Making XML Parsing Easy in Playwright API Tests with fast-xml-parser
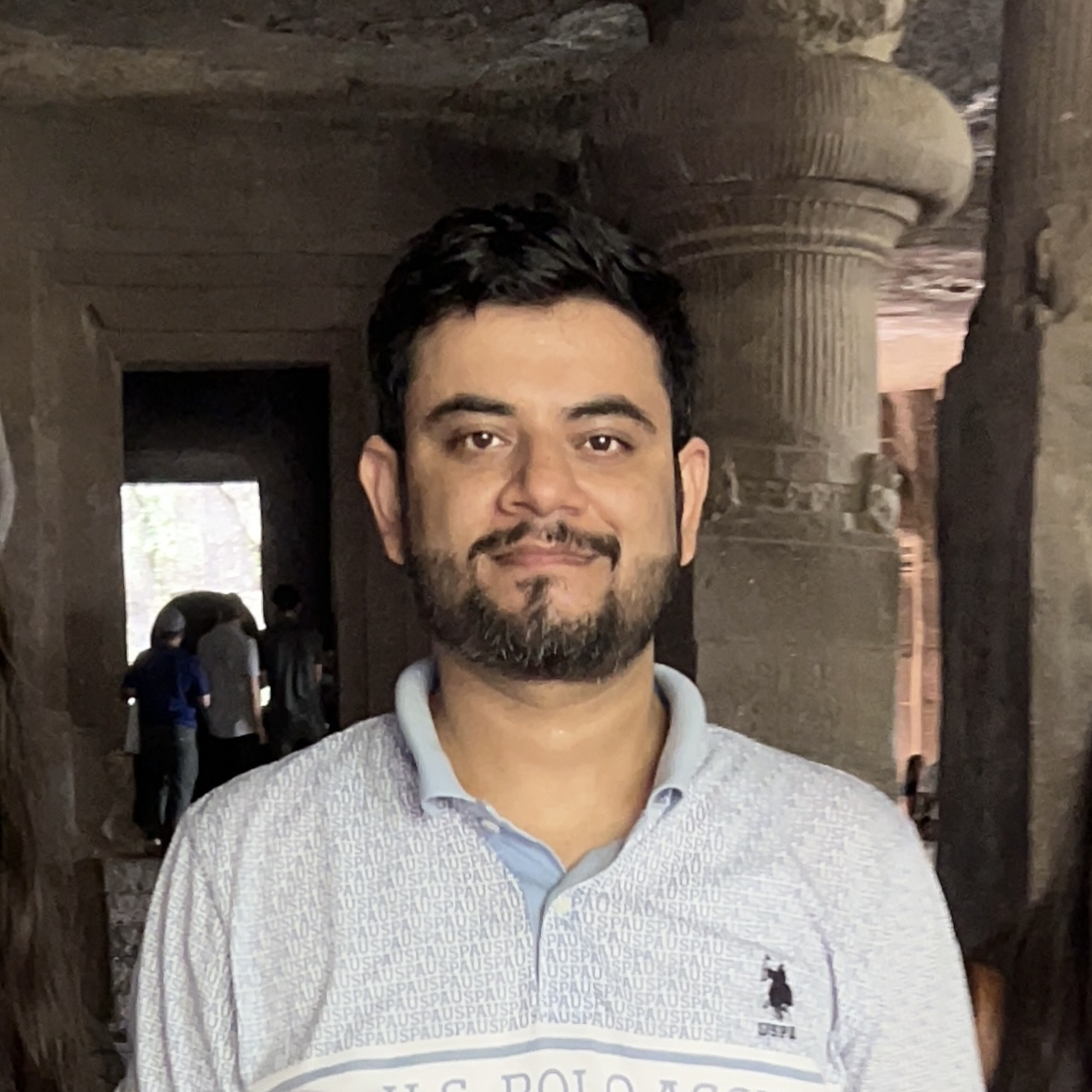

I recently started working on a project where the APIs accept requests and return responses in XML format. While exploring Playwright for API testing, I found that it provides a mechanism to handle requests and responses in JSON format, but lacks a straightforward way to manage XML requests and responses. This posed a challenge, but I discovered a solution using the fast-xml-parser library.
