Understanding Memory Allocation, Shallow Copy and Deep Copy in JavaScript

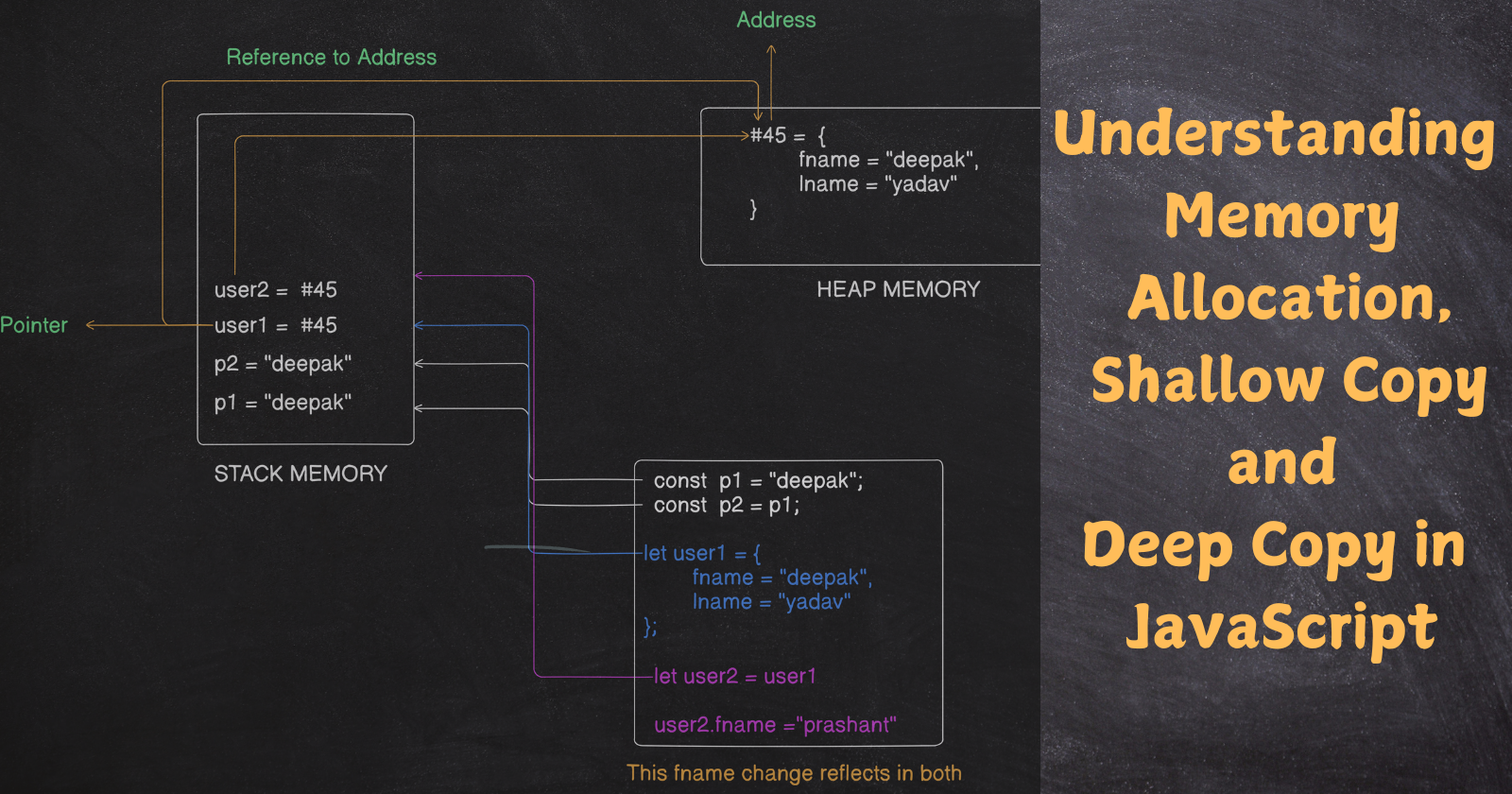
Memory Allocation in JavaScript
JavaScript manages memory differently for primitive and non-primitive data types.
