Solving Mysteries with JavaScript: A Detective's Guide to Array Methods
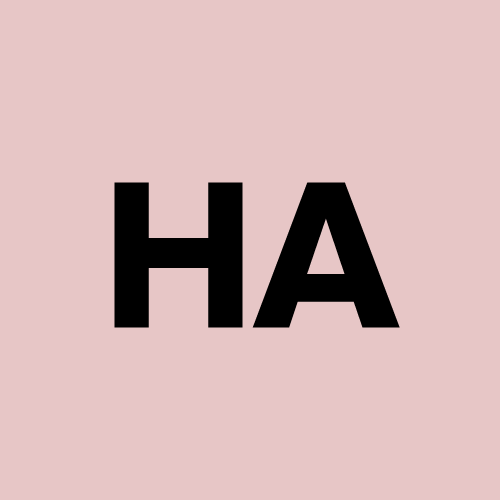
Table of contents
- Step 1: Listing the Guests
- Step 2: Who Left Early?
- Step 3: Finding the Suspects
- Step 4: Checking for Witnesses
- Step 5: Removing Unrelated Guests
- Step 6: Checking CCTV Footage
- Step 7: Checking If the Thief is Still There
- Step 8: Finding the Thief’s Last Actions
- Step 9: What Else Was Stolen?
- Step 10: Confronting the Thief
- Final Outcome: The Necklace is Found!
- Conclusion
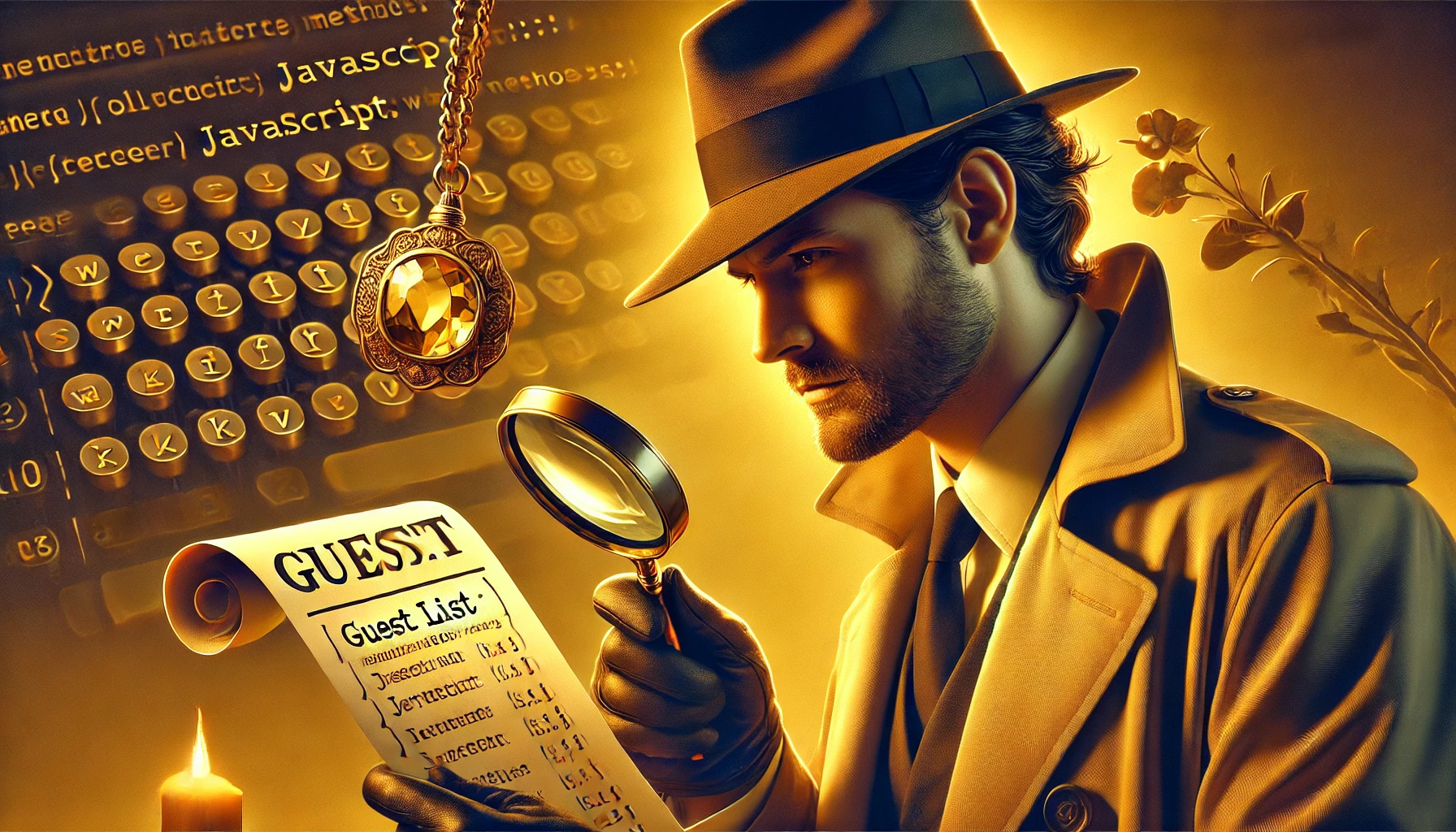
When Asha's gold necklace vanished at a family event, a complex mystery unfolded among many guests.
Step 1: Listing the Guests
Detective Ravi first noted down all the guests who attended the gathering.
Method Used: .push()
Ravi also added a latecomer to the list.
let guests = ["Amit", "Priya", "Rahul", "Sita", "Arun", "Neha", "Ramesh", "Kiran", "Raj", "Pooja"];
console.log("Guest List:", guests);
// Adding a latecomer
guests.push("Shyam");
console.log("Updated Guest List:", guests);
//[Updated Guest List] → Amit, Priya, Rahul, Sita, Arun, Neha, Ramesh, Kiran, Raj, Pooja, Shyam
What .push()
does?
