10 JavaScript Array Methods Youโve Probably Never Used ( But Should ! )
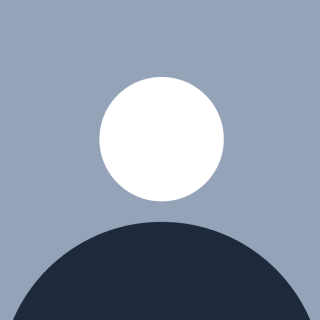

JavaScript arrays are a fundamental part of the language, and as developers, we use them constantly. ๐ Most of us are familiar with common array methods like map()
, filter()
, and reduce()
. But did you know thereโs a whole treasure trove of lesser-known array methods that can make your life as a coder much easier? ๐ต๏ธโโ๏ธ
In this article, weโll dive into 10 powerful, yet often overlooked, JavaScript array methods that can take your coding skills to the next level. ๐ฅ From simplifying nested data to modifying arrays like a pro, youโre about to discover some hidden gems!
Ready to level up your array game? Letโs get started! ๐
1๏ธโฃ Array.flatMap()
โ Nested Arrays Ko Flat Karna ๐
flatMap()
is a powerful JavaScript method that combines map()
and flat()
. Agar aap kisi array ke elements modify karna chahte ho aur result me nested arrays ban rahe hain, then flatMap()
best option hai! Yeh map()
ki tarah kaam karta hai but ek level tak flatten bhi karta hai.
So, for both transformation and flattening in one step, flatMap()
is perfect! ๐๐
๐ฅ Example with flatMap()
:
Arjun was reading a magical book where each page had an adventure with its sub-hints. Agar Arjun chahta ki sab hints ek saath ikatha ho, toh map()
use karne se nested arrays milte. But flatMap()
se woh sare hints ek flat list me aa jate, jo dekhna aur organize karna easy bana deta.
So, flatMap()
made his clue search much simpler and more efficient! โจ
const pages = [
{ adventure: "Jungle", hints: ["Right turn", "Cross river"] },
{ adventure: "Mountain", hints: ["Left turn", "Climb mountain"] }
];
const allHints = pages.flatMap(page => page.hints);
console.log(allHints);
// Output: ["Right turn", "Cross river", "Left turn", "Climb mountain"]
Is tarah, flatMap()
ne Arjun ko sab hints ko ek hi list mein arrange karne mein madad ki.
2๏ธโฃ Array.with()
โ Kisi Bhi Index Ka Value Change Karna
The Array.with()
method in JavaScript is used to create a new array by modifying the value at a specific index. It allows you to "set" a new value at a particular position in the array while keeping the other elements intact. ๐ ๏ธโจ
Syntax:
javascriptCopyEditarr.findIndex(callback(element, index, array), thisArg)
Explanation: The Array.with()
method takes two arguments:
The index where you want to modify the value. ๐
The new value that you want to set at that index. ๐
๐ฅ Example with Array.with()
:
you're managing an emoji collection and you have an array of emojis representing different feelings. Ek din, aap decide karte ho ki ek specific index par emoji ko update karna hai taaki aapka mood aur accha express ho sake.
Before: ['๐', '๐ข', '๐ก', '๐', '๐']
You want to change the emoji at index 2
(which is ๐ก) to ๐
to reflect that you're no longer angry.
let emojis = ['๐', '๐ข', '๐ก', '๐', '๐'];
let updatedEmojis = emojis.with(2, '๐
'); // Changing ๐ก to ๐
console.log(emojis); // ['๐', '๐ข', '๐ก', '๐', '๐'] (Original array)
console.log(updatedEmojis); // ['๐', '๐ข', '๐
', '๐', '๐'] (Updated array)
ho gaya ji aapka mood achha badal diya Array.with()
ne 2 index
ko khoshi ๐
se
3๏ธโฃ Array.copyWithin():
Ek Hi Array Me Data Shift Karna ๐ญ
The Array.copyWithin()
method allows you to copy and move elements within the same array without
changing its length. It modifies the original array. โก
To samjo kam kaise karta hai ?
Yeh method kisi bhi existing array ke elements ko ek naye position par copy karta hai, bina naye elements add kiye. Matlab, aap kisi bhi index ke elements ko move kar sakte ho, lekin array ki length same rahegi. ๐๐ This method modifies the array in place and does not create a new array. ๐
target ๐ฏ: Where to paste the copied elements.
start ๐: Where to start copying from.
end โ: Where to stop copying (optional)๐
๐ฅ Example: Movie Tickets ๐ฟ
a group of friends buying movie tickets. Unko ek row me 5 seats mili.
