π Chapter 17: Mastering Higher-Order Functions (HOF) & Callbacks in JavaScript for Full Stack Development
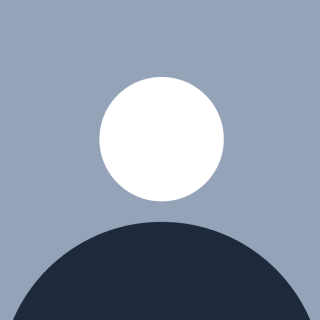
Table of contents
- Introduction: Mastering Higher-Order Functions (HOFs) and Callbacks in JavaScript
- How Mastering These Concepts Empowers Developers for Scalable and Maintainable Code
- Key Objectives of This Chapter
- Section 1: What are Higher-Order Functions and Callbacks?
- Section 2: Understanding forEach and Other Array Iteration Methods
- Section 3: Core Higher-Order Functions Explained
- 1. map()
- 2. filter()
- 3. reduce()
- Section 4: Destructuring in JavaScript
- 1. Array Destructuring
- 2. Object Destructuring
- 1. To-Do List with Callbacks: Manage To-Do Tasks using HOFs
- 2. Student Marks Calculator: Use reduce to Compute Total and Average Marks
- 3. Weather Dashboard: Filter and Map Weather Data Dynamically
- 4. String Transformation Tool: Apply map for String Formatting
- 5. Expense Tracker App: Use HOFs to Analyze Expenses
- Section 6: Mini Project - Online Shopping Cart System
- Project Features:
- Project Breakdown:
- Key Code Snippets:
- Additional Features to Enhance the Project:
- Conclusion:
- Interview Questions on Higher-Order Functions (HOFs)
- Conclusion:
- Other Series:

Introduction: Mastering Higher-Order Functions (HOFs) and Callbacks in JavaScript
In the ever-evolving landscape of web development, writing clean, efficient, and maintainable code is a skill every developer must master. One of the most powerful paradigms that aid in achieving this goal is the use of Higher-Order Functions (HOFs) and Callbacks in JavaScript. These concepts not only enhance code readability but also open up new avenues for handling asynchronous operations, data transformations, and event-driven programming.
Importance of Higher-Order Functions (HOF) in Modern Web Development
Higher-Order Functions are foundational to many programming paradigms, especially functional programming, which emphasizes immutability and first-class functions. They simplify complex operations by breaking them down into smaller, reusable functions. In modern web development, where efficiency and scalability are critical, HOFs help developers:
Abstract complex logic: Instead of writing repetitive code, HOFs allow us to encapsulate logic into reusable functions.
Handle asynchronous operations: Functions like
setTimeout
,setInterval
, andPromise
callbacks rely heavily on the HOF and callback structure.Efficient data processing: Methods like
map()
,filter()
, andreduce()
help in efficiently transforming and filtering data without mutating the original arrays.
Real-World Use Cases and Scenarios Where Callbacks and HOFs Excel
Event Handling: Listening to user actions such as button clicks or form submissions requires callbacks.
API Requests: Fetching data from external APIs often requires handling asynchronous responses using callbacks or promises.
Data Manipulation: Large-scale applications often require efficient transformations and filtering of datasets, which is made simple by using HOFs.
Animation Control: Callbacks are crucial in animation libraries for scheduling tasks and sequencing transitions.
By embracing these paradigms, developers can write code that is not only elegant but also optimized for performance and maintenance.
How Mastering These Concepts Empowers Developers for Scalable and Maintainable Code
When developers understand how to effectively use HOFs and callbacks, their ability to handle real-world challenges improves significantly. Some key benefits include:
Improved Modularity: Functions can be composed together, making it easier to maintain and update code.
Code Reusability: Higher-order functions encourage a design pattern where smaller, focused functions can be reused in various contexts.
Better Error Handling: Structured callbacks help isolate and handle errors gracefully without affecting the main code flow.
Scalability: With cleaner and more abstract code using HOFs, scaling the application becomes less cumbersome and error-prone.
