LLD - Composite Design Pattern

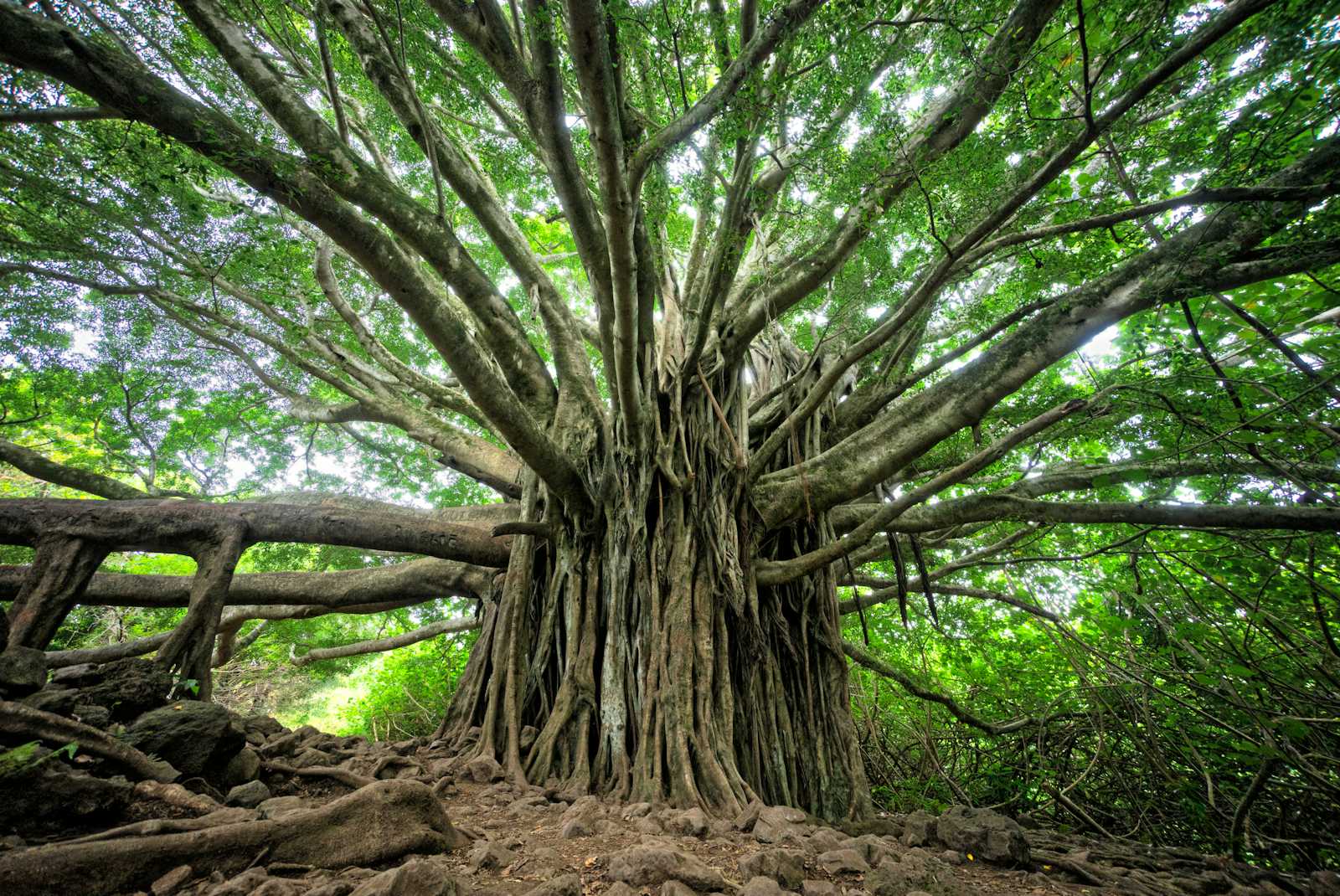
Composite Design Pattern
This is a structural pattern that allows you to compose objects into a tree-like structure and helps the client to treat individual objects (leaf) and groups of objects uniformly.
The composite pattern is based on two ideas-
Objects can contain other objects of the same type
Individual objects (leaf) and groups of objects should be treated the same way.
Blueprint of Composite Design Pattern
Component Interface or Abstract Class: This shares the common operations shared by both leaf and composite objects.
Leaf Class: This represents the individual object in the hierarchy that implements the Component interface and doesn’t have any child.
Composite Class: This class contains the child component and implements the methods in the Component interface.
Client: The client interacts with the objects using the component interface, treating leaf and composite objects uniformly.
Case Study - File System
A file system needs to manage a tree-like structure of files and folders. Each file is the leaf node containing data and each folder can contain multiple files and other folders.
Code - Without Composite Pattern
public class File {
private String name;
public File(String name) {
this.name = name;
}
public void display() {
System.out.println("File: " + name);
}
}
public class Folder {
private String name;
private List<Object> contents = new ArrayList<>();
public Folder(String name) {
this.name = name;
}
public void add(Object obj) {
contents.add(obj);
}
public void display() {
System.out.println("Folder: " + name);
for (Object obj : contents) {
if (obj instanceof File) {
((File) obj).display();
} else if (obj instanceof Folder) {
((Folder) obj).display();
}
}
}
}
Folders can store both File and Folder objects in List<Object>
Type checking & casting is required when iterating over the List. (Not a good design)
If we add a new type like SubFolder or ShortcutFile, we need to update
display()
logic in Folder
Code - With Composite Pattern
public interface FileSystemComponent {
void display();
}
public class File implements FileSystemComponent {
private String name;
public File(String name) {
this.name = name;
}
@Override
public void display() {
System.out.println("File: " + name);
}
}
public class Folder implements FileSystemComponent {
private String name;
private List<FileSystemComponent> contents = new ArrayList<>();
public Folder(String name) {
this.name = name;
}
public void add(FileSystemComponent component) {
contents.add(component);
}
public void remove(FileSystemComponent component) {
contents.remove(component);
}
@Override
public void display() {
System.out.println("Folder: " + name);
for (FileSystemComponent component : contents) {
component.display();
}
}
}
public class Main {
public static void main(String[] args) {
System.out.println("---------------- File System Structure ----------------");
File file1 = new File("Image.jpg");
File file2 = new File("Doc1.txt");
File file3 = new File("Doc2.txt");
Folder folder1 = new Folder("Personal Project");
folder1.add(file1);
folder1.add(file2);
Folder folder2 = new Folder("Project");
folder2.add(file3);
folder2.add(folder1);
folder2.display();
}
}
Here’s the Output
Benefits of Composite Pattern
Files and Folders share a common interface, simplifying operations
Eliminates type checks and casting
Recursive traversal is naturally handled
Subscribe to my newsletter
Read articles from Manish Pushkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manish Pushkar
Manish Pushkar
Software Engineer - Backend