Simple Flask API Creation: A Quick Start Guide For Beginners
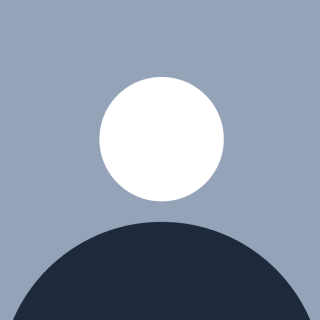
Table of contents
- What is an API?
- The Task
- Solution
- What is a Python Function?
- Prime Number Function
- Perfect Number Function
- Digit Sum Function
- Armstrong Number Function
- Python Libraries
- Import Libraries
- Numbers Fun Fact Function
- Define a Route for the Endpoint
- Classify Number Function
- Start the Flask Web Server
- Running the API on Visual Studio Code
- Running the API on Replit
- Closing Remark
- References
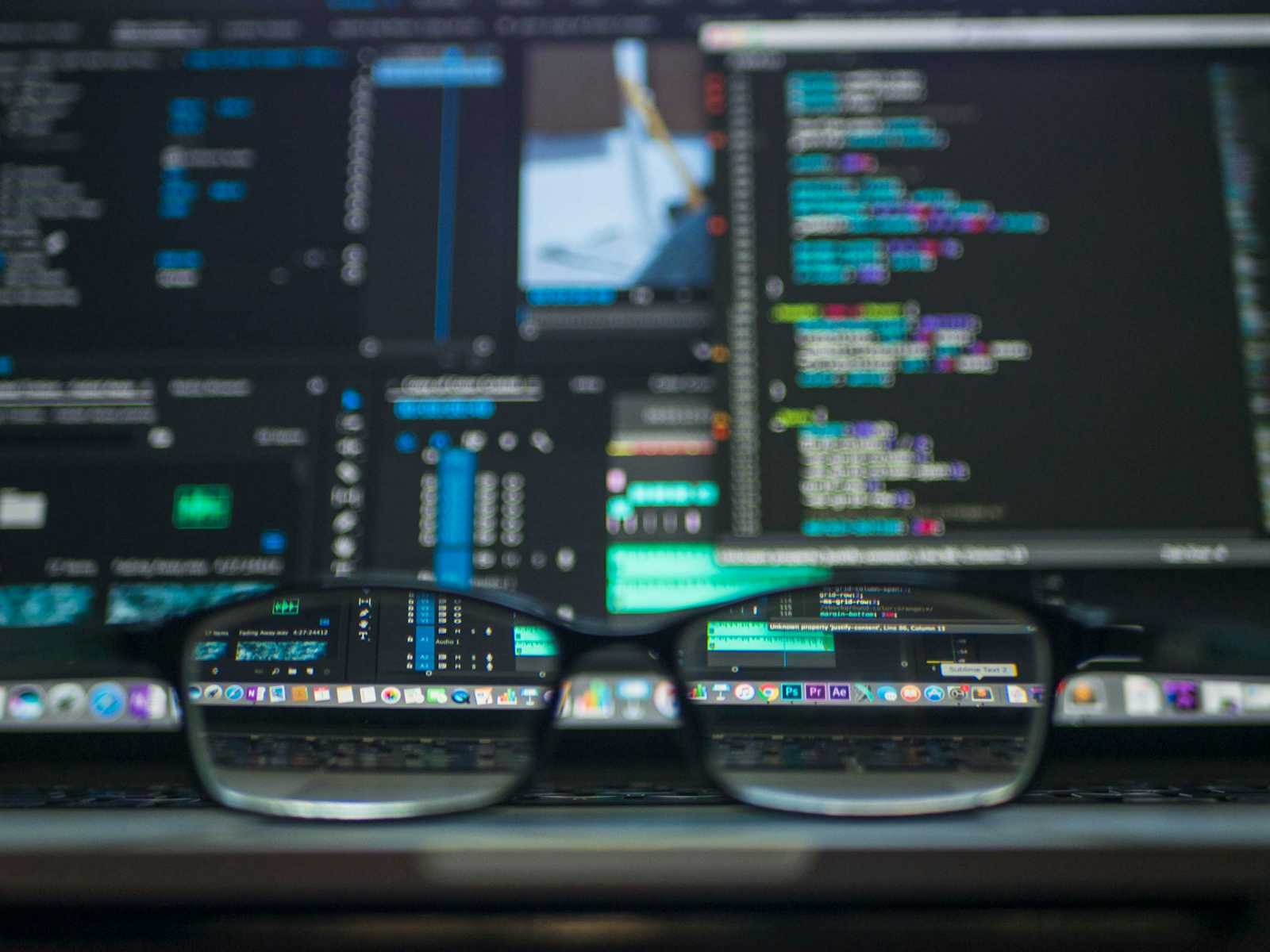
Learn Python with this one project!