Primitive Data Types (Stored in Stack)
Primitive types include string, number, boolean, null, undefined, symbol, and bigInt. These are stored directly in the stack and when assigned to a new variable, a copy of the value is created.
Example:
let p1 = "Deepak";
let p2 = p1; // A copy of p1 is created
p2 = "Prashant"; // Only p2 is changed
console.log(p1); // Output: Deepak
console.log(p2); // Output: Prashant
Explanation:
Since p1 is a string (primitive type), it is stored in the stack.
p2 gets a copy of p1, so modifying p2 does not affect p1.
Non-Primitive Data Types (Stored in Heap)
Objects, arrays and functions are stored in heap memory and the stack stores a reference (pointer) to the heap location.
✅ Example:
let o1 = {
fname: "Deepak",
lname: "Yadav",
address: {
hNo: 1,
colony: "Shiv Colony",
city: "Gwalior",
pincode: 474005
}
};
let o2 = o1; // Both o1 and o2 point to the same object in heap
o2.fname = "Prashant"; // Changes reflect in o1 as well
console.log(o1);
console.log(o2);
Explanation:
o1 is an object stored in heap memory and o1 itself is a reference stored in stack memory.
o2 = o1 does not create a new object; instead, o2 also points to the same object in heap.
Changing o2.fname also changes o1.fname because both reference the same object in heap.
Arrays (Behave Like Objects in Heap Memory)
Arrays follow the same behavior as objects.
Example:
let arr1 = ["Ironman", "Batman"];
let arr2 = arr1;
arr2[1] = "Thor";
console.log(arr1); // Output: ["Ironman", "Thor"]
console.log(arr2); // Output: ["Ironman", "Thor"]
Explanation:
Both arr1 and arr2 reference the same array in heap memory.
Changing arr2[1] affects arr1 as well.
Shallow Copy vs Deep Copy
Shallow Copy (First-Level Copy, Nested Objects Still Referenced)
A shallow copy copies only the first level of properties. If the object contains nested objects or arrays, the nested parts are still referenced, meaning changes in one will affect the other.
Example (Using Spread Operator ...
):
let o1 = {
fname: "Deepak",
lname: "Yadav",
address: {
hNo: 1,
colony: "Shiv Colony",
city: "Gwalior",
pincode: 474005
}
};
let o2 = { ...o1 }; // Shallow copy
o2.fname = "Prashant"; // This change is independent
o2.address.hNo = 4; // This change affects both o1 and o2
console.log(o1);
console.log(o2);
Issue:
The … spread operator only copies the first level.
o2.address is still referencing the same object in heap, so changing o2.address.hNo also changes o1.address.hNo
Deep Copy (Completely Independent Copy)
A deep copy creates a completely new object in heap memory, ensuring changes do not affect the original object.
Solution: Serialization and Deserialization (JSON Method)
let o1String = JSON.stringify(o1); // Convert object to string (serialization)
let o3 = JSON.parse(o1String); // Convert back to object (deserialization)
o3.fname = "Ducket"; // Independent change
o3.address.city = "England"; // Independent change
console.log(o1);
console.log(o3);
Why This Works:
JSON.stringyfy(o1) converts the object into a string, breaking all references.
JSON.parse(o1String) creates a new object in heap memory.
Now, o3 is completely independent of o1.
Summary Table
Concept | Memory Type | Copy Behavior |
Primitive Types | Stack | Stored directly, copied by value |
Non-Primitive Types | Heap (Stack stores reference) | Copied by reference |
Shallow Copy | Heap | First level copied, nested objects remain referenced |
Deep Copy | Heap | Fully independent copy |
Key Takeaways
Primitive types (string, number, etc.) → Stored in stack, copied by value
Non-primitive types (objects, arrays, functions) → Stored in heap, copied by reference
Shallow Copy ( . . . spread, Object.assign()) → First level copied, nested objects still referenced
Deep Copy (JSON.stringyfy() + JSON.parse()) → Full independent copy
Subscribe to my newsletter
Read articles from deepak yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

deepak yadav
deepak yadav
Full Stack web Developer