Dora: The JavaScript Explorer

Table of contents
- Packing My Bag: The map Adventure
- The First Obstacle: The Unwanted Tools
- The Map: Getting the Right Directions
- Adding More Tools: The push Adventure
- Sorting the Tools: The sort Adventure
- Time to Eat: The splice Snack Break
- Counting the Tools: The reduce Challenge
- The Big Question: some or every?
- The Final Step: Finding the Treasure
- The End of the Adventure
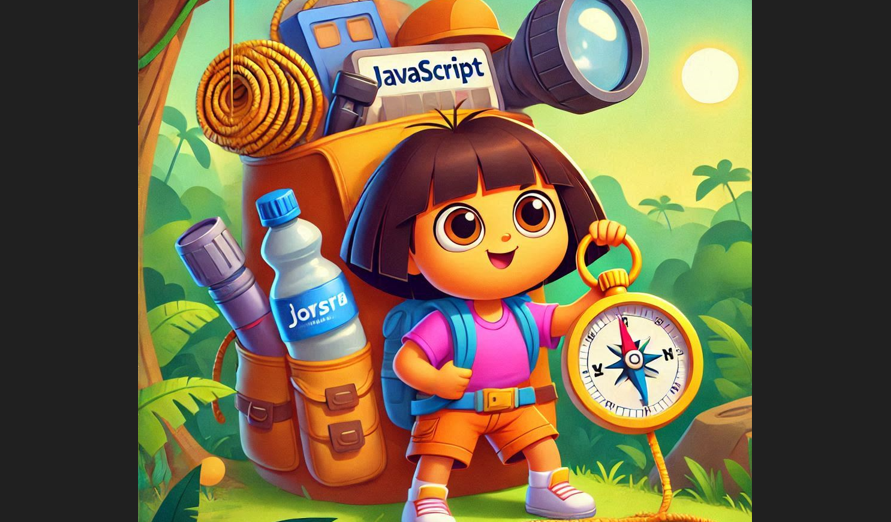
Hola, amigos! It’s me, Dora, and today I’m going on a special adventure to find a treasure hidden deep in the Mystic Jungle! I’m going to need your help, so get ready for some fun! As we go on our journey, we’re going to learn some cool JavaScript array methods along the way. Let’s start the adventure!
Packing My Bag: The
map
Adventure
Before we leave the house, I need to pack my bag for the journey. I have some tools, but I need to double their sizes so they’re more useful! My compass, flashlight, and rope are too small to carry on the big adventure. What should I do?
I use map
, the transformation tool, to make everything bigger!
javascript
Copy
let tools = ['compass', 'flashlight', 'rope'];
let bigTools = tools.map(tool => tool + ' (big)');
console.log(bigTools); // ['compass (big)', 'flashlight (big)', 'rope (big)']
Now, my tools are ready for anything! “These are perfect for the journey!” I say, excited.
The First Obstacle: The Unwanted Tools
As we walk down the path, I notice that some of my tools are too heavy. I don’t need extra snacks or a broken pencil in my bag. I need to filter out the tools I don't need.
filter
is here to help! I can remove the unwanted items. I tell Boots, “We’ll use filter
to make my bag lighter!”
javascript
Copy
let tools = ['compass', 'flashlight', 'broken pencil', 'extra snacks'];
let usefulTools = tools.filter(tool => tool !== 'broken pencil' && tool !== 'extra snacks');
console.log(usefulTools); // ['compass', 'flashlight']
“Look! My bag is much lighter now!” I say with a smile. Boots agrees, “Great job, Dora!”
The Map: Getting the Right Directions
We need a map to find the treasure, but it’s too big and there are too many places marked on it. We need to find the first treasure location on the map. But how can we find it quickly?
I know! We’ll use find
, the fastest method to help us search. I look closely at the map and use find
to find the first treasure spot:
javascript
Copy
let mapLocations = ['cave', 'waterfall', 'treasure spot', 'mountain'];
let treasureLocation = mapLocations.find(location => location === 'treasure spot');
console.log(treasureLocation); // 'treasure spot'
“Yay! We found the treasure location!” I say, pointing to the map. Boots jumps in excitement, “Let’s go, Dora! We’re getting closer!”