Key Objectives of This Chapter
In this chapter, we aim to explore Higher-Order Functions and Callbacks in-depth to help developers gain a solid understanding of these critical concepts. The key objectives include:
Understand what HOFs and Callbacks are:
Grasp the definitions, characteristics, and the importance of using these functions in JavaScript.Explore various built-in HOFs (
map()
,filter()
,reduce()
, etc.):
Analyze how these functions work and when to use each in real-world scenarios.Learn best practices and potential pitfalls:
Avoid common mistakes while adopting HOFs and callbacks, and follow best practices to write clean, maintainable code.Hands-on coding examples and projects:
Apply the knowledge gained through interactive coding examples, small projects on each concept, and a comprehensive mini-project to solidify your understanding.
By the end of this chapter, you will be well-equipped to use HOFs and callbacks effectively in your web development projects, paving the way for cleaner, scalable, and maintainable code.
Section 1: What are Higher-Order Functions and Callbacks?
Definition:
A Higher-Order Function (HOF) is a function that either:
Accepts other functions as arguments (callbacks)
Returns a function as its result
This ability makes HOFs a powerful tool in functional programming, enabling developers to write cleaner and more abstract code.
Callback Functions:
A callback function is a function passed as an argument to another function and is called after the completion of that function. The callback pattern is a key feature of asynchronous programming in JavaScript.
Key Points:
The callback function is invoked by the higher-order function at a specific point during execution.
Callbacks are widely used in event handling, API requests, and data processing in JavaScript.
Why Use Higher-Order Functions?
Code Abstraction: HOFs encapsulate repetitive logic, improving code reusability.
Cleaner Code: Callbacks help maintain logical separation, keeping code DRY (Don't Repeat Yourself).
Asynchronous Programming: Functions like
setTimeout()
andsetInterval()
leverage callbacks to perform non-blocking operations.Functional Paradigm: They enable developers to use declarative coding instead of imperative, focusing on what to do rather than how to do it.
Example 1: Cube Calculation using Callback Function
const callback = (n) => {
return n ** 2; // Square of a number
}
function cube(callback, n) {
return callback(n) * n; // Square * n
}
console.log(cube(callback, 2)); // Output: 8
Explanation:
callback(n)
computes the square ofn
.The result of the callback is multiplied by
n
inside thecube
function.The function
cube()
is a Higher-Order Function because it accepts a function (callback
) as an argument.
Example 2: Using setTimeout with a Callback
setTimeout(() => {
console.log("Hello, Rohit!");
}, 2000);
Explanation:
setTimeout()
is a higher-order function that takes a callback function as its first argument and a delay time (in milliseconds) as its second argument.The callback is executed after 2 seconds, printing "Hello, Rohit!" to the console.
Key Real-World Use Case:
Event Listeners in the Browser:
const button = document.querySelector('button');
button.addEventListener('click', () => {
console.log('Button Clicked!');
});
Here, addEventListener()
is a higher-order function, and the arrow function is the callback executed when the button is clicked.
Section 2: Understanding forEach and Other Array Iteration Methods
forEach: Iterates Over Each Element of an Array
The forEach()
method executes a provided function once for each array element.
Example: Using forEach with a Callback
const arr = ["Rohit", "Shikhar", "Virat", "Shreyas", "Hardik"];
arr.forEach((val) => {
console.log(val);
});
Explanation:
The
forEach()
method iterates through each element in the array.For every element, the callback function logs the value to the console.
Comparison Table: forEach vs map
Method | Purpose | Return Value | Mutates Array? |
forEach() | Iteration | undefined | No |
map() | Transformation | New Array | No |
Example 1: map() vs forEach()
const numbers = [1, 2, 3, 4, 5];
// Using forEach
const result1 = [];
numbers.forEach((num) => result1.push(num * 2));
console.log(result1); // [2, 4, 6, 8, 10]
// Using map
const result2 = numbers.map((num) => num * 2);
console.log(result2); // [2, 4, 6, 8, 10]
Key Difference:
forEach()
does not return a new array; we must manually push results to an array.map()
automatically creates and returns a new array without additional logic.
forEach Use Case: Displaying Elements Dynamically
const users = ["Rohit", "Shikhar", "Virat", "Shreyas"];
let userList = "";
users.forEach((user) => {
userList += `<li>${user}</li>`;
});
console.log(userList);
Section 3: Core Higher-Order Functions Explained
Higher-order functions are essential tools in functional programming. They simplify operations on arrays, improve code readability, and allow developers to write clean and concise logic. Let's break down three essential higher-order functions in JavaScript: map()
, filter()
, and reduce()
.
