Master Intermediate Svelte: Simplify Reactivity, Stores, Slots, and More!

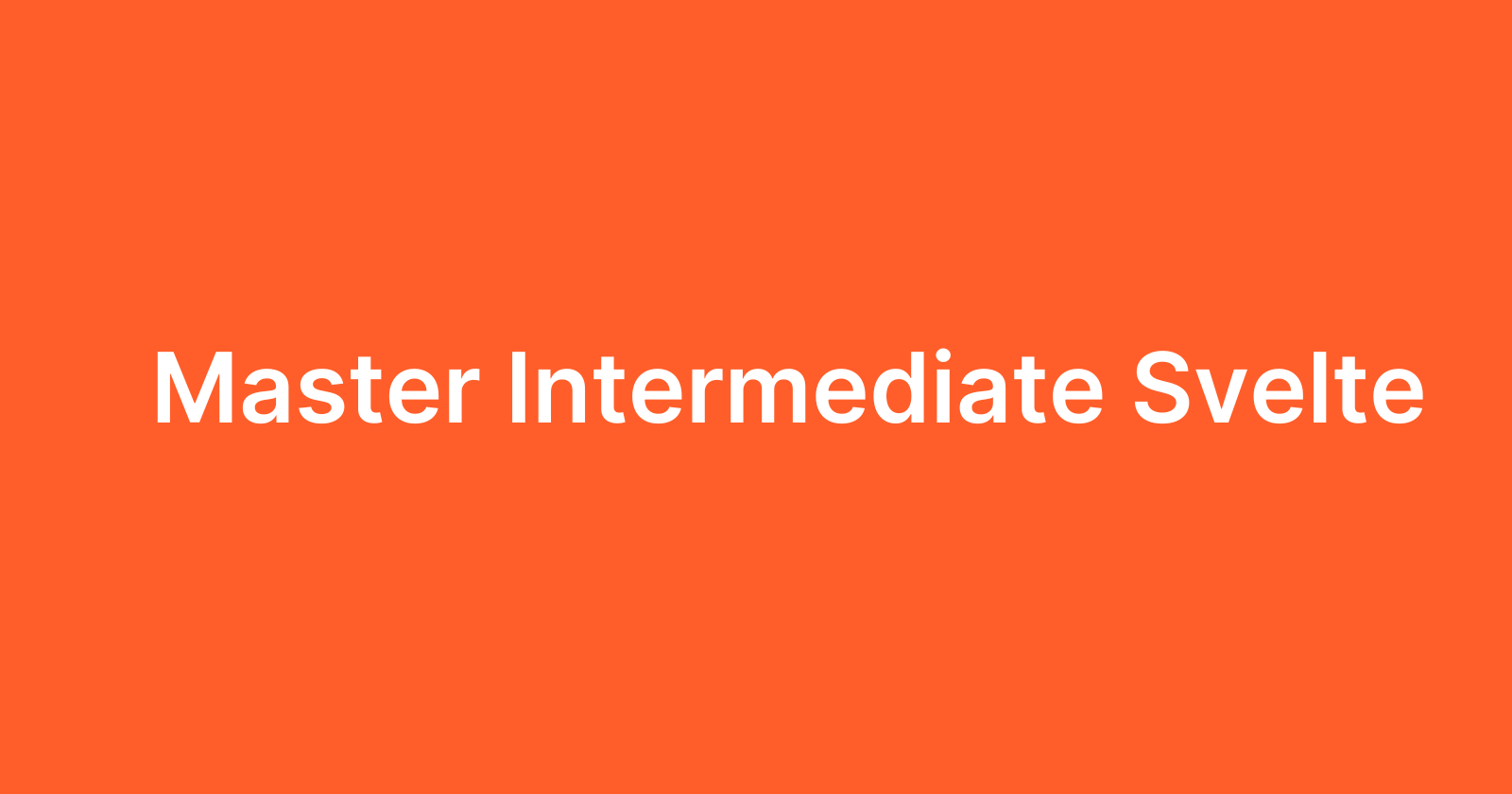
1. Reactivity Beyond the Basics
What it is: Automatically updates values when something changes.
Simpler Term: Updates when things change, like a spreadsheet auto-calculating values.
Example:
$:
makes things reactive.<script> let count = 0; $: doubled = count * 2; // Automatically updates when count changes </script> <p>{doubled}</p>
2. Stores (Global State Management)
What it is: Allows components to share state without passing data through every level.
Simpler Term: A shared notebook that everyone can read and write to.
Example:
// store.js import { writable } from 'svelte/store'; export const count = writable(0);
<script> import { count } from './store.js'; function increment() { count.update(n => n + 1); } </script> <button on:click={increment}>Clicks: {$count}</button>
3. Slots (Component Content Injection)
What it is: Allows content to be passed into a component from the parent.
Simpler Term: A "fill-in-the-blank" in your component.
Example:
<!-- Box.svelte --> <div class="box"> <slot>Default content if none provided</slot> </div>
4. Context API (Component "Whispering")
What it is: Share data between deeply nested components without props.
Simpler Term: A secret message passed directly to a child, skipping the middle.
Example:
// Parent.svelte import { setContext } from 'svelte'; setContext('theme', 'dark');
<!-- Child.svelte --> <script> import { getContext } from 'svelte'; const theme = getContext('theme'); // 'dark' </script> <p>{theme}</p>
5. Transitions and Animations
What it is: Built-in effects to animate elements.
Simpler Term: Smooth transitions for element changes.
Example:
<script> import { fade } from 'svelte/transition'; </script> <div transition:fade> This will fade in and out! </div>
6. Lifecycle Functions
What it is: Code that runs when components are created, updated, or destroyed.
Simpler Term: Set up/clean up when components come and go.
Example:
<script> import { onMount, onDestroy } from 'svelte'; onMount(() => console.log("Component mounted")); onDestroy(() => console.log("Component destroyed")); </script>
7. Module Context (`)
What it is: Shared state across all instances of a component.
Simpler Term: Shared workspace for all component instances.
Example:
<script context="module"> let totalInstances = 0; // Shared across all component instances </script>
8. Actions (use:action
)
What it is: Enhance DOM elements with reusable functions.
Simpler Term: Give elements superpowers.
Example:
// tooltip.js export function tooltip(node) { node.addEventListener('mouseover', () => { // Show tooltip }); }
<div use:tooltip>Hover me!</div>
9. Error Boundaries ({@error}
)
What it is: Handle errors gracefully in child components.
Simpler Term: Catch and display errors.
Example:
{#await somePromise} Loading... {:then result} <p>{result}</p> {:catch error} {@error error} <p>Error: {error.message}</p> {/error} {/await}
10. Custom Events
What it is: Allow child components to send messages to the parent.
Simpler Term: A child passing a note to the parent.
Example:
<script> import { createEventDispatcher } from 'svelte'; const dispatch = createEventDispatcher(); function sendMessage() { dispatch('message', { text: 'Hello!' }); } </script> <button on:click={sendMessage}>Click me</button>
Key Takeaways
Reactivity: Automatically update values using
$:
and stores.Slots/Context: Easily pass data and content without deep prop drilling.
Transitions/Actions: Add smooth animations and interactive effects.
Lifecycle/Module: Control component behavior at various points.
Svelte makes things easy by handling a lot of logic behind the scenes through its compiler.
Subscribe to my newsletter
Read articles from Pravin Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pravin Jadhav
Pravin Jadhav
Hi, My goal is to demystify DevOps, break down complex concepts into approachable lessons, and build a resource that not only tracks my progress but also helps others accelerate their own learning curves. Thank you for joining me on this journey—let's explore the future of development and operations together!