Tackling My Hydration Error and What I Learned
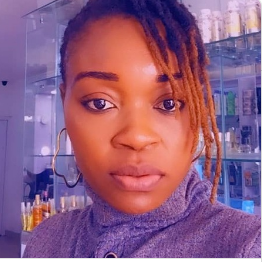
So far, the project I am working on has been quite an adventure. Last week, I ran into a Node version error, and I wrote about it and broke it down step by step in the simplest way possible. Before you could even say "Jack Robinson," boom, another error popped up: a hydration error! Honestly, I love these errors because they’re teaching me a lot.
Before I jump into how I fixed it, let’s first break down hydration properly. I want to make sure you get the full picture, kind of like walking you through it step by step for a better understanding.
Client-Side Rendering (CSR)
Client-side rendering happens right in your browser. When you visit a website, the server sends a basic HTML shell, and JavaScript (any framework) fills in the content, making it interactive. Once the page loads, any updates happen instantly without needing a full reload, only the parts that need interactivity or updates change. The first load can be a bit slow since the browser has to process JavaScript before showing anything. Also, search engines might struggle to read the content because the initial page is mostly empty, which can affect SEO.
With CSR, everything, buttons, animations, and data updates, happens in your browser, reducing pressure on the server. Popular frameworks like React, Vue, and Angular use CSR to make websites feel smooth and interactive.
Server-Side Rendering (SSR) & Hydration
Server-side rendering happens on a remote computer (the server). This means when you open a website, you immediately see a fully loaded page, no waiting for JavaScript to build it. That’s why SSR is great for SEO and fast first loads. Unlike CSR, where a blank page appears until React or any other framework is fully loaded, SSR pre-renders the content on request before sending it to the browser.
But what happens when you start clicking around? Instead of reloading the whole page, React uses hydration, a process where JavaScript takes control of the pre-loaded content and makes it interactive without the need to refresh everything.
Frameworks like Next.js give us the best of both worlds by supporting both server-side and client-side rendering.
Hydration
Hydration comes up when working with server-side rendered content. The client sends a request to the server for a page, and the server responds by sending the content without interactivity. Interactivity is added in the browser, and this process is called hydration. In a client-side application, the JS is received from the server and is responsible for populating the DOM. For server-side rendering, the JS on the client is not responsible for populating the DOM since the DOM is already populated with the content it gets from the server.
Why is Hydration Needed in SSR?
Hydration is needed in SSR because the HTML the server sends over is static, it doesn’t do anything interactive. Hydration is like the magic that turns that static page into a fully interactive one with React, so things like buttons work, input fields accept text, and the whole page comes to life. Without hydration, the only thing you get is a basic HTML page, that doesn’t respond to anything.
Let me walk you through an example. In CSR, the server sends an empty div like <div id="root"></div>, and then React builds the whole page in the browser, so you end up seeing something like <h1>Hello, World!</h1>.
In SSR, the server sends the HTML already filled in, like <div id="root"><h1>Hello, World!</h1></div>. Once the page loads, JavaScript runs, and React "hooks" into that existing HTML, adding interactivity like clicks and form submissions, making it come to life.
What Happens During Hydration?
During hydration, the server sends pre-rendered HTML to the browser before JavaScript has loaded. The React takes over by adding event listeners and other interactivity to the existing HTML. React doesn't reload the page it only changes what’s necessary for the updates and interactivity.
Hydration Problem
The problem happens when the HTML the server sent and the DOM React tries to build don’t match up. This can cause a few issues:
Content mismatches – The page might look different from React’s virtual DOM, leading to glitches or warnings.
Performance problems – Hydration can slow down the page because it uses JavaScript to make everything interactive, blocking the rendering process.
Timing issues – Some things, like fetching data or animations, might work better if they happen after hydration is done.
In server-side rendering (SSR), certain features like window, localStorage, and document don’t exist on the server side. If you try to use them in SSR, React can break. For example, using something like window.matchMedia() directly in SSR will cause issues because it only works on the client side.
To avoid breaking React in SSR, you can use hooks like useEffect. This ensures that client-specific features are only accessed after the page is loaded in the browser, not when the server renders the page. So, instead of trying to use window.matchMedia() directly in SSR, you can make sure the code only runs on the client side by wrapping it in useEffect. This way, you avoid errors and keep everything working smoothly.
Back to my Error and How I Fixed It
I used the suppressHudrationWarning and set it to true and applied it to the body, this is a way of telling React to ignore any mismatch between the server-rendered HTML and the client-rendered DOM for a specific element and its children.
SuppressHydrationWarning and the <body> Tag
When you use suppressHydrationWarning on the <body> tag, React won’t complain if the content from the server doesn’t match what React builds during hydration. Since the <body> tag holds almost everything on the page, this basically turns off mismatch warnings for the entire app. It’s useful if you expect small differences between the server and client but be careful, hiding warnings doesn’t fix the actual problem!
When to Use suppressHydrationWarning
Use suppressHydrationWarning when you know the content from the server will be different from what the browser renders, and you don’t want React to throw warnings. This is useful in cases like fonts or styles changing based on user settings, content relying on browser-only features like window or navigator, or things like animations and measurements that don’t need to match the initial HTML. It helps prevent unnecessary errors while keeping your app running smoothly.
Wrapping It Up – Got Any Thoughts?
So, that’s how I tackled my hydration error. I used suppressHydrationWarning, set it to true, and applied it to the <body>. This tells React to chill out and ignore any mismatches between the server-rendered HTML and what the client ends up rendering.
Now, don’t get me wrong, this doesn’t actually fix the mismatch; it just stops React from throwing warnings about it. It’s useful when you expect slight differences, like styles adapting to user settings or content relying on browser-only features like window or navigator. But if the mismatch is a real issue, this won’t magically solve it, it just keeps things running smoothly without React complaining.
That said, I’m not 100% sure I tackled this problem the best way. Maybe there’s a better approach I haven’t thought of? If you’ve handled hydration errors before or have a different fix, I would love to hear your take! Let’s swap ideas and make this even better. Drop your thoughts in the comments or reach out, I am all ears.
Subscribe to my newsletter
Read articles from Nwakaego-Ego directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
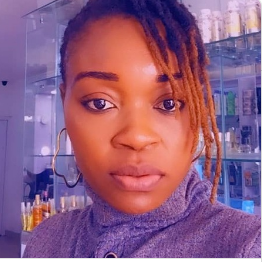
Nwakaego-Ego
Nwakaego-Ego
Writing about my journey to become a React Developer