1. map()
Description:
map()
is used to create a new array by applying a given callback function to each element of the original array.It does not modify the original array.
Syntax:
const newArray = array.map(callbackFunction(currentValue, index, array))
Parameter | Description |
currentValue | The current element being processed in the array |
index (optional) | The index of the current element |
array (optional) | The original array being processed |
Example 1: Basic Usage
const num = [1, 2, 3, 4, 5, 6];
const numSq = num.map((n) => n * n);
console.log(numSq); // [1, 4, 9, 16, 25, 36]
Explanation:
The callback function
(n) => n * n
squares each element of thenum
array.The result is a new array
[1, 4, 9, 16, 25, 36]
.
Example 2: Converting Temperatures
const fahrenheitTemps = [32, 45, 50, 72, 85];
const celsiusTemps = fahrenheitTemps.map((f) => ((f - 32) * 5) / 9);
console.log(celsiusTemps); // [0, 7.22, 10, 22.22, 29.44]
Use Case:
map()
is useful for transforming arrays, such as formatting strings, converting units, or applying mathematical operations.
2. filter()
Description:
filter()
creates a new array containing elements that satisfy a given condition defined in a callback function.It does not modify the original array.
Syntax:
const newArray = array.filter(callbackFunction(currentValue, index, array))
Parameter | Description |
currentValue | The current element being processed in the array |
index (optional) | The index of the current element |
array (optional) | The original array being processed |
Example 1: Filtering Strings with a Condition
const countries = ["India", "England", "Ireland", "Finland"];
const store = countries.filter((val) => val.includes("land"));
console.log(store); // ["England", "Ireland", "Finland"]
Explanation:
The callback function
(val) => val.includes("land")
checks whether each country name contains the substring"land"
.The result is a filtered array
["England", "Ireland", "Finland"]
.
Example 2: Filtering Even Numbers
const numbers = [1, 2, 3, 4, 5, 6];
const evenNumbers = numbers.filter((num) => num % 2 === 0);
console.log(evenNumbers); // [2, 4, 6]
Use Case:
filter()
is excellent for selecting specific elements based on conditions, such as finding even numbers, filtering user inputs, or searching for specific items in an array.
3. reduce()
Description:
reduce()
applies a function to an accumulator and each element of an array, reducing the array to a single value.It is often used for summation, aggregation, or concatenation tasks.
Syntax:
const result = array.reduce(callbackFunction(accumulator, currentValue, index, array), initialValue)
Parameter | Description |
accumulator | Accumulates the result of the callback function |
currentValue | The current element being processed |
index (optional) | The index of the current element |
array (optional) | The original array being processed |
initialValue (optional) | Initial value for the accumulator |
Example 1: Sum of Array Elements
const num = [1, 2, 3, 4, 5, 6];
const sum = num.reduce((acc, curr) => acc + curr, 0);
console.log(sum); // 21
Explanation:
The callback function
(acc, curr) => acc + curr
adds the current element (curr
) to the accumulator (acc
).The initial value is set to
0
, and the final result is21
.
Example 2: Finding the Largest Number
const numbers = [10, 25, 7, 50, 3];
const largest = numbers.reduce((acc, curr) => (curr > acc ? curr : acc), 0);
console.log(largest); // 50
Use Case:
reduce()
is powerful for aggregation tasks like summing orders in an e-commerce cart, calculating averages, or finding the highest value.
Comparison Table: map()
, filter()
, and reduce()
Method | Purpose | Return Value | Mutates Array? |
map() | Transforms elements | New array | No |
filter() | Selects elements by condition | New array | No |
reduce() | Aggregates values | Single value | No |
Section 4: Destructuring in JavaScript
Destructuring allows us to extract values from arrays or properties from objects and assign them to variables. This technique simplifies code and makes it more readable.
1. Array Destructuring
Array destructuring enables extracting elements from an array and assigning them to individual variables in a single statement.
