Deep Learning with Keras and Tensorflow
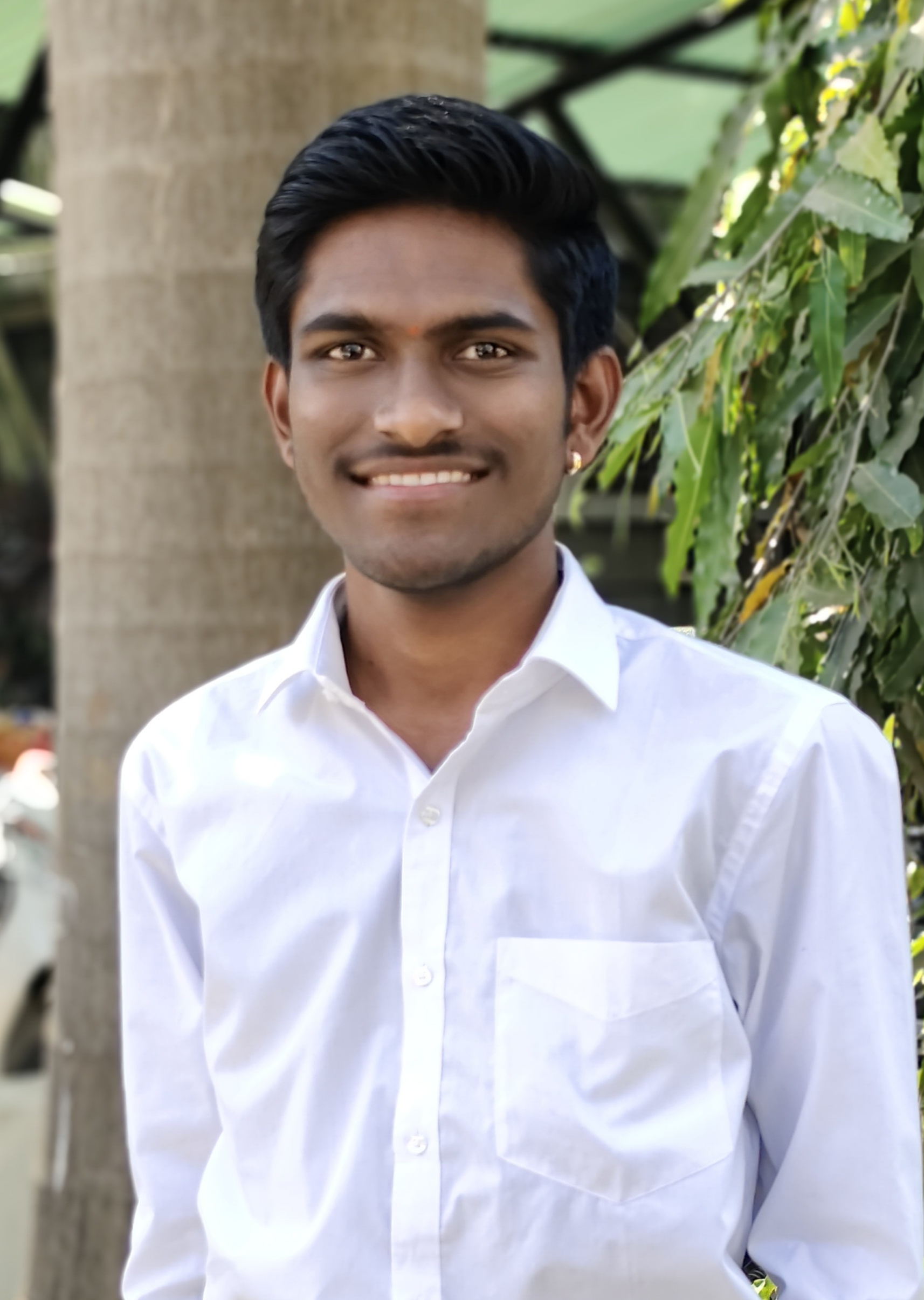
Table of contents

Data augmentation is a key technique in machine learning to enhance dataset size and diversity by applying transformations like rotation and noise addition, improving model generalization and performance. In Keras, ImageDataGenerator facilitates real-time data augmentation.
Transfer learning, particularly using architectures like VGG16, leverages pre-trained models to enhance performance and efficiency, especially with limited data.
Transpose convolution, crucial in tasks like image generation, increases spatial dimensions and is implemented using Keras's Conv2DTranspose.
