Getting Started with API Development Using Bun.js

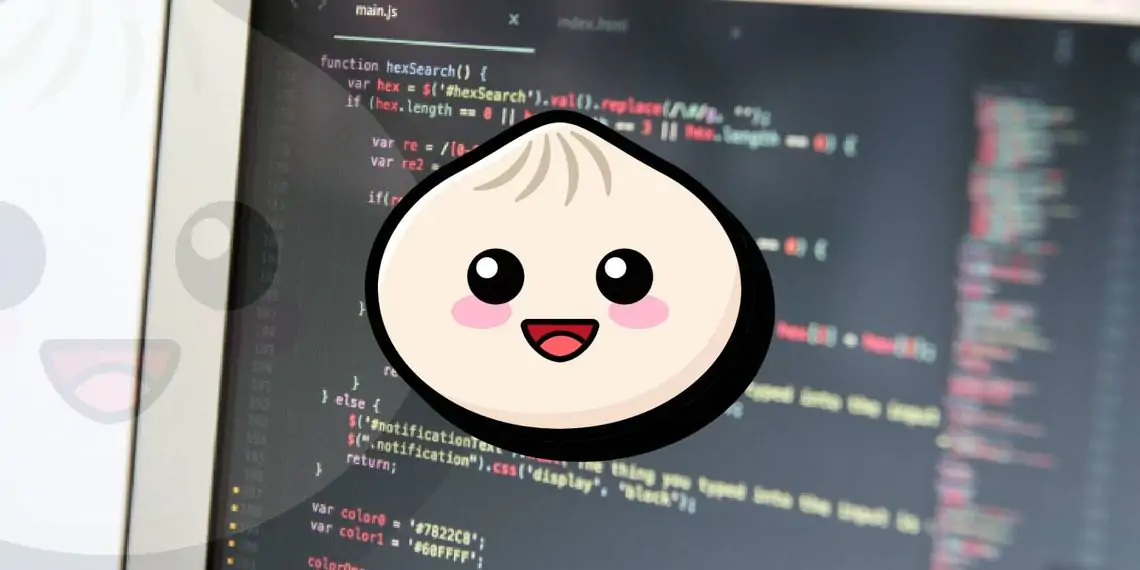
Introduction ๐
Bun.js is a modern JavaScript runtime thatโs designed to be blazing fast and incredibly simple to use. โก Itโs built to provide an alternative to traditional runtimes like Node.js and Deno, but with a focus on speed and ease of use. Bun is optimized for performance and offers a seamless experience when developing applications, especially APIs. ๐
Whether you're building a simple web server or a complex API, Bun helps you get started quickly and scale your application effortlessly. It provides an ultra-fast JavaScript runtime with a minimal footprint, making it a great choice for developers who need to handle high loads and low latency. ๐ป
But that's not all! With Bun, you can also enjoy the benefits of:
Fast startup times ๐โโ๏ธ: No more waiting around for your app to load! Bun gets your project up and running in a flash.
Zero dependencies ๐: Bunโs core is lightweight, and you wonโt need to install extra dependencies just to get started.
Built-in tools ๐ ๏ธ: Bun includes built-in support for bundling, transpiling, and serving static files, reducing the need for additional packages.
Supercharged development experience โก: Bun is designed to run at lightning speed, making it easier for you to build, test, and deploy your applications faster than ever before.
In this blog post, weโll walk you through the process of creating a simple API endpoint with Bun.js. ๐ฑ If you're new to Bun or just want to explore its features, this guide will give you a great starting point and help you harness the power of Bun to build efficient, fast, and scalable APIs. ๐
So, letโs dive into building our very first API with Bun and see how easy it is to get started! ๐๐ป
Bun.js vs. Other Frameworks: A Performance Comparison โก vs ๐ง
1. Performance
Bun.js ๐: Known for its blazing-fast startup times and handling of asynchronous tasks. It outperforms Node.js and Express.js in terms of raw speed, especially for I/O-heavy operations.
Node.js ๐ฅ๏ธ: A widely-used runtime, but it lags behind Bun in certain benchmarks, especially when it comes to request handling and overall throughput.
Express.js โก: As a framework built on top of Node.js, it inherits its performance characteristics, making it suitable for most web applications but slower compared to Bun.js.
Deno ๐: Deno offers a secure runtime with better tooling but tends to be slower in performance compared to Bun.js, especially in API handling.
2. Ease of Use
Bun.js ๐ ๏ธ: Offers a simple and minimalistic API, making it easy for developers to get started quickly.