This article will introduce you to some fundamental concepts of Python, such as declaring variables, type conversion, mathematical operations, and defining and calling functions. Additionally, it will delve into more advanced topics like error handling, using requests, Flask, and jsonify.
What is an API?
API stands for Application Programming Interface. APIs are mechanisms that enable two software components to communicate with each other using a set of definitions and protocols. An API is a request a client app forwards to a server. It contains operations to be executed (for example, GET to retrieve a resource or POST to send data), authentication details — for example, an API key that identifies the client, additional parameters, and a destination address — the URL of the API endpoint.
The Task
This is an HNG DevOps Stage 1 task. The task is to create an API that takes a number and returns interesting mathematical properties about it, along with a fun fact using this Numbers Link as an API resource.
API Specification
Endpoint:
GET** <
your-domain.com
>/api/classify-number?number=371
Required JSON Response Format (200 OK):
{
"number": 371,
"is_prime": false,
"is_perfect": false,
"properties": ["armstrong", "odd"],
"digit_sum": 11, // sum of its digits
"fun_fact": "371 is an Armstrong number because 3^3 + 7^3 + 1^3 = 371" //gotten from the numbers API
}
- Required JSON Response Format (400 Bad Request)
{
"number": "alphabet",
"error": true
}
Acceptance Criteria
Functionality
Accepts GET requests with a number parameter.
Returns JSON in the specified format.
Accepts all valid integers as the only possible inputs
Provides appropriate HTTP status codes.
Code Quality
Organized code structure.
Basic error handling and input validation.
Avoids hardcoded values.
Solution
The code will be written with an explanation provided for each line used to achieve the task.
Creating an API can be accomplished in various ways but the Flask web application will be used for this task and the API will be hosted locally on a PC using Visual Studio Code. In another article, an outline of the necessary steps taken to make the API publicly accessible will be documented.
According to the task, the API should return the prime number, perfect number, armstrong number, and the sum of the digit of the number(s) entered as a parameter. This can be achieved by defining a few functions to check for each of these properties.
What is a Python Function?
A Python function is a block of reusable code that performs a specific task when called, often accepting inputs (arguments) and returning outputs. The idea is to put some commonly or repeatedly done tasks together and make a function, calling the function to reuse the code contained in it over and over again.
There are two types of python function:
built-in library functions are standard functions that are available to use, eg
print()
,int()
,range()
etc.user-defined functions are functions that programmer creates based on their requirements like the functions later created in this article
Defining a function with arguments allows it to perform tasks using different inputs.
Prime Number Function
A prime number is a whole number with only two factors: itself and 1. A few notable prime numbers are 2, 3, 5, 7, etc. These numbers can only be divisible by themselves and 1.
To check for a prime number, we need to ensure that the numbers that can only divide them without a remainder are itself and 1.
Let’s define a function that takes an argument number
.
def is_prime(number):
# Prime numbers must be greater than 1
if number <= 1:
return False
# Check for factors from 2 to the square root of the number
for i in range(2, int(number**0.5) + 1):
if number % i == 0:
return False
return True
The first two lines after the defined function checks if the argument number
is less than or equal to 1 and returns False
if it is.
The for
loop iterates from the range
of 2 to the square root of the number that can divide the number without leaving a remainder. If it finds any such number, it means the original number can be divided by something other than 1 and itself, so it returns False
(not a prime).
return True
returns True
if the function doesn't find any numbers that can divide the original number without leaving a remainder.
Perfect Number Function
A perfect number is a positive integer equal to the sum of its positive divisors, excluding the number itself. Let’s take 6 for example, all the numbers that can divide 6 evenly are 1, 2 and 3. If we add 1, 2 and 3 together, we get the number 6.
