Nuxt 3: Why App Fails To Make Server-Side API Requests to Local Backend in Development.
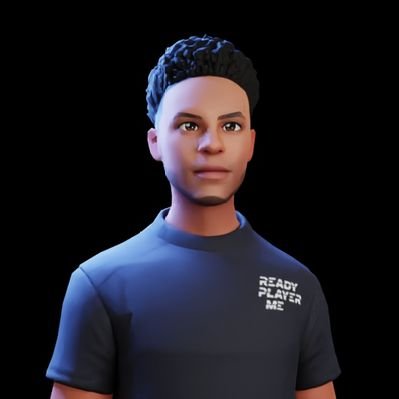
While working on a Nuxt 3 application, I needed to fetch data from my backend using useFetch
and useAsyncData
, which are commonly used for server-side data fetching in Nuxt. The requests worked fine when targeting a public URL, but when making requests to my local backend, they failed during the initial server-side request. This was a critical issue for me, as server-side rendering (SSR) is essential to my application’s functionality.
Interestingly, this error only occurred during the first server-side request. Once the Nuxt app loaded and subsequent requests were made from the browser, they succeeded without any issues.
If you're facing a similar problem, this article will walk you through the cause of the issue and the steps to properly fix it.
🔍 The Problem: SSL Certificate Not Recognized
In my Nuxt 3 app, I was making a server-side request to my local backend using useFetch
and useAsyncData
, which are commonly used for server-side data fetching in Nuxt.
const { data } = await useFetch("https://localhost:7003/api/courses?pageSize=500")
But the request failed with an error:
ERROR [GET] "https://localhost:7003/api/courses?pageSize=500": <no response> fetch failed
At first, I assumed it was a browser-related issue, but then there was no error log on the browser.
Why is this happening?
Server-side data fetching requests are made inside Nuxt server (Node.js), not the browser.
My backend was using a self-signed SSL certificate.
Node.js does not trust self-signed certificates by default.
Unlike browsers, which allow users to bypass SSL warnings, Node.js enforces strict SSL validation.
✅ Quick Temporary Fix
To verify that SSL was the issue, I added this line to my app.vue
file:
process.env.NODE_TLS_REJECT_UNAUTHORIZED = "0";
This effectively disabled SSL verification, allowing Node.js to accept any certificate. After this change, my server-side requests started working.
⚠ Warning: This is NOT a recommended solution for production! Disabling SSL verification exposes your application to security risks.
🔧 A More Permanent Solution: Installing the Certificate
Instead of disabling SSL verification, a more stable approach is to install your local backend’s SSL certificate in your Node.js environment.
Step 1: Export the Certificate
If you generated a self-signed certificate, you should have a .crt
file. If not, you can extract it from your backend (you can do this using OpenSSL)
For example, if you're using OpenSSL, you can find the certificate using:
openssl s_client -connect localhost:7003 -showcerts
localhost:7003
is the URL for my local backend API. Be sure to replace it with your own local backend URL.
Copy the certificate content (everything between -----BEGIN CERTIFICATE-----
and -----END CERTIFICATE-----
) and save it as localhost
.crt
.
Step 2: Add the Certificate to Node.js
Set the NODE_EXTRA_CA_CERTS
environment variable in your local node environment
set NODE_EXTRA_CA_CERTS=./certs/localhost.crt
Restart your Nuxt app, and your requests should now work without disabling SSL verification.
🎯 Conclusion
If your Nuxt 3 app fails to connect to a self-signed HTTPS backend, the issue is likely due to Node.js rejecting the certificate. While disabling SSL verification might seem like a quick fix, the proper solution is to install and configure your certificate correctly.
This approach keeps your development environment secure while ensuring smooth local API communication. 🚀
Would love to hear your thoughts! Have you encountered a similar issue? Let me know in the comments! 👇
Subscribe to my newsletter
Read articles from Ohien Stephen directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
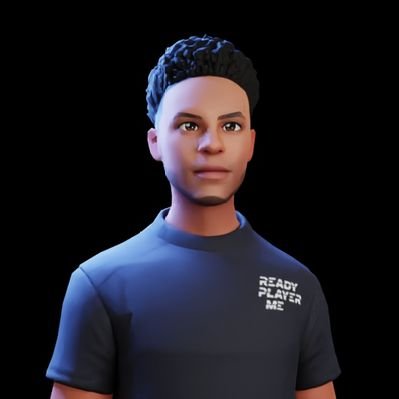