Example: Destructuring an array
const sci = [2.72, 3.14, 9.81, 37, 100];
let [e, pi, gravity, bodyTemp, boilTemp] = sci;
console.log(e, pi, gravity, bodyTemp, boilTemp);
Output:
2.72 3.14 9.81 37 100
Explanation:
The elements of the array
sci
are assigned to variablese
,pi
,gravity
,bodyTemp
, andboilTemp
.The left-hand side pattern
[e, pi, gravity, bodyTemp, boilTemp]
must match the structure of the array.
Skipping Array Elements
const arr = [1, 2, 3, 4, 5];
let [first, , third] = arr;
console.log(first, third); // Output: 1 3
- The second element is skipped by placing a comma without a variable.
2. Object Destructuring
Object destructuring allows us to extract properties from an object and assign them to variables with matching names.
Example: Destructuring an object
const person = { name: "Rohit", age: 25 };
let { name, age } = person;
console.log(name, age);
Output:
Rohit 25
Explanation:
- The properties
name
andage
from the objectperson
are assigned to variables with the same names.
Renaming Variables During Destructuring
const person = { name: "Rohit", age: 25 };
let { name: fullName, age: personAge } = person;
console.log(fullName, personAge); // Output: Rohit 25
name: fullName
renamesname
tofullName
, andage: personAge
does the same forage
.
Default Values
const student = { name: "Shikhar" };
let { name, age = 20 } = student;
console.log(name, age); // Output: Shikhar 20
- Default values are assigned when a property is missing in the object.
1. To-Do List with Callbacks: Manage To-Do Tasks using HOFs
Objective: Build a simple to-do list application where tasks can be added, marked as completed, or removed using HOFs like forEach
, filter
, and map
.
Key Features:
Add tasks
Mark tasks as completed
View all completed tasks
Remove specific tasks
Implementation:
class ToDoList {
constructor() {
this.tasks = [];
}
// Add a task
addTask(task) {
this.tasks.push({ task, completed: false });
}
// Mark a task as completed
completeTask(taskName) {
this.tasks = this.tasks.map(task =>
task.task === taskName ? { ...task, completed: true } : task
);
}
// View completed tasks
getCompletedTasks() {
return this.tasks.filter(task => task.completed);
}
// Remove a task
removeTask(taskName) {
this.tasks = this.tasks.filter(task => task.task !== taskName);
}
// Display tasks
displayTasks() {
this.tasks.forEach(task => {
console.log(`${task.task} - ${task.completed ? "Completed" : "Pending"}`);
});
}
}
const myToDo = new ToDoList();
myToDo.addTask("Complete JavaScript project");
myToDo.addTask("Write blog post");
myToDo.completeTask("Complete JavaScript project");
myToDo.displayTasks();
console.log("Completed Tasks:", myToDo.getCompletedTasks());
2. Student Marks Calculator: Use reduce to Compute Total and Average Marks
Objective: Create a system to manage student marks and compute total and average scores using reduce
.
Key Features:
Add student scores
Compute total and average marks
Handle empty score lists
Implementation:
const studentScores = [85, 90, 78, 92, 88];
// Compute total using reduce
const totalScore = studentScores.reduce((acc, score) => acc + score, 0);
console.log(`Total Score: ${totalScore}`);
// Compute average
const averageScore = totalScore / studentScores.length;
console.log(`Average Score: ${averageScore.toFixed(2)}`);
3. Weather Dashboard: Filter and Map Weather Data Dynamically
Objective: Build a weather dashboard that processes weather data and displays information dynamically using filter
and map
.
Key Features:
Filter cities with temperatures above a threshold
Display city names and their corresponding weather conditions
Sample Data:
const weatherData = [
{ city: "Mumbai", temperature: 35, condition: "Sunny" },
{ city: "Delhi", temperature: 40, condition: "Hot" },
{ city: "Bangalore", temperature: 28, condition: "Cloudy" },
{ city: "Shimla", temperature: 15, condition: "Cool" }
];
// Filter cities with temperature above 30 degrees
const hotCities = weatherData.filter(data => data.temperature > 30);
console.log("Hot Cities:", hotCities.map(data => data.city));
// Display weather dashboard
weatherData.map(data => {
console.log(`${data.city}: ${data.temperature}Β°C, ${data.condition}`);
});
4. String Transformation Tool: Apply map for String Formatting
Objective: Create a tool that transforms strings by applying various operations like capitalization, appending characters, or reversing strings using map
.
