Top 10 JS_arrayMethods

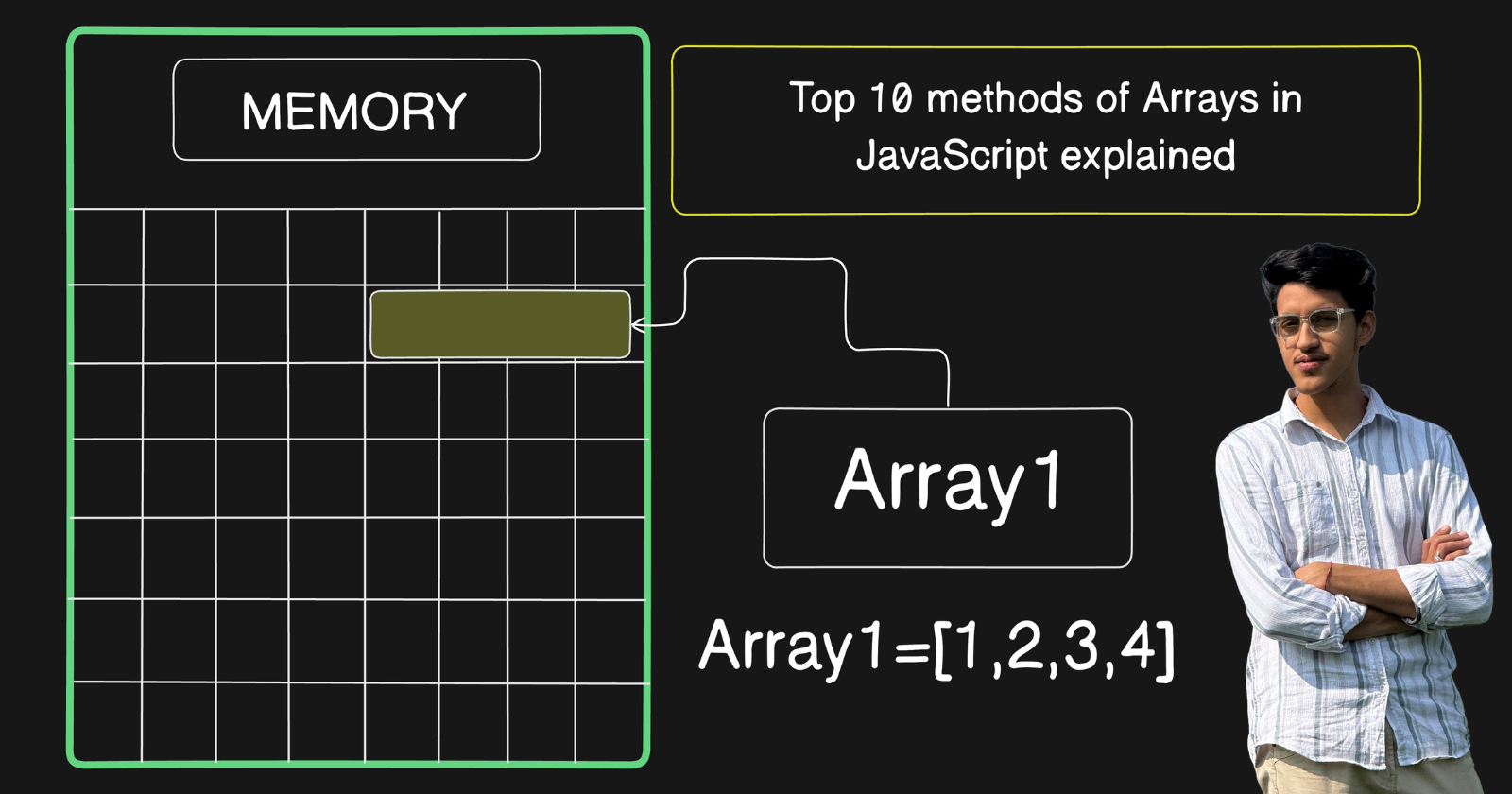
Arrays in JS
Arrays in JS ate the non-preemptive data types. Arrays are a special type of object. The ‘typeof’ operator in JavaScript returns "object" for arrays.
Arrays are the datatypes that have values(data) of similar data types.
When we declare an array in JS, a continuous location is allocated in the memory, that stores the elements of that array.
In this figure we can see that the array “Array1“ has given 4 continuous memory blocks, as the array is “Array1 = [1,2,3,4];“.
Top 10 Methods/Properties of Arrays in JS
.concat()
The .concat()
method of array in JS is used when we have to join two arrays together.
For example: Suppose we have two smaller teams (arrays) with four players(elements) each and at an auction one team, let’s say Rajasthan Royals, wants all the 8 players and hence purchases both the teams
So now the new RR team = Team1+Team2
Here's how you can write it in JavaScript:
let Team1 = [1,2,3,4];
let Team2 = [5,6,7,8];
let TeamRR = Team1.concat(Team2);
console.log(TeamRR);
Output : [1, 2, 3, 4, 5, 6, 7, 8]
.filter()
The .filter()
method in JavaScript is used to create a new array with all elements that pass a test implemented by a provided function.
For Example: Suppose we have a cricket team of seven players with different no of centuries, and we want to filter out the players who have hit more than 5 centuries.
Here's how you can write it in JavaScript:
let players = [3, 6, 8, 2, 7, 4, 10];
let highScorers = players.filter(function(score) {
return score > 5;
});
console.log(highScorers);
Output: [6, 8, 7, 10]
In this example, the filter()
method is used to create a new array highScorers
that contains only the scores greater than 5 from the players
array.
.find()
The .find()
method in JavaScript is used to return the value of the first element in an array that satisfies a provided testing function.
