Optimizing Payment Success: Leveraging Azure Durable Functions for Reliable Transactions
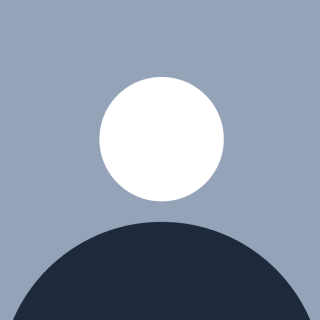
Why Payment Processing Needs Retries
Payment gateways (e.g., Stripe, PayPal) often face transient errors like:
Network timeouts
Rate limiting
Temporary bank API unavailability
Without retries, these failures lead to lost revenue and frustrated customers. But retries must be idempotent (no double charges) and stateful (track progress across attempts).
Enter Durable Functions: They provide built-in retry policies, state management, and compensation logic.
Architecture Overview
[Client] → [HTTP Starter] → [Orchestrator (Retry Logic)] → [Activity (Process Payment)]
│
└─→ [Human Approval (If retries fail)]
Step 1: Define the Orchestrator with Retry Policies
[FunctionName("ProcessPaymentOrchestrator")]
public static async Task RunOrchestrator(
[OrchestrationTrigger] IDurableOrchestrationContext context)
{
var paymentRequest = context.GetInput<PaymentRequest>();
// Retry configuration: 3 attempts with exponential backoff
var retryOptions = new RetryOptions(
firstRetryInterval: TimeSpan.FromSeconds(2),
maxNumberOfAttempts: 3)
{
BackoffCoefficient = 2,
Handle = ex => ex is PaymentException { IsTransient: true }
};
try
{
// Retry payment processing
await context.CallActivityWithRetryAsync<PaymentResult>(
"ProcessPaymentActivity",
retryOptions,
paymentRequest);
return "Payment succeeded!";
}
catch (PaymentException ex) when (!ex.IsTransient)
{
// Non-retriable error (e.g., invalid card)
await context.CallActivityAsync("NotifySupport", paymentRequest);
return "Payment failed (non-retriable).";
}
catch (Exception)
{
// After 3 failed attempts, escalate to human review
await context.CallActivityAsync("RequestHumanApproval", paymentRequest);
return "Payment pending manual review.";
}
}
Step 2: Implement the Payment Activity
[FunctionName("ProcessPaymentActivity")]
public static async Task<PaymentResult> ProcessPayment(
[ActivityTrigger] PaymentRequest request,
ILogger log)
{
// Idempotency check (prevent duplicate charges)
if (await _paymentRepository.IsDuplicate(request.IdempotencyKey))
throw new PaymentException("Duplicate request", isTransient: false);
try
{
// Call payment gateway (e.g., Stripe)
var result = await _stripeService.ChargeAsync(request);
await _paymentRepository.Save(result);
return result;
}
catch (StripeRateLimitException ex)
{
log.LogWarning($"Rate limited: {ex.Message}");
throw new PaymentException("Rate limited", isTransient: true);
}
catch (HttpRequestException ex)
{
log.LogError($"Network error: {ex.Message}");
throw new PaymentException("Network error", isTransient: true);
}
}
Step 3: HTTP Starter Function
[FunctionName("ProcessPayment_HttpStart")]
public static async Task<HttpResponseMessage> HttpStart(
[HttpTrigger(AuthorizationLevel.Function, "post")] HttpRequestMessage req,
[DurableClient] IDurableClient starter,
ILogger log)
{
var paymentRequest = await req.Content.ReadAsAsync<PaymentRequest>();
// Start orchestrator
string instanceId = await starter.StartNewAsync(
"ProcessPaymentOrchestrator",
paymentRequest);
log.LogInformation($"Started orchestration with ID = '{instanceId}'.");
return starter.CreateCheckStatusResponse(req, instanceId);
}
Key Features
1. Built-In Retry Policies
Exponential Backoff: Retries with growing delays (e.g., 2s → 4s → 8s).
Conditional Retries: Only retry transient errors (e.g., rate limits).
Max Attempts: Limit retries to avoid infinite loops.
2. Idempotency
Idempotency Key: Unique key per payment request to prevent duplicates.
Database Check: Verify if a payment was already processed.
3. Escalation to Human Review
- After 3 failed attempts, the orchestrator triggers a manual approval workflow.
Example:
[FunctionName("RequestHumanApproval")]
public static void RequestHumanApproval(
[ActivityTrigger] PaymentRequest request,
[SendGrid] out SendGridMessage message)
{
message = new SendGridMessage();
message.AddTo("support@company.com");
message.SetSubject($"Manual approval needed for payment {request.Id}");
message.SetFrom("noreply@company.com");
message.AddContent("text/html", $"Review payment: {request.Amount}");
}
Best Practices
1. Logging and Monitoring
Track retries and failures in Application Insights:
// host.json { "version": "2.0", "logging": { "applicationInsights": { "samplingSettings": { "isEnabled": true } } } }
2. Alerting
Set up alerts for:
PaymentException
(non-transient).Human escalation events.
3. Secure Credentials
- Store payment gateway keys in Azure Key Vault:
var stripeKey = await _secretClient.GetSecretAsync("StripeApiKey");
Real-World Use Case: E-Commerce Platform
Problem: A retail company lost 5% of revenue due to payment gateway timeouts during peak sales.
Solution:
Implemented Durable Functions with 3 retries (exponential backoff).
Reduced payment failures by 80%.
Integrated manual approval for high-risk transactions.
Outcome:
99.9% payment success rate during Black Friday.
Customer complaints dropped by 60%.
When to Avoid Durable Functions
Simple Retries: Use regular Functions with Polly for HTTP-triggered APIs.
Low-Volume Payments: Overhead may not justify Durable Functions’ cost.
Conclusion
Azure Durable Functions simplify building stateful, reliable payment systems with minimal code. By combining retries, idempotency, and human escalation, you can recover from transient errors while avoiding revenue loss.
Subscribe to my newsletter
Read articles from Anurag Dakalia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by