Offline-First Recipe Manager with AWS AppSync and Amplify

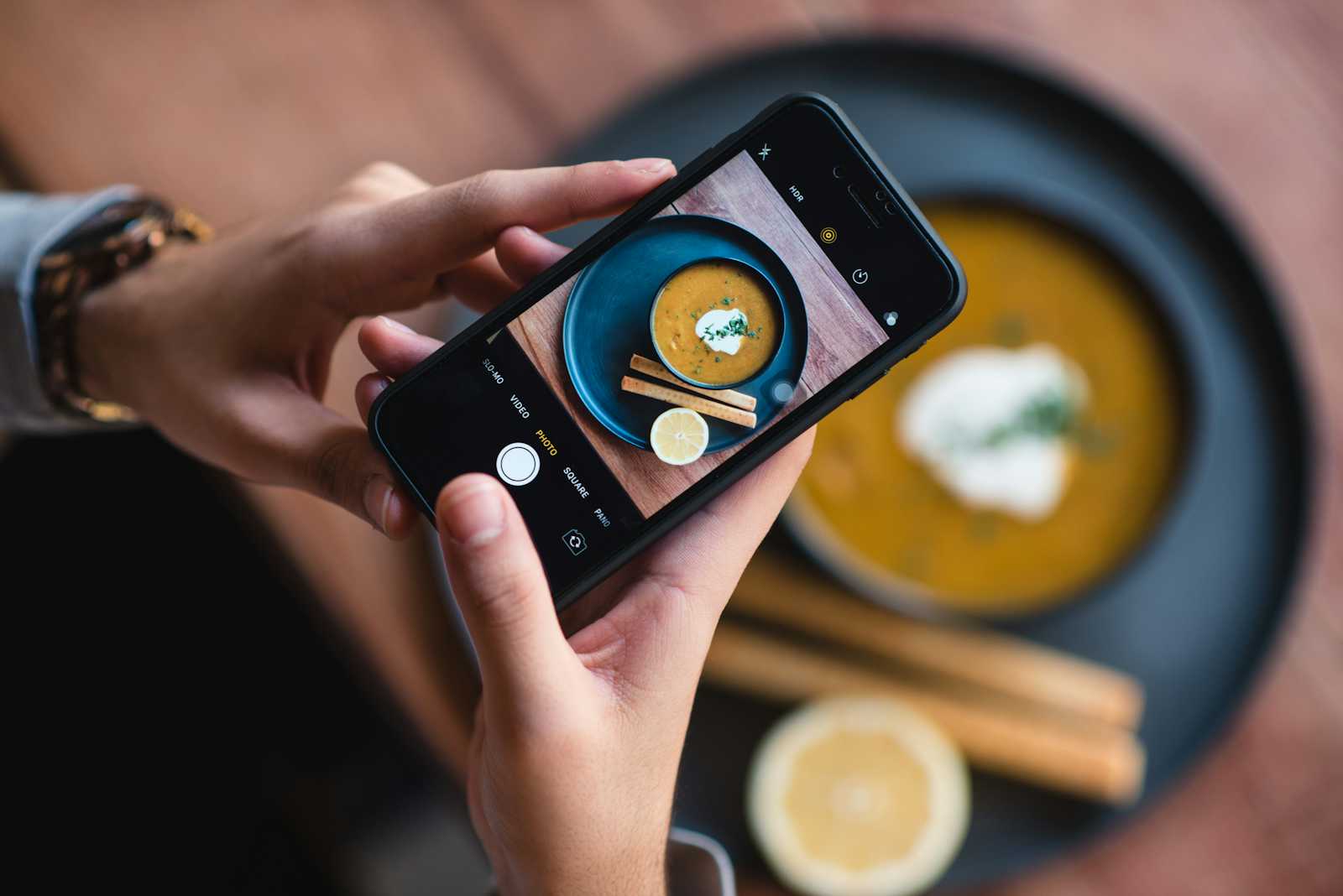
[Summary Generated with AI] This article discusses the importance of offline capabilities in mobile applications to prevent data loss due to connectivity issues. It highlights how AWS AppSync and Amplify simplify offline data synchronization, allowing developers to focus on business logic. The article provides a proof of concept for a recipe-managing app, featuring a backend using AWS AppSync, DynamoDB, and AWS CDK for Infrastructure as Code. It explains how to connect the backend to a mobile frontend using AWS Amplify's DataStore, ensuring seamless data handling when offline. The article concludes by emphasizing the benefits of an offline-first strategy for mobile apps to enhance user experience.
