Deep Dive into Components in React

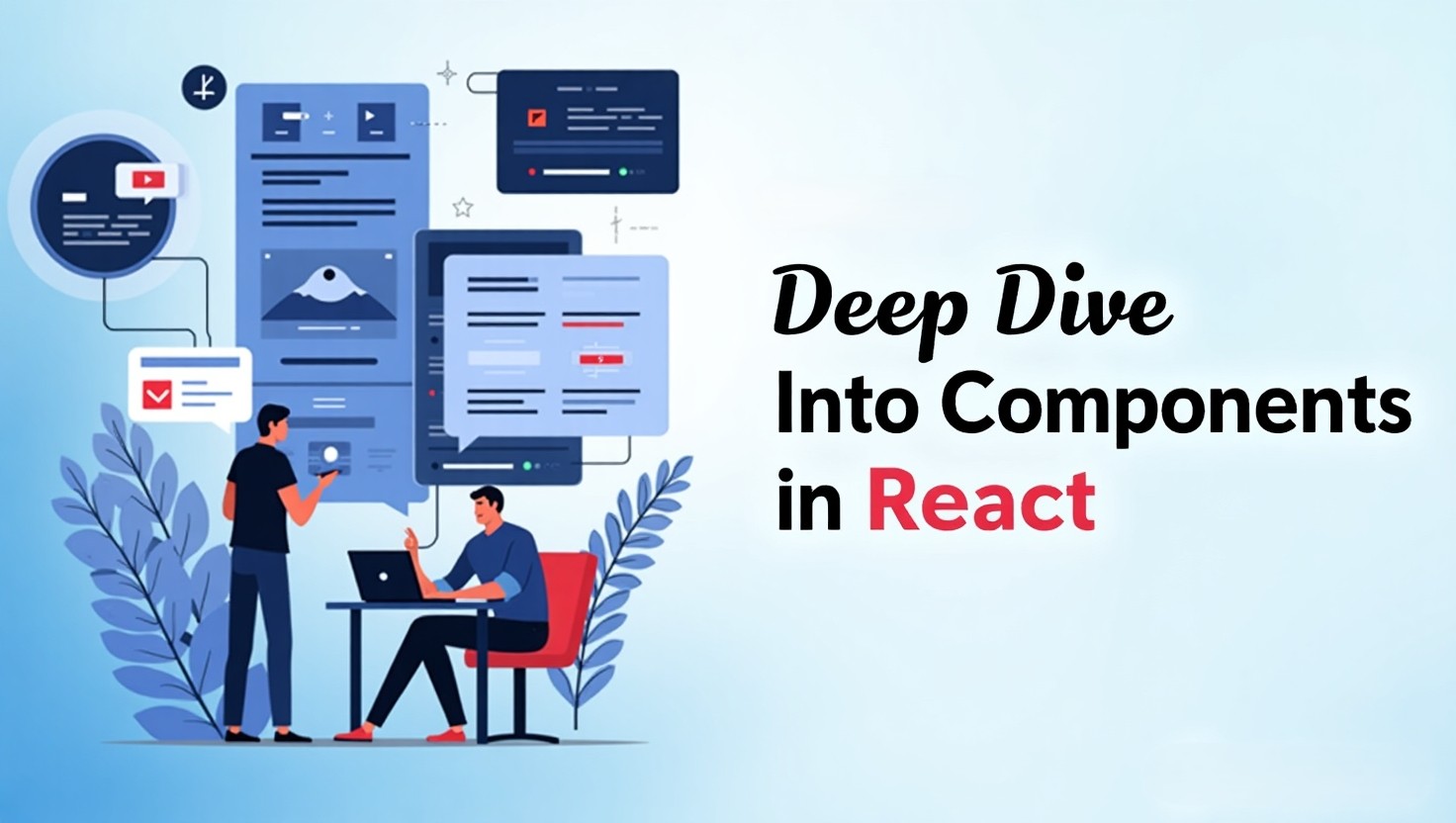
Introduction
React is a highly popular JavaScript library used for building user interfaces, particularly single-page applications where dynamic data is required. One of the key concepts that make React powerful is its component-based architecture. This blog post will take a deep dive into various aspects of React components, including component composition, Higher-Order Components (HOCs), and hooks, specifically useContext
, useReducer
, and custom hooks. We'll provide coding examples along the way to enrich understanding.
Component Composition
At its core, component composition is the practice of creating complex UIs by combining simpler components. React encourages developers to build applications through a hierarchy of smaller, reusable components. This leads to better organization, increased reusability, and improved testability.
Example of Component Composition
Consider a simple application with a header, a main content area, and a footer. We can break it down like this:
import React from 'react';
const Header = () => {
return <h1>My Application</h1>;
};
const Footer = () => {
return <footer>© 2023 My Application</footer>;
};
const MainContent = () => {
return (
<div>
<p>Welcome to my application!</p>
<p>Here is some content...</p>
</div>
);
};
const App = () => {
return (
<div>
<Header />
<MainContent />
<Footer />
</div>
);
};
export default App;
In this example, we have three simple components: Header
, Footer
, and MainContent
. They are composed into a parent component App
, which serves as the main container. This composition allows each part to be developed independently while still being able to function as a cohesive whole.