Data Augmentation
Data augmentation is a technique used in machine learning and deep learning to artificially expand the size of a training dataset by creating modified versions of existing data. This is done by applying various transformations to the original data, such as:
Rotations: Rotating images by a certain angle.
Translations: Shifting images horizontally or vertically.
Flipping: Flipping images horizontally or vertically.
Scaling: Resizing images.
Adding noise: Introducing random noise to images.
Importance of Data Augmentation:
Improves Model Generalization: By introducing variations in the training data, models learn to recognize patterns more robustly, which helps them perform better on unseen data.
Prevents Overfitting: Augmentation helps reduce overfitting by providing more diverse training examples, making it harder for the model to memorize the training data.
Enhances Performance: Models trained with augmented data often achieve better accuracy and performance metrics.
Utilizes Limited Data: In scenarios where collecting more data is expensive or time-consuming, data augmentation allows for effective use of the existing dataset.
Data Augmentation in Keras:
You can implement data augmentation in a Keras model using the ImageDataGenerator
class. This class allows you to apply various transformations to your images in real-time during training.
Import Required Libraries:
from keras.preprocessing.image import ImageDataGenerator
Initialize the ImageDataGenerator: You can specify the augmentation techniques you want to apply. For example:
datagen = ImageDataGenerator( rotation_range=40, # Randomly rotate images in the range (degrees) width_shift_range=0.2, # Randomly shift images horizontally height_shift_range=0.2, # Randomly shift images vertically shear_range=0.2, # Shear angle in counter-clockwise direction zoom_range=0.2, # Randomly zoom into images horizontal_flip=True, # Randomly flip images fill_mode='nearest' # Fill pixels that are newly created )
Load Your Image: Load an image that you want to augment:
from keras.preprocessing import image import numpy as np img = image.load_img('sample.jpg') # Load your image x = image.img_to_array(img) # Convert image to array x = np.expand_dims(x, axis=0) # Reshape to include batch dimension
Generate Augmented Images: Use the
flow
method to generate batches of augmented images:for batch in datagen.flow(x, batch_size=1): # Display or save the augmented images break # Use break to stop after generating one batch
Integrate with Model Training: You can use the
fit
method of your model to train it with augmented data:model.fit(datagen.flow(training_images, training_labels, batch_size=32), steps_per_epoch=len(training_images) / 32, epochs=50)
Advanced data augmentation techniques in Keras
Advanced data augmentation techniques in Keras can include methods like feature-wise normalization, sample-wise normalization, and custom augmentation functions.
1. Feature-wise Normalization
This technique normalizes the dataset to have a mean of 0 and a standard deviation of 1 across all images.
from keras.preprocessing.image import ImageDataGenerator
# Initialize ImageDataGenerator with feature-wise normalization
datagen = ImageDataGenerator(
featurewise_center=True, # Set mean to 0
featurewise_std_normalization=True # Set std deviation to 1
)
# Fit the generator on the training images
datagen.fit(training_images) # training_images is your dataset
Advantages:
Standardization Across the Dataset: This method normalizes the dataset to have a mean of 0 and a standard deviation of 1 across all features (e.g., pixel values in images). This helps in stabilizing the learning process and can lead to faster convergence during training.
Improved Model Performance: By ensuring that all features contribute equally to the model's learning, feature-wise normalization can enhance the model's performance, especially in cases where features have different scales.
2. Sample-wise Normalization
This technique normalizes each sample (image) individually.
# Initialize ImageDataGenerator with sample-wise normalization
datagen = ImageDataGenerator(
samplewise_center=True, # Set mean of each sample to 0
samplewise_std_normalization=True # Set std deviation of each sample to 1
)
# Fit the generator on the training images
datagen.fit(training_images)
Advantages:
Individual Sample Standardization: Sample-wise normalization normalizes each individual sample (image) to have a mean of 0 and a standard deviation of 1. This is particularly useful when the dataset contains images with varying lighting conditions or contrasts.
Enhanced Robustness: By normalizing each sample, the model becomes more robust to variations in individual images, which can improve its ability to generalize to new, unseen data.