To check for a perfect number in Python, we have to ensure that when we add the divisors of the number, the sum is equal to the number itself.
Let’s define a function that takes an argument number
:
def is_perfect(number):
if number < 1:
return False
perfect = sum ([num for num in range(1, number) if number % num == 0])
if perfect == number:
return True
else:
return False
The first block of codes after the defined function checks if the argument number
is less than 1, and returns False
when it does.
The next line of code perfect = sum ([num for num in range(1, number) if number % num == 0])
is a list comprehension that creates a list of numbers that meet the specified condition. What this list comprehension does is that, with range(1, number)
it generates a list of numbers from the range 1 to the number - 1 (so if your number is 6, the range will be from 1 to 5). It uses a condition if number % num == 0
which means that for each num
in the range, the condition checks if number
is divisible by num
without leaving a remainder. The sum function sum([…])
then calculates the sum of all the numbers that satisfy the condition in the list comprehension and then saves it in a perfect variable.
The condition block checks if the variable perfect
equals the argument number
. If it does, it will return True, if it doesn’t, it will return False.
Digit Sum Function
This is the sum of each digit in the number. So if there are 2 digits like 12, the sum of 1 and 2 will be 3 or if there are 3 digits like 317, the sum of 3, 1, and 7 will be 11.
Let’s define a function that takes in an argument number
.
def digit_sum(number):
# Convert the number to its absolute integer value and then to a string
number = str(abs(int(number)))
# Initialize the sum to 0
total_sum = 0
# Iterate over each digit in the string representation of the number
for digit in number:
# Convert the digit back to an integer and add it to the total sum
total_sum += int(digit)
return total_sum
number = str(abs(int(number)))
converts the number
to integer with int(number)
first and then to its absolute number, abs(int(number))
, which means that if the number is negative, it makes it positive. Then it converts the number to a string str(abs(int(number)))
and stores it in a variable number
.
total_sum = 0
initializes a variable total_sum
and sets it as 0.
The for loop iterates over each digit in the number, converts each digit to an integer int(digit)
, and then, adds it to the total sum. The return total_sum
returns the final value of the addition.
Armstrong Number Function
An Armstrong number is a number that is equal to the sum of its digits each raised to the power of the number of digits. For example, 371 is an Armstrong number because 3**3 + 7**3 + 1**3 equals 371.
Let’s define a function that takes in an argument number
:
def is_armstrong(number):
# Convert the number to its absolute integer value and then to a string
num_str = str(abs(int(number)))
len_num = len(num_str)
# Initialize the sum to 0
total_sum = 0
# Iterate over each digit in the string representation of the number
for digit in num_str:
# Convert the digit to an integer and raise it to the power of num, then add it to the total sum
total_sum += int(digit) ** len_num
# Check if the total sum is equal to the absolute value of the original number
return total_sum == abs(number)
number = str(abs(int(number)))
converts the number
to integer with int(number
) and then to its absolute number, abs(int(number))
, which means that if the number is negative, it makes it positive. Then it converts the number to a string str(abs(int(number)))
and stores it in a variable num_str
.
len_num = len(num_str)
returns the number of characters in the string num_str
and stores it in a variable len_num
.
total_sum = 0
initializes a variable total_sum
and sets it as 0.
The for loop iterates over each digit in the number and converts each digit to an integer, int(digit)
, multiplies it raised to the power of len_num
, and then, adds the total value to the total sum.
return total_sum == abs(number)
checks if the total_sum
equals the absolute number abs(number)
. If they are equal to each other, the function returns True
, if they are not, the function returns False
.
Python Libraries
Libraries in Python are collections of modules and packages that provide pre-written code to perform various tasks. a few Python libraries include Numpy, Django, Flask, math etc.
The Python libraries used in creating this API are requests and Flask.
Import Libraries
In order to use libraries in your program, it needs to be imported first. Therefore, the requests
library, along with some modules in the flask
library like Flask
, request
and jsonify
will be imported.
Placing imports at the top of the program makes it easy to see which external libraries or modules are being used in the program. Python scripts are executed from top to bottom. By importing libraries at the top, we ensure all necessary dependencies are available before any other code is executed.
You would have to install the libraries if they have not already been installed. On your terminal, type pip install requests
and pip install flask
to install the needed libraries.
import requests
from flask import Flask, request, jsonify
app = Flask(__name__)
import requests
imports the requests library while from flask import Flask, request, jsonify
imports the Flask, request, and jsonify modules.