Introduction
In today’s world standards, when planning to build a mobile application, it’s essential that offline capabilities are in place. This is important in order to avoid data loss, as mobile devices often suffer from bad connectivity and internet access may be limited
To offer the best user experience to users, an application that supports offline capabilities seamlessly is important. As a developer, manually managing updates that are in the device vs in the database can be very difficult to handle, as local device cache vs cloud database synchronizations may result in data being overwritten, deleted or ignored if not done properly
This is where AppSync and Amplify come to the rescue! The offline capabilities and data synchronization is already taken care by these two technologies, allowing the developers to focus on the business logic rather than spending too much time in carefully handling offline vs online synchronizations
Let’s take a look at how it works with a small POC for a simple recipe-managing app:
Recipe Managing POC
Use Cases
For illustrating the offline capabilities of AWS AppSync and Amplify, let’s build a simple poc app that lets a user manage recipes in a mobile device. Specifically, It allows for the two main use cases:
Create recipes (Title, Description, List of Ingredients and Cooking Instructions)
Get Recipe
A real recipe-app would have more use cases and be more robust, but for the purposes of illustrating the topic of this blog post, those should be enough
In order to fulfill these use cases, we can have a backend composed by a graphql AppSync API and a dynamodb table that holds the information. In the UI, we can use Amplify’s capabilities with DataStore for offline sync
Architecture
The architecture of the POC developed in this blog post involves a mobile app client that is using AWS Amplify to interact with AWS services. Amplify leverages the “DataStore”, which is connected to the Recipes graphql api, which uses a DynamoDb table “Recipes Database” to store recipes data
High-level overview of the architecture for the “Recipes App” POC. Own Creation
The Data Flow for this architecture starts from the mobile app client, which can be any modern framework to build mobile apps. Using an offline-first approach, the mobile framework can leverage AWS Amplify’s “DataStore” and integrate with the “Recipes API” to have the data synchronized between the device’s offline cache and the dynamodb “Recipes Database” table
Backend Implementation
Implementing this small poc is easy to do with the help of AWS CDK to define our resources as Infrastructure as Code. First, we can set-up a new cdk project with the following commands (make sure cdk’s latest version is installed in your system first)
# Install CDK globally (only if needed. run "cdk --version" to check)
npm install -g aws-cdk
# Create a new project
mkdir offline-first-recipe-manager
cd offline-first-recipe-manager
cdk init app --language typescript
This commands will create a new CDK stack called “offline-first-recipe-manager”.
After the project is bootstrapped, install the libraries for our aws resources:
npm install @aws-cdk/aws-appsync @aws-cdk/aws-dynamodb
Also make sure you have an aws account ready to use and you have a profile configured with programmatic access in your local machine. To find out how to do it, check this link
To check the implementation and code, please see the GitHub Repository
Connect the Backend to our mobile Frontend
Now that we have a backend poc ready, we can easily connect it to the mobile Frontend by using AWS Amplify’s configuration:
// If using React Native and Typescript
import Amplify from 'aws-amplify';
Amplify.configure({
aws_appsync_graphqlEndpoint: '', // replace from the CDK output
aws_appsync_region: 'us-east-1',
aws_appsync_authenticationType: 'API_KEY',
aws_appsync_apiKey: '', // replace from the CDK output
});
// If using Flutter and Dart, we can use the "initState" to initialize amplify:
@override
void initState() {
super.initState();
_configureAmplify();
}
// Configure Amplify with the DataStore plugin
Future<void> _configureAmplify() async {
final dataStorePlugin =
AmplifyDataStore(modelProvider: ModelProvider.instance);
await Amplify.addPlugin(dataStorePlugin);
try {
await Amplify.configure(amplifyconfig);
setState(() {
_isAmplifyConfigured = true;
});
} catch (e) {
print('Amplify configuration failed: $e');
}
}
Using the AWS Amplify’s DataStore is also easy to do. Using the SDK for the appropriate framework, we can use it to have an offline-first application:
// If using React Native and Typescript
import { DataStore } from 'aws-amplify'
async function fetchRecipes() {
try {
const recipes = await DataStore.query(Recipe)
console.log('Fetched recipes:', recipes)
} catch (error) {
console.error('Error fetching recipes:', error)
}
}
// If using Flutter and Dart
import 'package:amplify_flutter/amplify_flutter.dart';
import 'package:amplify_datastore/amplify_datastore.dart';
// ...
void _queryRecipes() async {
try {
final recipes = await Amplify.DataStore.query(Recipe.classType);
recipes.forEach((recipe) {
print("Recipe: ${recipe.title}");
});
} catch (e) {
print("Error querying recipes: $e");
}
}
By using AWS Amplify’s DataStore for fetching and saving the data instead of calling the API directly, we leave the data sync to Amplify. Even if the device is offline, the cache will store and sync later when connectivity is back
Conclusion
Building applications that handle data is a common day-to-day requirement. Thinking about mobile devices, where the connectivity can fail at times, we need to think about offline-first approach to data handling, which involves having data stored in the mobile device’s internal cache, and then synchronizing with the database (system of record)
Manually building this synchronization can be a very complex task. Luckily, AWS Amplify’s DataStore and AWS AppSync offer a seamless way to build these kind of offline-first apps. In this blog post I went over a simple POC for handling cooking recipes from a mobile device using this offline-first approach
With technologies such as AWS CDK, AppSync and DynamoDb, building the backend was simple and fast, while integrating with a mobile app was also simple by using Amplify’s configuration and SDK capabilities.
By using an offline-first strategy in your app you’re future-proofing your app and providing real value to users who may have spotty WiFi or internet connection, which may be common. Check out the GitHub Repository for the implementation and experiment with these technologies for you next project. Happy Coding!
References
Subscribe to my newsletter
Read articles from Lucas Vera Toro directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Lucas Vera Toro
Lucas Vera Toro
Technical Architect and Developer with 10 years of experience, specializing in scalable and efficient digital products powered by cloud technologies.