Creating a CI/CD Pipeline with GitHub Actions for a Web Application
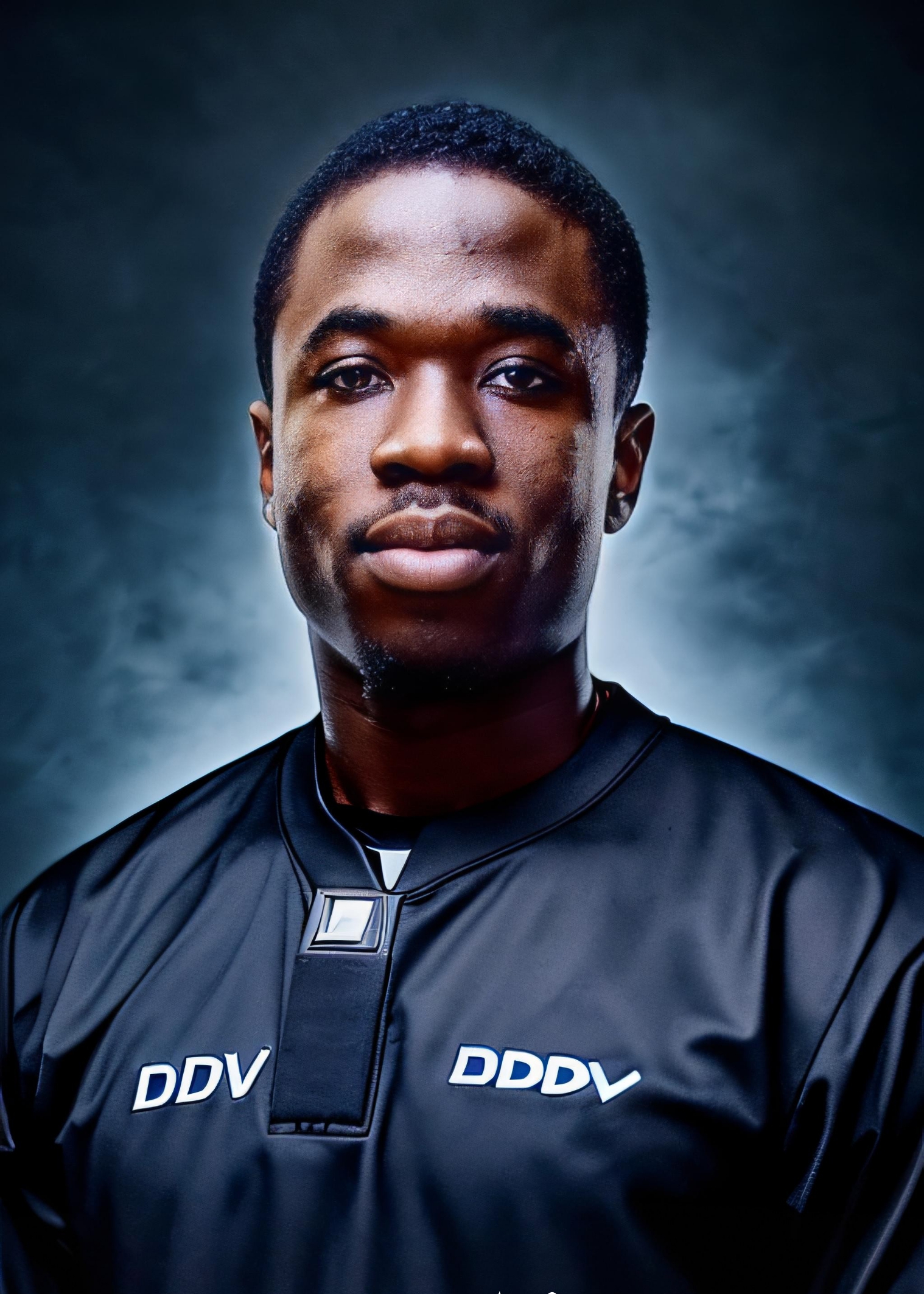

Introduction
Continuous Integration and Continuous Deployment (CI/CD) are essential for modern software development, enabling teams to automate testing, building, and deploying applications efficiently. A well-configured CI/CD pipeline reduces manual intervention, increases deployment speed, and ensures high software quality.
