Jenkins Shared Libraries: Reusable, Scalable, and Efficient Pipelines
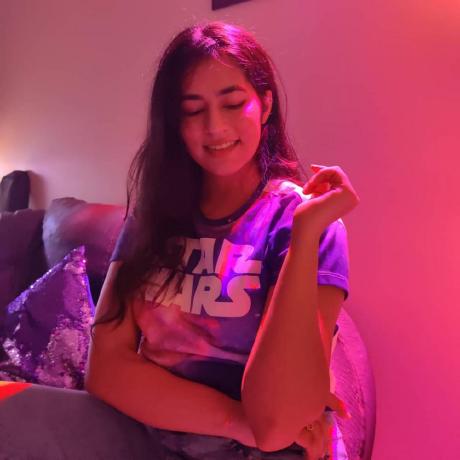
Jenkins Shared Libraries are reusable code snippets that help you centralize and modularize common Jenkins pipeline logic. They allow you to write custom functions, steps, or entire pipeline logic in Groovy and reuse them across multiple Jenkinsfiles.
πΉ Why Use Shared Libraries?
Code Reusability β Avoid duplicating pipeline code across multiple projects.
Maintainability β Easier to update pipeline logic in a single place.
Modularity β Separate business logic from pipeline code.
Security β Reduce security risks by defining approved scripts.
πΉ Setting Up a Jenkins Shared Library
1οΈβ£ Create a Repository for the Library
Shared libraries are typically stored in a Git repository.
2οΈβ£ Define the Library Structure
A Shared Library follows a specific structure:
vbnetCopyEditπ shared-library/
βββ π src/ # Groovy classes (optional)
β βββ com/example/MyClass.groovy
β
βββ π vars/ # Reusable pipeline functions (global variables)
β βββ myCustomStep.groovy
β βββ deployApp.groovy
β
βββ π resources/ # Static resources (config files, templates)
β
βββ π README.md # Documentation
βββ π build.gradle # Optional (if using Gradle)
βββ π pom.xml # Optional (if using Maven)
3οΈβ£ Writing a Simple Shared Library Function
Global Function (Inside vars/
)
Create a Groovy script inside the vars/
directory.
π vars/helloWorld.groovy
groovyCopyEditdef call(String name = 'User') {
echo "Hello, ${name}! Welcome to Jenkins Shared Libraries!"
}
β
This function can be used in any pipeline by calling helloWorld()
.
4οΈβ£ Configuring the Shared Library in Jenkins
Go to: Jenkins Dashboard β Manage Jenkins β Configure System.
Scroll to: "Global Pipeline Libraries."
Add a new library:
Name:
my-shared-library
Default version:
main
(or specify a branch/tag)Source Code Management: Select Git and provide the repo URL.
5οΈβ£ Using the Shared Library in a Pipeline
You can use the shared library in a Jenkinsfile
in two ways:
a) Load Implicitly (Recommended)
If the library is configured in Jenkins, use:
groovyCopyEdit@Library('my-shared-library') _
pipeline {
agent any
stages {
stage('Test Shared Library') {
steps {
helloWorld('DevOps Engineer')
}
}
}
}
b) Load Dynamically (Without Pre-configuring in Jenkins)
If you don't configure it globally, load it directly:
groovyCopyEdit@Library('github.com/my-org/shared-library@main') _
import helloWorld
pipeline {
agent any
stages {
stage('Test') {
steps {
helloWorld('John')
}
}
}
}
πΉ Advanced Example with a Class
If you need structured Groovy classes, define them inside src/
:
π src/com/example/Utils.groovy
groovyCopyEditpackage com.example
class Utils {
static String greet(String name) {
return "Hello, ${name}!"
}
}
π vars/greetUser.groovy
groovyCopyEditimport com.example.Utils
def call(String name) {
echo Utils.greet(name)
}
π Jenkinsfile
groovyCopyEdit@Library('my-shared-library') _
pipeline {
agent any
stages {
stage('Greeting') {
steps {
greetUser('Alice')
}
}
}
}
πΉ Best Practices
β
Use Versioning β Tag releases or use branches (@v1.0
, @develop
).
β
Avoid Hardcoding Credentials β Use Jenkins Credentials Plugin.
β
Write Tests β Validate shared libraries with Unit tests.
β
Document Functions β Keep a README.md
for usage instructions.
β
Use Static Code Analysis β Tools like SonarQube for quality checks.
Subscribe to my newsletter
Read articles from Ishika kesarwani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
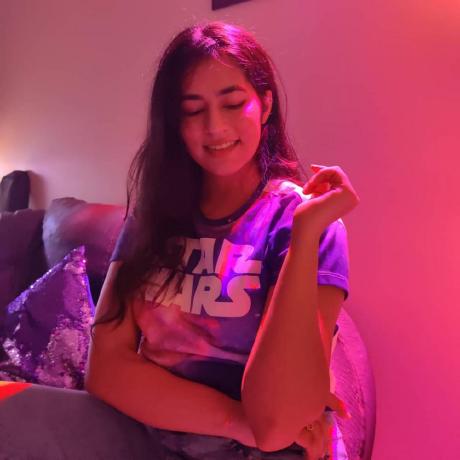