JavaScript Temporal
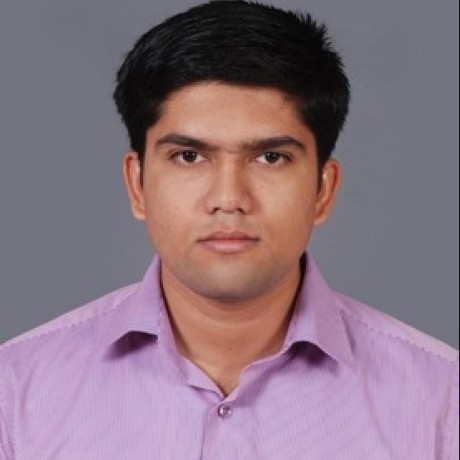

JavaScript Temporal a modern approach to the Date and Time Management
1.What is JavaScript Temporal
To understand Temporal, we need to look at JavaScript's existing Date object. When JavaScript was created in 1995, the Date object was based on Java’s early java.util.Date
implementation, which had several flaws. Java replaced this implementation in 1997, but JavaScript continued with the same API for nearly 30 years, with the known problems.
The primary issues with JavaScript’s Date object includes
It only supports the user’s local time and UTC, with no built-in time zone support
Parsing behavior is unreliable
The Date object is mutable, leading to potential bugs
Working with Daylight Saving Time (DST) and historical calendar changes is difficult
These issues make date and time handling in JavaScript complex and error-prone. Many developers rely on third-party libraries like Moment.js and date-fns to handle these challenges more effectively.
Temporal is designed as a full replacement for the Date object, making date and time management more reliable and predictable. It adds support for time zones, calendar systems, and a variety of built-in methods for conversions, comparisons, and computations. With over 200 utility methods.
2.Core concepts of Temporal
Temporal introduces several key concepts to handle different aspects of date and time
Duration:
Temporal.Duration
represents the difference between two points in time.Points in Time
Unique Points in Time
As a timestamp:
Temporal.Instant
A date-time with a time zone:
Temporal.ZonedDateTime
Time-zone-unaware date/time (“Plain”)
Full date and time:
Temporal.PlainDateTime
Just the date:
Temporal.PlainDate
Year and month:
Temporal.PlainYearMonth
Month and day:
Temporal.PlainMonthDay
Just the time:
Temporal.PlainTime
Now:
Temporal.now
retrieves the current time in different formats.
Let’s see some examples of Temporal
One of the most basic uses of Temporal is getting the current date and time as an ISO string. Unlike the Date object, Temporal allows specifying time zones, simplifying complex time calculations.
// The current date in the system's time zone
const dateTime = Temporal.Now.plainDateTimeISO();
console.log(dateTime); // e.g.: 2025-02-01T10:15:16.124
Working with different calendars is also streamlined. Temporal supports multiple calendar systems, including Hebrew, Chinese, and Islamic. The following example shows how to find the next Chinese New Year
const chineseNewYear = Temporal.PlainMonthDay.from({
monthCode: "M01",
day: 1,
calendar: "chinese",
});
const currentYear = Temporal.Now.plainDateISO().withCalendar("chinese").year;
let nextCNY = chineseNewYear.toPlainDate({ year: currentYear });
// If nextCNY is before the current date, move forward by 1 year
if (Temporal.PlainDate.compare(nextCNY, Temporal.Now.plainDateISO()) <= 0) {
nextCNY = nextCNY.add({ years: 1 });
}
console.log(
`The next Chinese New Year is on ${nextCNY.withCalendar("iso8601").toLocaleString()}`,
);
// The next Chinese New Year is on 2/17/2026
Handling Unix timestamps is another common use case. Many systems (APIs, databases) use Unix Epoch timestamps to represent times. The following example demonstrates how to create an instant from a Unix Epoch timestamp, get the current time using Temporal.now
const launch = Temporal.Instant.fromEpochMilliseconds(1851222399924);
const now = Temporal.Now.instant();
const duration = now.until(launch, { smallestUnit: "hour" });
console.log(`It will be ${duration.toLocaleString("en-US")} until the launch`);
// "It will be 31,600 hr until the launch" <- @js-temporal/polyfill
// "It will be PT31600H until the launch" <- Firefox Nightly
3.Browser Support and Implementation
Support for Temporal is gradually being introduced in experimental browser releases. Firefox currently has the most mature implementation, available in the Nightly version under the javascript.options.experimental.temporal
preference.
Here are the main browser implementation trackers:
Firefox: Building Temporal in Nightly by default.
Safari:
JSC
implementing Temporal.Chrome: Implementing the Temporal proposal.
You can also explore Temporal without modifying browser flags by visiting the TC39 Temporal proposal documentation at https://tc39.es/proposal-temporal/docs/, which includes the @js-temporal/polyfill
library. This allows you to try Temporal examples in the developer console of any browser.
Conclusion
With experimental implementations progressing, now is a great time to start using Temporal and familiarize yourself with what will become the modern approach to handling dates and times in JavaScript. By addressing long-standing issues in the Date object, Temporal provides a more robust and developer-friendly way to work with time in JavaScript applications.
Subscribe to my newsletter
Read articles from nidhinkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
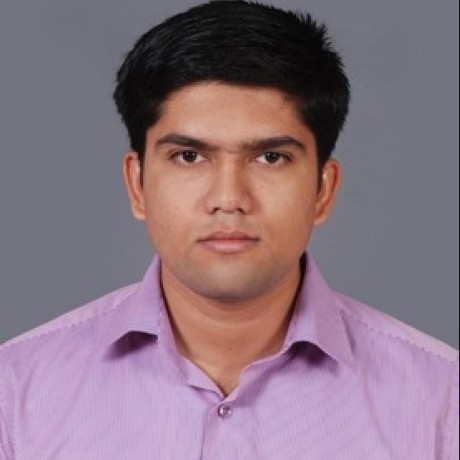