \
xargs -I {} aws ssm put-parameter --name "/new/parameter/name" --value "{}" --type "SecureString" --overwrite
β Done! Your new parameter is now created with the same value.
Method 2: Copying SSM Parameters Using Python (Boto3)
If you're working with AWS automation, using Python with Boto3 is a great approach. This method allows you to copy parameters in bulk or even across AWS accounts.
Step 1: Install Boto3 (If Not Installed)
pip install boto3
Step 2: Copy a Single Parameter
This script fetches a parameter and creates a new one:
import boto3
ssm = boto3.client('ssm')
def copy_parameter(old_name, new_name):
response = ssm.get_parameter(Name=old_name, WithDecryption=True)
value = response['Parameter']['Value']
param_type = response['Parameter']['Type']
ssm.put_parameter(
Name=new_name,
Value=value,
Type=param_type,
Overwrite=True
)
print(f"β
Copied {old_name} to {new_name}")
# Example usage
copy_parameter('/old/parameter/name', '/new/parameter/name')
Step 3: Copy Multiple Parameters Under a Path
For bulk copying (e.g., from /dev/
to /staging/
), use this script:
def copy_parameters(old_prefix, new_prefix):
response = ssm.get_parameters_by_path(Path=old_prefix, Recursive=True, WithDecryption=True)
for param in response['Parameters']:
old_name = param['Name']
new_name = old_name.replace(old_prefix, new_prefix, 1) # Replace prefix
ssm.put_parameter(Name=new_name, Value=param['Value'], Type=param['Type'], Overwrite=True)
print(f"β
Copied {old_name} β {new_name}")
# Example usage
copy_parameters('/dev/', '/staging/')
Best Practices for Managing SSM Parameters
πΉ Use Parameter Policies β Set expiration policies to manage secrets automatically.
πΉ Enable Encryption β Always use SecureString for sensitive data.
πΉ Organize Parameters by Environment β Follow a structure like /env/service/parameter-name
.
πΉ Use Tags β Categorize parameters for better management (--tags Key=Environment,Value=Production
).
Conclusion
Copying AWS SSM parameters is essential for environment migrations, backups, and automation workflows. Whether you prefer AWS CLI for quick tasks or Python scripts for automation, this guide has you covered.
β
CLI is great for quick, one-off copies.
β
Python is best for automating bulk copies or cross-account migration.
Now, you can easily duplicate AWS SSM parameters while maintaining security and efficiency. π
Let me know if you have any questions or need additional refinements! ππ¬
Need Custom Development? Get Expert Help! π
Facing complex tech challenges? Our expert developers can build, optimize, and scale your solution. Whether it's custom software, backend architecture, API integrations, or performance tuning, we have you covered.
π Letβs build something amazing together! Get in touch
Subscribe to my newsletter
Read articles from Ghanshyam Digital directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
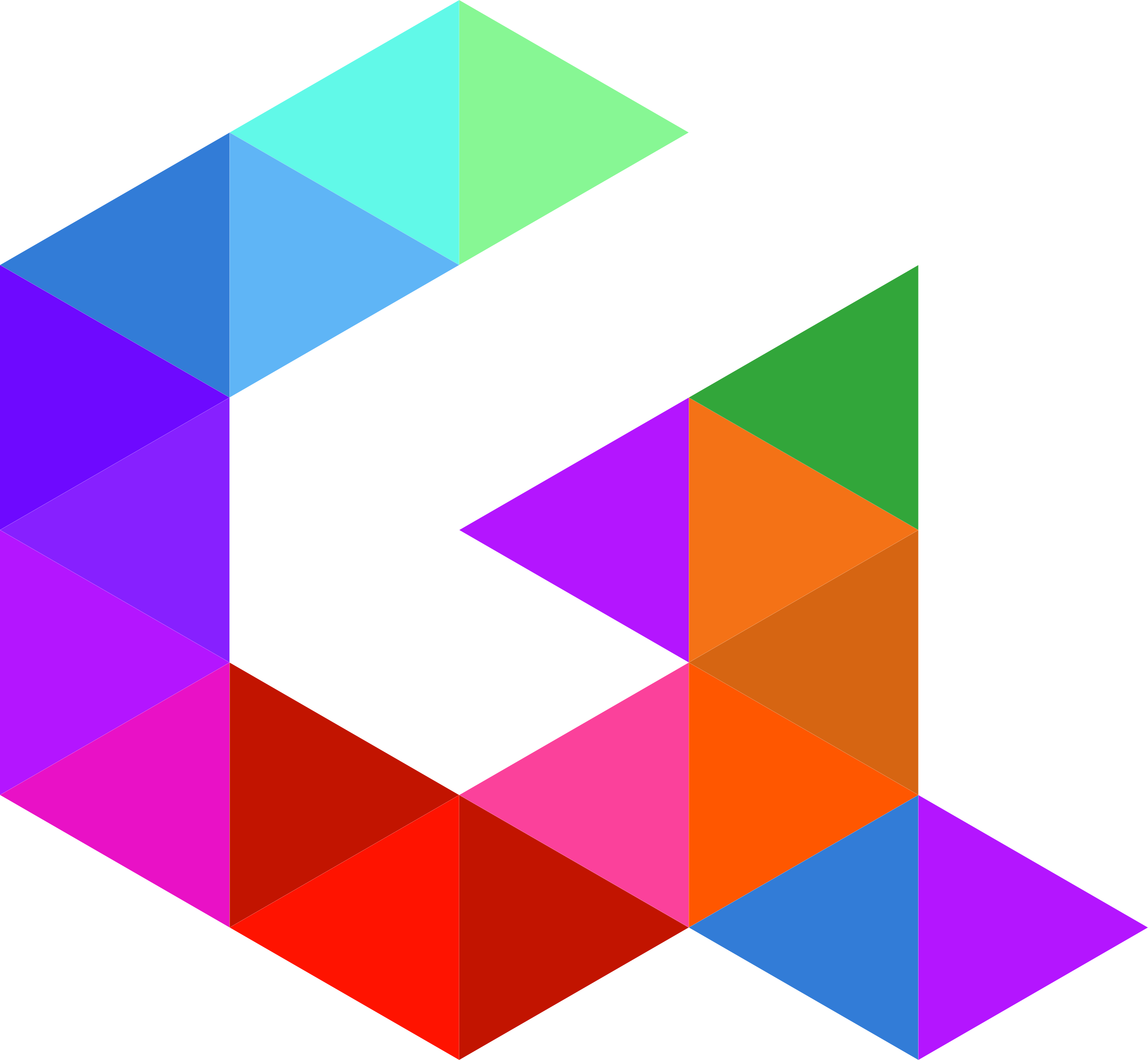
Ghanshyam Digital
Ghanshyam Digital
A company founded back in 2019 by two visionary Engineers to create and provide you with the best results possible. We are a web developing company with great vision and plans for the future to be a part of others success. We understand that you have deadlines and reputation to keep... so we will meet ours! You can rely on Ghanshyam Digital To handle your job expertly every time.