For Example: Suppose we have a bag of chocolates that contains seven chocolate boxes, with different numbers of chocolates in them, and we want to find the first box that contains more than 5 chocolates.
Here's how you can write it in JavaScript:
let chocoleteBox = [3, 6, 8, 2, 7, 4, 10];
let mostChocoletes = chocoleteBox.find(function(chocolete) {
return chocolete > 5;
});
console.log(mostChocoletes);
Output: 6
In this example, the find()
method is used to find the first box of chocoletes
that has more than 5 chocolates, which is box with 6 chocolets
.
.pop()
The .pop()
method is used to remove the last element from an array and return that element.
For Example: Suppose we have a car full of friends, represented as an array, and we want to remove the last friend who gets out of the car.
Here's how you can write it in JavaScript:
let friendsInCar = ["Karan", "Ravi", "Hitesh", "Rahul"];
let lastFriendOut = friendsInCar.pop();
console.log(lastFriendOut);
console.log(friendsInCar);
Output:
Rahul
["Karan", "Ravi", "Hitesh"]
In this example, the pop()
method is used to remove "David" from the friendsInCar
array, as he is the last friend to get out of the car. The array is then updated to reflect the remaining friends.
.push()
The .push()
method is used to add one or more elements to the end of an array.
For Example : Suppose we have a group of friends at an Indian wedding, and they are forming a queue to get their favourite sweets. As more friends join the queue, we can use the .push()
method to add them to the end of the line.
Here's how you can write it in JavaScript:
let sweetQueue = ["Rahul", "Priya", "Anjali"];
sweetQueue.push("Vikram");
sweetQueue.push("Sita", "Ravi");
console.log(sweetQueue);
Output : ["Rahul", "Priya", "Anjali", "Vikram", "Sita", "Ravi"]
In this example, the push()
method is used to add "Vikram" to the queue first, followed by "Sita" and "Ravi". The array sweetQueue
is updated to reflect the new order of friends eagerly waiting for their turn to grab some delicious Indian sweets.
.sort()
The .sort()
method in JavaScript is used to sort the elements of an array. The default sort order is ascending order.
For Example: Imagine a group of friends deciding to rank their favourite Bollywood movies. They have a list of movie ratings and they want to sort them in ascending order to see which movie is the most popular.
Here's how you can write it in JavaScript:
let movieRatings = [4.5, 3.2, 5.0, 4.8, 3.9];
movieRatings.sort(function(a, b) {
return a - b;
});
console.log(movieRatings);
Output:
[3.2, 3.9, 4.5, 4.8, 5.0]
In this example, the sort()
method is used to arrange the movieRatings
array in ascending order. The friends can now easily see that the movie with a rating of 5.0
is their top favourite, while the one with 3.2
might need a rewatch to appreciate its hidden charms!
.map()
The .map()
method is used to create a new array by applying a provided function to each element of the original array.
For Example: Imagine you have a group of cats, and each cat has a certain number of lives left. You want to give each cat an extra life because, well, why not? Cats are awesome! So, you decide to use the .map()
method to add one more life to each cat.
Here's how you can write it in JavaScript:
let cats = [8, 7, 5, 9]; // Lives left for each cat
let extraLifeCats = cats.map(lives => lives + 1);
console.log(extraLifeCats);
Output: [9, 8, 6, 10]
In this example, each cat in the cats
array gets an extra life, resulting in the extraLifeCats
array. Now, all the cats are happier and ready for more adventures!
.reverse()
The .map()
method is used to create a new array by applying a provided function to each element of the original array.
For Example: Imagine you have a group of plants, and each plant needs a certain amount of water in litres. You decide to double the amount of water for each plant to ensure they grow well. So, you use the .map()
method to double the water requirement for each plant.
Here's how you can write it in JavaScript:
let plants = [2, 3, 1.5, 4]; // Water needed for each plant in liters
let doubleWaterPlants = plants.map(water => water * 2);
console.log(doubleWaterPlants);
Output: [4, 6, 3, 8]
In this example, each plant in the plants
array gets its water requirement doubled, resulting in the doubleWaterPlants
array. Now, all the plants are well-hydrated and ready to thrive!
.shift()
The .shift()
method in JavaScript is used to remove the first element from an array and return that removed element. This method changes the length of the array and shifts all other elements to a lower index.
For Example: Imagine you have a line of people waiting to enter a concert. As the doors open, the first person in line enters the venue. You can use the .shift()
method to represent this action in JavaScript.
Here's how you can write it in JavaScript:
let concertQueue = ["Alice", "Bob", "Charlie", "David"];
let firstPerson = concertQueue.shift();
console.log(firstPerson); // Output: "Alice"
console.log(concertQueue); // Output: ["Bob", "Charlie", "David"]
Output: "Alice"
Output: ["Bob", "Charlie", "David"]
In this example, "Alice" is the first person in the concertQueue
array and is removed using the shift()
method. The array is then updated to reflect the remaining people in line.
.unshift()
The .unshift()
method in JavaScript is used to add one or more elements to the beginning of an array. This method changes the length of the array and shifts the existing elements to higher indexes.
For Example: Imagine you have a list of tasks for the day, and suddenly you remember an urgent task that needs to be done first. You can use the .unshift()
method to add this urgent task to the beginning of your task list.
Here's how you can write it in JavaScript:
let tasks = ["Check emails", "Attend meeting", "Submit report"];
tasks.unshift("Urgent: Call client");
console.log(tasks);
Output: ["Urgent: Call client", "Check emails", "Attend meeting", "Submit report"]
In this example, the urgent task "Urgent: Call client" is added to the beginning of the tasks
array using the unshift()
method. The array is then updated to reflect the new order of tasks, ensuring that the urgent task is prioritized.
Subscribe to my newsletter
Read articles from Karan Choudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
