Mastering Array Methods with Uncle Ji!

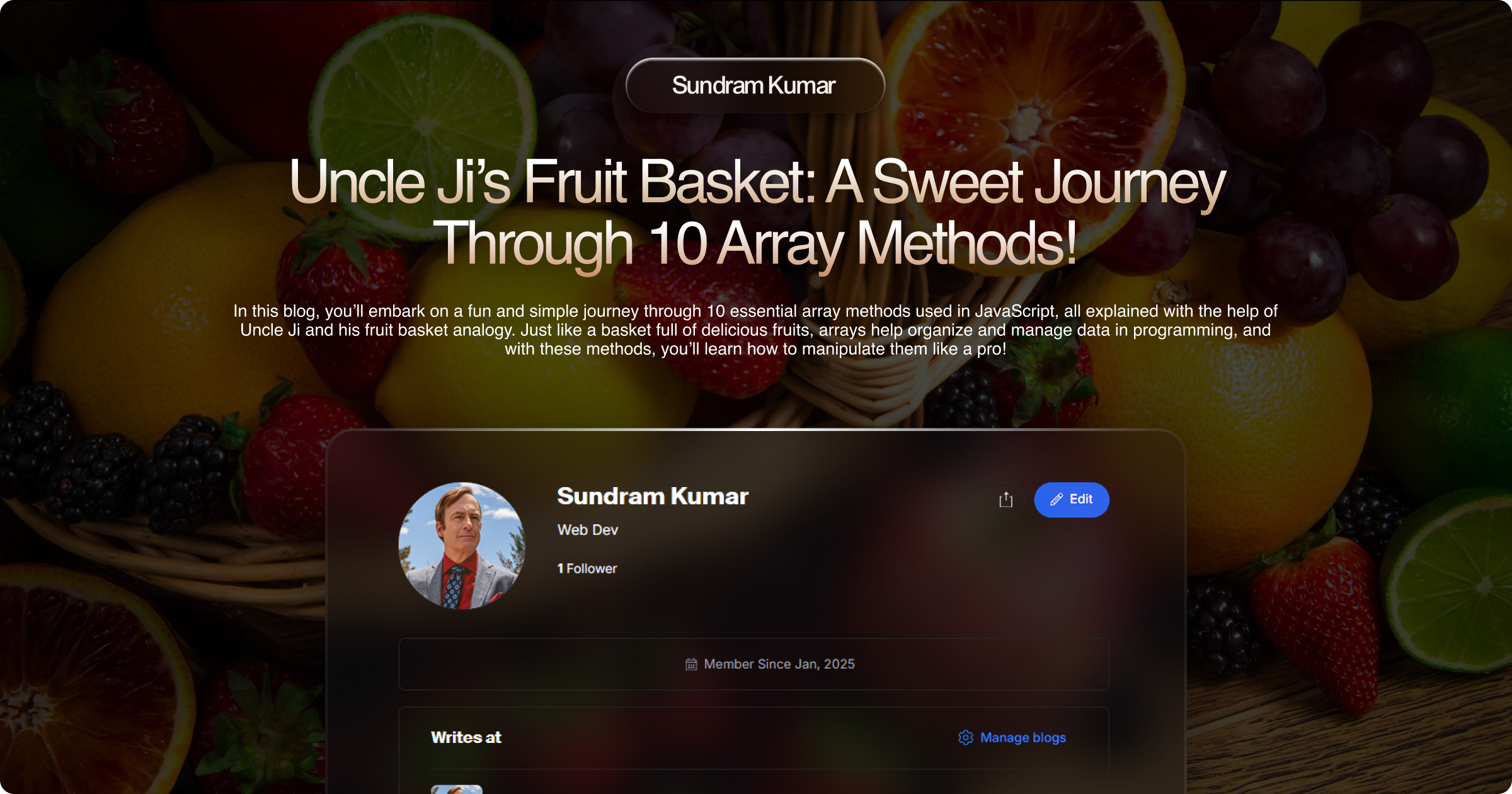
In a small town in India, where the streets are always bustling with the aroma of fresh samosas and the sound of rickshaws, there was a wise old man named Uncle Ji. He owned a little sweet shop and loved telling stories to anyone who passed by. One day, a curious young boy, Ravi, came to him with a question.
“Uncle Ji, what is an array, and how can I use it in my work?” Ravi asked, scratching his head.
Uncle Ji smiled. “Ah, arrays! They’re like a basket of fruits. Just like how you can organize different fruits in a neat row, arrays help you organize data in a computer.”
“But Uncle Ji,” Ravi continued, “What if I want to do something specific with my basket of fruits? Like picking one fruit or adding more?”
“Ah, now you’re asking the right questions! Let me teach you 10 important tricks or ‘methods’ to handle your basket of fruits, or as the computer world calls it, an array,” Uncle Ji replied with excitement.
1. push() – Adding Fruits to the Basket:
Uncle Ji picked a shiny mango and added it to the basket. "This, Ravi, is called push. Just like adding mangoes to your basket, you can add items to your array with this method."