3. Custom Augmentation Function
You can define your own augmentation functions to apply specific transformations, such as adding random noise to images.
import numpy as np
# Define a custom augmentation function
def add_random_noise(image):
noise = np.random.normal(loc=0.0, scale=0.1, size=image.shape) # Generate random noise
return np.clip(image + noise, 0, 255) # Add noise and clip values to valid range
# Initialize ImageDataGenerator with the custom function
datagen = ImageDataGenerator(
preprocessing_function=add_random_noise # Use the custom function
)
# Fit the generator on the training images
datagen.fit(training_images)
Advantages:
Tailored Transformations: Custom augmentation functions allow you to apply specific transformations that are particularly relevant to your dataset or problem domain. For example, adding random noise can help the model learn to ignore irrelevant variations.
Increased Flexibility: You can design custom functions to address specific challenges in your data, such as simulating real-world conditions or augmenting data in a way that aligns with the model's intended application.
Enhanced Data Diversity: Custom functions can introduce unique variations that may not be covered by standard augmentation techniques, further increasing the diversity of the training data.
Using the Augmented Data
You can use the augmented data in your model training as follows:
model.fit(datagen.flow(training_images, training_labels, batch_size=32),
steps_per_epoch=len(training_images) / 32, epochs=50)
Transfer Learning using VGG16
Transfer Learning / Fine Tunning
This technique allows you to leverage pre-trained models to improve performance and reduce training time, especially when working with limited data.
Transfer learning is a machine learning technique where a model developed for a specific task is reused as the starting point for a model on a second task
Transfer learning reuses knowledge from models trained on large datasets (like ImageNet) for new, related tasks.
For example, if a model is trained to recognize objects in images, it can be fine-tuned to identify specific types of objects with less data and time.
Benefits:
Reduced Training Time: Models start with pre-learned features, speeding up convergence.
Improved Performance: Pre-trained models are optimized on large datasets, enhancing accuracy.
Efficiency with Limited Data: Achieve high accuracy even with smaller datasets.
VGG16 Architecture
VGG16 is a convolutional neural network architecture that was developed by the Visual Geometry Group (VGG) at the University of Oxford.
Architecture:
VGG16 consists of 16 layers with learnable weights, including: 13 convolutional layers, 3 fully connected layers
Input Size: It takes input images of size 224x224 pixels with three color channels (RGB).
Depth: The model is known for its depth, which allows it to learn complex features from images.
Pre-trained Model: VGG16 is often used as a pre-trained model on large datasets like ImageNet, where it has learned to identify various features such as edges, textures, and shapes.
Applications: VGG16 is widely used in image classification tasks and can be fine-tuned for specific applications, making it a popular choice in transfer learning.
Implementation of Transfer Learning with Keras
To implement transfer learning with Keras, you can follow these general steps using a pre-trained model like VGG16.
Import Required Libraries:
from tensorflow.keras.applications import VGG16 from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense, Flatten from tensorflow.keras.preprocessing.image import ImageDataGenerator
Load the Pre-trained Model:
base_model = VGG16(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
Freeze the Base Model Layers:
for layer in base_model.layers: layer.trainable = False
Create a New Model:
model = Sequential() model.add(base_model) model.add(Flatten()) model.add(Dense(256, activation='relu')) model.add(Dense(1, activation='sigmoid')) # For binary classification
model = Sequential():
This line creates a new Sequential model in Keras. A Sequential model is a linear stack of layers, meaning you can add layers one after another.
model.add(base_model):
Here, you are adding a pretrained model (like VGG16 or ResNet) to your Sequential model. This base model will act as a feature extractor, processing the input data and extracting useful features.
model.add(Flatten()):
The Flatten layer converts the multi-dimensional output from the base model into a one-dimensional array. This is necessary because the next layer (Dense) expects a flat input.
model.add(Dense(256, activation='relu')):
This line adds a Dense layer with 256 neurons and uses the ReLU (Rectified Linear Unit) activation function. The Dense layer is a fully connected layer, meaning each neuron is connected to every neuron in the previous layer. ReLU helps introduce non-linearity, allowing the model to learn complex patterns.
model.add(Dense(1, activation='sigmoid')):
Finally, this adds another Dense layer with a single neuron and a sigmoid activation function. This layer is used for binary classification, where the output will be a value between 0 and 1, representing the probability of one of the two classes.
Compile the Model:
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
Prepare the Data:
train_datagen = ImageDataGenerator(rescale=1./255) train_generator = train_datagen.flow_from_directory( 'path_to_training_data', target_size=(224, 224), batch_size=32, class_mode='binary' )
organize your dataset in a directory structure where each class has its own subdirectory. For example:
training_data/ ├── cats/ └── dogs/
Train the Model:
model.fit(train_generator, epochs=10)
Fine-tuning (Optional): Unfreeze some layers of the base model and recompile.
for layer in base_model.layers[-4:]: layer.trainable = True model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) model.fit(train_generator, epochs=10)
This process allows you to leverage the pre-trained model's features while adapting it to your specific task.