GitHub Actions is a powerful CI/CD tool that integrates seamlessly with GitHub repositories. It allows developers to automate workflows, run tests, and deploy applications using YAML configuration files. Compared to other CI/CD solutions like Jenkins and GitLab CI/CD, GitHub Actions is highly accessible, easy to configure, and requires minimal setup.
This guide provides a step-by-step approach to setting up a CI/CD pipeline using GitHub Actions. Readers will learn how to:
Automate testing, building, and deployment of a web application
Run tests in a GitHub Actions workflow
Deploy an application to a cloud provider such as AWS, GCP, or Vercel
Use GitHub Secrets to manage sensitive information
Automate versioning and release management
By the end of this tutorial, readers will have a fully functional CI/CD pipeline that automates the development workflow.
Prerequisites
Before getting started, ensure the following:
A basic understanding of Git and GitHub
A web application built with any framework (e.g., Node.js, Django, Flask) hosted in a GitHub repository
A cloud deployment account (AWS, GCP, Vercel, or Netlify)
Familiarity with YAML syntax (optional but beneficial)
Setting Up GitHub Actions in Your Repository
GitHub Actions workflows are stored in the .github/workflows/
directory within a repository. The workflow files define the jobs and steps required to automate tasks.
To create a new workflow:
Navigate to the GitHub repository.
Click on the Actions tab.
Select New Workflow and choose "Set up a workflow yourself".
Save the workflow file as
.github/workflows/ci-cd.yml
.
Writing a GitHub Actions Workflow File
A workflow file is written in YAML and defines automation processes triggered by specific GitHub events.
Setting Up the Workflow File
The following YAML snippet creates a GitHub Actions workflow named CI/CD Pipeline. It is triggered on pushes and pull requests to the main
branch.
name: CI/CD Pipeline
on:
push:
branches: [main]
pull_request:
branches: [main]
Adding Job for Automated Testing
The first job ensures that the application passes all tests before proceeding to the build phase.
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v4
- name: Set up Node.js
uses: actions/setup-node@v4
with:
node-version: '18'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
Explanation:
The
checkout
action fetches the latest code from the repository.The
setup-node
action installs Node.js version 18.The workflow installs dependencies with
npm install
and runs tests withnpm test
.
Adding Job for Building the Application
Once the tests pass, the application is built before deployment.
build:
runs-on: ubuntu-latest
needs: test
steps:
- name: Checkout code
uses: actions/checkout@v4
- name: Install dependencies
run: npm install
- name: Build application
run: npm run build
Explanation:
The
needs: test
directive ensures that the build job runs only if tests pass.The build command compiles the application for production.
Deploying to a Cloud Provider
Deployment options include AWS, GCP, Vercel, and Netlify. This guide focuses on deploying to Vercel.
Setting Up Deployment Environment
Create a Vercel account and link it to GitHub.
Retrieve the Vercel Token from the Vercel dashboard.
Store the token in GitHub Secrets:
Navigate to Repository Settings → Secrets and Variables → Actions.
Add a new secret named
VERCEL_TOKEN
and paste the token value.
Adding a Deployment Job
The following workflow deploys the application to Vercel:
deploy:
runs-on: ubuntu-latest
needs: build
steps:
- name: Checkout code
uses: actions/checkout@v4
- name: Install Vercel CLI
run: npm install -g vercel
- name: Deploy to Vercel
run: vercel --prod --token ${{ secrets.VERCEL_TOKEN }}
Explanation:
The
needs: build
directive ensures deployment only occurs after a successful build.The Vercel CLI is installed globally.
The application is deployed using
vercel --prod
, authenticated with the storedVERCEL_TOKEN
.
Automating Versioning and Release Management
GitHub Actions can automate versioning and releases using GitHub Releases.
Creating a Release Workflow
release:
runs-on: ubuntu-latest
needs: deploy
steps:
- name: Checkout code
uses: actions/checkout@v4
- name: Bump version and push tag
run: |
git config --global user.name "github-actions"
git config --global user.email "actions@github.com"
npm version patch
git push origin main --tags
- name: Create GitHub Release
uses: softprops/action-gh-release@v1
with:
token: ${{ secrets.GITHUB_TOKEN }}
Explanation:
The workflow increments the application version.
A new GitHub release is created automatically.
Testing the Pipeline
To verify the pipeline:
Make a commit and push it to the
main
branch.Navigate to the Actions tab in the GitHub repository.
Check if the workflow executes successfully.
Fix any errors using the logs provided by GitHub Actions.