Numbers Fun Fact Function
This function will use a library called requests to access an API resource from the Numbers website. The function will take an argument number
:
def fun_fact(number):
# makes a GET request to the numbers API, returns an error message if status code does not return 200
response = requests.get(f"http://numbersapi.com/{number}/math?json")
response.raise_for_status()
fun_fact = response.json()
# extracts the text content of the response
return fun_fact.get("text")
response = requests.get(f”http://numbersapi.com/{number}/math?json”)
constructs a URL using the number provided as an argument and sends a GET request to the Numbers API where number
is replaced with the number parameter provided. The request.get()
function sends the GET request and stores the response in the variable response
.
response.raise_for_status()
function checks if the GET request was successful. If the status code of the response is anything other than 200 (which indicates success), it raises an HTTPError exception which helps handle potential errors in the request.
fun_fact = response.json()
parses the JSON content of the response and stores it in the variable fun_fact
. The response.json()
method converts the JSON response into a Python dictionary.
return fun_fact.get(“text")
extracts the value associated with the key "text"
from the fun_fact
dictionary and returns it. This is a fun fact about the number that was retrieved from the Numbers API.
Define a Route for the Endpoint
An API endpoint is a URL that acts as the point of contact between an API client and an API server.
We have to define a route for the endpoint which will handle GET requests. This can be achieved with this line of code:
@app.route("/api/classify-number", methods=["GET"])
@app.route()
is a decorator provided by the Flask web framework. A decorator in Python is a special function that modifies the behavior of another function. It tells the app what URL should trigger a special function. "/api/classify-number"
is the URL endpoint for the route. When a user visits the URL, the associated function will be executed. methods=["GET"]
specifies the HTTP method(s) that this route will respond to. In this case, it is set to GET
requests.
When you use @app.route("/api/classify-number", methods=["GET"])
, you're telling your Flask application to listen for GET requests made to the /api/classify-number
URL. When such a request is received, the function immediately following this decorator will be executed to handle the request.
Classify Number Function
After defining a route for our endpoint, we have to define a function that will be executed to handle the request. This function will not take in any argument.
def classify_number():
# allows users to pass a number as parameter when making a request to the /classify-number endpoint
number = request.args.get("number")
number = request.args.get(“number”)
:
request
is an object provided by Flask. It contains all the data related to the incoming request (like data sent by a client)..args
is a dictionary-like attribute of therequest
object that contains all the query parameters sent in the URL..get(“number”)
is a method call that retrieves the value associated with the key“number”
from therequest.args
dictionary. If the key"number”
is not found, it returns None by default.
Therefore, number = request.args.get(“number”)
gets the value of the "number"
query parameter from the incoming request URL and assigns it to the variable number
.
# returns a json response if the number parameter is not provided
if not(number):
return jsonify({"error": "no number provided"}), 400
if not(number): return jsonify({"error": "no number provided"}), 400
:
- If the
number
variable is empty orNone
, the code returns a JSON response with an error message"error": "no number provided"
and a status code400
. This status code means "Bad Request" and indicates that the server cannot process the request due to a client error (in this case, the absence of a number). This helps inform the client that they need to provide a valid number in their request.
try:
number = int(number)
except ValueError:
return jsonify({"error": "invalid number"}), 400
The try-except block is used to wrap code that might raise an exception. If an error occurs within the try
block, the code execution jumps to the corresponding except
block.
number = int(number)
attempts to convert the variable number
into an integer.
except ValueError:
catches the ValueError
exception. A ValueError
occurs if the int()
function fails to convert the string to an integer.
return jsonify({"error": "invalid number"}), 400
: If a ValueError
is raised, this line will be executed. It returns a JSON response with an error message and sets the HTTP status code to 400
which means Bad Request.
properties = []
if is_armstrong(number):
properties.append("armstrong")
properties.append("even" if number % 2 == 0 else "odd")
properties = []
, initializes an empty list.
Up until now, we have been defining functions, with this if
block of code, we will be calling the is_armstrong()
function with a number
argument and use the result to update our properties
list with properties.append(“armstrong”)
.
