Debouncing vs. Throttling: What’s the Difference and When to Use Them

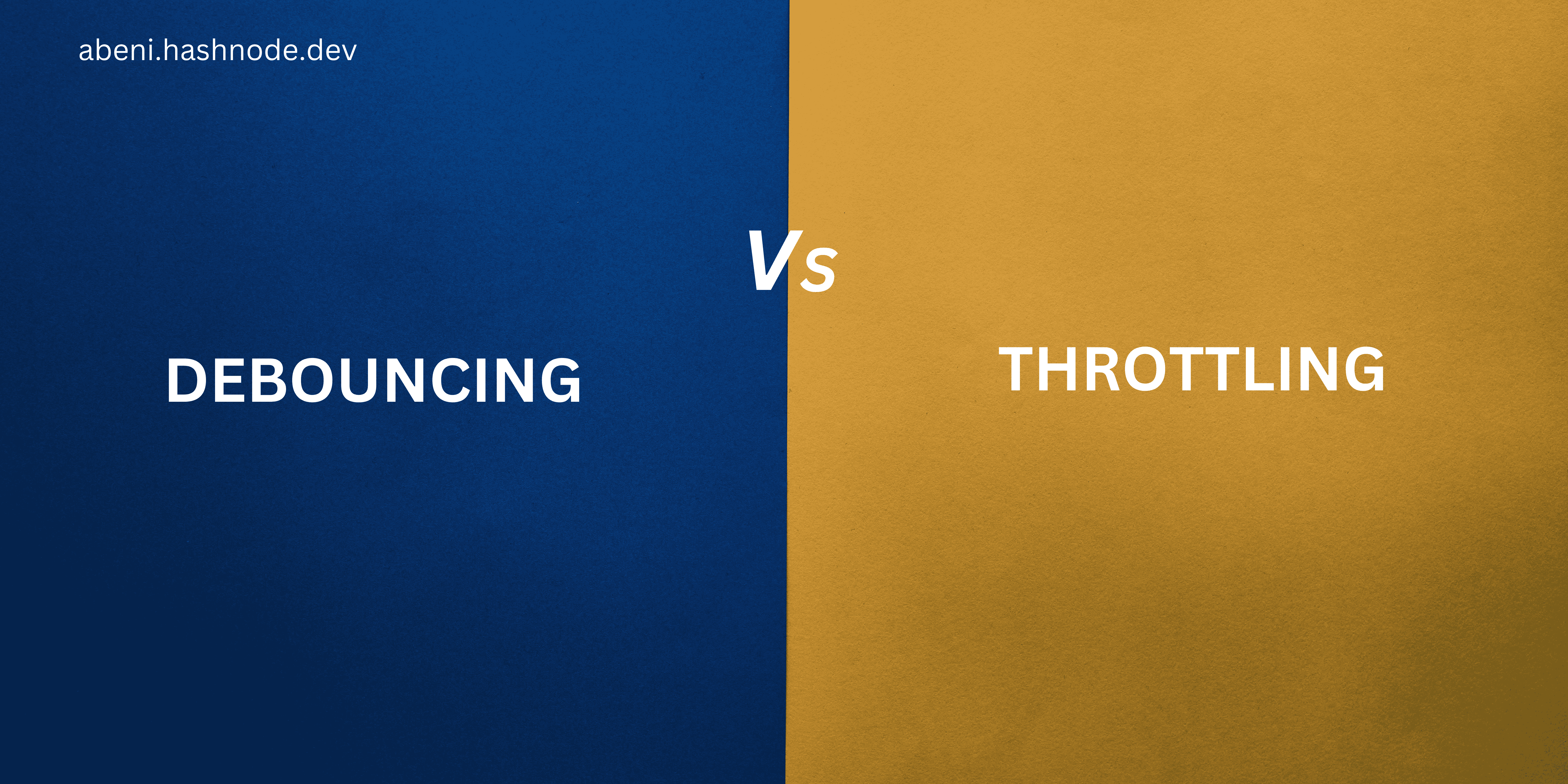
Ever wondered why your search bar doesn’t freak out with every keystroke or how your app stays smooth during rapid scrolling? Meet debouncing and throttling—two unsung heroes of web performance. Understanding these techniques can significantly enhance user experience and application efficiency. Let’s delve into the nuances of each method, explore their differences, and discover when to employ them effectively.