Conclusion
This tutorial demonstrated how to configure a CI/CD pipeline using GitHub Actions. The workflow included:
Automating testing, building, and deploying a web application
Using GitHub Secrets for secure authentication
Deploying an application to Vercel
Automating versioning and release management
For further improvements, consider:
Adding Slack or email notifications for build failures
Implementing rollback strategies for failed deployments
Running security and performance tests in the CI pipeline
Implementing CI/CD best practices ensures efficient, automated, and reliable software delivery.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
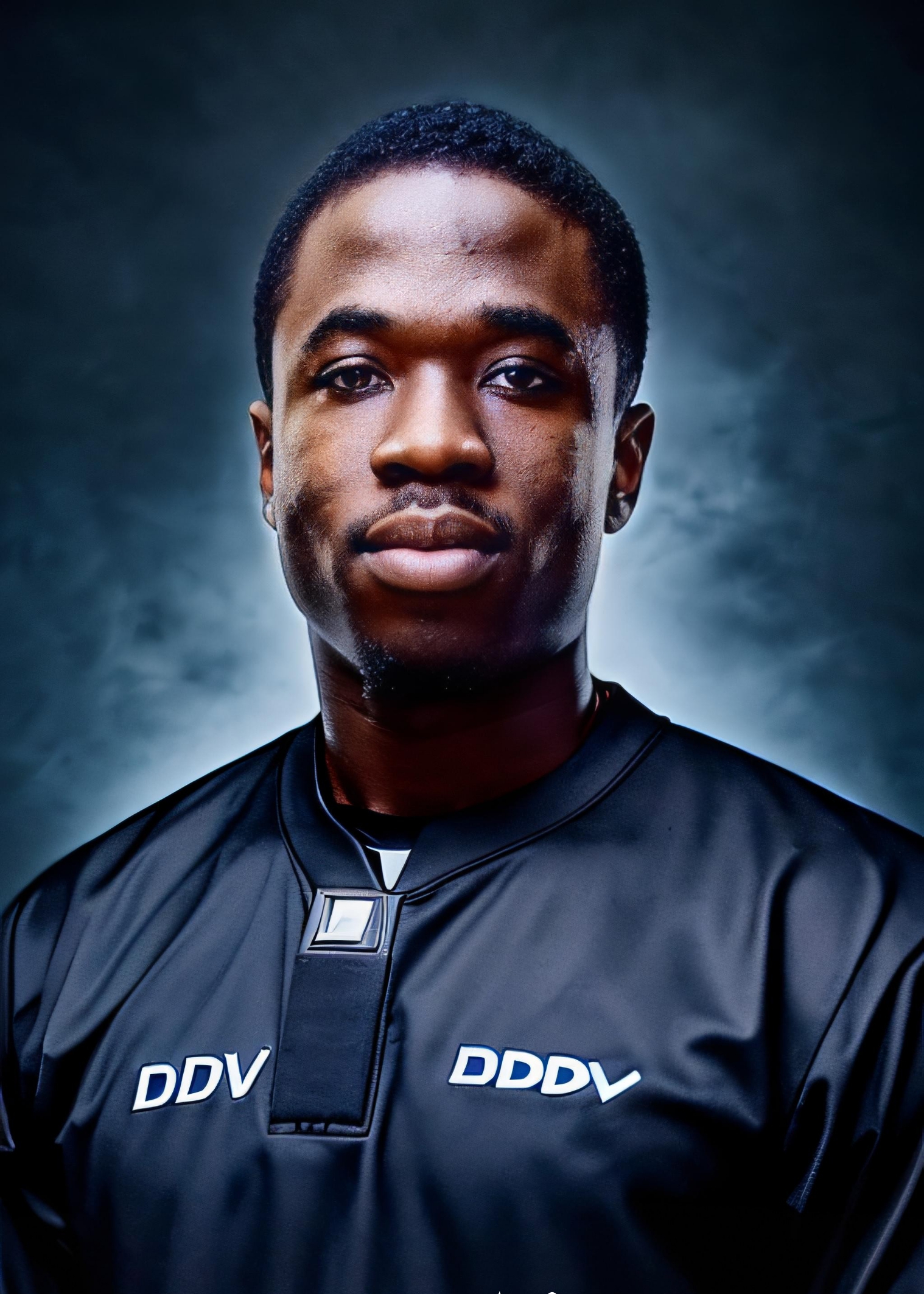
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.