Adding More Tools: The
push
Adventure
As we move forward, we realize we need more tools, like a shovel and a flashlight for exploring dark caves. What should we do?
We need to add these tools to my bag! I call on push
, the tool that helps us add things to the end of an array. I quickly use it:
javascript
Copy
let tools = ['compass', 'flashlight'];
tools.push('shovel', 'new flashlight');
console.log(tools); // ['compass', 'flashlight', 'shovel', 'new flashlight']
“Perfect! Now we’re ready for anything,” says Boots. My bag is fuller than ever!
Sorting the Tools: The
sort
Adventure
As we keep walking, I notice that my tools are all jumbled up in my bag! The flashlight is mixed with the shovel, and I need to organize them! What should I do?
It’s time to sort the tools! sort
will arrange them in the order I need. We organize them alphabetically so we can find everything quickly. I shout, “Let’s use sort
!”
javascript
Copy
let tools = ['shovel', 'compass', 'new flashlight', 'flashlight'];
tools.sort();
console.log(tools); // ['compass', 'flashlight', 'new flashlight', 'shovel']
Now everything is in order! Boots claps his hands, “Great job, Dora! Now we can find our tools easily!”
Time to Eat: The
splice
Snack Break
Suddenly, we get hungry and need to eat some snacks. But my bag is so full, I can’t find the energy bar! We need to remove some things to make room for the snacks.
I call on splice
, the perfect method to remove something from an array. I use it to make space for our snacks:
javascript
Copy
let tools = ['compass', 'flashlight', 'shovel', 'new flashlight'];
tools.splice(2, 1); // Remove 1 item at index 2 (shovel)
console.log(tools); // ['compass', 'flashlight', 'new flashlight']
“Yay! Now we have space for our snacks!” I say, and we take a break to eat. Boots enjoys his snack too. “This is the best part of the adventure!”
Counting the Tools: The
reduce
Challenge
After our snack, we need to figure out how many tools we have left. We can’t just count them one by one. Let’s add up all the tools quickly! How?
I use reduce
, the method that helps us sum up all the tools:
javascript
Copy
let tools = ['compass', 'flashlight', 'new flashlight'];
let toolCount = tools.reduce((count) => count + 1, 0);
console.log(toolCount); // 3
“Great! We have 3 tools left!” Boots says. “We’re almost there!”
The Big Question:
some
orevery
?
Now we need to make a decision. Are we sure that we have a flashlight in the bag? We’re almost at the treasure, and we need to know if we have what we need!
I can use some
to check if any of the tools are flashlights! But wait, I need to check if every tool is ready for the adventure. Should I use every
to check that all tools are good?
I decide to use some
, and every
, for both questions!
javascript
Copy
let tools = ['compass', 'flashlight', 'new flashlight'];
let hasFlashlight = tools.some(tool => tool === 'flashlight');
let allReady = tools.every(tool => tool !== 'broken pencil'); // Just checking that there's no broken tool
console.log(hasFlashlight); // true
console.log(allReady); // true
"Great! We have at least one flashlight and all our tools are ready!” I shout! Boots grins, “We’re ready for the treasure now!”
The Final Step: Finding the Treasure
At last, we reach the treasure spot, but we need to combine all our clues to open the chest. What can we do?
We need to combine two arrays—one with map clues and one with final tools! We use concat
, the tool that helps us combine them into one:
javascript
Copy
let mapClues = ['start at the waterfall', 'turn left at the mountain'];
let finalTools = ['compass', 'flashlight', 'shovel'];
let finalJourney = mapClues.concat(finalTools);
console.log(finalJourney); // ['start at the waterfall', 'turn left at the mountain', 'compass', 'flashlight', 'shovel']
“We did it! The treasure chest is open!” I scream with joy! Boots and I look at the sparkling treasure inside, and we jump with excitement. “Thank you for helping us, amigos!”
The End of the Adventure
We found the treasure, and it was all thanks to the amazing JavaScript array methods: map
, filter
, find
, push
, sort
, splice
, reduce
, some
, every
, and concat
. We used them all to solve problems, overcome obstacles, and reach our goal.
Thanks for joining me on this wonderful adventure! You helped me every step of the way, and now we have the treasure! 🎉
Subscribe to my newsletter
Read articles from Prajwal Mhaskar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