Definitions
At its core, debouncing is akin to a delayed reaction. Imagine you’re at a party, and every time you say something, your friend responds immediately. It can get overwhelming! Instead, if your friend waits a moment after you finish speaking before replying, it creates a more manageable conversation. In web development, debouncing ensures that a function is only executed after a specified period of inactivity. This is particularly useful for scenarios like search bars, where you want to wait until the user has paused typing before sending a request to the server.
On the other hand, throttling can be likened to a traffic light that allows cars to pass at regular intervals. Even if there’s a constant flow of vehicles, only a few can move through at a time. Throttling limits the execution of a function to a fixed interval, ensuring it runs at most once every specified duration. This is particularly beneficial for events that can occur frequently, such as scrolling or resizing windows, where continuous execution could degrade performance.
Key Differences
The primary distinction between debouncing and throttling lies in their timing and execution. Debouncing waits for a pause in user input before executing the function, thus preventing multiple calls in quick succession. In contrast, throttling allows the function to execute at regular intervals, regardless of how many times the event is triggered.
To illustrate:
Debouncing: If a user types rapidly in a search bar, the function to fetch search results will only trigger after the user stops typing for a predefined duration (e.g., 300 milliseconds).
Throttling: If a user scrolls down a webpage, the scroll event handler will execute at fixed intervals (e.g., every 200 milliseconds), ensuring that the function does not overwhelm the browser with too many calls.
Use Cases
Understanding when to use debouncing and throttling can make a significant difference in application performance:
Debouncing is ideal for:
Search Bars: To prevent excessive API calls while the user is typing.
Window Resizing: To avoid firing resize events multiple times while the user adjusts the window size.
Throttling is best suited for:
Scroll Events: To limit the frequency of event handling while a user scrolls through a page, ensuring smooth performance.
Button Clicks: To prevent multiple submissions of a form when a user clicks a button repeatedly.
Implementation
Here are simple code snippets to implement both techniques in modern web applications.
Debouncing Example
function debounce(func, delay) {
let timeout;
return function(...args) {
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(this, args), delay);
};
}
// Usage
const searchInput = document.getElementById('search');
searchInput.addEventListener('input', debounce(() => {
// Fetch search results
console.log('Fetching results...');
}, 300));
Throttling Example
function throttle(func, limit) {
let lastFunc;
let lastRan;
return function(...args) {
if (!lastRan) {
func.apply(this, args);
lastRan = Date.now();
} else {
clearTimeout(lastFunc);
lastFunc = setTimeout(() => {
if ((Date.now() - lastRan) >= limit) {
func.apply(this, args);
lastRan = Date.now();
}
}, limit - (Date.now() - lastRan));
}
};
}
// Usage
window.addEventListener('scroll', throttle(() => {
console.log('Scroll event triggered');
}, 200));
Performance Impact
Both debouncing and throttling play crucial roles in enhancing user experience and application performance. By minimizing unnecessary function calls, they reduce the load on the browser and server, leading to faster response times and smoother interactions. This ultimately results in a more enjoyable experience for users, who are less likely to encounter lag or unresponsiveness in the application.
So, next time you’re building a feature that deals with rapid user input, remember: debouncing and throttling are your best buddies. Use them wisely, and your app will thank you!
Subscribe to my newsletter
Read articles from Abenezer Teshome directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abenezer Teshome
Abenezer Teshome
MERN Stack & Next.js Enthusiast A computer science student sharing my development journey and connecting with fellow devs to grow together.