Advantages of Component Composition
Reusability: Components can be reused across different parts of the application.
Separation of concerns: Each component manages its own state and behavior, leading to cleaner code.
Clarity: Smaller components are easier to read and understand than a large monolithic component.
Higher-Order Components (HOCs)
Higher-Order Components (HOCs) are advanced patterns in React that allow developers to reuse component logic. An HOC is a function that takes a component and returns a new component with additional props or functionality. They don't modify the original component but rather encapsulate behavior to extend or alter it.
Example of a Higher-Order Component
Let's create an HOC that provides logging functionality:
import React from 'react';
const withLogging = (WrappedComponent) => {
return class extends React.Component {
componentDidMount() {
console.log(`Component ${WrappedComponent.name} mounted`);
}
componentWillUnmount() {
console.log(`Component ${WrappedComponent.name} will unmount`);
}
render() {
return <WrappedComponent {...this.props} />;
}
};
};
const MyComponent = () => {
return <div>Hello, World!</div>;
};
const LoggedMyComponent = withLogging(MyComponent);
export default LoggedMyComponent;
In this example, withLogging
is an HOC that logs messages when the wrapped component mounts and unmounts. This is a powerful way to enhance components with additional behavior without altering their source code.
When to Use HOCs
When you need to share functionality across multiple components.
When you want to abstract complex logic from the component itself.
When you need to inject additional props or state into components.
React Hooks Overview
React hooks were introduced in version 16.8 to provide a more straightforward way to manage state and side effects in functional components. They enable the use of state and lifecycle features without having to rely on class components.
useContext
The useContext
hook provides a way to consume context easily. It lets you toggle between components without having to pass props down manually through every level.
Example of useContext
import React, { useContext, createContext } from 'react';
const ThemeContext = createContext('light');
const ThemedComponent = () => {
const theme = useContext(ThemeContext);
return <div style={{ background: theme === 'dark' ? '#333' : '#FFF' }}>Current theme: {theme}</div>;
};
const App = () => {
return (
<ThemeContext.Provider value="dark">
<ThemedComponent />
</ThemeContext.Provider>
);
};
export default App;
In this example, we create a ThemeContext
and use the useContext
hook to access its value. The ThemedComponent
can render styles based on the context without having to grab props from parent components.
useReducer
The useReducer
hook is ideal for managing state when it gets complicated, particularly if state transitions are more elaborate than simple value toggles.
Example of useReducer
import React, { useReducer } from 'react';
const initialState = { count: 0 };
const reducer = (state, action) => {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
};
const Counter = () => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
Count: {state.count}
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
</div>
);
};
export default Counter;
In this example, we use useReducer
to manage the component’s count state. The reducer function defines how the state should change based on dispatched actions. This pattern is particularly useful when working with multiple state values or complex state logic.
Custom Hooks
Custom hooks allow developers to extract component logic into reusable functions. A custom hook is simply a function whose name starts with "use" and can call other hooks.
Example of a Custom Hook
import React, { useState, useEffect } from 'react';
const useFetch = (url) => {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
const fetchData = async () => {
const response = await fetch(url);
const result = await response.json();
setData(result);
setLoading(false);
};
fetchData();
}, [url]);
return { data, loading };
};
const DataDisplay = () => {
const { data, loading } = useFetch('https://api.example.com/data');
if (loading) return <div>Loading...</div>;
return <pre>{JSON.stringify(data, null, 2)}</pre>;
};
export default DataDisplay;
In this example, we implemented a custom hook useFetch
that handles data fetching logic. The hook abstracts the loading state and fetch execution, making it reusable across multiple components.
Conclusion
Understanding the various aspects of component composition, Higher-Order Components, and React hooks can greatly enhance your development experience in React. By embracing these concepts, developers can create more modular, maintainable, and scalable applications that offer a great user experience. Whether it's composing components for better organization, utilizing HOCs for shared logic, or leveraging hooks for state management, each of these features plays a vital role in the modern React ecosystem.
As you continue exploring React, remember that practice and experimentation are essential. The more you dive into these concepts, the more intuitive they will become, allowing you to harness the full power of React in your projects. Happy coding!
Subscribe to my newsletter
Read articles from Ganesh Jaiwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ganesh Jaiwal
Ganesh Jaiwal
Hello! I'm a dedicated software developer with a passion for coding and a belief in technology's impact on our world. My programming journey started a few years ago and has reshaped my career and mindset. I love tackling complex problems and creating efficient code. My skills cover various languages and technologies like JavaScript, Angular, ReactJS, NodeJs, and Go Lang. I stay updated on industry trends and enjoy learning new tools. Outside of coding, I cherish small routines that enhance my workday, like sipping tea, which fuels my creativity and concentration. Whether debugging or brainstorming, it helps me focus. When I'm not coding, I engage with fellow developers. I value teamwork and enjoy mentoring newcomers and sharing my knowledge to help them grow. Additionally, I explore the blend of technology and creativity through projects that incorporate art and data visualization. This keeps my perspective fresh and my passion alive. I'm always seeking new challenges, from open-source contributions to hackathons and exploring AI. Software development is more than a job for me—it's a passion that drives continuous learning and innovation.