Mastering Sorting Algorithms in Python

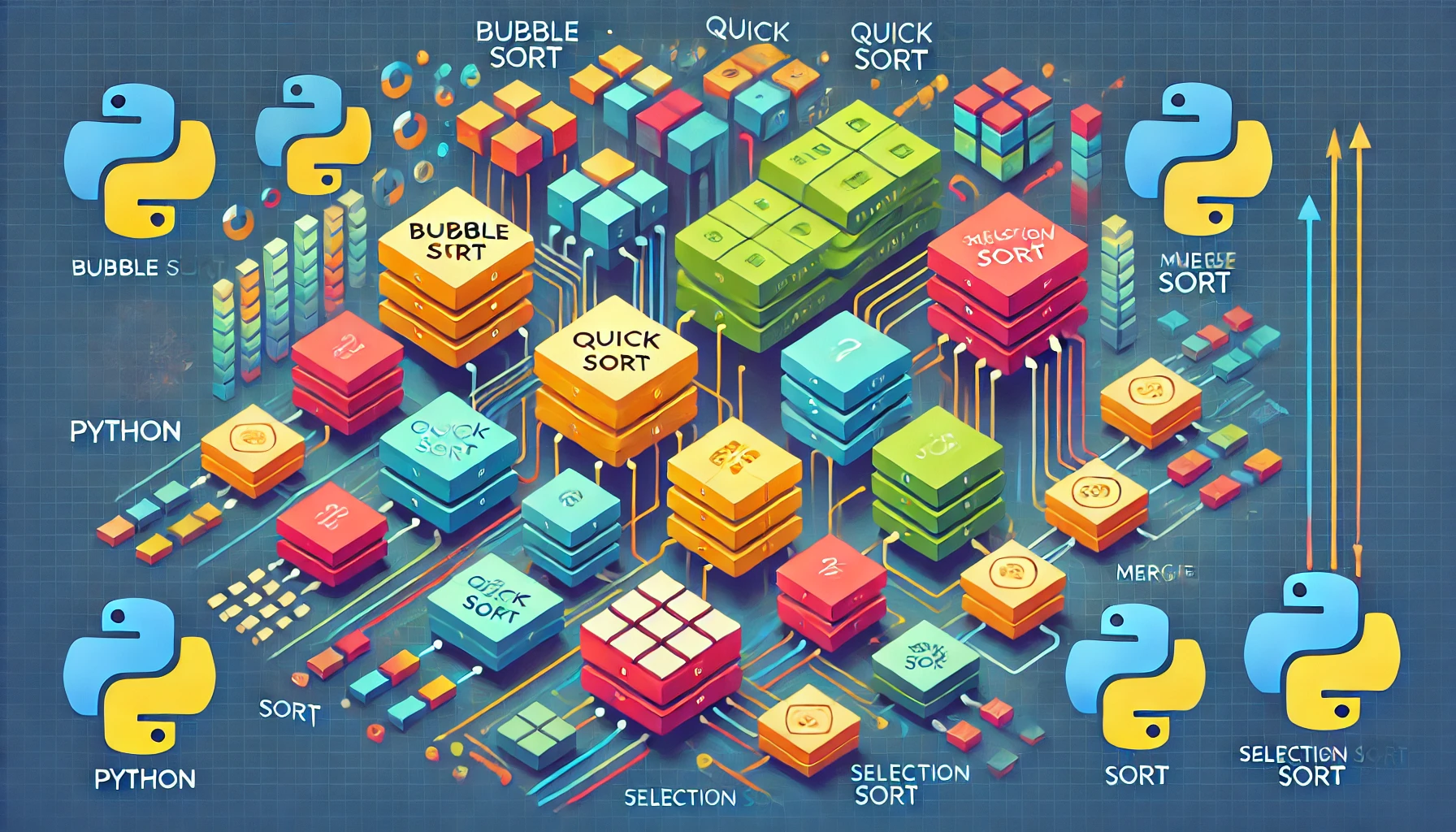
Sorting is one of the most fundamental operations in computer science. Whether it's organizing data for better readability or optimizing search algorithms, sorting plays a crucial role in efficient computing. Python provides multiple sorting algorithms, each with its unique advantages and use cases. In this blog, we will explore different sorting algorithms, their complexities, and their implementations in Python.