The if
block checks if the is_armstrong(number)
function returns True
and then updates the properties
list with “armstrong”
if it does.
properties.append("even" if number % 2 == 0 else "odd")
, appends the properties
list after doing some calculations. "even" if number % 2 == 0 else "odd"
: this checks if the number is divisible by 2, and updates the properties
list with “even”
if it is and “odd”
if it’s not.
# returns a json response containing the result of the functions on the number
try:
output = {
"number": number,
"is_prime": is_prime(number),
"is_perfect": is_perfect(number),
"digit_sum": digit_sum(number),
"fun_fact": fun_fact(number),
"properties": properties
}
return jsonify(output), 200
except Exception as e:
return jsonify({
"number": "alphabet",
"error": True
}), 400
This try-except block returns the result from the functions earlier declared in a dictionary format called output
.
The output
dictionary contains various pieces of information about the number
:
"number": number
: This is the original number that is input as a parameter in the URL."is_prime": is_prime(number)
: returns the result of theis_prime
function onnumber
."is_perfect": is_perfect(number)
: returns the result of theis_perfect
function onnumber
."digit_sum": digit_sum(number)
: returns the result of thedigit_sum
function onnumber
."fun_fact": fun_fact(number)
: retrieves the result of thenumber
gotten from the JSON in thefun_fact
function."properties": properties
: retrieves the content of theproperties
list (such as "even" or "odd" or “armstrong”) that describes the number.
The jsonify(output)
function converts the output
dictionary into a JSON response. The 200
is HTTP status code for "OK," indicating that the request was successful.
The except block, except Exception as e
, will handle any error that occurs in the execution of the try
block. It will return a JSON response with an error message to indicate an error occurred. The 400
is HTTP status code for "Bad Request," indicating that the server could not understand the request due to invalid input.
Putting the function classify_number()
together:
def classify_number():
# allows users to pass a number as parameter when making a request to the /classify-number endpoint
number = request.args.get("number")
# returns a json response if the number parameter is not provided
if not(number):
return jsonify({"error": "no number provided"}), 400
# if number conversion to integer fails, returns a json response with an error message
try:
number = int(number)
except ValueError:
return jsonify({"error": "invalid number"}), 400
properties = []
if is_armstrong(number):
properties.append("armstrong")
properties.append("even" if number % 2 == 0 else "odd")
# returns a json response containing the result of the functions on the number
try:
output = {
"number": number,
"is_prime": is_prime(number),
"is_perfect": is_perfect(number),
"digit_sum": digit_sum(number),
"fun_fact": fun_fact(number),
"properties": properties
}
return jsonify(output), 200
except Exception as e:
return jsonify({
"number": "alphabet",
"error": True
}), 400
Start the Flask Web Server
The block of code if __name__ == '__main__':
needs to be at the end of the script because it ensures the Flask application runs only when the script is executed directly, not when it is imported as a module in another script. It is usually located at the end of the program to ensure all routes and functions are defined before starting the server.
if __name__ == '__main__':
app.run(debug=True)
if __name__ == '__main__':
checks if the script is being run directly (not imported as a module in another script). __name__
is a special built-in variable that gets the value "__main__"
when the script is executed directly. If the script is imported into another module, __name__
will be set to the module's name, not "__main__"
.
app.run()
is a method in Flask that starts the web server. The debug
parameter controls whether to run the server in debug mode. Since debug=True
, the Flask web server will run in debug mode and will show a detailed error message in the web browser (if there is an error), automatically restarts your server when it notices a change in your code, and enables an interactive debugger which is useful for diagnosing issues.
