The Magic Behind JavaScript: How the V8 Engine Works

Table of contents
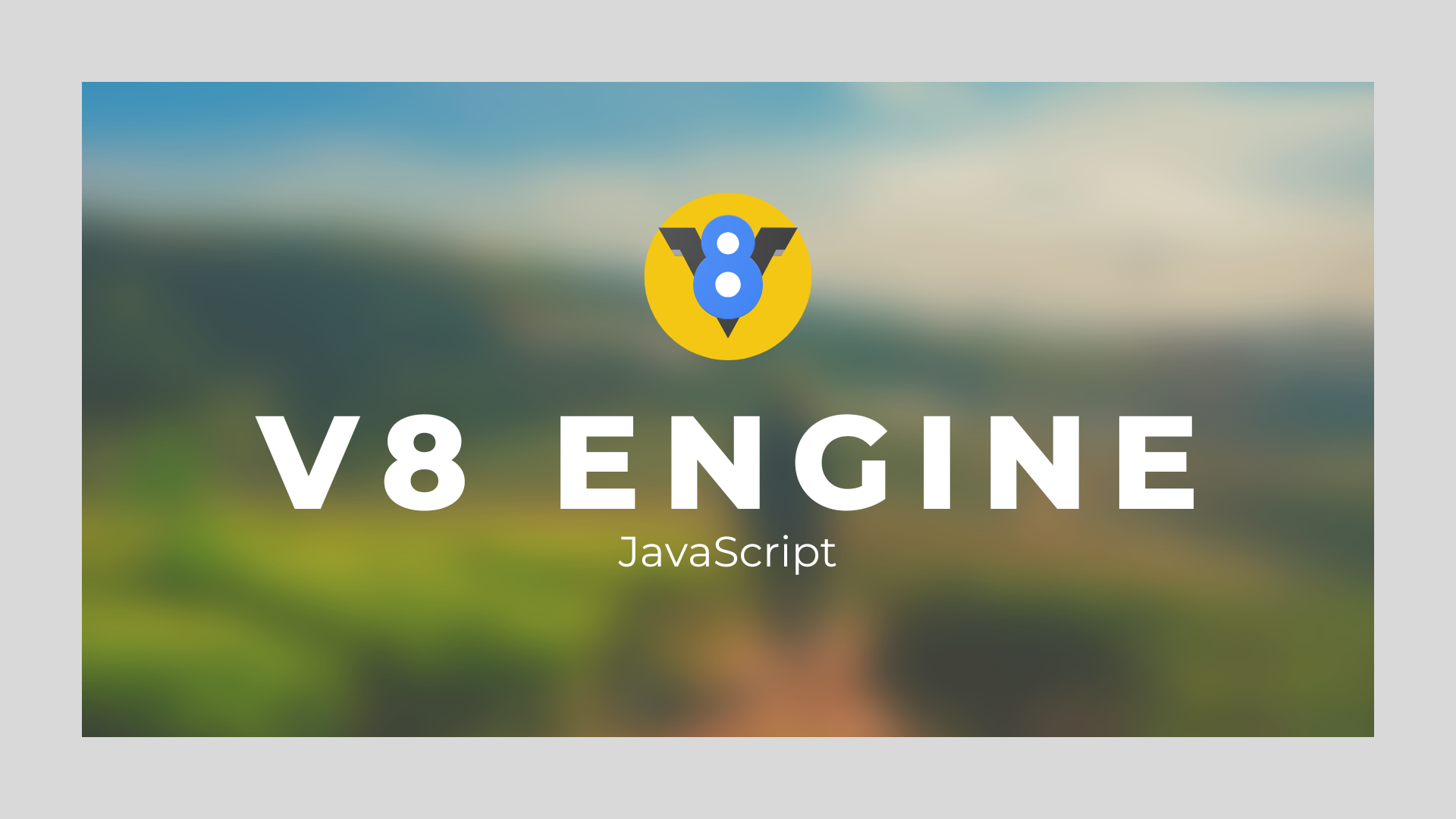
JavaScript Engine - What and Why?
What is a JavaScript Engine?
A JavaScript engine is a program that runs JavaScript code inside a web browser or server. It takes the JavaScript you write and converts it into machine code (which computers understand) so it can be executed quickly and efficiently.
Why Do We Need a JavaScript Engine?
To Run JavaScript Code – Browsers (like Chrome and Firefox) need a way to understand and execute JavaScript.
To Make JavaScript Fast – Engines use optimization techniques to improve speed.
To Handle Modern Web Apps – Today's websites are interactive (like social media, games, and online stores). A strong engine ensures smooth performance.
Each browser uses a Javascript engine. Here are a few of them:
V8 Engine – Used in Google Chrome & Node.js.
Chakra – Used in Internet Explorer
SpiderMonkey – Used in Mozilla Firefox.
JavaScriptCore – Used in Apple Safari.
Compiled or Interpreted?
There are two ways in which a language is translated into a machine-readable form.
An interpreter helps interpret the language line by line.
A compiler helps compile the whole code into machine language and then execute it.
The V8 engine is the JavaScript engine developed by Google that powers Google Chrome and Node.js. It is responsible for compiling and executing JavaScript code.
What is the V8 Engine?
V8 is a high-performance JavaScript engine written in C++
. It converts JavaScript code into machine code (code that computers can understand) instead of interpreting it line by line.
How Does V8 Work?
📍 When you write JavaScript, the browser doesn’t understand it directly.
The V8 engine processes the code in two steps:
Parsing & Tokenizing
Compilation & Execution
Parsing & Tokenizing:
V8 first breaks the code into small pieces (tokens) and converts it into an Abstract Syntax Tree (AST).
Example:
let x = 10;
→ This gets converted into a structured tree for further processing.
Compilation & Execution:
Instead of interpreting the code line by line, V8 uses Just-In-Time (JIT) Compilation.
JIT stands for Just-in-Time Compilation, and it's a technique used by the V8 Engine to make JavaScript faster by combining the best of both interpreters and compilers.
TurboFan (a V8 component) helps optimize the code further.
Key Components of V8
Ignition → The JavaScript Interpreter (converts code into bytecode).
TurboFan → The Compiler (optimizes bytecode and turns it into machine code).
Garbage Collector → Automatically removes unused memory for better performance.
📍 The heart of the V8 engine is Ignition and TurboFan.
How does JavaScript run in V8?
When you write JavaScript:
1️⃣ The V8 engine takes your code.
2️⃣ It compiles it into machine code using JIT compilation.
3️⃣ The event loop and callback queue handle asynchronous tasks.
Why is V8 So Fast?
Just-In-Time (JIT) Compilation – No need to interpret line by line.
Garbage Collection – Removes unused memory efficiently.
Optimized Machine Code – Uses TurboFan to make code run faster.
Conclusion
✅ V8 compiles JavaScript instead of interpreting it line by line.
✅ Uses Ignition (Interpreter) and TurboFan (Compiler) for speed and optimization.
✅ JIT Compilation combines the best of interpretation and compilation.
✅ Garbage Collection helps manage memory efficiently.
✅ V8 is not just for browsers—it also runs JavaScript on servers (Node.js).
Subscribe to my newsletter
Read articles from AaMna AnSari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

AaMna AnSari
AaMna AnSari
A passionate MERN Stack Developer who loves building scalable web applications and exploring new technologies. I have experience with MongoDB, Express.js, React, and Node.js, and I enjoy solving complex problems through code. I also participate in hackathons, work on exciting projects, and share my knowledge through blogs on web development, CSS tricks, JavaScript, React, and backend technologies.