Why Sorting Matters?
Sorting is essential for various applications such as:
Efficient searching (e.g., Binary Search requires sorted arrays)
Data organization and visualization
Improving computational efficiency in large datasets
Preparing data for further analysis in machine learning
Understanding different sorting algorithms allows developers to choose the best one based on time complexity, space constraints, and dataset characteristics.
Types of Sorting Algorithms
Sorting algorithms are mainly categorized into two types:
Comparison-based Sorting Algorithms: These involve comparing elements to determine their order (e.g., Bubble Sort, Merge Sort, Quick Sort).
Non-comparison-based Sorting Algorithms: These use different techniques like counting or hashing to sort data (e.g., Counting Sort, Radix Sort, Bucket Sort).
Common Sorting Algorithms in Python
Let's explore some of the most commonly used sorting algorithms with their implementations and performance analysis.
1. Bubble Sort
Bubble Sort repeatedly swaps adjacent elements if they are in the wrong order. It is simple but inefficient for large datasets.
Implementation:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
arr = [64, 25, 12, 22, 11]
print(bubble_sort(arr))
Time Complexity:
Best Case: O(n) (Already sorted list)
Average Case: O(n²)
Worst Case: O(n²)
2. Selection Sort
Selection Sort repeatedly finds the minimum element and places it at the beginning.
Implementation:
def selection_sort(arr):
n = len(arr)
for i in range(n):
min_idx = i
for j in range(i+1, n):
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
return arr
# Example usage
arr = [64, 25, 12, 22, 11]
print(selection_sort(arr))
Time Complexity:
Best Case: O(n²)
Average Case: O(n²)
Worst Case: O(n²)
3. Insertion Sort
Insertion Sort builds the sorted array one item at a time.
Implementation:
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
return arr
# Example usage
arr = [64, 25, 12, 22, 11]
print(insertion_sort(arr))
Time Complexity:
Best Case: O(n) (Nearly sorted list)
Average Case: O(n²)
Worst Case: O(n²)
4. Merge Sort
Merge Sort is a divide-and-conquer algorithm that recursively divides the array and merges sorted subarrays.
Implementation:
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
left = arr[:mid]
right = arr[mid:]
merge_sort(left)
merge_sort(right)
i = j = k = 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
arr[k] = left[i]
i += 1
else:
arr[k] = right[j]
j += 1
k += 1
while i < len(left):
arr[k] = left[i]
i += 1
k += 1
while j < len(right):
arr[k] = right[j]
j += 1
k += 1
return arr
# Example usage
arr = [64, 25, 12, 22, 11]
print(merge_sort(arr))
Time Complexity:
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
5. Quick Sort
Quick Sort uses a pivot element to partition the array and recursively sorts the subarrays.
Implementation:
def quick_sort(arr):
if len(arr) <= 1:
return arr
pivot = arr[len(arr) // 2]
left = [x for x in arr if x < pivot]
middle = [x for x in arr if x == pivot]
right = [x for x in arr if x > pivot]
return quick_sort(left) + middle + quick_sort(right)
# Example usage
arr = [64, 25, 12, 22, 11]
print(quick_sort(arr))
Time Complexity:
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n²) (Unbalanced partitioning)
Choosing the Right Sorting Algorithm
For small datasets: Insertion Sort or Selection Sort can work efficiently.
For large datasets: Merge Sort and Quick Sort are preferred due to their O(n log n) complexity.
For nearly sorted arrays: Insertion Sort performs well.
For stability requirements: Merge Sort is stable, whereas Quick Sort is not always stable.
Python’s built-in sorted()
function and list.sort()
method use Timsort, which is a hybrid sorting algorithm combining Merge Sort and Insertion Sort.
By understanding these algorithms, you can make informed choices for optimizing performance in your applications. Happy coding!
Subscribe to my newsletter
Read articles from Raj Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