Key Features:
Capitalize all strings
Append a suffix to each string
Reverse each string
Implementation:
const strings = ["hello", "world", "rohit", "javascript"];
// Capitalize all strings
const capitalizedStrings = strings.map(str => str.toUpperCase());
console.log("Capitalized Strings:", capitalizedStrings);
// Append a suffix to each string
const suffixedStrings = strings.map(str => `${str}_suffix`);
console.log("Suffixed Strings:", suffixedStrings);
// Reverse each string
const reversedStrings = strings.map(str => str.split("").reverse().join(""));
console.log("Reversed Strings:", reversedStrings);
5. Expense Tracker App: Use HOFs to Analyze Expenses
Objective: Build an expense tracker app that analyzes expenses and categorizes them using HOFs like map
, filter
, and reduce
.
Key Features:
Add expenses with categories
Compute total expenses
Filter expenses by category
Display summarized data
Implementation:
const expenses = [
{ category: "Food", amount: 150 },
{ category: "Transport", amount: 50 },
{ category: "Entertainment", amount: 200 },
{ category: "Groceries", amount: 300 },
{ category: "Food", amount: 100 }
];
// Compute total expenses
const totalExpenses = expenses.reduce((acc, expense) => acc + expense.amount, 0);
console.log(`Total Expenses: $${totalExpenses}`);
// Filter expenses by category
const foodExpenses = expenses.filter(expense => expense.category === "Food");
console.log("Food Expenses:", foodExpenses);
// Summarize expenses by category
const categorySummary = expenses.reduce((acc, expense) => {
acc[expense.category] = (acc[expense.category] || 0) + expense.amount;
return acc;
}, {});
console.log("Category-wise Summary:", categorySummary);
These expanded examples provide a practical understanding of Higher-Order Functions and Callbacks.
Section 6: Mini Project - Online Shopping Cart System
Objective:
Create an interactive online shopping cart system that allows users to add items to the cart, calculate the total price using reduce()
, and filter available items based on user preferences using filter()
.
Project Features:
Add Items to the Cart: Allow users to select products to add to their cart.
Calculate Total Prices: Use the
reduce()
function to compute the total price of all cart items dynamically.Filter Available Items: Use
filter()
to display items based on price range or categories.
Project Breakdown:
1. Cart Item Addition
We maintain an array of objects where each object represents an item with its name and price. Users can dynamically add products to the cart.
2. Calculate Total Prices Using reduce()
The reduce()
function is used to compute the total cost of all items in the cart efficiently.
3. Filter Items Based on Conditions Using filter()
Users can filter items by specific criteria, such as price range or categories.
Key Code Snippets:
Initialize Cart Items
const availableItems = [
{ name: "Phone", price: 500 },
{ name: "Laptop", price: 1000 },
{ name: "Headphones", price: 100 },
{ name: "Smart Watch", price: 200 },
{ name: "Tablet", price: 400 }
];
let cartItems = [];
Function to Add Items to the Cart
function addItemToCart(itemName) {
const item = availableItems.find(product => product.name === itemName);
if (item) {
cartItems.push(item);
console.log(`Added ${itemName} to the cart.`);
} else {
console.log(`${itemName} is not available.`);
}
}
addItemToCart("Phone");
addItemToCart("Smart Watch");
console.log("Cart Items:", cartItems);
Calculate Total Price Using reduce()
const total = cartItems.reduce((acc, item) => acc + item.price, 0);
console.log(`Total Cart Price: $${total}`);
Filter Items Based on Price Range Using filter()
function filterItemsByPrice(maxPrice) {
return availableItems.filter(item => item.price <= maxPrice);
}
const affordableItems = filterItemsByPrice(500);
console.log("Items under $500:", affordableItems);
Complete Example with User Interaction
class ShoppingCart {
constructor() {
this.cart = [];
}
// Add item to the cart
addItem(itemName) {
const item = availableItems.find(product => product.name === itemName);
if (item) {
this.cart.push(item);
console.log(`Added ${itemName} to the cart.`);
} else {
console.log(`${itemName} is not available.`);
}
}
// Calculate total cart price
calculateTotal() {
return this.cart.reduce((acc, item) => acc + item.price, 0);
}
// Filter available items by price
filterAvailableItems(maxPrice) {
return availableItems.filter(item => item.price <= maxPrice);
}
// Display cart items
displayCart() {
this.cart.forEach(item => console.log(`${item.name}: $${item.price}`));
console.log(`Total Price: $${this.calculateTotal()}`);
}
}
// Usage Example
const myCart = new ShoppingCart();
myCart.addItem("Phone");
myCart.addItem("Laptop");
myCart.displayCart();
const affordableItems = myCart.filterAvailableItems(500);
console.log("Items under $500:", affordableItems);
Additional Features to Enhance the Project:
User Authentication: Allow users to sign in and maintain their cart data.