Node.js/Express.js ๐ง: Well-established, with a massive ecosystem, but it may require more setup and configuration for certain tasks.
Deno ๐: Provides a more secure environment out-of-the-box but requires developers to adapt to its new ecosystem and API.
3. Ecosystem and Tooling
Bun.js ๐ฑ: While Bun is newer, it includes built-in support for hot-reloading, TypeScript, and more. However, the ecosystem is still growing compared to Node.js.
Node.js/Express.js ๐: Offers a rich ecosystem with tons of libraries and tools, making it the go-to for many developers.
Deno ๐: Comes with a more opinionated, secure ecosystem but has less library support than Node.js, making it challenging for larger projects.
4. Use Cases
Bun.js โก: Best for applications where speed is critical and rapid development is necessary, such as real-time applications, microservices, or high-performance APIs.
Node.js/Express.js ๐: Best suited for general-purpose web development and applications with existing libraries.
Deno ๐: Ideal for security-first applications but may not be the top choice for high-performance APIs yet.
Prerequisites
Before diving in, ensure you have the following:
Node.js Installed: Although Bun is independent, youโll need Node.js to manage your environment.
Bun Installed: Follow the official Bun installation guide to get started.
Basic Knowledge of JavaScript: Familiarity with JavaScript and REST APIs will be helpful.
Installation on macOS ๐
Using Homebrew (Recommended):
If you have Homebrew installed, you can easily install Bun with the following command:bashbrew install bun
Using the Official Script:
You can also install Bun using the official installation script. Open your terminal and run:bashcurl -fsSL https://bun.sh/install | bash
Installation on Windows ๐ช
Step 1: Installing Bun
To install Bun, open your terminal and run the following command:
curl -fsSL https://bun.sh/install | bash
This script will download and install Bun. After installation, ensure that Bun is added to your PATH environment variable. You can verify the installation by running:
bun --version
Step 2: Setting Up the Bun Project
First, create a new Bun project. Run the following command:
bun create app my-bun-api
Navigate to your project directory:
cd my-bun-api
Install necessary dependencies if any. Bunโs built-in package manager makes this process super fast.
Step 3: Setting Up a Basic Server
Bun provides a simple way to set up a server using its Bun.serve
method. Letโs create a basic server file.
Create a new file named server.js
in your projectโs root directory:
const server = Bun.serve({
port: 3000,
fetch(req) {
return new Response("Hello, World!", { status: 200 });
},
});
console.log(`Server is running at http://localhost:${server.port}`);
Step 4: Creating an API Endpoint
Letโs enhance the server to include a /api/greet
endpoint that accepts a GET
request.
Update your server.js
file:
const server = Bun.serve({
port: 3000,
fetch(req) {
const url = new URL(req.url);
if (url.pathname === "/api/greet" && req.method === "GET") {
const name = url.searchParams.get("name") || "Guest";
return new Response(JSON.stringify({ message: `Hello, ${name}!` }), {
status: 200,
headers: {
"Content-Type": "application/json",
},
});
}
return new Response("Not Found", { status: 404 });
},
});
console.log(`Server is running at http://localhost:${server.port}`);
Step 5: Testing the API
Start the server:
bun run server.js
Open your browser or use a tool like curl
or Postman to test the endpoint.
Example Request:
curl "http://localhost:3000/api/greet?name=Harsh"
Response:
{
"message": "Hello, Harsh!"
}
If you omit the name
parameter, it will default to "Guest."
Step 6: Structuring the Project
For a more scalable API, structure your project into different folders:
my-bun-api/
|-- controllers/
| |-- greetController.js
|-- server.js
Move the endpoint logic to controllers/greetController.js
:
export function greetController(req, url) {
const name = url.searchParams.get("name") || "Guest";
return new Response(JSON.stringify({ message: `Hello, ${name}!` }), {
status: 200,
headers: {
"Content-Type": "application/json",
},
});
}
Update server.js
:
import { greetController } from "./controllers/greetController.js";
const server = Bun.serve({
port: 3000,
fetch(req) {
const url = new URL(req.url);
if (url.pathname === "/api/greet" && req.method === "GET") {
return greetController(req, url);
}
return new Response("Not Found", { status: 404 });
},
});
console.log(`Server is running at http://localhost:${server.port}`);
Conclusion
Congratulations! Youโve created your first API endpoint using Bun.js. This lightweight runtime makes API development quick and efficient. From here, you can expand your application by adding more routes, integrating a database, or deploying it to a cloud platform.
Feel free to share your experience or improvements. Happy coding with Bun!
Subscribe to my newsletter
Read articles from Harsh Choudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
