A Day in the Life of a JavaScript Developer: Managing Tasks with Array Methods

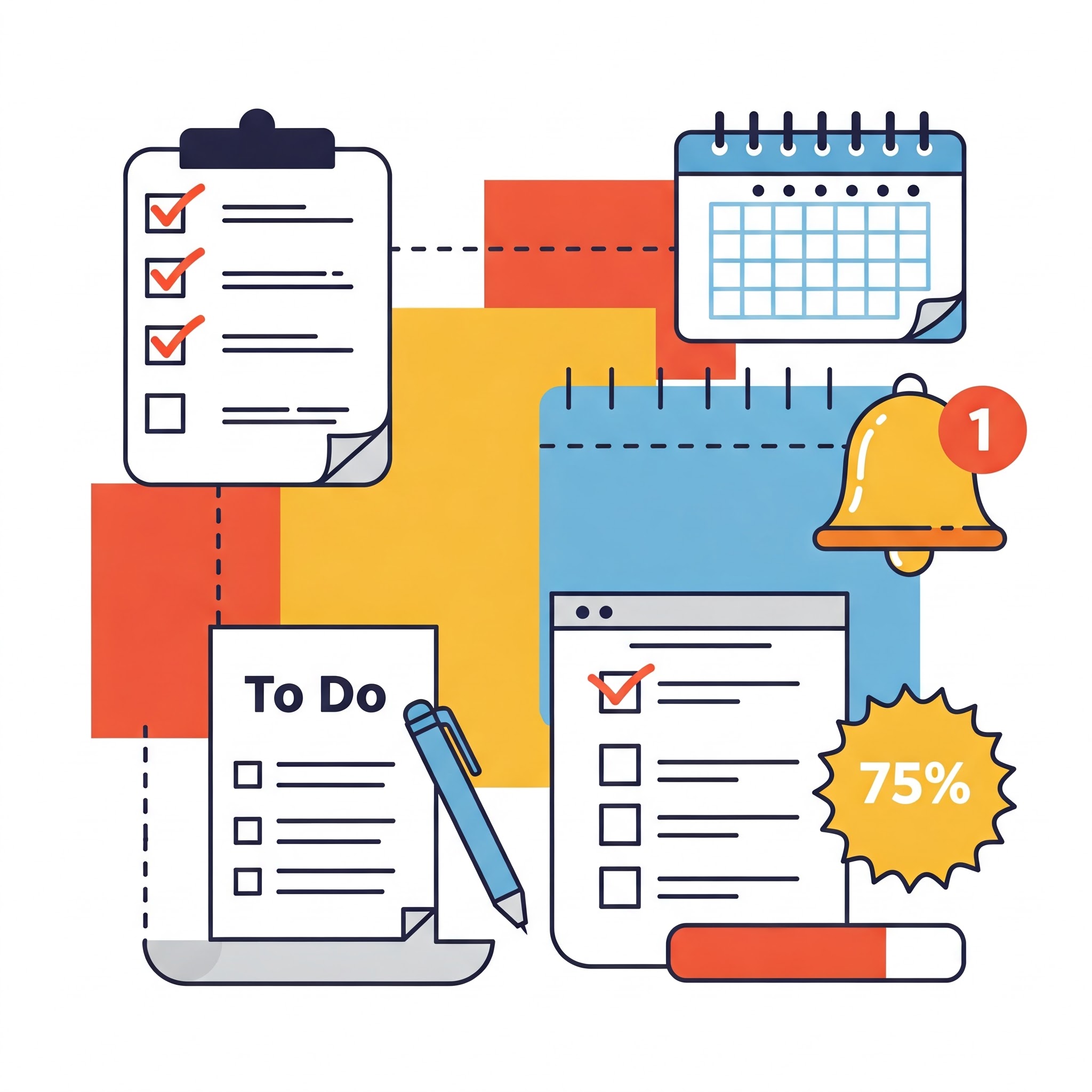
Every day as a developer is an adventure in coding, problem-solving, and—surprisingly—organizing my daily routine with JavaScript. Today, I want to share how I use array methods to manage my tasks. Each step of my day is marked by a different built-in array method.
1. push() – Adding a New Task
When I wake up I start reviewing my task list, which is stored as an array:
let tasks = [
'dsa -2ques',
'attend college lectures',
'attend cohort lecture',
'go out with frineds fro evening chai',
'solve challenge questions'
];
Realizing I need to squeeze in a workout, I add a new task using push():
tasks.push('Exercise 30mins');
2. forEach() – Reviewing the Task List
Before diving into the day, I want to quickly review my tasks. I use the forEach() method to print each one:
tasks.forEach(task => console.log(task));
3. splice() – Removing Unimportant Tasks
As the day unfolds, I recognize that some tasks aren’t critical. I decide to remove unnescessary task by using splice(). In this case, I remove 1 tasks starting at index 3:
tasks.splice(3, 1);
After the removal, my list looks like this:
tasks = ['dsa -2ques', 'attend college lectures', 'attend cohort lecture', 'solve challenge question']
4. length – Counting Remaining Tasks
Before proceeding, I check how many tasks are left with the length property:
console.log("Total tasks to complete: " + tasks.length);
5. at() – Marking Completed Tasks
I then complete the first three tasks of the day. To access each specific task by its index, I use the at() method inside a loop:
for (let i = 0; i < 3; i++) {
console.log(`Tasks completed: ${tasks.at(i)}`);
}
By midday, after marking these as done, only one task remains: solving the challenge question.
6. find() – Verifying the Pending Task
At the end of the day, I need to ensure that the pending task—solving the challenge question—is still on my list. I use find() to locate it:
let pendingTask = tasks.find(task => task.includes("solve"));
console.log("Pending task: " + pendingTask);
7. concat() – Merging Tasks for Tomorrow
Planning ahead, I think of extra tasks I want to do tomorrow. I create an array of these extra tasks:
let extraTasks = ["read documentation", "practice coding", "review notes"];
To prepare for tomorrow without altering today’s list, I merge my current tasks with the extra ones using concat():
let tomorrowTasks = tasks.concat(extraTasks);
console.log("Tomorrow's tasks: ", tomorrowTasks);
8. sort() – Organizing Tomorrow's Tasks
To make my plan clear, I sort tomorrow’s tasks alphabetically using sort():
tomorrowTasks.sort();
console.log("Sorted tasks for tomorrow: ", tomorrowTasks);
9. join() – Creating a Summary String
For a quick overview, I join all the tasks into one summary string. The join() method helps me combine them with a pipe separator:
summary = tomorrowTasks.join(" | ");
console.log("Task summary: " + summary);
10. map() – Formatting the Final Plan
Finally, I add a personal touch by transforming each task into a formatted message. I use map() to prepend “Scheduled:” to each task, creating my final plan:
let finalPlan = tomorrowTasks.map(task => "Scheduled: " + task);
console.log("Final plan: " + finalPlan.join(", "));
Thanks for reading
Subscribe to my newsletter
Read articles from rohit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