Category Filtering: Let users filter items by categories such as electronics, accessories, etc.
Discount Functionality: Apply discount codes to reduce the total price using
map()
or custom callback functions.Persistent Storage: Save cart data to local storage so that it persists after page reload.
UI Enhancement: Use HTML/CSS to create a user-friendly interface.
Conclusion:
This mini-project demonstrates how Higher-Order Functions (HOFs) like reduce()
and filter()
can be used to build interactive and efficient web applications. It's a great starting point for developers looking to understand the power of functional programming in JavaScript.
Interview Questions on Higher-Order Functions (HOFs)
1. What is a higher-order function in JavaScript?
A higher-order function is a function that either:
Takes one or more functions as arguments, or
Returns a function as its result.
In JavaScript, functions are first-class citizens, meaning they can be passed around as arguments to other functions and can also be returned from other functions. Examples of higher-order functions in JavaScript include map()
, filter()
, reduce()
, forEach()
, and setTimeout()
.
Example:
const add = (a, b) => a + b;
const higherOrderFunction = (callback) => {
return callback(5, 10); // Calls the passed function with 5 and 10
}
console.log(higherOrderFunction(add)); // Output: 15
In this example, higherOrderFunction
is a higher-order function because it takes add
(a function) as an argument.
2. How does reduce()
differ from map()
?
Both reduce()
and map()
are array methods, but they serve different purposes:
map()
: It creates a new array populated with the results of calling a provided function on every element in the array.It does not change the original array.
It returns an array of the same length as the original.
Example:
const numbers = [1, 2, 3, 4];
const squares = numbers.map(num => num * num);
console.log(squares); // Output: [1, 4, 9, 16]
reduce()
: It applies a function (called a reducer) to each element of the array to reduce it to a single value (e.g., sum, product, concatenation). It takes an accumulator and the current value as arguments, and optionally an initial value for the accumulator.It iterates through the entire array and accumulates the result.
It returns a single value (not an array).
Example:
const numbers = [1, 2, 3, 4];
const sum = numbers.reduce((acc, num) => acc + num, 0);
console.log(sum); // Output: 10
3. What are the advantages of using callbacks?
Callbacks offer several advantages:
Asynchronous Programming: Callbacks allow JavaScript to perform asynchronous operations. For example, when using a
setTimeout()
or an API call, the callback function is executed when the operation completes, without blocking the execution of other tasks.Example:
setTimeout(() => { console.log("This will be printed after 2 seconds."); }, 2000);
Code Reusability: Callbacks allow the reuse of functions in different contexts. A function can accept a callback that defines how it should behave, making the code more flexible.
Separation of Concerns: By passing functions as callbacks, we can separate the logic of what to do from the logic of when to do it, which leads to cleaner and more modular code.
4. Can you explain callback hell and how to avoid it?
Callback hell refers to the situation where callbacks are nested within one another, making the code hard to read, debug, and maintain. This usually happens in asynchronous code, especially when there are multiple sequential or dependent operations.
Example of Callback Hell:
fs.readFile('file1.txt', (err, data1) => {
fs.readFile('file2.txt', (err, data2) => {
fs.readFile('file3.txt', (err, data3) => {
// More nested callbacks
});
});
});
This leads to "pyramid" or "ladder" code, which is difficult to manage.