Ravi’s eyes widened. “Oh! So, it’s like adding fruits to the basket!”
Example:
basket = ['apple', 'banana'];
basket.push('mango');
console.log(basket); // ['apple', 'banana', 'mango']
2. pop() – Removing Fruits from the Basket:
Uncle Ji picked a ripe banana and tossed it aside. “This is pop. It removes the last fruit from the basket, just like taking the last mango from your pile!”
Ravi laughed. "That’s easy!"
Example:
basket = ['apple', 'banana', 'mango'];
basket.pop();
console.log(basket); // ['apple', 'banana']
3. shift() – Taking the First Fruit Out:
“What if I need the first fruit?” Ravi asked.
Uncle Ji nodded. “shift does just that! It removes the first item, just like taking the first apple from a fresh basket.”
Example:
basket = ['apple', 'banana', 'mango'];
basket.shift();
console.log(basket); // ['banana', 'mango']
4. unshift() – Adding Fruits to the Front:
“Now, if I want to add a fruit right at the front?” Ravi asked.
Uncle Ji’s eyes twinkled. “That’s unshift! It places a fruit at the beginning, just like adding a sweet pomegranate to the front of the basket.”
Example:
basket = ['banana', 'mango'];
basket.unshift('pomegranate');
console.log(basket); // ['pomegranate', 'banana', 'mango']
5. indexOf() – Finding a Specific Fruit:
“What if I want to find a specific fruit?” Ravi asked.
Uncle Ji grinned. “That’s where indexOf helps. It tells you the position of the fruit in your basket.”
Example:
basket = ['apple', 'banana', 'mango'];
let index = basket.indexOf('banana');
console.log(index); // 1
6. .reverse()
– Changing the Order of Fruits:
“Sometimes, you might want to mix things up,” Uncle Ji said, as he shook the basket playfully. “reverse helps you change the order of the fruits, just like flipping the basket upside down!”
Example:
basket = ['apple', 'banana', 'mango'];
let reversedBasket = basket.reverse();
console.log(reversedBasket); // ['mango', 'banana', 'apple']
7..length
– Finding the Number of Fruits in the Basket:
Uncle Ji smiled and said, “Ravi, imagine your basket of fruits is like your array. The .length
method tells you how many fruits are in that basket—whether they are apples, bananas, or mangoes!”
Ravi’s eyes lit up. “Ah! So, if I want to know how many fruits I have in my basket, I just use .length
?”
“Exactly!” Uncle Ji nodded. “It counts the total number of items in the array.”
Example:
Let’s say you have a basket filled with fruits:
basket = ['apple', 'banana', 'mango'];
console.log(basket.length); // 3
8. .includes()
– Checking if a Fruit Exists in the Basket:
Ravi smiled, “But Uncle Ji, what if I just want to check if a fruit is in the basket?”
“That’s where includes comes in,” said Uncle Ji. “It tells you if the fruit is there.”
Example:
basket = ['apple', 'banana', 'mango'];
let hasMango = basket.includes('mango');
console.log(hasMango); // true
9. .slice()
– Picking a Part of the Basket:
“Sometimes, you only want a few fruits, not the entire basket,” Uncle Ji said, picking just a handful of fruits. “This is slice. It picks a part of the basket.”
Ravi grinned, "Like when I just want to grab a couple of bananas for a snack!"
Example:
basket = ['apple', 'banana', 'mango'];
let slicedBasket = basket.slice(1, 2);
console.log(slicedBasket); // ['banana', 'mango']
10. .sort()
– Organizing Your Fruits:
Uncle Ji was watching Ravi as he sorted through his fruits. “Sometimes, you want to arrange your fruits in a specific order, whether it's by size or by sweetness. That’s where .sort()
comes in—it helps you sort your array in either ascending or descending order!”
Ravi's eyes lit up. “Ah! So .sort()
is like organizing the fruits in order, just like arranging them in the basket by size or color?”
“Exactly!” Uncle Ji replied with a smile. “You can use it to put everything in order, whether you want numbers from smallest to largest or words in alphabetical order.”
How It Works:
The
.sort()
method arranges the elements in an array.It sorts alphabetically for strings and numerically for numbers by default, but it can also be customized.
Example: Sorting Strings Alphabetically:
Let’s say you have a basket with some fruits in a random order:
basket = ['mango', 'apple', 'banana'];
basket.sort();
console.log(basket); // ['apple', 'banana', 'mango']
Here’s what happens:
.sort()
arranges the fruit names in alphabetical order from 'apple' to 'mango'.
Conclusion:
Ravi learned from Uncle Ji how arrays are like a basket of fruits—organized and easy to manage with the right methods. Uncle Ji taught him 10 key tricks like .push() for adding, .pop() for removing, .reverse() for flipping, and more. By the end, Ravi understood how these methods help you organize and manipulate data just like arranging fruits in a basket.
Moral of the Story: Arrays are simple to handle with the right tools. Whether adding, removing, or checking items, these array methods make coding fun and easy—just like managing your favorite fruits! Happy coding! 🍎🍌🍍
Subscribe to my newsletter
Read articles from Sundram Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
