Does Variable Access Use Dynamic Binding in Java?
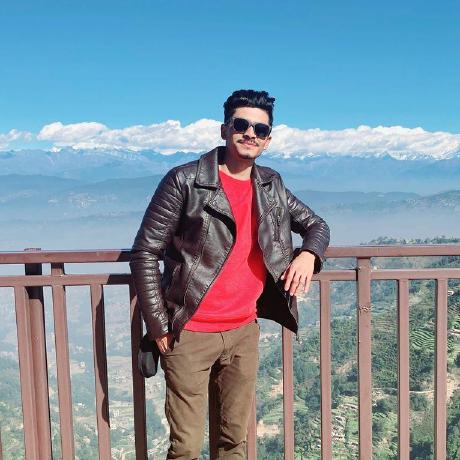
No, variable access does not use dynamic binding in Java. Instead, variables are resolved at compile-time using static binding. However, methods use dynamic binding (runtime polymorphism).
Static vs. Dynamic Binding
Feature | Methods (Dynamic Binding) | Variables (Static Binding) |
Binding Time | Runtime (late binding) | Compile-time (early binding) |
Depends On | Actual object type | Reference type (declared type) |
Polymorphism? | Yes, overridden methods | No, variables are not overridden |
Example: Method vs. Variable Access
class Animal {
String sound = "generic animal sound";
void makeSound() {
System.out.println("Some animal sound");
}
}
class Dog extends Animal {
String sound = "bark";
@Override
void makeSound() {
System.out.println("Bark");
}
}
public class DynamicBindingExample {
public static void main(String[] args) {
Animal myAnimal = new Dog();
// Dynamic binding for the method
myAnimal.makeSound(); // Outputs: Bark
// Variable binding is static, not dynamic
System.out.println(myAnimal.sound); // Outputs: generic animal sound
}
}
Conclusion
Variables are statically bound → Resolved at compile-time based on reference type.
Methods use dynamic binding → Resolved at runtime based on actual object.
This is why overriding applies only to methods, not variables in Java.
Subscribe to my newsletter
Read articles from Bikash Mainali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
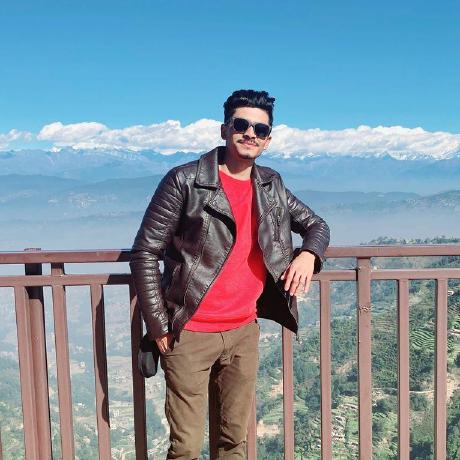
Bikash Mainali
Bikash Mainali
Experienced Java/JavaScript full-stack developer over 6 years of extensive expertise serving key role on elite technical teams developing enterprise software for healthcare, apple ad-platform, banking, and e-commerce. Adaptable problem-solver with high levels of skill in Groovy, Java, Spring, Spring Boot, Hibernate, JavaScript, TypeScript, Angular, Node, Express, React, MongoDB, IBM DB2, Oracle, PL/SQL, Docker, Kubernetes, CI/CD pipelines, AWS, Micro-service and Agile/Scrum. Strong technical skills paired with business-savvy UI design expertise. Personable team player with experience collaborating with diverse cross-functional teams.