๐ช A (Rahul) | ๐ช B (Priya) | ๐ช C (Aman) | ๐ช D (Neha) | ๐ช E (Sahil)
Now, Aman kehata hai, "Let's shift our first 3 seats to the right!"
let seats = ['Rahul', 'Priya', 'Aman', 'Neha', 'Sahil'];
seats.copyWithin(2, 0, 3); // Copy Rahul, Priya, Aman -> Start at index 2
console.log(seats);
// Output: ['Rahul', 'Priya', 'Rahul', 'Priya', 'Aman']
Lo ji, copyWithin()
ne seats ko shift kar diya! ๐ญ
๐ช A (Rahul) | ๐ช B (Priya) | ๐ช C (Rahul) | ๐ช D (Priya) | ๐ช E (Aman)
๐ Notes:
copyWithin(target, start, end)
โtarget
se lekarstart
aurend
ke data ko shift karta hai.Ye method original array modify karti hai.
4๏ธโฃ ๐ JavaScript Array.flat()
: Chhoti Chhoti Arrays Ko Ek Banane Ka Tarika
The Array.flat()
method in JavaScript flattens nested arrays into a single-level array, making data easier to handle. ๐
Agar array ke andar arrays hain, to flat()
unko ek level tak flat kar deta hai. Yeh method data ko simple aur manageable banata hai. ๐
Itโs great for simplifying complex data structures quickly! ๐
๐ฅExample: Packing Luggage for a Trip ๐
you're packing for a trip, and your suitcase has multiple compartments with smaller bags inside. Ab aap soch rahe ho ki sab cheezon ko ek main section me rakhna hai, taaki jab zarurat ho, sab kuch easily access ho sake. It's just like using Array.flat()
to convert nested arrays into a single-level array, making it easier to manage and access everything! ๐๐ฆ
let luggage = [['Shirt', 'Jeans'], ['Shoes', ['Socks', 'Belt']], 'Sunglasses'];
let packedLuggage = luggage.flat(2); // Flatten up to 2 levels
console.log(packedLuggage);
// Output:['Shirt', 'Jeans', 'Shoes', 'Socks', 'Belt', 'Sunglasses'];
Now, all your items are in one place instead of being scattered in different compartments! ๐
5๏ธโฃ๐ JavaScript Array.toSorted()
: Sorting Made Easy !
The Array.toSorted()
method in JavaScript creates a new array that is sorted without modifying the original array. ๐โจ
Jab aapko apne array ko sort karna ho, lekin original array ko bina modify kiye, tab toSorted()
ka use kar sakte ho. Yeh method sorted array return karta hai, aur original array unchanged rehti hai. ๐
๐ฅ Example: Sorting Books in a Library ๐
in a library where books are stacked in random order on the shelves. The librarian decides to sort them alphabetically to make it easier for readers. ๐
let books = ['JavaScript Basics', 'React for Beginners', 'Node.js in Action', 'CSS Guide'];
let sortedBooks = books.toSorted(); // Sort alphabetically without changing the original array
console.log(sortedBooks);
// ๐Output:['CSS Guide', 'JavaScript Basics', 'Node.js in Action', 'React for Beginners']
Now, the books are sorted alphabetically, but the original stack of books remains as it was! ๐โจ
6๏ธโฃ๐ JavaScript Array.fromAsync()
: Convert Asynchronous Data to Arrays !
The Array.fromAsync()
method in JavaScript (which is not a built-in method) creates an array from asynchronous data, allowing you to easily handle async operations and convert them into arrays. ๐๐ป
Jab aap asynchronous data ke saath kaam kar rahe hote ho, Array.fromAsync()
aapko us data ko easily array mein convert karne ka option deta hai. Yeh async operations ko efficiently handle karta hai. โณ๐
Itโs a great tool for working with promises or async operations and turning them into usable arrays quickly ๐
Note: Array.fromAsync()
is not part of the standard JavaScript API, but you may encounter it in custom implementations or third-party libraries designed to handle asynchronous operations when converting array-like objects. Below is an example where you can create your own Array.fromAsync
function.
๐ฌExample: Collecting Messages from a Chat App ๐ฌ
you are chatting with a friend, and messages are coming in asynchronously. You want to collect them into a list to display later, but since theyโre coming one by one, you need to convert them into an array as they arrive. ๐ฒ
async function getMessages() {
let messageStream = async function*() {
yield 'Hey!';
yield 'How are you?';
yield 'Letโs catch up soon!';
};
let messages = await Array.fromAsync(messageStream());
console.log(messages);
}
getMessages();
// Output: ['Hey!', 'How are you?', 'Letโs catch up soon!']
the chat messages are collected asynchronously and converted into a nice array without missing any! ๐ฌโจ
note 1 : he
yield
acts like areturn
keyword, but instead of ending the function, it "pauses" and gives a value, letting the function continue later
7๏ธโฃ๐ JavaScript toLocaleString()
: Format Your Data for Different Locales !