In this article, we will discuss the step-by-step process of building, parsing, and validating XML requests and responses.
Install and set-up playwright using typescript
https://playwright.dev/docs/getting-started-vscode
When automating API testing with Playwright, the focus is on sending requests and validating responses programmatically, rather than interacting with a web page through a browser. Therefore, it is not necessary to have browsers installed for API testing purposes.
Install fast-xml-parser library using npm
Open a terminal window in VS Code and run the following command to install fast-xml-parser library.
npm i fast-xml-parser
The fast-xml-parser
library has been installed and added to the dependencies section of the package.json
file.
{
"name": "learn-automation-playwright",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {},
"keywords": [],
"author": "",
"license": "ISC",
"devDependencies": {
"@playwright/test": "^1.50.0",
"@types/node": "^22.10.5"
},
"dependencies": {
"dotenv": "^16.4.7",
"fast-xml-parser": "^4.5.1"
}
}
Swagger document of the test API
Build XML request using XMLBuilder class
The XMLBuilder
class in the fast-xml-parser
library is used to convert JavaScript objects into XML format. It provides a way to build XML documents programmatically by defining the structure and content through JavaScript objects.
We are going to add a new pet object to the pet store.
Swagger reference of POST /pet API
Step 1: import XMLBuilder
class from fast-xml-parser
library
import { XMLBuilder } from "fast-xml-parser"
Step 2: Create a JavaScript object of Pet.
// Pet javascipt object
const Pet = {
"Pet": {
"id": 12345,
"Category": {
"id": 1,
"name": "BullDog"
},
"name": "Pixie",
"photoUrls": [{
"photoUrl": "string"
}],
"tags": [
{
"Tag": {
"id": 1,
"name": "PixieTheBullDog"
}
}
],
"status": "available"
}
}
Step 3: Create an XmlBuilderOptions
object. XmlBuilderOptions
is an interface in the fast-xml-parser
library that allows customization of the behavior of the XMLBuilder
class when converting JavaScript objects to XML. We are using the format attribute, which formats the XML output with indentation.
const options = { format: true };
Step 4: Create a new object of the XMLBuilder
class with the given options. The XMLBuilder
class accepts an options object, which is of type XmlBuilderOptions
.
const builder = new XMLBuilder(options);
Step 5: Convert the JavaScript object into XML.
let xmlDataStr = builder.build(Pet);
Create POST /pet api test using playwright
Step 1: Create a header object that specifies the response should be in XML format, while the content type of the request payload is also XML.
const header = {
'Accept': 'application/xml',
'Content-Type': 'application/xml'
}
Step 2: Since we will be making an HTTPS request, it is advisable to ignore HTTPS errors by using the test.use
block within the test.describe
function.
Step 3: Add a Playwright test to send the HTTP POST request, including the URL, header, and the payload we generated using the XMLBuilder
class above.
test.describe('Pet Store Test Suite', () => {
test.use({ ignoreHTTPSErrors: true, });
test('test', async ({ request }) => {
console.log('Request: ', xmlDataStr);
const url = 'https://petstore.swagger.io/v2/pet'
let response = await request.post(url, {
headers: header, data: xmlDataStr
});
console.log('Response: ', await response.text());
});
});
Parse the received XML response using XMLParser
Step 1: Create a reference to the XMLParser class
const xmlParser = new XMLParser();
Step 2: Parse the response using the XMLParser.parse()
method, which will convert XML data into a JavaScript object. This method takes an XML string as input and parses it, allowing us to work with the data in a more convenient JavaScript object format. Let's store the output for future reference.
const xmlResponse = xmlParser.parse(await response.text());
Step 3: Extract the value of a specific field from the response. For example, get the Id of the Pet object that is created.
let petId = xmlResponse.Pet.id;
Other fields can also be extracted from the response by using the object's path, which lets you navigate through the structure to access specific data.
Source code
Here is the link to the GitHub repository containing the source code for the tests.
Conclusion
In summary, using the fast-xml-parser
library with Playwright makes handling XML requests and responses in API testing much easier. By using XMLBuilder
and XMLParser
, one can efficiently create, parse, and check XML data in the tests. This method simplifies working with XML in Playwright and improves the effectiveness of API testing.