Tips for Transfer Learning Implementation
Transfer learning is a powerful technique that enables the use of pre-trained models on new tasks, significantly saving time and computational resources. Here are key tips for implementing transfer learning effectively:
Choose the right pre-trained model: Select a model trained on a dataset similar to your target task to enhance performance. Popular models like VGG16, ResNet, or InceptionV3 are particularly effective for image-related tasks. Ensure that the architecture aligns with your specific problem requirements.
Freeze early layers: In the initial training stages, freeze the early layers of the pre-trained model to preserve their learned features. This approach is beneficial when working with small datasets or datasets that closely resemble the original dataset the model was trained on.
Fine-tune later layers: As training progresses, gradually unfreeze the deeper layers and fine-tune them. These layers capture task-specific features, and fine-tuning allows the model to adapt better to the nuances of your new dataset.
Adjust learning rates: Use a lower learning rate for fine-tuning to prevent catastrophic forgetting of the pre-trained knowledge. High learning rates during this phase can disrupt the learned features and degrade model performance.
Use data augmentation: Implement data augmentation techniques, particularly for image tasks, to increase variability within the dataset. This practice helps prevent overfitting and enhances the model's ability to generalize.
Consider domain adaptation: If there is a significant disparity between the domain of the pre-trained model and your target task, consider applying domain adaptation techniques. These methods can help align the source and target datasets, improving the model's performance.
Transpose Convolution
Transpose convolution is a technique used in deep learning, particularly in image processing, to increase the spatial dimensions of an input feature map.
What is Transpose Convolution?
Inverse Operation: Transpose convolution is often referred to as the inverse of standard convolution (Deconvolution). While standard convolution reduces the spatial dimensions of an input (e.g., an image), transpose convolution increases them.
Zero Insertion: It works by inserting zeros between the elements of the input feature map. This process is followed by applying a convolution operation, which results in an up-sampled output.
Output: The output retains the characteristics of the original input but at a higher resolution.
Need for Transpose Convolution
Up-sampling: In tasks where you need to generate higher-resolution images from lower-resolution inputs (e.g., image generation, super-resolution), transpose convolution is essential.
Image Generation: In Generative Adversarial Networks (GANs), transpose convolution helps in generating images from latent vectors.
Semantic Segmentation: It is used to produce pixel-wise classification maps, allowing for detailed segmentation of images.
Feature Map Expansion: It allows for the expansion of feature maps in neural networks, which is crucial for tasks that require detailed spatial information.
Implementing Transpose Convolution with Keras
To implement transpose convolution in Keras, you can use the Conv2DTranspose
layer.
Import Libraries:
from keras.models import Sequential from keras.layers import Conv2DTranspose, InputLayer
Create the Model:
model = Sequential() model.add(InputLayer(input_shape=(height, width, channels))) # Specify input shape model.add(Conv2DTranspose(filters=32, kernel_size=(3, 3), strides=(2, 2), activation='relu')) model.add(Conv2DTranspose(filters=1, kernel_size=(3, 3), activation='sigmoid')) # Output layer
Compile the Model:
model.compile(optimizer='adam', loss='mean_squared_error')
Explanation of Parameters:
filters: The number of output filters in the convolution.
kernel_size: The height and width of the 2D convolution window.
strides: The strides of the convolution along the height and width.
activation: The activation function to use (e.g., 'relu' for hidden layers, 'sigmoid' for output).
potential issues of transpose convolution and ways to mitigate them:
Potential Issues:
Checkerboard Artifacts: These artifacts appear as unwanted patterns in the output images, often due to uneven overlapping of the convolution kernels during the up-sampling process.
Loss of Spatial Information: Transpose convolution can sometimes lead to a loss of fine details in the up-sampled images, affecting the overall quality.
Mitigation Strategies:
Use of Additional Techniques:
Bilinear Up-sampling: Before applying the transpose convolution, you can use bilinear up-sampling to increase the spatial dimensions. This helps in reducing artifacts.
Regular Convolution Layer: After the transpose convolution, applying a standard convolution layer can refine the output and help recover lost details.