The toLocaleString()
method in JavaScript converts a value (like a number or date) into a string based on the local formatting rules. ๐
Yeh method kisi bhi value ko, jaise number ya date ko, local language aur region ke hisaab se format karke string me convert karta hai. Isse aap apne data ko local preferences ke according show kar sakte ho. ๐ฎ๐ณ๐บ๐ธ
Itโs a handy tool for displaying data in a user-friendly format according to the user's locale! ๐
locales ๐: (Optional) A string or array of strings representing the locales to use.
options โ๏ธ: (Optional) An object to customize the formatting
๐ฌExample: Displaying Today's Date in Different Countries ๐
manlo Ki aap bana rahe ho ek website and that shows the current date to users from different countries, and you want to display the date in the local format. Letโs check how the date looks for users in the US and Japan. ๐๏ธ
let today = new Date();
console.log(today.toLocaleString('en-US')); // US format (MM/DD/YYYY)
console.log(today.toLocaleString('ja-JP')); // Japan format (YYYY/MM/DD)
// Output: 2/3/2025 (US)
// Output: 2025/02/03 (Japan)
jaise ki aap dekha sakte ho , the date format changes based on the locale! In the US, itโs MM/DD/YYYY, while in Japan itโs YYYY/MM/DD. ๐บ๐ธ๐ฏ๐ต
8๏ธโฃ ๐ JavaScript Array.findIndex()
: Find the Position Like a Detective!๐
The Array.findIndex()
method in JavaScript returns the index of the first element that satisfies a given condition. If no element matches, it returns -1
. ๐ต๏ธโโ๏ธ
callback: A function that runs for each element to check if it matches the condition.
thisArg: (Optional) Value to use as
this
when executing the callback.
Example: Finding the First Failing Student in a Class ๐
"Manlo, school ke exams ho gaye aur teacher marks check kar rahe hain. Now, they want to find out kaun-kaun fail hua hai (scored below 40)!
let students = [
{ name: 'John', marks: 85 },
{ name: 'Priya', marks: 92 },
{ name: 'Aman', marks: 35 }, // Failing student
{ name: 'Neha', marks: 76 }
];
let failingIndex = students.findIndex(student => student.marks < 40);
console.log(failingIndex); //Output: 2
The first failing student is at index 2
, so the method returns 2
.
9๏ธโฃ๐ JavaScript Array.prototype
: The Blueprint of All Arrays!
In JavaScript, Array.prototype
is like a blueprint (prototype) that contains all built-in array methods (like map()
, filter()
, forEach()
, etc.). Every JavaScript array inherits from this prototype.
๐ฅExample: Adding a Custom Method to All Arrays!
Imagine if every array could automatically double its numbers without you doing anything extra! ๐ช๐ Well, with Array.prototype
, you can add a custom method to all arrays in your program. Letโs make our arrays bodybuilders! ๐๏ธโโ๏ธ
Array.prototype.double = function() {
return this.map(num => num * 2);
};
let numbers = [1, 2, 3, 4];
console.log(numbers.double()); // Output: [2, 4, 6, 8]
We're adding a custom method
double()
to theArray.prototype
.This means that now, all arrays will have the
double()
method, which multiplies each number by 2. ๐
Important Note:
Array.prototype
is great for extending the functionality of arrays, but be cautious when adding custom methods because it can affect all arrays in your program. It's best used when you're sure of your changes or in specific cases where it makes sense.This custom method is not a built-in array methodโit's a custom method added to the prototype for demonstration.
๐๐ JavaScript structuredClone()
: Deep Copy Your Arrays Like a Pro!
The structuredClone()
method in JavaScript creates a deep copy of an object or an array, meaning it duplicates all elements, including nested objects, without keeping any reference to the original. ๐
๐ Simple Example of structuredClone()
in JavaScript
manalo aapke pass ek to-do list hai๐, and you want to create a duplicate of it without affecting the original list.
let todoList = ['Buy Milk', 'Go to Gym', 'Study JavaScript'];
let newTodoList = structuredClone(todoList);
// Modifying the new list
newTodoList.push('Read a Book');
console.log(todoList); // ['Buy Milk', 'Go to Gym', 'Study JavaScript']
console.log(newTodoList); // ['Buy Milk', 'Go to Gym', 'Study JavaScript', 'Read a Book']
Here, structuredClone()
creates a deep copy, so modifying newTodoList
does not change the original todoList
. This method is perfect for duplicating arrays safely! ๐
๐ Why Use structuredClone()
?
Aap kahoge, 'Bhai, hum to programmer hain! Hmm... spread operator (...
) use karenge, Object.assign()
karenge, yeh kyun use karu?'
Par programmer ji, yeh sab sirf shallow copies banate hain! Matlab agar object me nested objects hain, toh still referenced rahenge. Aur agar copied object me koi change kiya, toh original object bhi dukhi ho sakta hai! ๐
.
๐น structuredClone()
isko solve karta hai by ensuring a complete deep copy, taaki original object safe rahe! ๐ฅ
Note:
structuredClone()
is a built-in JavaScript method, but it may not be available in older versions of browsers or Node.js. Always check compatibility before using it.Conclusion ๐
These hidden JavaScript array methods, like
flatMap()
,Array.with()
,copyWithin()
, andstructuredClone()
, can make your coding life much easier and more fun! Try them out and level up your array game! ๐
#chaicode
Subscribe to my newsletter
Read articles from sumit mokasare directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
sumit mokasare
sumit mokasare
Hi, I'm Sumit! A first-year BCA student passionate about full-stack development. I share my learning journey and coding concepts to help others grow with me.