How to Avoid Callback Hell:
Use Named Functions: Extract the callback functions into separate named functions to improve readability and reusability.
function handleFile1(err, data1) { // Handle file1 } fs.readFile('file1.txt', handleFile1);
Use Promises: Promises provide a cleaner and more manageable way to handle asynchronous code, allowing chaining of
.then()
and.catch()
instead of nesting.fs.promises.readFile('file1.txt') .then(data1 => { return fs.promises.readFile('file2.txt'); }) .then(data2 => { return fs.promises.readFile('file3.txt'); }) .catch(err => { console.log("Error: ", err); });
Use Async/Await:
async/await
makes asynchronous code look synchronous and more readable, avoiding the nesting problem entirely.async function readFiles() { try { const data1 = await fs.promises.readFile('file1.txt'); const data2 = await fs.promises.readFile('file2.txt'); const data3 = await fs.promises.readFile('file3.txt'); } catch (err) { console.log("Error: ", err); } } readFiles();
By using Promises or async/await, we can avoid the deep nesting of callbacks, leading to cleaner, more maintainable code.
Conclusion:
Recap Key Concepts:
Higher-order functions (HOFs) are functions that either take one or more functions as arguments or return a function as a result. They are fundamental in JavaScript for managing asynchronous tasks, operating on arrays, and making code more flexible.
Callbacks are functions passed as arguments to other functions and are executed once a task is complete. They are crucial for handling asynchronous code but can lead to challenges like callback hell if not managed properly.
Common Higher-Order Functions in JavaScript:
map()
: Transforms arrays.reduce()
: Accumulates values into a single result.filter()
: Filters arrays based on conditions.forEach()
: Loops through an array and performs an operation on each element.
Best Practices:
Avoid nesting callbacks deeply. Use named functions, Promises, and async/await to handle asynchronous tasks more cleanly.
Understand the importance of pure functions when using HOFs, as they can lead to predictable and testable code.
Use array methods like
map()
,filter()
, andreduce()
for cleaner and more concise code when dealing with collections.
Importance of HOFs in Modern Development: Higher-order functions are at the heart of modern JavaScript development, especially with the rise of asynchronous programming, functional programming, and reactive programming. HOFs make JavaScript code more modular, reusable, and readable, and are heavily used in frameworks like React and Node.js.
Call to Action: Now that you have a solid understanding of Higher-Order Functions, it's time to practice! Use the mini-projects provided to apply these concepts in real-world scenarios. Additionally, explore other built-in HOFs like find()
, some()
, every()
, and sort()
to expand your knowledge.
Challenge yourself with more complex tasks and enhance your problem-solving skills with functional programming principles. Practice regularly, and HOFs will become second nature in your JavaScript coding journey!
Other Series:
Connect with Me
Stay updated with my latest posts and projects by following me on social media:
LinkedIn: Connect with me for professional updates and insights.
GitHub: Explore my repository and contributions to various projects.
LeetCode: Check out my coding practice and challenges.
Your feedback and engagement are invaluable. Feel free to reach out with questions, comments, or suggestions. Happy coding!
Rohit Gawande
Full Stack Java Developer | Blogger | Coding Enthusiast
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
π Tech Enthusiast | Full Stack Developer | System Design Explorer π» Passionate About Building Scalable Solutions and Sharing Knowledge Hi, Iβm Rohit Gawande! πI am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What Iβm Currently Doing πΉ Writing an in-depth System Design Series to help developers master complex design concepts.πΉ Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.πΉ Exploring advanced Java concepts and modern web technologies. What You Can Expect Here β¨ Detailed technical blogs with examples, diagrams, and real-world use cases.β¨ Practical guides on Java, System Design, and Full Stack Development.β¨ Community-driven discussions to learn and grow together. Letβs Connect! π GitHub β Explore my projects and contributions.πΌ LinkedIn β Connect for opportunities and collaborations.π LeetCode β Check out my problem-solving journey. π‘ "Learning is a journey, not a destination. Letβs grow together!" Feel free to customize or add more based on your preferences! π