Careful Kernel Design: Choose kernel sizes and strides that minimize the risk of artifacts. Experimenting with different configurations can help find the best setup for your specific application.
Batch Normalization: Incorporating batch normalization layers can stabilize the learning process and improve the quality of the output.
Skip Connections: In architectures like U-Net, using skip connections can help retain spatial information from earlier layers, improving the overall output quality.
Subscribe to my newsletter
Read articles from Omkar Kasture directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
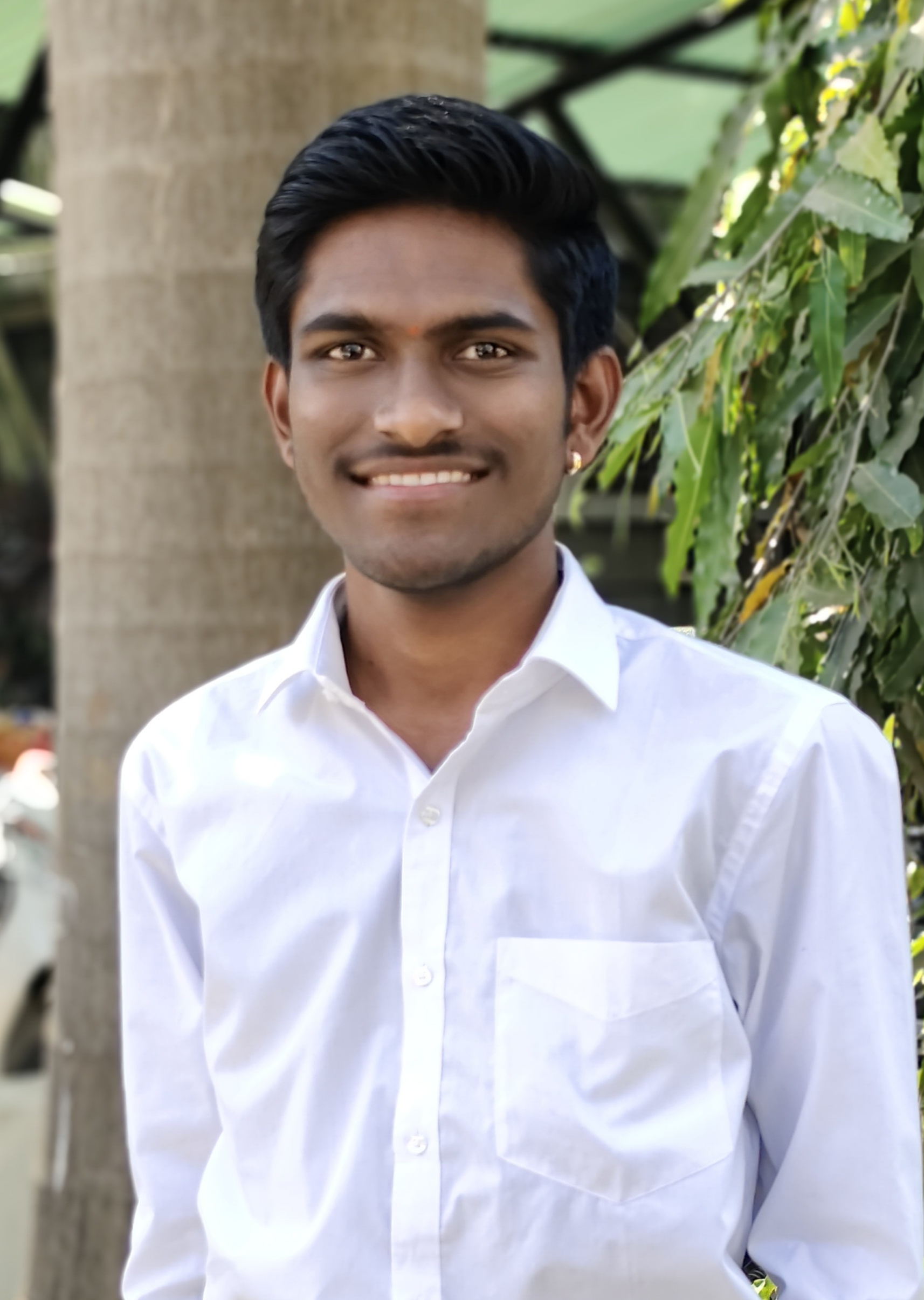
Omkar Kasture
Omkar Kasture
MERN Stack Developer, Machine learning & Deep Learning