This is what the full program code looks like:
# this is an api that delivers fun facts about mathematical numbers
import requests
from flask import Flask, request, jsonify
# creates a Flask application instance to determine the root path of the application
app = Flask(__name__)
# checking for prime number using sympy library
def is_prime(number):
# Prime numbers must be greater than 1
if number <= 1:
return False
# Check for factors from 2 to the square root of the number
for i in range(2, int(number**0.5) + 1):
if number % i == 0:
return False
return True
# checking if number is a perfect number
def is_perfect(number):
if number < 1:
return False
perfect = sum ([num for num in range(1, number) if number % num == 0])
if perfect == number:
return True
else:
return False
# adds the numbers together individually
def digit_sum(number):
# Convert the number to its absolute integer value and then to a string
number = str(abs(int(number)))
# Initialize the sum to 0
total_sum = 0
# Iterate over each digit in the string representation of the number
for digit in number:
# Convert the digit back to an integer and add it to the total sum
total_sum += int(digit)
return total_sum
# checking if the number is an armstrong number
def is_armstrong(number):
# Convert the number to its absolute integer value and then to a string
num_str = str(abs(int(number)))
len_num = len(num_str)
# Initialize the sum to 0
total_sum = 0
# Iterate over each digit in the string representation of the number
for digit in num_str:
# Convert the digit to an integer and raise it to the power of num, then add it to the total sum
total_sum += int(digit) ** len_num
# Check if the total sum is equal to the absolute value of the original number
return total_sum == abs(number)
def fun_fact(number):
# makes a GET request to the numbers API, returns an error message if status code does not return 200
response = requests.get(f"http://numbersapi.com/{number}/math?json")
response.raise_for_status()
fun_fact = response.json()
# extracts the text content of the response
return fun_fact.get("text")
# defines a route for the endpoint which will handle GET requests
@app.route("/api/classify-number", methods=["GET"])
def classify_number():
# allows users to pass a number as parameter when making a request to the /number-info endpoint
number = request.args.get("number")
# returns a json response if the number parameter is not provided
if not(number):
return jsonify({"error": "no number provided"}), 400
# if number conversion to integer fails, returns a json response with an error message
try:
number = int(number)
except ValueError:
return jsonify({"error": "invalid number"}), 400
properties = []
if is_armstrong(number):
properties.append("armstrong")
properties.append("even" if number % 2 == 0 else "odd")
# returns a json response containing the result of the functions on the number
try:
output = {
"number": number,
"is_prime": is_prime(number),
"is_perfect": is_perfect(number),
"digit_sum": digit_sum(number),
"fun_fact": fun_fact(number),
"properties": properties
}
return jsonify(output), 200
except Exception as e:
return jsonify({
"number": "alphabet",
"error": True
}), 400
if __name__ == '__main__':
app.run(debug=True)
Running the API on Visual Studio Code
If you already have an IDE (Integrated Development Environment) for running Python codes, for example, Visual Studio Code, you will have to save it and then on the terminal, run the code using python <program_name>.py.
You should see this on your terminal:
* Serving Flask app 'api' # (“api” is my program name)
*Debug mode: on
WARNING: This is a development server. Do not use it in a production deployment. Use a production WSGI server instead.
*Running on http://127.0.0.1:5000
Press CTRL+C to quit
*Restarting with stat
*Debugger is active!
*Debugger PIN: 115-507-333
Now go to a new browser tab and type in http://127.0.0.1:5000/api/classify-number?number=371 on the URL. You should get a JSON response with:
{
"digit_sum": 11,
"fun_fact": "371 is a narcissistic number.",
"is_perfect": false,
"is_prime": false,
"number": 371,
"properties": [
"armstrong",
"odd"
]
}
You can change the number at the end of the URL to any number to see an interesting fact about the number. It works with negative numbers, valid integers and floating whole numbers. You can also input a letter, floating-point numbers and even omit the number to see the error message displayed.
While doing these, if you check your terminal, you can see the resulting GET requests like:
Each line shows a GET request was made with URL: /api/classify-number?. The last number 400 status code for the first 3 lines shows there was a bad request and there was an error in validating the number. The last 3 lines show the 200 status code which indicates the HTTP request was successful. On your keyboard, Press CTRL+C to stop the program when you are done.
Running the API on Replit
Replit is a web-based IDE that can be used with a variety of programming languages. It can be used to write, run, and share code.
You will need an account with Replit to run the code. Create a Python file and paste the code. Then click “Run” at the top of the page. On the web URL, paste /api/classify-number?number=371 and press enter. You should see the resulting JSON response.
Closing Remark
This project covers a few Python functions, libraries, mathematical operations, and a simple web app. This project is aimed at beginners and those who would like to refresh their memory on some Python basics.
References
https://docs.python.org/3/library/index.html
https://www.altexsoft.com/blog/what-is-api-definition-types-specifications-documentation/
https://www.geeksforgeeks.org/python-functions/
https://github.com/omiete01/numbers_api/blob/main/numbers_api.py
Subscribe to my newsletter
Read articles from Omiete directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by