Reference
https://github.com/NaturalIntelligence/fast-xml-parser
https://github.com/NaturalIntelligence/fast-xml-parser/blob/master/docs/v4/3.XMLBuilder.md
Subscribe to my newsletter
Read articles from Himanshu Soni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
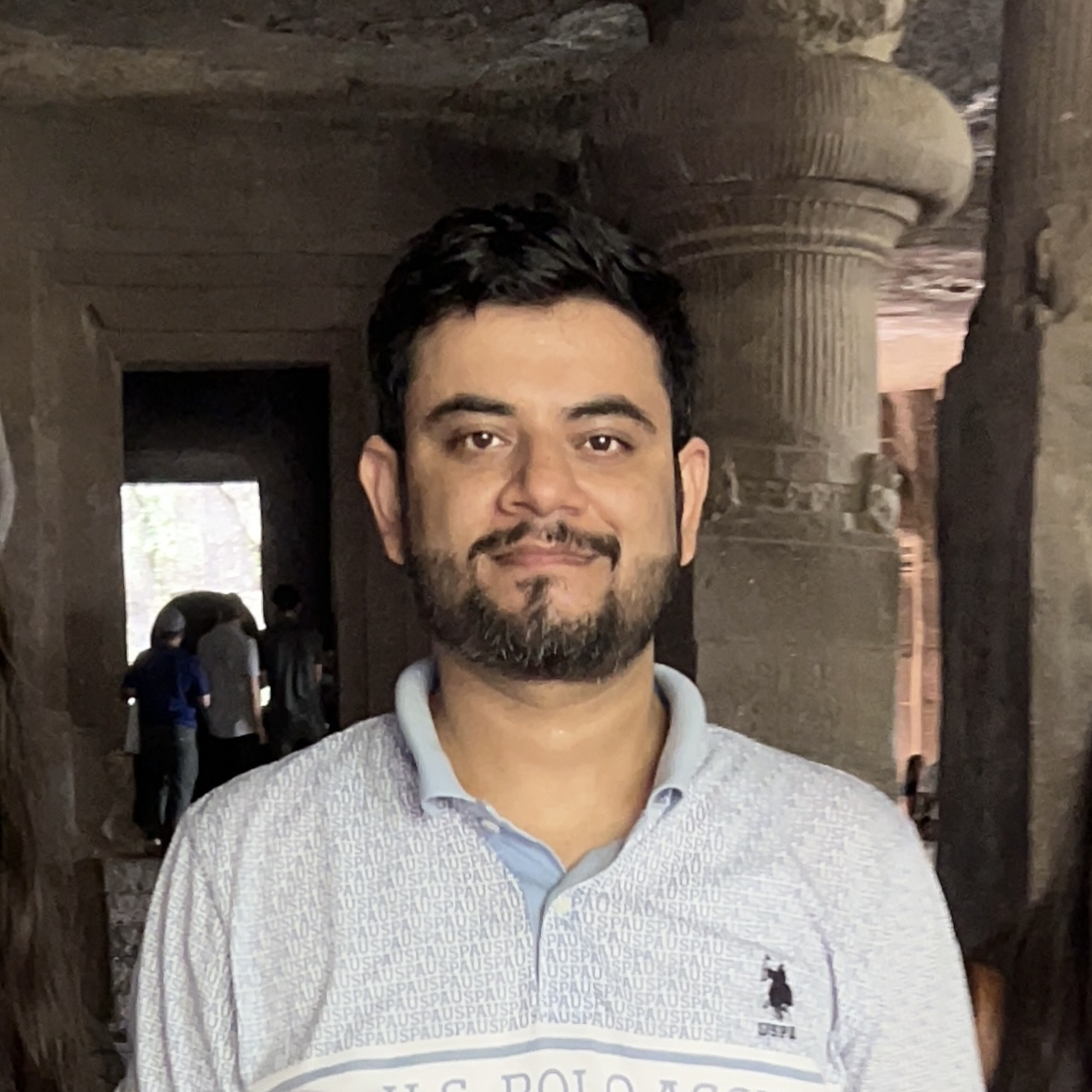
Himanshu Soni
Himanshu Soni
Seasoned QA Engineer with experience in Software Testing across Banking, Travel, and Telecom domains.