It adds a new element to the end of an array.
Step 2: Who Left Early?
To narrow down suspects, Ravi checked who had left before the necklace went missing.
Method Used: .pop()
He removed the last guest who left early from the list.
let earlyLeavers = ["Rahul", "Kiran", "Shyam"];
let lastLeft = earlyLeavers.pop();
console.log("Last person who left early:", lastLeft);
//Last person who left early: Shyam
//[Updated List] → Rahul, Kiran
What .pop()
does?
It removes the last element from an array and returns it.
Step 3: Finding the Suspects
Ravi identified the guests who were near the necklace before it disappeared.
Method Used: .slice()
He extracted a portion of the guest list to focus on.
let suspects = guests.slice(2, 6);
console.log("Possible Suspects:", suspects);
//[Possible Suspects] → Rahul, Sita, Arun, Neha
What .slice()
does?
It extracts a part of an array without modifying the original array.
Step 4: Checking for Witnesses
Some guests witnessed suspicious activity near the jewelry box.
Method Used: .indexOf()
Ravi found the position of a key witness in the guest list.
let witness = "Priya";
let position = guests.indexOf(witness);
console.log("Witness found at position:", position);
//Witness found at position: 1
What .indexOf()
does?
It returns the index of the first occurrence of an element in an array.
Step 5: Removing Unrelated Guests
Some guests were busy eating food and couldn’t be suspects.
Method Used: .filter()
Ravi removed an innocent person from the suspect list.
let suspiciousGuests = suspects.filter(name => name !== "Sita");
console.log("More Focused Suspects:", suspiciousGuests);
//[Updated Suspects] → Rahul, Arun, Neha
What .filter()
does?
It creates a new array with elements that match a condition.
Step 6: Checking CCTV Footage
The CCTV camera near the jewelry box recorded a guest wearing a red kurta taking something.
Method Used: .find()
Ravi identified the first matching person in the footage.
let guestClothing = [
{ name: "Amit", outfit: "Blue Shirt" },
{ name: "Priya", outfit: "Red Kurta" },
{ name: "Arun", outfit: "Black T-shirt" }
];
let thief = guestClothing.find(guest => guest.outfit === "Red Kurta");
console.log("Thief Identified:", thief.name);
//Thief Identified → Priya
What .find()
does?
It returns the first element that matches a condition.
Step 7: Checking If the Thief is Still There
Ravi needed to confirm if the thief was still at the party.
Method Used: .includes()
He checked if the thief’s name was still in the guest list.
if (guests.includes(thief.name)) {
console.log("The thief is still at the party!");
} else {
console.log("The thief has already left.");
}
//Thief Still at Party? → ✅ Yes
What .includes()
does?
It checks if an element exists in an array and returns true
or false
.
Step 8: Finding the Thief’s Last Actions
Ravi checked the thief’s recent actions.
Method Used: .shift()
He removed the first recorded action from the list.
let lastActions = ["Drinking juice", "Talking to Arun", "Hiding something in bag"];
let firstAction = lastActions.shift();
console.log("First action removed:", firstAction);
console.log("Remaining actions:", lastActions);
//[Removed Actions] → Drinking juice
//[Updated Actions] → Talking to Arun, Hiding something in bag
What .shift()
does?
It removes the first element from an array and shifts everything left.
Step 9: What Else Was Stolen?
Apart from the necklace, another item was missing.
Method Used: .concat()
Ravi combined both missing item lists.
let missingItems = ["Necklace"];
let extraMissing = ["Gold ring"];
let totalMissing = missingItems.concat(extraMissing);
console.log("Total Missing Items:", totalMissing);
//[Total Missing Items] → Necklace, Gold ring
What .concat()
does?
It merges two or more arrays into one.
Step 10: Confronting the Thief
Ravi finally revealed the thief’s name to everyone.
Method Used: .join()
He created a sentence announcing the thief.
console.log("The thief is " + thief.name + "! Caught red-handed.");
//The thief is Priya! Caught red-handed.
What .join()
does?
It joins array elements into a single string.
Final Outcome: The Necklace is Found!
Thanks to Ravi’s smart use of arrays, the thief was caught, and Asha’s necklace was recovered.
Moral of the Story
Presence of mind and logic are stronger than deception!
Conclusion
This fun detective story helped us learn 10 useful JavaScript array methods:
.push()
– Add an item.pop()
– Remove the last item.slice()
– Extract part of an array.indexOf()
– Find an element’s position.filter()
– Remove unwanted elements.find()
– Locate the first match.includes()
– Check if an element exists.shift()
– Remove the first item.concat()
– Merge arrays.join()
– Convert an array into a sentence
Subscribe to my newsletter
Read articles from Hera Ahmad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
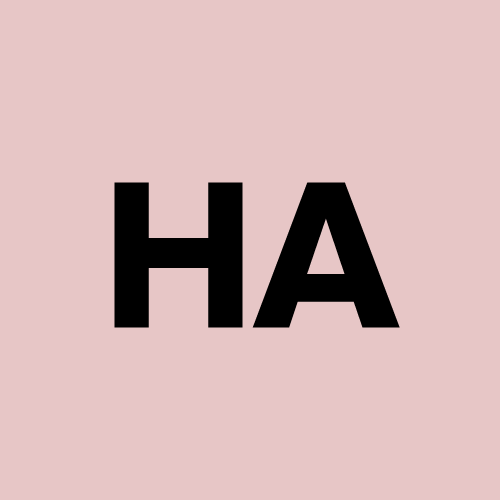